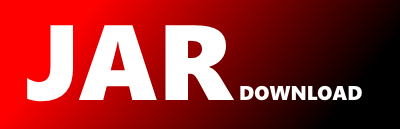
com.azure.resourcemanager.apimanagement.models.AdditionalLocation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-apimanagement Show documentation
Show all versions of azure-resourcemanager-apimanagement Show documentation
This package contains Microsoft Azure SDK for ApiManagement Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. ApiManagement Client. Package tag package-2022-08.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.apimanagement.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* Description of an additional API Management resource location.
*/
@Fluent
public final class AdditionalLocation implements JsonSerializable {
/*
* The location name of the additional region among Azure Data center regions.
*/
private String location;
/*
* SKU properties of the API Management service.
*/
private ApiManagementServiceSkuProperties sku;
/*
* A list of availability zones denoting where the resource needs to come from.
*/
private List zones;
/*
* Public Static Load Balanced IP addresses of the API Management service in the additional location. Available only
* for Basic, Standard, Premium and Isolated SKU.
*/
private List publicIpAddresses;
/*
* Private Static Load Balanced IP addresses of the API Management service which is deployed in an Internal Virtual
* Network in a particular additional location. Available only for Basic, Standard, Premium and Isolated SKU.
*/
private List privateIpAddresses;
/*
* Public Standard SKU IP V4 based IP address to be associated with Virtual Network deployed service in the
* location. Supported only for Premium SKU being deployed in Virtual Network.
*/
private String publicIpAddressId;
/*
* Virtual network configuration for the location.
*/
private VirtualNetworkConfiguration virtualNetworkConfiguration;
/*
* Gateway URL of the API Management service in the Region.
*/
private String gatewayRegionalUrl;
/*
* Property can be used to enable NAT Gateway for this API Management service.
*/
private NatGatewayState natGatewayState;
/*
* Outbound public IPV4 address prefixes associated with NAT Gateway deployed service. Available only for Premium
* SKU on stv2 platform.
*/
private List outboundPublicIpAddresses;
/*
* Property only valid for an Api Management service deployed in multiple locations. This can be used to disable the
* gateway in this additional location.
*/
private Boolean disableGateway;
/*
* Compute Platform Version running the service.
*/
private PlatformVersion platformVersion;
/**
* Creates an instance of AdditionalLocation class.
*/
public AdditionalLocation() {
}
/**
* Get the location property: The location name of the additional region among Azure Data center regions.
*
* @return the location value.
*/
public String location() {
return this.location;
}
/**
* Set the location property: The location name of the additional region among Azure Data center regions.
*
* @param location the location value to set.
* @return the AdditionalLocation object itself.
*/
public AdditionalLocation withLocation(String location) {
this.location = location;
return this;
}
/**
* Get the sku property: SKU properties of the API Management service.
*
* @return the sku value.
*/
public ApiManagementServiceSkuProperties sku() {
return this.sku;
}
/**
* Set the sku property: SKU properties of the API Management service.
*
* @param sku the sku value to set.
* @return the AdditionalLocation object itself.
*/
public AdditionalLocation withSku(ApiManagementServiceSkuProperties sku) {
this.sku = sku;
return this;
}
/**
* Get the zones property: A list of availability zones denoting where the resource needs to come from.
*
* @return the zones value.
*/
public List zones() {
return this.zones;
}
/**
* Set the zones property: A list of availability zones denoting where the resource needs to come from.
*
* @param zones the zones value to set.
* @return the AdditionalLocation object itself.
*/
public AdditionalLocation withZones(List zones) {
this.zones = zones;
return this;
}
/**
* Get the publicIpAddresses property: Public Static Load Balanced IP addresses of the API Management service in the
* additional location. Available only for Basic, Standard, Premium and Isolated SKU.
*
* @return the publicIpAddresses value.
*/
public List publicIpAddresses() {
return this.publicIpAddresses;
}
/**
* Get the privateIpAddresses property: Private Static Load Balanced IP addresses of the API Management service
* which is deployed in an Internal Virtual Network in a particular additional location. Available only for Basic,
* Standard, Premium and Isolated SKU.
*
* @return the privateIpAddresses value.
*/
public List privateIpAddresses() {
return this.privateIpAddresses;
}
/**
* Get the publicIpAddressId property: Public Standard SKU IP V4 based IP address to be associated with Virtual
* Network deployed service in the location. Supported only for Premium SKU being deployed in Virtual Network.
*
* @return the publicIpAddressId value.
*/
public String publicIpAddressId() {
return this.publicIpAddressId;
}
/**
* Set the publicIpAddressId property: Public Standard SKU IP V4 based IP address to be associated with Virtual
* Network deployed service in the location. Supported only for Premium SKU being deployed in Virtual Network.
*
* @param publicIpAddressId the publicIpAddressId value to set.
* @return the AdditionalLocation object itself.
*/
public AdditionalLocation withPublicIpAddressId(String publicIpAddressId) {
this.publicIpAddressId = publicIpAddressId;
return this;
}
/**
* Get the virtualNetworkConfiguration property: Virtual network configuration for the location.
*
* @return the virtualNetworkConfiguration value.
*/
public VirtualNetworkConfiguration virtualNetworkConfiguration() {
return this.virtualNetworkConfiguration;
}
/**
* Set the virtualNetworkConfiguration property: Virtual network configuration for the location.
*
* @param virtualNetworkConfiguration the virtualNetworkConfiguration value to set.
* @return the AdditionalLocation object itself.
*/
public AdditionalLocation withVirtualNetworkConfiguration(VirtualNetworkConfiguration virtualNetworkConfiguration) {
this.virtualNetworkConfiguration = virtualNetworkConfiguration;
return this;
}
/**
* Get the gatewayRegionalUrl property: Gateway URL of the API Management service in the Region.
*
* @return the gatewayRegionalUrl value.
*/
public String gatewayRegionalUrl() {
return this.gatewayRegionalUrl;
}
/**
* Get the natGatewayState property: Property can be used to enable NAT Gateway for this API Management service.
*
* @return the natGatewayState value.
*/
public NatGatewayState natGatewayState() {
return this.natGatewayState;
}
/**
* Set the natGatewayState property: Property can be used to enable NAT Gateway for this API Management service.
*
* @param natGatewayState the natGatewayState value to set.
* @return the AdditionalLocation object itself.
*/
public AdditionalLocation withNatGatewayState(NatGatewayState natGatewayState) {
this.natGatewayState = natGatewayState;
return this;
}
/**
* Get the outboundPublicIpAddresses property: Outbound public IPV4 address prefixes associated with NAT Gateway
* deployed service. Available only for Premium SKU on stv2 platform.
*
* @return the outboundPublicIpAddresses value.
*/
public List outboundPublicIpAddresses() {
return this.outboundPublicIpAddresses;
}
/**
* Get the disableGateway property: Property only valid for an Api Management service deployed in multiple
* locations. This can be used to disable the gateway in this additional location.
*
* @return the disableGateway value.
*/
public Boolean disableGateway() {
return this.disableGateway;
}
/**
* Set the disableGateway property: Property only valid for an Api Management service deployed in multiple
* locations. This can be used to disable the gateway in this additional location.
*
* @param disableGateway the disableGateway value to set.
* @return the AdditionalLocation object itself.
*/
public AdditionalLocation withDisableGateway(Boolean disableGateway) {
this.disableGateway = disableGateway;
return this;
}
/**
* Get the platformVersion property: Compute Platform Version running the service.
*
* @return the platformVersion value.
*/
public PlatformVersion platformVersion() {
return this.platformVersion;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (location() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException("Missing required property location in model AdditionalLocation"));
}
if (sku() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException("Missing required property sku in model AdditionalLocation"));
} else {
sku().validate();
}
if (virtualNetworkConfiguration() != null) {
virtualNetworkConfiguration().validate();
}
}
private static final ClientLogger LOGGER = new ClientLogger(AdditionalLocation.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("location", this.location);
jsonWriter.writeJsonField("sku", this.sku);
jsonWriter.writeArrayField("zones", this.zones, (writer, element) -> writer.writeString(element));
jsonWriter.writeStringField("publicIpAddressId", this.publicIpAddressId);
jsonWriter.writeJsonField("virtualNetworkConfiguration", this.virtualNetworkConfiguration);
jsonWriter.writeStringField("natGatewayState",
this.natGatewayState == null ? null : this.natGatewayState.toString());
jsonWriter.writeBooleanField("disableGateway", this.disableGateway);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AdditionalLocation from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AdditionalLocation if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the AdditionalLocation.
*/
public static AdditionalLocation fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AdditionalLocation deserializedAdditionalLocation = new AdditionalLocation();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("location".equals(fieldName)) {
deserializedAdditionalLocation.location = reader.getString();
} else if ("sku".equals(fieldName)) {
deserializedAdditionalLocation.sku = ApiManagementServiceSkuProperties.fromJson(reader);
} else if ("zones".equals(fieldName)) {
List zones = reader.readArray(reader1 -> reader1.getString());
deserializedAdditionalLocation.zones = zones;
} else if ("publicIPAddresses".equals(fieldName)) {
List publicIpAddresses = reader.readArray(reader1 -> reader1.getString());
deserializedAdditionalLocation.publicIpAddresses = publicIpAddresses;
} else if ("privateIPAddresses".equals(fieldName)) {
List privateIpAddresses = reader.readArray(reader1 -> reader1.getString());
deserializedAdditionalLocation.privateIpAddresses = privateIpAddresses;
} else if ("publicIpAddressId".equals(fieldName)) {
deserializedAdditionalLocation.publicIpAddressId = reader.getString();
} else if ("virtualNetworkConfiguration".equals(fieldName)) {
deserializedAdditionalLocation.virtualNetworkConfiguration
= VirtualNetworkConfiguration.fromJson(reader);
} else if ("gatewayRegionalUrl".equals(fieldName)) {
deserializedAdditionalLocation.gatewayRegionalUrl = reader.getString();
} else if ("natGatewayState".equals(fieldName)) {
deserializedAdditionalLocation.natGatewayState = NatGatewayState.fromString(reader.getString());
} else if ("outboundPublicIPAddresses".equals(fieldName)) {
List outboundPublicIpAddresses = reader.readArray(reader1 -> reader1.getString());
deserializedAdditionalLocation.outboundPublicIpAddresses = outboundPublicIpAddresses;
} else if ("disableGateway".equals(fieldName)) {
deserializedAdditionalLocation.disableGateway = reader.getNullable(JsonReader::getBoolean);
} else if ("platformVersion".equals(fieldName)) {
deserializedAdditionalLocation.platformVersion = PlatformVersion.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedAdditionalLocation;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy