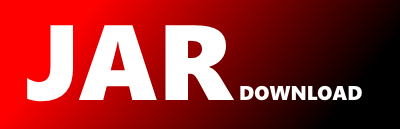
com.azure.resourcemanager.apimanagement.implementation.IssueContractImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-apimanagement Show documentation
Show all versions of azure-resourcemanager-apimanagement Show documentation
This package contains Microsoft Azure SDK for ApiManagement Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. ApiManagement Client. Package tag package-2022-08.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.apimanagement.implementation;
import com.azure.core.util.Context;
import com.azure.resourcemanager.apimanagement.fluent.models.IssueContractInner;
import com.azure.resourcemanager.apimanagement.models.IssueContract;
import com.azure.resourcemanager.apimanagement.models.IssueUpdateContract;
import com.azure.resourcemanager.apimanagement.models.State;
import java.time.OffsetDateTime;
public final class IssueContractImpl implements IssueContract, IssueContract.Definition, IssueContract.Update {
private IssueContractInner innerObject;
private final com.azure.resourcemanager.apimanagement.ApiManagementManager serviceManager;
public String id() {
return this.innerModel().id();
}
public String name() {
return this.innerModel().name();
}
public String type() {
return this.innerModel().type();
}
public String title() {
return this.innerModel().title();
}
public String description() {
return this.innerModel().description();
}
public String userId() {
return this.innerModel().userId();
}
public OffsetDateTime createdDate() {
return this.innerModel().createdDate();
}
public State state() {
return this.innerModel().state();
}
public String apiId() {
return this.innerModel().apiId();
}
public String resourceGroupName() {
return resourceGroupName;
}
public IssueContractInner innerModel() {
return this.innerObject;
}
private com.azure.resourcemanager.apimanagement.ApiManagementManager manager() {
return this.serviceManager;
}
private String resourceGroupName;
private String serviceName;
private String apiId;
private String issueId;
private String createIfMatch;
private String updateIfMatch;
private IssueUpdateContract updateParameters;
public IssueContractImpl withExistingApi(String resourceGroupName, String serviceName, String apiId) {
this.resourceGroupName = resourceGroupName;
this.serviceName = serviceName;
this.apiId = apiId;
return this;
}
public IssueContract create() {
this.innerObject = serviceManager.serviceClient()
.getApiIssues()
.createOrUpdateWithResponse(resourceGroupName, serviceName, apiId, issueId, this.innerModel(),
createIfMatch, Context.NONE)
.getValue();
return this;
}
public IssueContract create(Context context) {
this.innerObject = serviceManager.serviceClient()
.getApiIssues()
.createOrUpdateWithResponse(resourceGroupName, serviceName, apiId, issueId, this.innerModel(),
createIfMatch, context)
.getValue();
return this;
}
IssueContractImpl(String name, com.azure.resourcemanager.apimanagement.ApiManagementManager serviceManager) {
this.innerObject = new IssueContractInner();
this.serviceManager = serviceManager;
this.issueId = name;
this.createIfMatch = null;
}
public IssueContractImpl update() {
this.updateIfMatch = null;
this.updateParameters = new IssueUpdateContract();
return this;
}
public IssueContract apply() {
this.innerObject = serviceManager.serviceClient()
.getApiIssues()
.updateWithResponse(resourceGroupName, serviceName, apiId, issueId, updateIfMatch, updateParameters,
Context.NONE)
.getValue();
return this;
}
public IssueContract apply(Context context) {
this.innerObject = serviceManager.serviceClient()
.getApiIssues()
.updateWithResponse(resourceGroupName, serviceName, apiId, issueId, updateIfMatch, updateParameters,
context)
.getValue();
return this;
}
IssueContractImpl(IssueContractInner innerObject,
com.azure.resourcemanager.apimanagement.ApiManagementManager serviceManager) {
this.innerObject = innerObject;
this.serviceManager = serviceManager;
this.resourceGroupName = ResourceManagerUtils.getValueFromIdByName(innerObject.id(), "resourceGroups");
this.serviceName = ResourceManagerUtils.getValueFromIdByName(innerObject.id(), "service");
this.apiId = ResourceManagerUtils.getValueFromIdByName(innerObject.id(), "apis");
this.issueId = ResourceManagerUtils.getValueFromIdByName(innerObject.id(), "issues");
}
public IssueContract refresh() {
Boolean localExpandCommentsAttachments = null;
this.innerObject = serviceManager.serviceClient()
.getApiIssues()
.getWithResponse(resourceGroupName, serviceName, apiId, issueId, localExpandCommentsAttachments,
Context.NONE)
.getValue();
return this;
}
public IssueContract refresh(Context context) {
Boolean localExpandCommentsAttachments = null;
this.innerObject = serviceManager.serviceClient()
.getApiIssues()
.getWithResponse(resourceGroupName, serviceName, apiId, issueId, localExpandCommentsAttachments, context)
.getValue();
return this;
}
public IssueContractImpl withTitle(String title) {
if (isInCreateMode()) {
this.innerModel().withTitle(title);
return this;
} else {
this.updateParameters.withTitle(title);
return this;
}
}
public IssueContractImpl withDescription(String description) {
if (isInCreateMode()) {
this.innerModel().withDescription(description);
return this;
} else {
this.updateParameters.withDescription(description);
return this;
}
}
public IssueContractImpl withUserId(String userId) {
if (isInCreateMode()) {
this.innerModel().withUserId(userId);
return this;
} else {
this.updateParameters.withUserId(userId);
return this;
}
}
public IssueContractImpl withCreatedDate(OffsetDateTime createdDate) {
if (isInCreateMode()) {
this.innerModel().withCreatedDate(createdDate);
return this;
} else {
this.updateParameters.withCreatedDate(createdDate);
return this;
}
}
public IssueContractImpl withState(State state) {
if (isInCreateMode()) {
this.innerModel().withState(state);
return this;
} else {
this.updateParameters.withState(state);
return this;
}
}
public IssueContractImpl withApiId(String apiId) {
if (isInCreateMode()) {
this.innerModel().withApiId(apiId);
return this;
} else {
this.updateParameters.withApiId(apiId);
return this;
}
}
public IssueContractImpl withIfMatch(String ifMatch) {
if (isInCreateMode()) {
this.createIfMatch = ifMatch;
return this;
} else {
this.updateIfMatch = ifMatch;
return this;
}
}
private boolean isInCreateMode() {
return this.innerModel().id() == null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy