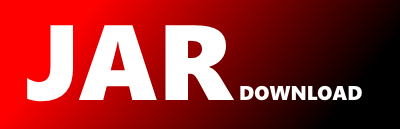
com.azure.resourcemanager.appcontainers.implementation.ContainerAppsApiClientImpl Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.implementation;
import com.azure.core.annotation.ServiceClient;
import com.azure.core.http.HttpHeaderName;
import com.azure.core.http.HttpHeaders;
import com.azure.core.http.HttpPipeline;
import com.azure.core.http.HttpResponse;
import com.azure.core.http.rest.Response;
import com.azure.core.management.AzureEnvironment;
import com.azure.core.management.exception.ManagementError;
import com.azure.core.management.exception.ManagementException;
import com.azure.core.management.polling.PollerFactory;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.CoreUtils;
import com.azure.core.util.logging.ClientLogger;
import com.azure.core.util.polling.AsyncPollResponse;
import com.azure.core.util.polling.LongRunningOperationStatus;
import com.azure.core.util.polling.PollerFlux;
import com.azure.core.util.serializer.SerializerAdapter;
import com.azure.core.util.serializer.SerializerEncoding;
import com.azure.resourcemanager.appcontainers.fluent.AvailableWorkloadProfilesClient;
import com.azure.resourcemanager.appcontainers.fluent.BillingMetersClient;
import com.azure.resourcemanager.appcontainers.fluent.CertificatesClient;
import com.azure.resourcemanager.appcontainers.fluent.ConnectedEnvironmentsCertificatesClient;
import com.azure.resourcemanager.appcontainers.fluent.ConnectedEnvironmentsClient;
import com.azure.resourcemanager.appcontainers.fluent.ConnectedEnvironmentsDaprComponentsClient;
import com.azure.resourcemanager.appcontainers.fluent.ConnectedEnvironmentsStoragesClient;
import com.azure.resourcemanager.appcontainers.fluent.ContainerAppsApiClient;
import com.azure.resourcemanager.appcontainers.fluent.ContainerAppsAuthConfigsClient;
import com.azure.resourcemanager.appcontainers.fluent.ContainerAppsClient;
import com.azure.resourcemanager.appcontainers.fluent.ContainerAppsDiagnosticsClient;
import com.azure.resourcemanager.appcontainers.fluent.ContainerAppsRevisionReplicasClient;
import com.azure.resourcemanager.appcontainers.fluent.ContainerAppsRevisionsClient;
import com.azure.resourcemanager.appcontainers.fluent.ContainerAppsSourceControlsClient;
import com.azure.resourcemanager.appcontainers.fluent.DaprComponentsClient;
import com.azure.resourcemanager.appcontainers.fluent.JobsClient;
import com.azure.resourcemanager.appcontainers.fluent.JobsExecutionsClient;
import com.azure.resourcemanager.appcontainers.fluent.ManagedCertificatesClient;
import com.azure.resourcemanager.appcontainers.fluent.ManagedEnvironmentDiagnosticsClient;
import com.azure.resourcemanager.appcontainers.fluent.ManagedEnvironmentsClient;
import com.azure.resourcemanager.appcontainers.fluent.ManagedEnvironmentsDiagnosticsClient;
import com.azure.resourcemanager.appcontainers.fluent.ManagedEnvironmentsStoragesClient;
import com.azure.resourcemanager.appcontainers.fluent.ManagedEnvironmentUsagesClient;
import com.azure.resourcemanager.appcontainers.fluent.NamespacesClient;
import com.azure.resourcemanager.appcontainers.fluent.OperationsClient;
import com.azure.resourcemanager.appcontainers.fluent.ResourceProvidersClient;
import com.azure.resourcemanager.appcontainers.fluent.UsagesClient;
import java.io.IOException;
import java.lang.reflect.Type;
import java.nio.ByteBuffer;
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.time.Duration;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* Initializes a new instance of the ContainerAppsApiClientImpl type.
*/
@ServiceClient(builder = ContainerAppsApiClientBuilder.class)
public final class ContainerAppsApiClientImpl implements ContainerAppsApiClient {
/**
* The ID of the target subscription.
*/
private final String subscriptionId;
/**
* Gets The ID of the target subscription.
*
* @return the subscriptionId value.
*/
public String getSubscriptionId() {
return this.subscriptionId;
}
/**
* server parameter.
*/
private final String endpoint;
/**
* Gets server parameter.
*
* @return the endpoint value.
*/
public String getEndpoint() {
return this.endpoint;
}
/**
* Api Version.
*/
private final String apiVersion;
/**
* Gets Api Version.
*
* @return the apiVersion value.
*/
public String getApiVersion() {
return this.apiVersion;
}
/**
* The HTTP pipeline to send requests through.
*/
private final HttpPipeline httpPipeline;
/**
* Gets The HTTP pipeline to send requests through.
*
* @return the httpPipeline value.
*/
public HttpPipeline getHttpPipeline() {
return this.httpPipeline;
}
/**
* The serializer to serialize an object into a string.
*/
private final SerializerAdapter serializerAdapter;
/**
* Gets The serializer to serialize an object into a string.
*
* @return the serializerAdapter value.
*/
SerializerAdapter getSerializerAdapter() {
return this.serializerAdapter;
}
/**
* The default poll interval for long-running operation.
*/
private final Duration defaultPollInterval;
/**
* Gets The default poll interval for long-running operation.
*
* @return the defaultPollInterval value.
*/
public Duration getDefaultPollInterval() {
return this.defaultPollInterval;
}
/**
* The ContainerAppsAuthConfigsClient object to access its operations.
*/
private final ContainerAppsAuthConfigsClient containerAppsAuthConfigs;
/**
* Gets the ContainerAppsAuthConfigsClient object to access its operations.
*
* @return the ContainerAppsAuthConfigsClient object.
*/
public ContainerAppsAuthConfigsClient getContainerAppsAuthConfigs() {
return this.containerAppsAuthConfigs;
}
/**
* The AvailableWorkloadProfilesClient object to access its operations.
*/
private final AvailableWorkloadProfilesClient availableWorkloadProfiles;
/**
* Gets the AvailableWorkloadProfilesClient object to access its operations.
*
* @return the AvailableWorkloadProfilesClient object.
*/
public AvailableWorkloadProfilesClient getAvailableWorkloadProfiles() {
return this.availableWorkloadProfiles;
}
/**
* The BillingMetersClient object to access its operations.
*/
private final BillingMetersClient billingMeters;
/**
* Gets the BillingMetersClient object to access its operations.
*
* @return the BillingMetersClient object.
*/
public BillingMetersClient getBillingMeters() {
return this.billingMeters;
}
/**
* The ConnectedEnvironmentsClient object to access its operations.
*/
private final ConnectedEnvironmentsClient connectedEnvironments;
/**
* Gets the ConnectedEnvironmentsClient object to access its operations.
*
* @return the ConnectedEnvironmentsClient object.
*/
public ConnectedEnvironmentsClient getConnectedEnvironments() {
return this.connectedEnvironments;
}
/**
* The ConnectedEnvironmentsCertificatesClient object to access its operations.
*/
private final ConnectedEnvironmentsCertificatesClient connectedEnvironmentsCertificates;
/**
* Gets the ConnectedEnvironmentsCertificatesClient object to access its operations.
*
* @return the ConnectedEnvironmentsCertificatesClient object.
*/
public ConnectedEnvironmentsCertificatesClient getConnectedEnvironmentsCertificates() {
return this.connectedEnvironmentsCertificates;
}
/**
* The ConnectedEnvironmentsDaprComponentsClient object to access its operations.
*/
private final ConnectedEnvironmentsDaprComponentsClient connectedEnvironmentsDaprComponents;
/**
* Gets the ConnectedEnvironmentsDaprComponentsClient object to access its operations.
*
* @return the ConnectedEnvironmentsDaprComponentsClient object.
*/
public ConnectedEnvironmentsDaprComponentsClient getConnectedEnvironmentsDaprComponents() {
return this.connectedEnvironmentsDaprComponents;
}
/**
* The ConnectedEnvironmentsStoragesClient object to access its operations.
*/
private final ConnectedEnvironmentsStoragesClient connectedEnvironmentsStorages;
/**
* Gets the ConnectedEnvironmentsStoragesClient object to access its operations.
*
* @return the ConnectedEnvironmentsStoragesClient object.
*/
public ConnectedEnvironmentsStoragesClient getConnectedEnvironmentsStorages() {
return this.connectedEnvironmentsStorages;
}
/**
* The ContainerAppsClient object to access its operations.
*/
private final ContainerAppsClient containerApps;
/**
* Gets the ContainerAppsClient object to access its operations.
*
* @return the ContainerAppsClient object.
*/
public ContainerAppsClient getContainerApps() {
return this.containerApps;
}
/**
* The ContainerAppsRevisionsClient object to access its operations.
*/
private final ContainerAppsRevisionsClient containerAppsRevisions;
/**
* Gets the ContainerAppsRevisionsClient object to access its operations.
*
* @return the ContainerAppsRevisionsClient object.
*/
public ContainerAppsRevisionsClient getContainerAppsRevisions() {
return this.containerAppsRevisions;
}
/**
* The ContainerAppsRevisionReplicasClient object to access its operations.
*/
private final ContainerAppsRevisionReplicasClient containerAppsRevisionReplicas;
/**
* Gets the ContainerAppsRevisionReplicasClient object to access its operations.
*
* @return the ContainerAppsRevisionReplicasClient object.
*/
public ContainerAppsRevisionReplicasClient getContainerAppsRevisionReplicas() {
return this.containerAppsRevisionReplicas;
}
/**
* The ContainerAppsDiagnosticsClient object to access its operations.
*/
private final ContainerAppsDiagnosticsClient containerAppsDiagnostics;
/**
* Gets the ContainerAppsDiagnosticsClient object to access its operations.
*
* @return the ContainerAppsDiagnosticsClient object.
*/
public ContainerAppsDiagnosticsClient getContainerAppsDiagnostics() {
return this.containerAppsDiagnostics;
}
/**
* The ManagedEnvironmentDiagnosticsClient object to access its operations.
*/
private final ManagedEnvironmentDiagnosticsClient managedEnvironmentDiagnostics;
/**
* Gets the ManagedEnvironmentDiagnosticsClient object to access its operations.
*
* @return the ManagedEnvironmentDiagnosticsClient object.
*/
public ManagedEnvironmentDiagnosticsClient getManagedEnvironmentDiagnostics() {
return this.managedEnvironmentDiagnostics;
}
/**
* The ManagedEnvironmentsDiagnosticsClient object to access its operations.
*/
private final ManagedEnvironmentsDiagnosticsClient managedEnvironmentsDiagnostics;
/**
* Gets the ManagedEnvironmentsDiagnosticsClient object to access its operations.
*
* @return the ManagedEnvironmentsDiagnosticsClient object.
*/
public ManagedEnvironmentsDiagnosticsClient getManagedEnvironmentsDiagnostics() {
return this.managedEnvironmentsDiagnostics;
}
/**
* The JobsClient object to access its operations.
*/
private final JobsClient jobs;
/**
* Gets the JobsClient object to access its operations.
*
* @return the JobsClient object.
*/
public JobsClient getJobs() {
return this.jobs;
}
/**
* The OperationsClient object to access its operations.
*/
private final OperationsClient operations;
/**
* Gets the OperationsClient object to access its operations.
*
* @return the OperationsClient object.
*/
public OperationsClient getOperations() {
return this.operations;
}
/**
* The JobsExecutionsClient object to access its operations.
*/
private final JobsExecutionsClient jobsExecutions;
/**
* Gets the JobsExecutionsClient object to access its operations.
*
* @return the JobsExecutionsClient object.
*/
public JobsExecutionsClient getJobsExecutions() {
return this.jobsExecutions;
}
/**
* The ResourceProvidersClient object to access its operations.
*/
private final ResourceProvidersClient resourceProviders;
/**
* Gets the ResourceProvidersClient object to access its operations.
*
* @return the ResourceProvidersClient object.
*/
public ResourceProvidersClient getResourceProviders() {
return this.resourceProviders;
}
/**
* The ManagedEnvironmentsClient object to access its operations.
*/
private final ManagedEnvironmentsClient managedEnvironments;
/**
* Gets the ManagedEnvironmentsClient object to access its operations.
*
* @return the ManagedEnvironmentsClient object.
*/
public ManagedEnvironmentsClient getManagedEnvironments() {
return this.managedEnvironments;
}
/**
* The CertificatesClient object to access its operations.
*/
private final CertificatesClient certificates;
/**
* Gets the CertificatesClient object to access its operations.
*
* @return the CertificatesClient object.
*/
public CertificatesClient getCertificates() {
return this.certificates;
}
/**
* The ManagedCertificatesClient object to access its operations.
*/
private final ManagedCertificatesClient managedCertificates;
/**
* Gets the ManagedCertificatesClient object to access its operations.
*
* @return the ManagedCertificatesClient object.
*/
public ManagedCertificatesClient getManagedCertificates() {
return this.managedCertificates;
}
/**
* The NamespacesClient object to access its operations.
*/
private final NamespacesClient namespaces;
/**
* Gets the NamespacesClient object to access its operations.
*
* @return the NamespacesClient object.
*/
public NamespacesClient getNamespaces() {
return this.namespaces;
}
/**
* The DaprComponentsClient object to access its operations.
*/
private final DaprComponentsClient daprComponents;
/**
* Gets the DaprComponentsClient object to access its operations.
*
* @return the DaprComponentsClient object.
*/
public DaprComponentsClient getDaprComponents() {
return this.daprComponents;
}
/**
* The ManagedEnvironmentsStoragesClient object to access its operations.
*/
private final ManagedEnvironmentsStoragesClient managedEnvironmentsStorages;
/**
* Gets the ManagedEnvironmentsStoragesClient object to access its operations.
*
* @return the ManagedEnvironmentsStoragesClient object.
*/
public ManagedEnvironmentsStoragesClient getManagedEnvironmentsStorages() {
return this.managedEnvironmentsStorages;
}
/**
* The ContainerAppsSourceControlsClient object to access its operations.
*/
private final ContainerAppsSourceControlsClient containerAppsSourceControls;
/**
* Gets the ContainerAppsSourceControlsClient object to access its operations.
*
* @return the ContainerAppsSourceControlsClient object.
*/
public ContainerAppsSourceControlsClient getContainerAppsSourceControls() {
return this.containerAppsSourceControls;
}
/**
* The UsagesClient object to access its operations.
*/
private final UsagesClient usages;
/**
* Gets the UsagesClient object to access its operations.
*
* @return the UsagesClient object.
*/
public UsagesClient getUsages() {
return this.usages;
}
/**
* The ManagedEnvironmentUsagesClient object to access its operations.
*/
private final ManagedEnvironmentUsagesClient managedEnvironmentUsages;
/**
* Gets the ManagedEnvironmentUsagesClient object to access its operations.
*
* @return the ManagedEnvironmentUsagesClient object.
*/
public ManagedEnvironmentUsagesClient getManagedEnvironmentUsages() {
return this.managedEnvironmentUsages;
}
/**
* Initializes an instance of ContainerAppsApiClient client.
*
* @param httpPipeline The HTTP pipeline to send requests through.
* @param serializerAdapter The serializer to serialize an object into a string.
* @param defaultPollInterval The default poll interval for long-running operation.
* @param environment The Azure environment.
* @param subscriptionId The ID of the target subscription.
* @param endpoint server parameter.
*/
ContainerAppsApiClientImpl(HttpPipeline httpPipeline, SerializerAdapter serializerAdapter,
Duration defaultPollInterval, AzureEnvironment environment, String subscriptionId, String endpoint) {
this.httpPipeline = httpPipeline;
this.serializerAdapter = serializerAdapter;
this.defaultPollInterval = defaultPollInterval;
this.subscriptionId = subscriptionId;
this.endpoint = endpoint;
this.apiVersion = "2024-03-01";
this.containerAppsAuthConfigs = new ContainerAppsAuthConfigsClientImpl(this);
this.availableWorkloadProfiles = new AvailableWorkloadProfilesClientImpl(this);
this.billingMeters = new BillingMetersClientImpl(this);
this.connectedEnvironments = new ConnectedEnvironmentsClientImpl(this);
this.connectedEnvironmentsCertificates = new ConnectedEnvironmentsCertificatesClientImpl(this);
this.connectedEnvironmentsDaprComponents = new ConnectedEnvironmentsDaprComponentsClientImpl(this);
this.connectedEnvironmentsStorages = new ConnectedEnvironmentsStoragesClientImpl(this);
this.containerApps = new ContainerAppsClientImpl(this);
this.containerAppsRevisions = new ContainerAppsRevisionsClientImpl(this);
this.containerAppsRevisionReplicas = new ContainerAppsRevisionReplicasClientImpl(this);
this.containerAppsDiagnostics = new ContainerAppsDiagnosticsClientImpl(this);
this.managedEnvironmentDiagnostics = new ManagedEnvironmentDiagnosticsClientImpl(this);
this.managedEnvironmentsDiagnostics = new ManagedEnvironmentsDiagnosticsClientImpl(this);
this.jobs = new JobsClientImpl(this);
this.operations = new OperationsClientImpl(this);
this.jobsExecutions = new JobsExecutionsClientImpl(this);
this.resourceProviders = new ResourceProvidersClientImpl(this);
this.managedEnvironments = new ManagedEnvironmentsClientImpl(this);
this.certificates = new CertificatesClientImpl(this);
this.managedCertificates = new ManagedCertificatesClientImpl(this);
this.namespaces = new NamespacesClientImpl(this);
this.daprComponents = new DaprComponentsClientImpl(this);
this.managedEnvironmentsStorages = new ManagedEnvironmentsStoragesClientImpl(this);
this.containerAppsSourceControls = new ContainerAppsSourceControlsClientImpl(this);
this.usages = new UsagesClientImpl(this);
this.managedEnvironmentUsages = new ManagedEnvironmentUsagesClientImpl(this);
}
/**
* Gets default client context.
*
* @return the default client context.
*/
public Context getContext() {
return Context.NONE;
}
/**
* Merges default client context with provided context.
*
* @param context the context to be merged with default client context.
* @return the merged context.
*/
public Context mergeContext(Context context) {
return CoreUtils.mergeContexts(this.getContext(), context);
}
/**
* Gets long running operation result.
*
* @param activationResponse the response of activation operation.
* @param httpPipeline the http pipeline.
* @param pollResultType type of poll result.
* @param finalResultType type of final result.
* @param context the context shared by all requests.
* @param type of poll result.
* @param type of final result.
* @return poller flux for poll result and final result.
*/
public PollerFlux, U> getLroResult(Mono>> activationResponse,
HttpPipeline httpPipeline, Type pollResultType, Type finalResultType, Context context) {
return PollerFactory.create(serializerAdapter, httpPipeline, pollResultType, finalResultType,
defaultPollInterval, activationResponse, context);
}
/**
* Gets the final result, or an error, based on last async poll response.
*
* @param response the last async poll response.
* @param type of poll result.
* @param type of final result.
* @return the final result, or an error.
*/
public Mono getLroFinalResultOrError(AsyncPollResponse, U> response) {
if (response.getStatus() != LongRunningOperationStatus.SUCCESSFULLY_COMPLETED) {
String errorMessage;
ManagementError managementError = null;
HttpResponse errorResponse = null;
PollResult.Error lroError = response.getValue().getError();
if (lroError != null) {
errorResponse = new HttpResponseImpl(lroError.getResponseStatusCode(), lroError.getResponseHeaders(),
lroError.getResponseBody());
errorMessage = response.getValue().getError().getMessage();
String errorBody = response.getValue().getError().getResponseBody();
if (errorBody != null) {
// try to deserialize error body to ManagementError
try {
managementError = this.getSerializerAdapter()
.deserialize(errorBody, ManagementError.class, SerializerEncoding.JSON);
if (managementError.getCode() == null || managementError.getMessage() == null) {
managementError = null;
}
} catch (IOException | RuntimeException ioe) {
LOGGER.logThrowableAsWarning(ioe);
}
}
} else {
// fallback to default error message
errorMessage = "Long running operation failed.";
}
if (managementError == null) {
// fallback to default ManagementError
managementError = new ManagementError(response.getStatus().toString(), errorMessage);
}
return Mono.error(new ManagementException(errorMessage, errorResponse, managementError));
} else {
return response.getFinalResult();
}
}
private static final class HttpResponseImpl extends HttpResponse {
private final int statusCode;
private final byte[] responseBody;
private final HttpHeaders httpHeaders;
HttpResponseImpl(int statusCode, HttpHeaders httpHeaders, String responseBody) {
super(null);
this.statusCode = statusCode;
this.httpHeaders = httpHeaders;
this.responseBody = responseBody == null ? null : responseBody.getBytes(StandardCharsets.UTF_8);
}
public int getStatusCode() {
return statusCode;
}
public String getHeaderValue(String s) {
return httpHeaders.getValue(HttpHeaderName.fromString(s));
}
public HttpHeaders getHeaders() {
return httpHeaders;
}
public Flux getBody() {
return Flux.just(ByteBuffer.wrap(responseBody));
}
public Mono getBodyAsByteArray() {
return Mono.just(responseBody);
}
public Mono getBodyAsString() {
return Mono.just(new String(responseBody, StandardCharsets.UTF_8));
}
public Mono getBodyAsString(Charset charset) {
return Mono.just(new String(responseBody, charset));
}
}
private static final ClientLogger LOGGER = new ClientLogger(ContainerAppsApiClientImpl.class);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy