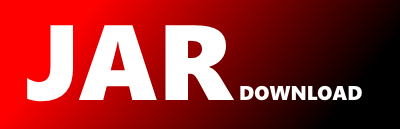
com.azure.resourcemanager.appcontainers.models.JobExecutionContainer Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* Container Apps Jobs execution container definition.
*/
@Fluent
public final class JobExecutionContainer implements JsonSerializable {
/*
* Container image tag.
*/
private String image;
/*
* Custom container name.
*/
private String name;
/*
* Container start command.
*/
private List command;
/*
* Container start command arguments.
*/
private List args;
/*
* Container environment variables.
*/
private List env;
/*
* Container resource requirements.
*/
private ContainerResources resources;
/**
* Creates an instance of JobExecutionContainer class.
*/
public JobExecutionContainer() {
}
/**
* Get the image property: Container image tag.
*
* @return the image value.
*/
public String image() {
return this.image;
}
/**
* Set the image property: Container image tag.
*
* @param image the image value to set.
* @return the JobExecutionContainer object itself.
*/
public JobExecutionContainer withImage(String image) {
this.image = image;
return this;
}
/**
* Get the name property: Custom container name.
*
* @return the name value.
*/
public String name() {
return this.name;
}
/**
* Set the name property: Custom container name.
*
* @param name the name value to set.
* @return the JobExecutionContainer object itself.
*/
public JobExecutionContainer withName(String name) {
this.name = name;
return this;
}
/**
* Get the command property: Container start command.
*
* @return the command value.
*/
public List command() {
return this.command;
}
/**
* Set the command property: Container start command.
*
* @param command the command value to set.
* @return the JobExecutionContainer object itself.
*/
public JobExecutionContainer withCommand(List command) {
this.command = command;
return this;
}
/**
* Get the args property: Container start command arguments.
*
* @return the args value.
*/
public List args() {
return this.args;
}
/**
* Set the args property: Container start command arguments.
*
* @param args the args value to set.
* @return the JobExecutionContainer object itself.
*/
public JobExecutionContainer withArgs(List args) {
this.args = args;
return this;
}
/**
* Get the env property: Container environment variables.
*
* @return the env value.
*/
public List env() {
return this.env;
}
/**
* Set the env property: Container environment variables.
*
* @param env the env value to set.
* @return the JobExecutionContainer object itself.
*/
public JobExecutionContainer withEnv(List env) {
this.env = env;
return this;
}
/**
* Get the resources property: Container resource requirements.
*
* @return the resources value.
*/
public ContainerResources resources() {
return this.resources;
}
/**
* Set the resources property: Container resource requirements.
*
* @param resources the resources value to set.
* @return the JobExecutionContainer object itself.
*/
public JobExecutionContainer withResources(ContainerResources resources) {
this.resources = resources;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (env() != null) {
env().forEach(e -> e.validate());
}
if (resources() != null) {
resources().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("image", this.image);
jsonWriter.writeStringField("name", this.name);
jsonWriter.writeArrayField("command", this.command, (writer, element) -> writer.writeString(element));
jsonWriter.writeArrayField("args", this.args, (writer, element) -> writer.writeString(element));
jsonWriter.writeArrayField("env", this.env, (writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("resources", this.resources);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of JobExecutionContainer from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of JobExecutionContainer if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the JobExecutionContainer.
*/
public static JobExecutionContainer fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
JobExecutionContainer deserializedJobExecutionContainer = new JobExecutionContainer();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("image".equals(fieldName)) {
deserializedJobExecutionContainer.image = reader.getString();
} else if ("name".equals(fieldName)) {
deserializedJobExecutionContainer.name = reader.getString();
} else if ("command".equals(fieldName)) {
List command = reader.readArray(reader1 -> reader1.getString());
deserializedJobExecutionContainer.command = command;
} else if ("args".equals(fieldName)) {
List args = reader.readArray(reader1 -> reader1.getString());
deserializedJobExecutionContainer.args = args;
} else if ("env".equals(fieldName)) {
List env = reader.readArray(reader1 -> EnvironmentVar.fromJson(reader1));
deserializedJobExecutionContainer.env = env;
} else if ("resources".equals(fieldName)) {
deserializedJobExecutionContainer.resources = ContainerResources.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedJobExecutionContainer;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy