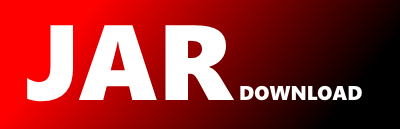
com.azure.resourcemanager.appcontainers.models.GithubActionConfiguration Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Configuration properties that define the mutable settings of a Container App SourceControl.
*/
@Fluent
public final class GithubActionConfiguration implements JsonSerializable {
/*
* Registry configurations.
*/
private RegistryInfo registryInfo;
/*
* AzureCredentials configurations.
*/
private AzureCredentials azureCredentials;
/*
* Context path
*/
private String contextPath;
/*
* One time Github PAT to configure github environment
*/
private String githubPersonalAccessToken;
/*
* Image name
*/
private String image;
/*
* Code or Image
*/
private String publishType;
/*
* Operation system
*/
private String os;
/*
* Runtime stack
*/
private String runtimeStack;
/*
* Runtime version
*/
private String runtimeVersion;
/**
* Creates an instance of GithubActionConfiguration class.
*/
public GithubActionConfiguration() {
}
/**
* Get the registryInfo property: Registry configurations.
*
* @return the registryInfo value.
*/
public RegistryInfo registryInfo() {
return this.registryInfo;
}
/**
* Set the registryInfo property: Registry configurations.
*
* @param registryInfo the registryInfo value to set.
* @return the GithubActionConfiguration object itself.
*/
public GithubActionConfiguration withRegistryInfo(RegistryInfo registryInfo) {
this.registryInfo = registryInfo;
return this;
}
/**
* Get the azureCredentials property: AzureCredentials configurations.
*
* @return the azureCredentials value.
*/
public AzureCredentials azureCredentials() {
return this.azureCredentials;
}
/**
* Set the azureCredentials property: AzureCredentials configurations.
*
* @param azureCredentials the azureCredentials value to set.
* @return the GithubActionConfiguration object itself.
*/
public GithubActionConfiguration withAzureCredentials(AzureCredentials azureCredentials) {
this.azureCredentials = azureCredentials;
return this;
}
/**
* Get the contextPath property: Context path.
*
* @return the contextPath value.
*/
public String contextPath() {
return this.contextPath;
}
/**
* Set the contextPath property: Context path.
*
* @param contextPath the contextPath value to set.
* @return the GithubActionConfiguration object itself.
*/
public GithubActionConfiguration withContextPath(String contextPath) {
this.contextPath = contextPath;
return this;
}
/**
* Get the githubPersonalAccessToken property: One time Github PAT to configure github environment.
*
* @return the githubPersonalAccessToken value.
*/
public String githubPersonalAccessToken() {
return this.githubPersonalAccessToken;
}
/**
* Set the githubPersonalAccessToken property: One time Github PAT to configure github environment.
*
* @param githubPersonalAccessToken the githubPersonalAccessToken value to set.
* @return the GithubActionConfiguration object itself.
*/
public GithubActionConfiguration withGithubPersonalAccessToken(String githubPersonalAccessToken) {
this.githubPersonalAccessToken = githubPersonalAccessToken;
return this;
}
/**
* Get the image property: Image name.
*
* @return the image value.
*/
public String image() {
return this.image;
}
/**
* Set the image property: Image name.
*
* @param image the image value to set.
* @return the GithubActionConfiguration object itself.
*/
public GithubActionConfiguration withImage(String image) {
this.image = image;
return this;
}
/**
* Get the publishType property: Code or Image.
*
* @return the publishType value.
*/
public String publishType() {
return this.publishType;
}
/**
* Set the publishType property: Code or Image.
*
* @param publishType the publishType value to set.
* @return the GithubActionConfiguration object itself.
*/
public GithubActionConfiguration withPublishType(String publishType) {
this.publishType = publishType;
return this;
}
/**
* Get the os property: Operation system.
*
* @return the os value.
*/
public String os() {
return this.os;
}
/**
* Set the os property: Operation system.
*
* @param os the os value to set.
* @return the GithubActionConfiguration object itself.
*/
public GithubActionConfiguration withOs(String os) {
this.os = os;
return this;
}
/**
* Get the runtimeStack property: Runtime stack.
*
* @return the runtimeStack value.
*/
public String runtimeStack() {
return this.runtimeStack;
}
/**
* Set the runtimeStack property: Runtime stack.
*
* @param runtimeStack the runtimeStack value to set.
* @return the GithubActionConfiguration object itself.
*/
public GithubActionConfiguration withRuntimeStack(String runtimeStack) {
this.runtimeStack = runtimeStack;
return this;
}
/**
* Get the runtimeVersion property: Runtime version.
*
* @return the runtimeVersion value.
*/
public String runtimeVersion() {
return this.runtimeVersion;
}
/**
* Set the runtimeVersion property: Runtime version.
*
* @param runtimeVersion the runtimeVersion value to set.
* @return the GithubActionConfiguration object itself.
*/
public GithubActionConfiguration withRuntimeVersion(String runtimeVersion) {
this.runtimeVersion = runtimeVersion;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (registryInfo() != null) {
registryInfo().validate();
}
if (azureCredentials() != null) {
azureCredentials().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("registryInfo", this.registryInfo);
jsonWriter.writeJsonField("azureCredentials", this.azureCredentials);
jsonWriter.writeStringField("contextPath", this.contextPath);
jsonWriter.writeStringField("githubPersonalAccessToken", this.githubPersonalAccessToken);
jsonWriter.writeStringField("image", this.image);
jsonWriter.writeStringField("publishType", this.publishType);
jsonWriter.writeStringField("os", this.os);
jsonWriter.writeStringField("runtimeStack", this.runtimeStack);
jsonWriter.writeStringField("runtimeVersion", this.runtimeVersion);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of GithubActionConfiguration from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of GithubActionConfiguration if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IOException If an error occurs while reading the GithubActionConfiguration.
*/
public static GithubActionConfiguration fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
GithubActionConfiguration deserializedGithubActionConfiguration = new GithubActionConfiguration();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("registryInfo".equals(fieldName)) {
deserializedGithubActionConfiguration.registryInfo = RegistryInfo.fromJson(reader);
} else if ("azureCredentials".equals(fieldName)) {
deserializedGithubActionConfiguration.azureCredentials = AzureCredentials.fromJson(reader);
} else if ("contextPath".equals(fieldName)) {
deserializedGithubActionConfiguration.contextPath = reader.getString();
} else if ("githubPersonalAccessToken".equals(fieldName)) {
deserializedGithubActionConfiguration.githubPersonalAccessToken = reader.getString();
} else if ("image".equals(fieldName)) {
deserializedGithubActionConfiguration.image = reader.getString();
} else if ("publishType".equals(fieldName)) {
deserializedGithubActionConfiguration.publishType = reader.getString();
} else if ("os".equals(fieldName)) {
deserializedGithubActionConfiguration.os = reader.getString();
} else if ("runtimeStack".equals(fieldName)) {
deserializedGithubActionConfiguration.runtimeStack = reader.getString();
} else if ("runtimeVersion".equals(fieldName)) {
deserializedGithubActionConfiguration.runtimeVersion = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedGithubActionConfiguration;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy