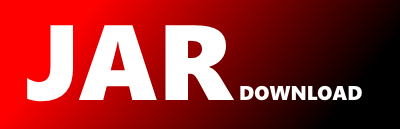
com.azure.resourcemanager.appcontainers.models.Configuration Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* Non versioned Container App configuration properties that define the mutable settings of a Container app.
*/
@Fluent
public final class Configuration implements JsonSerializable {
/*
* Collection of secrets used by a Container app
*/
private List secrets;
/*
* ActiveRevisionsMode controls how active revisions are handled for the Container app:
* - Multiple: multiple revisions can be active.
- Single: Only one revision can be active at a
* time. Revision weights can not be used in this mode. If no value if provided, this is the default.
*/
private ActiveRevisionsMode activeRevisionsMode;
/*
* Ingress configurations.
*/
private Ingress ingress;
/*
* Collection of private container registry credentials for containers used by the Container app
*/
private List registries;
/*
* Dapr configuration for the Container App.
*/
private Dapr dapr;
/*
* Optional. Max inactive revisions a Container App can have.
*/
private Integer maxInactiveRevisions;
/*
* Container App to be a dev Container App Service
*/
private Service service;
/**
* Creates an instance of Configuration class.
*/
public Configuration() {
}
/**
* Get the secrets property: Collection of secrets used by a Container app.
*
* @return the secrets value.
*/
public List secrets() {
return this.secrets;
}
/**
* Set the secrets property: Collection of secrets used by a Container app.
*
* @param secrets the secrets value to set.
* @return the Configuration object itself.
*/
public Configuration withSecrets(List secrets) {
this.secrets = secrets;
return this;
}
/**
* Get the activeRevisionsMode property: ActiveRevisionsMode controls how active revisions are handled for the
* Container app:
* <list><item>Multiple: multiple revisions can be active.</item><item>Single: Only one
* revision can be active at a time. Revision weights can not be used in this mode. If no value if provided, this is
* the default.</item></list>.
*
* @return the activeRevisionsMode value.
*/
public ActiveRevisionsMode activeRevisionsMode() {
return this.activeRevisionsMode;
}
/**
* Set the activeRevisionsMode property: ActiveRevisionsMode controls how active revisions are handled for the
* Container app:
* <list><item>Multiple: multiple revisions can be active.</item><item>Single: Only one
* revision can be active at a time. Revision weights can not be used in this mode. If no value if provided, this is
* the default.</item></list>.
*
* @param activeRevisionsMode the activeRevisionsMode value to set.
* @return the Configuration object itself.
*/
public Configuration withActiveRevisionsMode(ActiveRevisionsMode activeRevisionsMode) {
this.activeRevisionsMode = activeRevisionsMode;
return this;
}
/**
* Get the ingress property: Ingress configurations.
*
* @return the ingress value.
*/
public Ingress ingress() {
return this.ingress;
}
/**
* Set the ingress property: Ingress configurations.
*
* @param ingress the ingress value to set.
* @return the Configuration object itself.
*/
public Configuration withIngress(Ingress ingress) {
this.ingress = ingress;
return this;
}
/**
* Get the registries property: Collection of private container registry credentials for containers used by the
* Container app.
*
* @return the registries value.
*/
public List registries() {
return this.registries;
}
/**
* Set the registries property: Collection of private container registry credentials for containers used by the
* Container app.
*
* @param registries the registries value to set.
* @return the Configuration object itself.
*/
public Configuration withRegistries(List registries) {
this.registries = registries;
return this;
}
/**
* Get the dapr property: Dapr configuration for the Container App.
*
* @return the dapr value.
*/
public Dapr dapr() {
return this.dapr;
}
/**
* Set the dapr property: Dapr configuration for the Container App.
*
* @param dapr the dapr value to set.
* @return the Configuration object itself.
*/
public Configuration withDapr(Dapr dapr) {
this.dapr = dapr;
return this;
}
/**
* Get the maxInactiveRevisions property: Optional. Max inactive revisions a Container App can have.
*
* @return the maxInactiveRevisions value.
*/
public Integer maxInactiveRevisions() {
return this.maxInactiveRevisions;
}
/**
* Set the maxInactiveRevisions property: Optional. Max inactive revisions a Container App can have.
*
* @param maxInactiveRevisions the maxInactiveRevisions value to set.
* @return the Configuration object itself.
*/
public Configuration withMaxInactiveRevisions(Integer maxInactiveRevisions) {
this.maxInactiveRevisions = maxInactiveRevisions;
return this;
}
/**
* Get the service property: Container App to be a dev Container App Service.
*
* @return the service value.
*/
public Service service() {
return this.service;
}
/**
* Set the service property: Container App to be a dev Container App Service.
*
* @param service the service value to set.
* @return the Configuration object itself.
*/
public Configuration withService(Service service) {
this.service = service;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (secrets() != null) {
secrets().forEach(e -> e.validate());
}
if (ingress() != null) {
ingress().validate();
}
if (registries() != null) {
registries().forEach(e -> e.validate());
}
if (dapr() != null) {
dapr().validate();
}
if (service() != null) {
service().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeArrayField("secrets", this.secrets, (writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("activeRevisionsMode",
this.activeRevisionsMode == null ? null : this.activeRevisionsMode.toString());
jsonWriter.writeJsonField("ingress", this.ingress);
jsonWriter.writeArrayField("registries", this.registries, (writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("dapr", this.dapr);
jsonWriter.writeNumberField("maxInactiveRevisions", this.maxInactiveRevisions);
jsonWriter.writeJsonField("service", this.service);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of Configuration from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of Configuration if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the Configuration.
*/
public static Configuration fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
Configuration deserializedConfiguration = new Configuration();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("secrets".equals(fieldName)) {
List secrets = reader.readArray(reader1 -> Secret.fromJson(reader1));
deserializedConfiguration.secrets = secrets;
} else if ("activeRevisionsMode".equals(fieldName)) {
deserializedConfiguration.activeRevisionsMode = ActiveRevisionsMode.fromString(reader.getString());
} else if ("ingress".equals(fieldName)) {
deserializedConfiguration.ingress = Ingress.fromJson(reader);
} else if ("registries".equals(fieldName)) {
List registries
= reader.readArray(reader1 -> RegistryCredentials.fromJson(reader1));
deserializedConfiguration.registries = registries;
} else if ("dapr".equals(fieldName)) {
deserializedConfiguration.dapr = Dapr.fromJson(reader);
} else if ("maxInactiveRevisions".equals(fieldName)) {
deserializedConfiguration.maxInactiveRevisions = reader.getNullable(JsonReader::getInt);
} else if ("service".equals(fieldName)) {
deserializedConfiguration.service = Service.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedConfiguration;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy