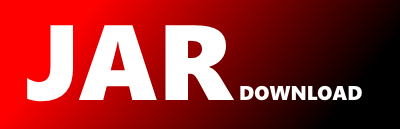
com.azure.resourcemanager.appcontainers.implementation.ContainerAppImpl Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.implementation;
import com.azure.core.http.rest.Response;
import com.azure.core.management.Region;
import com.azure.core.management.SystemData;
import com.azure.core.util.Context;
import com.azure.resourcemanager.appcontainers.fluent.models.ContainerAppInner;
import com.azure.resourcemanager.appcontainers.models.Configuration;
import com.azure.resourcemanager.appcontainers.models.ContainerApp;
import com.azure.resourcemanager.appcontainers.models.ContainerAppAuthToken;
import com.azure.resourcemanager.appcontainers.models.ContainerAppProvisioningState;
import com.azure.resourcemanager.appcontainers.models.CustomHostnameAnalysisResult;
import com.azure.resourcemanager.appcontainers.models.ExtendedLocation;
import com.azure.resourcemanager.appcontainers.models.ManagedServiceIdentity;
import com.azure.resourcemanager.appcontainers.models.SecretsCollection;
import com.azure.resourcemanager.appcontainers.models.Template;
import java.util.Collections;
import java.util.List;
import java.util.Map;
public final class ContainerAppImpl implements ContainerApp, ContainerApp.Definition, ContainerApp.Update {
private ContainerAppInner innerObject;
private final com.azure.resourcemanager.appcontainers.ContainerAppsApiManager serviceManager;
public String id() {
return this.innerModel().id();
}
public String name() {
return this.innerModel().name();
}
public String type() {
return this.innerModel().type();
}
public String location() {
return this.innerModel().location();
}
public Map tags() {
Map inner = this.innerModel().tags();
if (inner != null) {
return Collections.unmodifiableMap(inner);
} else {
return Collections.emptyMap();
}
}
public ExtendedLocation extendedLocation() {
return this.innerModel().extendedLocation();
}
public ManagedServiceIdentity identity() {
return this.innerModel().identity();
}
public String managedBy() {
return this.innerModel().managedBy();
}
public SystemData systemData() {
return this.innerModel().systemData();
}
public ContainerAppProvisioningState provisioningState() {
return this.innerModel().provisioningState();
}
public String managedEnvironmentId() {
return this.innerModel().managedEnvironmentId();
}
public String environmentId() {
return this.innerModel().environmentId();
}
public String workloadProfileName() {
return this.innerModel().workloadProfileName();
}
public String latestRevisionName() {
return this.innerModel().latestRevisionName();
}
public String latestReadyRevisionName() {
return this.innerModel().latestReadyRevisionName();
}
public String latestRevisionFqdn() {
return this.innerModel().latestRevisionFqdn();
}
public String customDomainVerificationId() {
return this.innerModel().customDomainVerificationId();
}
public Configuration configuration() {
return this.innerModel().configuration();
}
public Template template() {
return this.innerModel().template();
}
public List outboundIpAddresses() {
List inner = this.innerModel().outboundIpAddresses();
if (inner != null) {
return Collections.unmodifiableList(inner);
} else {
return Collections.emptyList();
}
}
public String eventStreamEndpoint() {
return this.innerModel().eventStreamEndpoint();
}
public Region region() {
return Region.fromName(this.regionName());
}
public String regionName() {
return this.location();
}
public String resourceGroupName() {
return resourceGroupName;
}
public ContainerAppInner innerModel() {
return this.innerObject;
}
private com.azure.resourcemanager.appcontainers.ContainerAppsApiManager manager() {
return this.serviceManager;
}
private String resourceGroupName;
private String containerAppName;
public ContainerAppImpl withExistingResourceGroup(String resourceGroupName) {
this.resourceGroupName = resourceGroupName;
return this;
}
public ContainerApp create() {
this.innerObject = serviceManager.serviceClient()
.getContainerApps()
.createOrUpdate(resourceGroupName, containerAppName, this.innerModel(), Context.NONE);
return this;
}
public ContainerApp create(Context context) {
this.innerObject = serviceManager.serviceClient()
.getContainerApps()
.createOrUpdate(resourceGroupName, containerAppName, this.innerModel(), context);
return this;
}
ContainerAppImpl(String name, com.azure.resourcemanager.appcontainers.ContainerAppsApiManager serviceManager) {
this.innerObject = new ContainerAppInner();
this.serviceManager = serviceManager;
this.containerAppName = name;
}
public ContainerAppImpl update() {
return this;
}
public ContainerApp apply() {
this.innerObject = serviceManager.serviceClient()
.getContainerApps()
.update(resourceGroupName, containerAppName, this.innerModel(), Context.NONE);
return this;
}
public ContainerApp apply(Context context) {
this.innerObject = serviceManager.serviceClient()
.getContainerApps()
.update(resourceGroupName, containerAppName, this.innerModel(), context);
return this;
}
ContainerAppImpl(ContainerAppInner innerObject,
com.azure.resourcemanager.appcontainers.ContainerAppsApiManager serviceManager) {
this.innerObject = innerObject;
this.serviceManager = serviceManager;
this.resourceGroupName = ResourceManagerUtils.getValueFromIdByName(innerObject.id(), "resourceGroups");
this.containerAppName = ResourceManagerUtils.getValueFromIdByName(innerObject.id(), "containerApps");
}
public ContainerApp refresh() {
this.innerObject = serviceManager.serviceClient()
.getContainerApps()
.getByResourceGroupWithResponse(resourceGroupName, containerAppName, Context.NONE)
.getValue();
return this;
}
public ContainerApp refresh(Context context) {
this.innerObject = serviceManager.serviceClient()
.getContainerApps()
.getByResourceGroupWithResponse(resourceGroupName, containerAppName, context)
.getValue();
return this;
}
public Response listCustomHostnameAnalysisWithResponse(String customHostname,
Context context) {
return serviceManager.containerApps()
.listCustomHostnameAnalysisWithResponse(resourceGroupName, containerAppName, customHostname, context);
}
public CustomHostnameAnalysisResult listCustomHostnameAnalysis() {
return serviceManager.containerApps().listCustomHostnameAnalysis(resourceGroupName, containerAppName);
}
public Response listSecretsWithResponse(Context context) {
return serviceManager.containerApps().listSecretsWithResponse(resourceGroupName, containerAppName, context);
}
public SecretsCollection listSecrets() {
return serviceManager.containerApps().listSecrets(resourceGroupName, containerAppName);
}
public Response getAuthTokenWithResponse(Context context) {
return serviceManager.containerApps().getAuthTokenWithResponse(resourceGroupName, containerAppName, context);
}
public ContainerAppAuthToken getAuthToken() {
return serviceManager.containerApps().getAuthToken(resourceGroupName, containerAppName);
}
public ContainerApp start() {
return serviceManager.containerApps().start(resourceGroupName, containerAppName);
}
public ContainerApp start(Context context) {
return serviceManager.containerApps().start(resourceGroupName, containerAppName, context);
}
public ContainerApp stop() {
return serviceManager.containerApps().stop(resourceGroupName, containerAppName);
}
public ContainerApp stop(Context context) {
return serviceManager.containerApps().stop(resourceGroupName, containerAppName, context);
}
public ContainerAppImpl withRegion(Region location) {
this.innerModel().withLocation(location.toString());
return this;
}
public ContainerAppImpl withRegion(String location) {
this.innerModel().withLocation(location);
return this;
}
public ContainerAppImpl withTags(Map tags) {
this.innerModel().withTags(tags);
return this;
}
public ContainerAppImpl withExtendedLocation(ExtendedLocation extendedLocation) {
this.innerModel().withExtendedLocation(extendedLocation);
return this;
}
public ContainerAppImpl withIdentity(ManagedServiceIdentity identity) {
this.innerModel().withIdentity(identity);
return this;
}
public ContainerAppImpl withManagedBy(String managedBy) {
this.innerModel().withManagedBy(managedBy);
return this;
}
public ContainerAppImpl withManagedEnvironmentId(String managedEnvironmentId) {
this.innerModel().withManagedEnvironmentId(managedEnvironmentId);
return this;
}
public ContainerAppImpl withEnvironmentId(String environmentId) {
this.innerModel().withEnvironmentId(environmentId);
return this;
}
public ContainerAppImpl withWorkloadProfileName(String workloadProfileName) {
this.innerModel().withWorkloadProfileName(workloadProfileName);
return this;
}
public ContainerAppImpl withConfiguration(Configuration configuration) {
this.innerModel().withConfiguration(configuration);
return this;
}
public ContainerAppImpl withTemplate(Template template) {
this.innerModel().withTemplate(template);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy