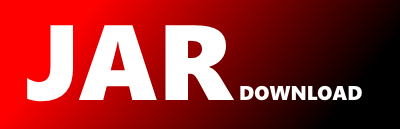
com.azure.resourcemanager.appcontainers.models.VnetConfiguration Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Configuration properties for apps environment to join a Virtual Network.
*/
@Fluent
public final class VnetConfiguration implements JsonSerializable {
/*
* Boolean indicating the environment only has an internal load balancer. These environments do not have a public
* static IP resource. They must provide infrastructureSubnetId if enabling this property
*/
private Boolean internal;
/*
* Resource ID of a subnet for infrastructure components. Must not overlap with any other provided IP ranges.
*/
private String infrastructureSubnetId;
/*
* CIDR notation IP range assigned to the Docker bridge, network. Must not overlap with any other provided IP
* ranges.
*/
private String dockerBridgeCidr;
/*
* IP range in CIDR notation that can be reserved for environment infrastructure IP addresses. Must not overlap with
* any other provided IP ranges.
*/
private String platformReservedCidr;
/*
* An IP address from the IP range defined by platformReservedCidr that will be reserved for the internal DNS
* server.
*/
private String platformReservedDnsIp;
/**
* Creates an instance of VnetConfiguration class.
*/
public VnetConfiguration() {
}
/**
* Get the internal property: Boolean indicating the environment only has an internal load balancer. These
* environments do not have a public static IP resource. They must provide infrastructureSubnetId if enabling this
* property.
*
* @return the internal value.
*/
public Boolean internal() {
return this.internal;
}
/**
* Set the internal property: Boolean indicating the environment only has an internal load balancer. These
* environments do not have a public static IP resource. They must provide infrastructureSubnetId if enabling this
* property.
*
* @param internal the internal value to set.
* @return the VnetConfiguration object itself.
*/
public VnetConfiguration withInternal(Boolean internal) {
this.internal = internal;
return this;
}
/**
* Get the infrastructureSubnetId property: Resource ID of a subnet for infrastructure components. Must not overlap
* with any other provided IP ranges.
*
* @return the infrastructureSubnetId value.
*/
public String infrastructureSubnetId() {
return this.infrastructureSubnetId;
}
/**
* Set the infrastructureSubnetId property: Resource ID of a subnet for infrastructure components. Must not overlap
* with any other provided IP ranges.
*
* @param infrastructureSubnetId the infrastructureSubnetId value to set.
* @return the VnetConfiguration object itself.
*/
public VnetConfiguration withInfrastructureSubnetId(String infrastructureSubnetId) {
this.infrastructureSubnetId = infrastructureSubnetId;
return this;
}
/**
* Get the dockerBridgeCidr property: CIDR notation IP range assigned to the Docker bridge, network. Must not
* overlap with any other provided IP ranges.
*
* @return the dockerBridgeCidr value.
*/
public String dockerBridgeCidr() {
return this.dockerBridgeCidr;
}
/**
* Set the dockerBridgeCidr property: CIDR notation IP range assigned to the Docker bridge, network. Must not
* overlap with any other provided IP ranges.
*
* @param dockerBridgeCidr the dockerBridgeCidr value to set.
* @return the VnetConfiguration object itself.
*/
public VnetConfiguration withDockerBridgeCidr(String dockerBridgeCidr) {
this.dockerBridgeCidr = dockerBridgeCidr;
return this;
}
/**
* Get the platformReservedCidr property: IP range in CIDR notation that can be reserved for environment
* infrastructure IP addresses. Must not overlap with any other provided IP ranges.
*
* @return the platformReservedCidr value.
*/
public String platformReservedCidr() {
return this.platformReservedCidr;
}
/**
* Set the platformReservedCidr property: IP range in CIDR notation that can be reserved for environment
* infrastructure IP addresses. Must not overlap with any other provided IP ranges.
*
* @param platformReservedCidr the platformReservedCidr value to set.
* @return the VnetConfiguration object itself.
*/
public VnetConfiguration withPlatformReservedCidr(String platformReservedCidr) {
this.platformReservedCidr = platformReservedCidr;
return this;
}
/**
* Get the platformReservedDnsIp property: An IP address from the IP range defined by platformReservedCidr that will
* be reserved for the internal DNS server.
*
* @return the platformReservedDnsIp value.
*/
public String platformReservedDnsIp() {
return this.platformReservedDnsIp;
}
/**
* Set the platformReservedDnsIp property: An IP address from the IP range defined by platformReservedCidr that will
* be reserved for the internal DNS server.
*
* @param platformReservedDnsIp the platformReservedDnsIp value to set.
* @return the VnetConfiguration object itself.
*/
public VnetConfiguration withPlatformReservedDnsIp(String platformReservedDnsIp) {
this.platformReservedDnsIp = platformReservedDnsIp;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeBooleanField("internal", this.internal);
jsonWriter.writeStringField("infrastructureSubnetId", this.infrastructureSubnetId);
jsonWriter.writeStringField("dockerBridgeCidr", this.dockerBridgeCidr);
jsonWriter.writeStringField("platformReservedCidr", this.platformReservedCidr);
jsonWriter.writeStringField("platformReservedDnsIP", this.platformReservedDnsIp);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of VnetConfiguration from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of VnetConfiguration if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the VnetConfiguration.
*/
public static VnetConfiguration fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
VnetConfiguration deserializedVnetConfiguration = new VnetConfiguration();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("internal".equals(fieldName)) {
deserializedVnetConfiguration.internal = reader.getNullable(JsonReader::getBoolean);
} else if ("infrastructureSubnetId".equals(fieldName)) {
deserializedVnetConfiguration.infrastructureSubnetId = reader.getString();
} else if ("dockerBridgeCidr".equals(fieldName)) {
deserializedVnetConfiguration.dockerBridgeCidr = reader.getString();
} else if ("platformReservedCidr".equals(fieldName)) {
deserializedVnetConfiguration.platformReservedCidr = reader.getString();
} else if ("platformReservedDnsIP".equals(fieldName)) {
deserializedVnetConfiguration.platformReservedDnsIp = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedVnetConfiguration;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy