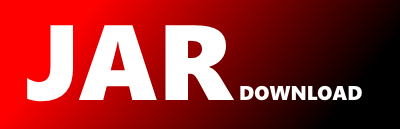
com.azure.resourcemanager.appcontainers.models.LogAnalyticsConfiguration Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Log Analytics configuration, must only be provided when destination is configured as 'log-analytics'.
*/
@Fluent
public final class LogAnalyticsConfiguration implements JsonSerializable {
/*
* Log analytics customer id
*/
private String customerId;
/*
* Log analytics customer key
*/
private String sharedKey;
/**
* Creates an instance of LogAnalyticsConfiguration class.
*/
public LogAnalyticsConfiguration() {
}
/**
* Get the customerId property: Log analytics customer id.
*
* @return the customerId value.
*/
public String customerId() {
return this.customerId;
}
/**
* Set the customerId property: Log analytics customer id.
*
* @param customerId the customerId value to set.
* @return the LogAnalyticsConfiguration object itself.
*/
public LogAnalyticsConfiguration withCustomerId(String customerId) {
this.customerId = customerId;
return this;
}
/**
* Get the sharedKey property: Log analytics customer key.
*
* @return the sharedKey value.
*/
public String sharedKey() {
return this.sharedKey;
}
/**
* Set the sharedKey property: Log analytics customer key.
*
* @param sharedKey the sharedKey value to set.
* @return the LogAnalyticsConfiguration object itself.
*/
public LogAnalyticsConfiguration withSharedKey(String sharedKey) {
this.sharedKey = sharedKey;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("customerId", this.customerId);
jsonWriter.writeStringField("sharedKey", this.sharedKey);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of LogAnalyticsConfiguration from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of LogAnalyticsConfiguration if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IOException If an error occurs while reading the LogAnalyticsConfiguration.
*/
public static LogAnalyticsConfiguration fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
LogAnalyticsConfiguration deserializedLogAnalyticsConfiguration = new LogAnalyticsConfiguration();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("customerId".equals(fieldName)) {
deserializedLogAnalyticsConfiguration.customerId = reader.getString();
} else if ("sharedKey".equals(fieldName)) {
deserializedLogAnalyticsConfiguration.sharedKey = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedLogAnalyticsConfiguration;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy