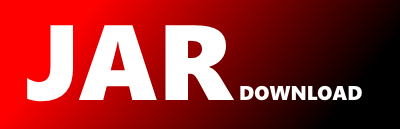
com.azure.resourcemanager.advisor.models.Recommendations Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-advisor Show documentation
Show all versions of azure-resourcemanager-advisor Show documentation
This package contains Microsoft Azure SDK for Advisor Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. REST APIs for Azure Advisor. Package tag package-2020-01.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.advisor.models;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.util.Context;
import java.util.UUID;
/**
* Resource collection API of Recommendations.
*/
public interface Recommendations {
/**
* Initiates the recommendation generation or computation process for a subscription. This operation is
* asynchronous. The generated recommendations are stored in a cache in the Advisor service.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response.
*/
RecommendationsGenerateResponse generateWithResponse(Context context);
/**
* Initiates the recommendation generation or computation process for a subscription. This operation is
* asynchronous. The generated recommendations are stored in a cache in the Advisor service.
*
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void generate();
/**
* Retrieves the status of the recommendation computation or generation process. Invoke this API after calling the
* generation recommendation. The URI of this API is returned in the Location field of the response header.
*
* @param operationId The operation ID, which can be found from the Location field in the generate recommendation
* response header.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response}.
*/
Response getGenerateStatusWithResponse(UUID operationId, Context context);
/**
* Retrieves the status of the recommendation computation or generation process. Invoke this API after calling the
* generation recommendation. The URI of this API is returned in the Location field of the response header.
*
* @param operationId The operation ID, which can be found from the Location field in the generate recommendation
* response header.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
void getGenerateStatus(UUID operationId);
/**
* Obtains cached recommendations for a subscription. The recommendations are generated or computed by invoking
* generateRecommendations.
*
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the list of Advisor recommendations as paginated response with {@link PagedIterable}.
*/
PagedIterable list();
/**
* Obtains cached recommendations for a subscription. The recommendations are generated or computed by invoking
* generateRecommendations.
*
* @param filter The filter to apply to the recommendations.<br>Filter can be applied to properties
* ['ResourceId', 'ResourceGroup', 'RecommendationTypeGuid', '[Category](#category)'] with operators ['eq', 'and',
* 'or'].<br>Example:<br>- $filter=Category eq 'Cost' and ResourceGroup eq 'MyResourceGroup'.
* @param top The number of recommendations per page if a paged version of this API is being used.
* @param skipToken The page-continuation token to use with a paged version of this API.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the list of Advisor recommendations as paginated response with {@link PagedIterable}.
*/
PagedIterable list(String filter, Integer top, String skipToken, Context context);
/**
* Obtains details of a cached recommendation.
*
* @param resourceUri The fully qualified Azure Resource Manager identifier of the resource to which the
* recommendation applies.
* @param recommendationId The recommendation ID.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return advisor Recommendation along with {@link Response}.
*/
Response getWithResponse(String resourceUri, String recommendationId, Context context);
/**
* Obtains details of a cached recommendation.
*
* @param resourceUri The fully qualified Azure Resource Manager identifier of the resource to which the
* recommendation applies.
* @param recommendationId The recommendation ID.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return advisor Recommendation.
*/
ResourceRecommendationBase get(String resourceUri, String recommendationId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy