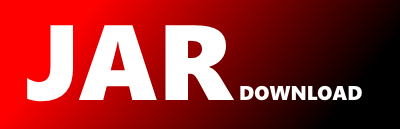
com.azure.resourcemanager.appcontainers.models.ContainerAppProbe Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Probe describes a health check to be performed against a container to determine whether it is alive or ready to
* receive traffic.
*/
@Fluent
public final class ContainerAppProbe implements JsonSerializable {
/*
* Minimum consecutive failures for the probe to be considered failed after having succeeded. Defaults to 3. Minimum
* value is 1. Maximum value is 10.
*/
private Integer failureThreshold;
/*
* HTTPGet specifies the http request to perform.
*/
private ContainerAppProbeHttpGet httpGet;
/*
* Number of seconds after the container has started before liveness probes are initiated. Minimum value is 1.
* Maximum value is 60.
*/
private Integer initialDelaySeconds;
/*
* How often (in seconds) to perform the probe. Default to 10 seconds. Minimum value is 1. Maximum value is 240.
*/
private Integer periodSeconds;
/*
* Minimum consecutive successes for the probe to be considered successful after having failed. Defaults to 1. Must
* be 1 for liveness and startup. Minimum value is 1. Maximum value is 10.
*/
private Integer successThreshold;
/*
* TCPSocket specifies an action involving a TCP port. TCP hooks not yet supported.
*/
private ContainerAppProbeTcpSocket tcpSocket;
/*
* Optional duration in seconds the pod needs to terminate gracefully upon probe failure. The grace period is the
* duration in seconds after the processes running in the pod are sent a termination signal and the time when the
* processes are forcibly halted with a kill signal. Set this value longer than the expected cleanup time for your
* process. If this value is nil, the pod's terminationGracePeriodSeconds will be used. Otherwise, this value
* overrides the value provided by the pod spec. Value must be non-negative integer. The value zero indicates stop
* immediately via the kill signal (no opportunity to shut down). This is an alpha field and requires enabling
* ProbeTerminationGracePeriod feature gate. Maximum value is 3600 seconds (1 hour)
*/
private Long terminationGracePeriodSeconds;
/*
* Number of seconds after which the probe times out. Defaults to 1 second. Minimum value is 1. Maximum value is
* 240.
*/
private Integer timeoutSeconds;
/*
* The type of probe.
*/
private Type type;
/**
* Creates an instance of ContainerAppProbe class.
*/
public ContainerAppProbe() {
}
/**
* Get the failureThreshold property: Minimum consecutive failures for the probe to be considered failed after
* having succeeded. Defaults to 3. Minimum value is 1. Maximum value is 10.
*
* @return the failureThreshold value.
*/
public Integer failureThreshold() {
return this.failureThreshold;
}
/**
* Set the failureThreshold property: Minimum consecutive failures for the probe to be considered failed after
* having succeeded. Defaults to 3. Minimum value is 1. Maximum value is 10.
*
* @param failureThreshold the failureThreshold value to set.
* @return the ContainerAppProbe object itself.
*/
public ContainerAppProbe withFailureThreshold(Integer failureThreshold) {
this.failureThreshold = failureThreshold;
return this;
}
/**
* Get the httpGet property: HTTPGet specifies the http request to perform.
*
* @return the httpGet value.
*/
public ContainerAppProbeHttpGet httpGet() {
return this.httpGet;
}
/**
* Set the httpGet property: HTTPGet specifies the http request to perform.
*
* @param httpGet the httpGet value to set.
* @return the ContainerAppProbe object itself.
*/
public ContainerAppProbe withHttpGet(ContainerAppProbeHttpGet httpGet) {
this.httpGet = httpGet;
return this;
}
/**
* Get the initialDelaySeconds property: Number of seconds after the container has started before liveness probes
* are initiated. Minimum value is 1. Maximum value is 60.
*
* @return the initialDelaySeconds value.
*/
public Integer initialDelaySeconds() {
return this.initialDelaySeconds;
}
/**
* Set the initialDelaySeconds property: Number of seconds after the container has started before liveness probes
* are initiated. Minimum value is 1. Maximum value is 60.
*
* @param initialDelaySeconds the initialDelaySeconds value to set.
* @return the ContainerAppProbe object itself.
*/
public ContainerAppProbe withInitialDelaySeconds(Integer initialDelaySeconds) {
this.initialDelaySeconds = initialDelaySeconds;
return this;
}
/**
* Get the periodSeconds property: How often (in seconds) to perform the probe. Default to 10 seconds. Minimum value
* is 1. Maximum value is 240.
*
* @return the periodSeconds value.
*/
public Integer periodSeconds() {
return this.periodSeconds;
}
/**
* Set the periodSeconds property: How often (in seconds) to perform the probe. Default to 10 seconds. Minimum value
* is 1. Maximum value is 240.
*
* @param periodSeconds the periodSeconds value to set.
* @return the ContainerAppProbe object itself.
*/
public ContainerAppProbe withPeriodSeconds(Integer periodSeconds) {
this.periodSeconds = periodSeconds;
return this;
}
/**
* Get the successThreshold property: Minimum consecutive successes for the probe to be considered successful after
* having failed. Defaults to 1. Must be 1 for liveness and startup. Minimum value is 1. Maximum value is 10.
*
* @return the successThreshold value.
*/
public Integer successThreshold() {
return this.successThreshold;
}
/**
* Set the successThreshold property: Minimum consecutive successes for the probe to be considered successful after
* having failed. Defaults to 1. Must be 1 for liveness and startup. Minimum value is 1. Maximum value is 10.
*
* @param successThreshold the successThreshold value to set.
* @return the ContainerAppProbe object itself.
*/
public ContainerAppProbe withSuccessThreshold(Integer successThreshold) {
this.successThreshold = successThreshold;
return this;
}
/**
* Get the tcpSocket property: TCPSocket specifies an action involving a TCP port. TCP hooks not yet supported.
*
* @return the tcpSocket value.
*/
public ContainerAppProbeTcpSocket tcpSocket() {
return this.tcpSocket;
}
/**
* Set the tcpSocket property: TCPSocket specifies an action involving a TCP port. TCP hooks not yet supported.
*
* @param tcpSocket the tcpSocket value to set.
* @return the ContainerAppProbe object itself.
*/
public ContainerAppProbe withTcpSocket(ContainerAppProbeTcpSocket tcpSocket) {
this.tcpSocket = tcpSocket;
return this;
}
/**
* Get the terminationGracePeriodSeconds property: Optional duration in seconds the pod needs to terminate
* gracefully upon probe failure. The grace period is the duration in seconds after the processes running in the pod
* are sent a termination signal and the time when the processes are forcibly halted with a kill signal. Set this
* value longer than the expected cleanup time for your process. If this value is nil, the pod's
* terminationGracePeriodSeconds will be used. Otherwise, this value overrides the value provided by the pod spec.
* Value must be non-negative integer. The value zero indicates stop immediately via the kill signal (no opportunity
* to shut down). This is an alpha field and requires enabling ProbeTerminationGracePeriod feature gate. Maximum
* value is 3600 seconds (1 hour).
*
* @return the terminationGracePeriodSeconds value.
*/
public Long terminationGracePeriodSeconds() {
return this.terminationGracePeriodSeconds;
}
/**
* Set the terminationGracePeriodSeconds property: Optional duration in seconds the pod needs to terminate
* gracefully upon probe failure. The grace period is the duration in seconds after the processes running in the pod
* are sent a termination signal and the time when the processes are forcibly halted with a kill signal. Set this
* value longer than the expected cleanup time for your process. If this value is nil, the pod's
* terminationGracePeriodSeconds will be used. Otherwise, this value overrides the value provided by the pod spec.
* Value must be non-negative integer. The value zero indicates stop immediately via the kill signal (no opportunity
* to shut down). This is an alpha field and requires enabling ProbeTerminationGracePeriod feature gate. Maximum
* value is 3600 seconds (1 hour).
*
* @param terminationGracePeriodSeconds the terminationGracePeriodSeconds value to set.
* @return the ContainerAppProbe object itself.
*/
public ContainerAppProbe withTerminationGracePeriodSeconds(Long terminationGracePeriodSeconds) {
this.terminationGracePeriodSeconds = terminationGracePeriodSeconds;
return this;
}
/**
* Get the timeoutSeconds property: Number of seconds after which the probe times out. Defaults to 1 second. Minimum
* value is 1. Maximum value is 240.
*
* @return the timeoutSeconds value.
*/
public Integer timeoutSeconds() {
return this.timeoutSeconds;
}
/**
* Set the timeoutSeconds property: Number of seconds after which the probe times out. Defaults to 1 second. Minimum
* value is 1. Maximum value is 240.
*
* @param timeoutSeconds the timeoutSeconds value to set.
* @return the ContainerAppProbe object itself.
*/
public ContainerAppProbe withTimeoutSeconds(Integer timeoutSeconds) {
this.timeoutSeconds = timeoutSeconds;
return this;
}
/**
* Get the type property: The type of probe.
*
* @return the type value.
*/
public Type type() {
return this.type;
}
/**
* Set the type property: The type of probe.
*
* @param type the type value to set.
* @return the ContainerAppProbe object itself.
*/
public ContainerAppProbe withType(Type type) {
this.type = type;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (httpGet() != null) {
httpGet().validate();
}
if (tcpSocket() != null) {
tcpSocket().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeNumberField("failureThreshold", this.failureThreshold);
jsonWriter.writeJsonField("httpGet", this.httpGet);
jsonWriter.writeNumberField("initialDelaySeconds", this.initialDelaySeconds);
jsonWriter.writeNumberField("periodSeconds", this.periodSeconds);
jsonWriter.writeNumberField("successThreshold", this.successThreshold);
jsonWriter.writeJsonField("tcpSocket", this.tcpSocket);
jsonWriter.writeNumberField("terminationGracePeriodSeconds", this.terminationGracePeriodSeconds);
jsonWriter.writeNumberField("timeoutSeconds", this.timeoutSeconds);
jsonWriter.writeStringField("type", this.type == null ? null : this.type.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ContainerAppProbe from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ContainerAppProbe if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the ContainerAppProbe.
*/
public static ContainerAppProbe fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ContainerAppProbe deserializedContainerAppProbe = new ContainerAppProbe();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("failureThreshold".equals(fieldName)) {
deserializedContainerAppProbe.failureThreshold = reader.getNullable(JsonReader::getInt);
} else if ("httpGet".equals(fieldName)) {
deserializedContainerAppProbe.httpGet = ContainerAppProbeHttpGet.fromJson(reader);
} else if ("initialDelaySeconds".equals(fieldName)) {
deserializedContainerAppProbe.initialDelaySeconds = reader.getNullable(JsonReader::getInt);
} else if ("periodSeconds".equals(fieldName)) {
deserializedContainerAppProbe.periodSeconds = reader.getNullable(JsonReader::getInt);
} else if ("successThreshold".equals(fieldName)) {
deserializedContainerAppProbe.successThreshold = reader.getNullable(JsonReader::getInt);
} else if ("tcpSocket".equals(fieldName)) {
deserializedContainerAppProbe.tcpSocket = ContainerAppProbeTcpSocket.fromJson(reader);
} else if ("terminationGracePeriodSeconds".equals(fieldName)) {
deserializedContainerAppProbe.terminationGracePeriodSeconds
= reader.getNullable(JsonReader::getLong);
} else if ("timeoutSeconds".equals(fieldName)) {
deserializedContainerAppProbe.timeoutSeconds = reader.getNullable(JsonReader::getInt);
} else if ("type".equals(fieldName)) {
deserializedContainerAppProbe.type = Type.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedContainerAppProbe;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy