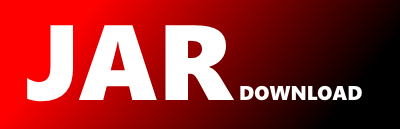
com.azure.resourcemanager.appcontainers.fluent.models.ManagedEnvironmentProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.appcontainers.models.AppLogsConfiguration;
import com.azure.resourcemanager.appcontainers.models.CustomDomainConfiguration;
import com.azure.resourcemanager.appcontainers.models.DaprConfiguration;
import com.azure.resourcemanager.appcontainers.models.EnvironmentProvisioningState;
import com.azure.resourcemanager.appcontainers.models.KedaConfiguration;
import com.azure.resourcemanager.appcontainers.models.ManagedEnvironmentPropertiesPeerAuthentication;
import com.azure.resourcemanager.appcontainers.models.ManagedEnvironmentPropertiesPeerTrafficConfiguration;
import com.azure.resourcemanager.appcontainers.models.VnetConfiguration;
import com.azure.resourcemanager.appcontainers.models.WorkloadProfile;
import java.io.IOException;
import java.util.List;
/**
* Managed environment resource specific properties.
*/
@Fluent
public final class ManagedEnvironmentProperties implements JsonSerializable {
/*
* Provisioning state of the Environment.
*/
private EnvironmentProvisioningState provisioningState;
/*
* Azure Monitor instrumentation key used by Dapr to export Service to Service communication telemetry
*/
private String daprAIInstrumentationKey;
/*
* Application Insights connection string used by Dapr to export Service to Service communication telemetry
*/
private String daprAIConnectionString;
/*
* Vnet configuration for the environment
*/
private VnetConfiguration vnetConfiguration;
/*
* Any errors that occurred during deployment or deployment validation
*/
private String deploymentErrors;
/*
* Default Domain Name for the cluster
*/
private String defaultDomain;
/*
* Static IP of the Environment
*/
private String staticIp;
/*
* Cluster configuration which enables the log daemon to export
* app logs to a destination. Currently only "log-analytics" is
* supported
*/
private AppLogsConfiguration appLogsConfiguration;
/*
* Whether or not this Managed Environment is zone-redundant.
*/
private Boolean zoneRedundant;
/*
* Custom domain configuration for the environment
*/
private CustomDomainConfiguration customDomainConfiguration;
/*
* The endpoint of the eventstream of the Environment.
*/
private String eventStreamEndpoint;
/*
* Workload profiles configured for the Managed Environment.
*/
private List workloadProfiles;
/*
* The configuration of Keda component.
*/
private KedaConfiguration kedaConfiguration;
/*
* The configuration of Dapr component.
*/
private DaprConfiguration daprConfiguration;
/*
* Name of the platform-managed resource group created for the Managed Environment to host infrastructure resources.
* If a subnet ID is provided, this resource group will be created in the same subscription as the subnet.
*/
private String infrastructureResourceGroup;
/*
* Peer authentication settings for the Managed Environment
*/
private ManagedEnvironmentPropertiesPeerAuthentication peerAuthentication;
/*
* Peer traffic settings for the Managed Environment
*/
private ManagedEnvironmentPropertiesPeerTrafficConfiguration peerTrafficConfiguration;
/**
* Creates an instance of ManagedEnvironmentProperties class.
*/
public ManagedEnvironmentProperties() {
}
/**
* Get the provisioningState property: Provisioning state of the Environment.
*
* @return the provisioningState value.
*/
public EnvironmentProvisioningState provisioningState() {
return this.provisioningState;
}
/**
* Get the daprAIInstrumentationKey property: Azure Monitor instrumentation key used by Dapr to export Service to
* Service communication telemetry.
*
* @return the daprAIInstrumentationKey value.
*/
public String daprAIInstrumentationKey() {
return this.daprAIInstrumentationKey;
}
/**
* Set the daprAIInstrumentationKey property: Azure Monitor instrumentation key used by Dapr to export Service to
* Service communication telemetry.
*
* @param daprAIInstrumentationKey the daprAIInstrumentationKey value to set.
* @return the ManagedEnvironmentProperties object itself.
*/
public ManagedEnvironmentProperties withDaprAIInstrumentationKey(String daprAIInstrumentationKey) {
this.daprAIInstrumentationKey = daprAIInstrumentationKey;
return this;
}
/**
* Get the daprAIConnectionString property: Application Insights connection string used by Dapr to export Service to
* Service communication telemetry.
*
* @return the daprAIConnectionString value.
*/
public String daprAIConnectionString() {
return this.daprAIConnectionString;
}
/**
* Set the daprAIConnectionString property: Application Insights connection string used by Dapr to export Service to
* Service communication telemetry.
*
* @param daprAIConnectionString the daprAIConnectionString value to set.
* @return the ManagedEnvironmentProperties object itself.
*/
public ManagedEnvironmentProperties withDaprAIConnectionString(String daprAIConnectionString) {
this.daprAIConnectionString = daprAIConnectionString;
return this;
}
/**
* Get the vnetConfiguration property: Vnet configuration for the environment.
*
* @return the vnetConfiguration value.
*/
public VnetConfiguration vnetConfiguration() {
return this.vnetConfiguration;
}
/**
* Set the vnetConfiguration property: Vnet configuration for the environment.
*
* @param vnetConfiguration the vnetConfiguration value to set.
* @return the ManagedEnvironmentProperties object itself.
*/
public ManagedEnvironmentProperties withVnetConfiguration(VnetConfiguration vnetConfiguration) {
this.vnetConfiguration = vnetConfiguration;
return this;
}
/**
* Get the deploymentErrors property: Any errors that occurred during deployment or deployment validation.
*
* @return the deploymentErrors value.
*/
public String deploymentErrors() {
return this.deploymentErrors;
}
/**
* Get the defaultDomain property: Default Domain Name for the cluster.
*
* @return the defaultDomain value.
*/
public String defaultDomain() {
return this.defaultDomain;
}
/**
* Get the staticIp property: Static IP of the Environment.
*
* @return the staticIp value.
*/
public String staticIp() {
return this.staticIp;
}
/**
* Get the appLogsConfiguration property: Cluster configuration which enables the log daemon to export
* app logs to a destination. Currently only "log-analytics" is
* supported.
*
* @return the appLogsConfiguration value.
*/
public AppLogsConfiguration appLogsConfiguration() {
return this.appLogsConfiguration;
}
/**
* Set the appLogsConfiguration property: Cluster configuration which enables the log daemon to export
* app logs to a destination. Currently only "log-analytics" is
* supported.
*
* @param appLogsConfiguration the appLogsConfiguration value to set.
* @return the ManagedEnvironmentProperties object itself.
*/
public ManagedEnvironmentProperties withAppLogsConfiguration(AppLogsConfiguration appLogsConfiguration) {
this.appLogsConfiguration = appLogsConfiguration;
return this;
}
/**
* Get the zoneRedundant property: Whether or not this Managed Environment is zone-redundant.
*
* @return the zoneRedundant value.
*/
public Boolean zoneRedundant() {
return this.zoneRedundant;
}
/**
* Set the zoneRedundant property: Whether or not this Managed Environment is zone-redundant.
*
* @param zoneRedundant the zoneRedundant value to set.
* @return the ManagedEnvironmentProperties object itself.
*/
public ManagedEnvironmentProperties withZoneRedundant(Boolean zoneRedundant) {
this.zoneRedundant = zoneRedundant;
return this;
}
/**
* Get the customDomainConfiguration property: Custom domain configuration for the environment.
*
* @return the customDomainConfiguration value.
*/
public CustomDomainConfiguration customDomainConfiguration() {
return this.customDomainConfiguration;
}
/**
* Set the customDomainConfiguration property: Custom domain configuration for the environment.
*
* @param customDomainConfiguration the customDomainConfiguration value to set.
* @return the ManagedEnvironmentProperties object itself.
*/
public ManagedEnvironmentProperties
withCustomDomainConfiguration(CustomDomainConfiguration customDomainConfiguration) {
this.customDomainConfiguration = customDomainConfiguration;
return this;
}
/**
* Get the eventStreamEndpoint property: The endpoint of the eventstream of the Environment.
*
* @return the eventStreamEndpoint value.
*/
public String eventStreamEndpoint() {
return this.eventStreamEndpoint;
}
/**
* Get the workloadProfiles property: Workload profiles configured for the Managed Environment.
*
* @return the workloadProfiles value.
*/
public List workloadProfiles() {
return this.workloadProfiles;
}
/**
* Set the workloadProfiles property: Workload profiles configured for the Managed Environment.
*
* @param workloadProfiles the workloadProfiles value to set.
* @return the ManagedEnvironmentProperties object itself.
*/
public ManagedEnvironmentProperties withWorkloadProfiles(List workloadProfiles) {
this.workloadProfiles = workloadProfiles;
return this;
}
/**
* Get the kedaConfiguration property: The configuration of Keda component.
*
* @return the kedaConfiguration value.
*/
public KedaConfiguration kedaConfiguration() {
return this.kedaConfiguration;
}
/**
* Set the kedaConfiguration property: The configuration of Keda component.
*
* @param kedaConfiguration the kedaConfiguration value to set.
* @return the ManagedEnvironmentProperties object itself.
*/
public ManagedEnvironmentProperties withKedaConfiguration(KedaConfiguration kedaConfiguration) {
this.kedaConfiguration = kedaConfiguration;
return this;
}
/**
* Get the daprConfiguration property: The configuration of Dapr component.
*
* @return the daprConfiguration value.
*/
public DaprConfiguration daprConfiguration() {
return this.daprConfiguration;
}
/**
* Set the daprConfiguration property: The configuration of Dapr component.
*
* @param daprConfiguration the daprConfiguration value to set.
* @return the ManagedEnvironmentProperties object itself.
*/
public ManagedEnvironmentProperties withDaprConfiguration(DaprConfiguration daprConfiguration) {
this.daprConfiguration = daprConfiguration;
return this;
}
/**
* Get the infrastructureResourceGroup property: Name of the platform-managed resource group created for the Managed
* Environment to host infrastructure resources. If a subnet ID is provided, this resource group will be created in
* the same subscription as the subnet.
*
* @return the infrastructureResourceGroup value.
*/
public String infrastructureResourceGroup() {
return this.infrastructureResourceGroup;
}
/**
* Set the infrastructureResourceGroup property: Name of the platform-managed resource group created for the Managed
* Environment to host infrastructure resources. If a subnet ID is provided, this resource group will be created in
* the same subscription as the subnet.
*
* @param infrastructureResourceGroup the infrastructureResourceGroup value to set.
* @return the ManagedEnvironmentProperties object itself.
*/
public ManagedEnvironmentProperties withInfrastructureResourceGroup(String infrastructureResourceGroup) {
this.infrastructureResourceGroup = infrastructureResourceGroup;
return this;
}
/**
* Get the peerAuthentication property: Peer authentication settings for the Managed Environment.
*
* @return the peerAuthentication value.
*/
public ManagedEnvironmentPropertiesPeerAuthentication peerAuthentication() {
return this.peerAuthentication;
}
/**
* Set the peerAuthentication property: Peer authentication settings for the Managed Environment.
*
* @param peerAuthentication the peerAuthentication value to set.
* @return the ManagedEnvironmentProperties object itself.
*/
public ManagedEnvironmentProperties
withPeerAuthentication(ManagedEnvironmentPropertiesPeerAuthentication peerAuthentication) {
this.peerAuthentication = peerAuthentication;
return this;
}
/**
* Get the peerTrafficConfiguration property: Peer traffic settings for the Managed Environment.
*
* @return the peerTrafficConfiguration value.
*/
public ManagedEnvironmentPropertiesPeerTrafficConfiguration peerTrafficConfiguration() {
return this.peerTrafficConfiguration;
}
/**
* Set the peerTrafficConfiguration property: Peer traffic settings for the Managed Environment.
*
* @param peerTrafficConfiguration the peerTrafficConfiguration value to set.
* @return the ManagedEnvironmentProperties object itself.
*/
public ManagedEnvironmentProperties
withPeerTrafficConfiguration(ManagedEnvironmentPropertiesPeerTrafficConfiguration peerTrafficConfiguration) {
this.peerTrafficConfiguration = peerTrafficConfiguration;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (vnetConfiguration() != null) {
vnetConfiguration().validate();
}
if (appLogsConfiguration() != null) {
appLogsConfiguration().validate();
}
if (customDomainConfiguration() != null) {
customDomainConfiguration().validate();
}
if (workloadProfiles() != null) {
workloadProfiles().forEach(e -> e.validate());
}
if (kedaConfiguration() != null) {
kedaConfiguration().validate();
}
if (daprConfiguration() != null) {
daprConfiguration().validate();
}
if (peerAuthentication() != null) {
peerAuthentication().validate();
}
if (peerTrafficConfiguration() != null) {
peerTrafficConfiguration().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("daprAIInstrumentationKey", this.daprAIInstrumentationKey);
jsonWriter.writeStringField("daprAIConnectionString", this.daprAIConnectionString);
jsonWriter.writeJsonField("vnetConfiguration", this.vnetConfiguration);
jsonWriter.writeJsonField("appLogsConfiguration", this.appLogsConfiguration);
jsonWriter.writeBooleanField("zoneRedundant", this.zoneRedundant);
jsonWriter.writeJsonField("customDomainConfiguration", this.customDomainConfiguration);
jsonWriter.writeArrayField("workloadProfiles", this.workloadProfiles,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("kedaConfiguration", this.kedaConfiguration);
jsonWriter.writeJsonField("daprConfiguration", this.daprConfiguration);
jsonWriter.writeStringField("infrastructureResourceGroup", this.infrastructureResourceGroup);
jsonWriter.writeJsonField("peerAuthentication", this.peerAuthentication);
jsonWriter.writeJsonField("peerTrafficConfiguration", this.peerTrafficConfiguration);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ManagedEnvironmentProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ManagedEnvironmentProperties if the JsonReader was pointing to an instance of it, or null
* if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the ManagedEnvironmentProperties.
*/
public static ManagedEnvironmentProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ManagedEnvironmentProperties deserializedManagedEnvironmentProperties = new ManagedEnvironmentProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("provisioningState".equals(fieldName)) {
deserializedManagedEnvironmentProperties.provisioningState
= EnvironmentProvisioningState.fromString(reader.getString());
} else if ("daprAIInstrumentationKey".equals(fieldName)) {
deserializedManagedEnvironmentProperties.daprAIInstrumentationKey = reader.getString();
} else if ("daprAIConnectionString".equals(fieldName)) {
deserializedManagedEnvironmentProperties.daprAIConnectionString = reader.getString();
} else if ("vnetConfiguration".equals(fieldName)) {
deserializedManagedEnvironmentProperties.vnetConfiguration = VnetConfiguration.fromJson(reader);
} else if ("deploymentErrors".equals(fieldName)) {
deserializedManagedEnvironmentProperties.deploymentErrors = reader.getString();
} else if ("defaultDomain".equals(fieldName)) {
deserializedManagedEnvironmentProperties.defaultDomain = reader.getString();
} else if ("staticIp".equals(fieldName)) {
deserializedManagedEnvironmentProperties.staticIp = reader.getString();
} else if ("appLogsConfiguration".equals(fieldName)) {
deserializedManagedEnvironmentProperties.appLogsConfiguration
= AppLogsConfiguration.fromJson(reader);
} else if ("zoneRedundant".equals(fieldName)) {
deserializedManagedEnvironmentProperties.zoneRedundant = reader.getNullable(JsonReader::getBoolean);
} else if ("customDomainConfiguration".equals(fieldName)) {
deserializedManagedEnvironmentProperties.customDomainConfiguration
= CustomDomainConfiguration.fromJson(reader);
} else if ("eventStreamEndpoint".equals(fieldName)) {
deserializedManagedEnvironmentProperties.eventStreamEndpoint = reader.getString();
} else if ("workloadProfiles".equals(fieldName)) {
List workloadProfiles
= reader.readArray(reader1 -> WorkloadProfile.fromJson(reader1));
deserializedManagedEnvironmentProperties.workloadProfiles = workloadProfiles;
} else if ("kedaConfiguration".equals(fieldName)) {
deserializedManagedEnvironmentProperties.kedaConfiguration = KedaConfiguration.fromJson(reader);
} else if ("daprConfiguration".equals(fieldName)) {
deserializedManagedEnvironmentProperties.daprConfiguration = DaprConfiguration.fromJson(reader);
} else if ("infrastructureResourceGroup".equals(fieldName)) {
deserializedManagedEnvironmentProperties.infrastructureResourceGroup = reader.getString();
} else if ("peerAuthentication".equals(fieldName)) {
deserializedManagedEnvironmentProperties.peerAuthentication
= ManagedEnvironmentPropertiesPeerAuthentication.fromJson(reader);
} else if ("peerTrafficConfiguration".equals(fieldName)) {
deserializedManagedEnvironmentProperties.peerTrafficConfiguration
= ManagedEnvironmentPropertiesPeerTrafficConfiguration.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedManagedEnvironmentProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy