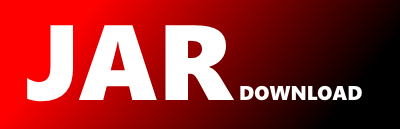
com.azure.resourcemanager.appcontainers.fluent.models.AuthConfigProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-appcontainers Show documentation
Show all versions of azure-resourcemanager-appcontainers Show documentation
This package contains Microsoft Azure SDK for ContainerAppsApi Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-03.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.appcontainers.models.AuthPlatform;
import com.azure.resourcemanager.appcontainers.models.EncryptionSettings;
import com.azure.resourcemanager.appcontainers.models.GlobalValidation;
import com.azure.resourcemanager.appcontainers.models.HttpSettings;
import com.azure.resourcemanager.appcontainers.models.IdentityProviders;
import com.azure.resourcemanager.appcontainers.models.Login;
import java.io.IOException;
/**
* AuthConfig resource specific properties.
*/
@Fluent
public final class AuthConfigProperties implements JsonSerializable {
/*
* The configuration settings of the platform of ContainerApp Service Authentication/Authorization.
*/
private AuthPlatform platform;
/*
* The configuration settings that determines the validation flow of users using Service
* Authentication/Authorization.
*/
private GlobalValidation globalValidation;
/*
* The configuration settings of each of the identity providers used to configure ContainerApp Service
* Authentication/Authorization.
*/
private IdentityProviders identityProviders;
/*
* The configuration settings of the login flow of users using ContainerApp Service Authentication/Authorization.
*/
private Login login;
/*
* The configuration settings of the HTTP requests for authentication and authorization requests made against
* ContainerApp Service Authentication/Authorization.
*/
private HttpSettings httpSettings;
/*
* The configuration settings of the secrets references of encryption key and signing key for ContainerApp Service
* Authentication/Authorization.
*/
private EncryptionSettings encryptionSettings;
/**
* Creates an instance of AuthConfigProperties class.
*/
public AuthConfigProperties() {
}
/**
* Get the platform property: The configuration settings of the platform of ContainerApp Service
* Authentication/Authorization.
*
* @return the platform value.
*/
public AuthPlatform platform() {
return this.platform;
}
/**
* Set the platform property: The configuration settings of the platform of ContainerApp Service
* Authentication/Authorization.
*
* @param platform the platform value to set.
* @return the AuthConfigProperties object itself.
*/
public AuthConfigProperties withPlatform(AuthPlatform platform) {
this.platform = platform;
return this;
}
/**
* Get the globalValidation property: The configuration settings that determines the validation flow of users using
* Service Authentication/Authorization.
*
* @return the globalValidation value.
*/
public GlobalValidation globalValidation() {
return this.globalValidation;
}
/**
* Set the globalValidation property: The configuration settings that determines the validation flow of users using
* Service Authentication/Authorization.
*
* @param globalValidation the globalValidation value to set.
* @return the AuthConfigProperties object itself.
*/
public AuthConfigProperties withGlobalValidation(GlobalValidation globalValidation) {
this.globalValidation = globalValidation;
return this;
}
/**
* Get the identityProviders property: The configuration settings of each of the identity providers used to
* configure ContainerApp Service Authentication/Authorization.
*
* @return the identityProviders value.
*/
public IdentityProviders identityProviders() {
return this.identityProviders;
}
/**
* Set the identityProviders property: The configuration settings of each of the identity providers used to
* configure ContainerApp Service Authentication/Authorization.
*
* @param identityProviders the identityProviders value to set.
* @return the AuthConfigProperties object itself.
*/
public AuthConfigProperties withIdentityProviders(IdentityProviders identityProviders) {
this.identityProviders = identityProviders;
return this;
}
/**
* Get the login property: The configuration settings of the login flow of users using ContainerApp Service
* Authentication/Authorization.
*
* @return the login value.
*/
public Login login() {
return this.login;
}
/**
* Set the login property: The configuration settings of the login flow of users using ContainerApp Service
* Authentication/Authorization.
*
* @param login the login value to set.
* @return the AuthConfigProperties object itself.
*/
public AuthConfigProperties withLogin(Login login) {
this.login = login;
return this;
}
/**
* Get the httpSettings property: The configuration settings of the HTTP requests for authentication and
* authorization requests made against ContainerApp Service Authentication/Authorization.
*
* @return the httpSettings value.
*/
public HttpSettings httpSettings() {
return this.httpSettings;
}
/**
* Set the httpSettings property: The configuration settings of the HTTP requests for authentication and
* authorization requests made against ContainerApp Service Authentication/Authorization.
*
* @param httpSettings the httpSettings value to set.
* @return the AuthConfigProperties object itself.
*/
public AuthConfigProperties withHttpSettings(HttpSettings httpSettings) {
this.httpSettings = httpSettings;
return this;
}
/**
* Get the encryptionSettings property: The configuration settings of the secrets references of encryption key and
* signing key for ContainerApp Service Authentication/Authorization.
*
* @return the encryptionSettings value.
*/
public EncryptionSettings encryptionSettings() {
return this.encryptionSettings;
}
/**
* Set the encryptionSettings property: The configuration settings of the secrets references of encryption key and
* signing key for ContainerApp Service Authentication/Authorization.
*
* @param encryptionSettings the encryptionSettings value to set.
* @return the AuthConfigProperties object itself.
*/
public AuthConfigProperties withEncryptionSettings(EncryptionSettings encryptionSettings) {
this.encryptionSettings = encryptionSettings;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (platform() != null) {
platform().validate();
}
if (globalValidation() != null) {
globalValidation().validate();
}
if (identityProviders() != null) {
identityProviders().validate();
}
if (login() != null) {
login().validate();
}
if (httpSettings() != null) {
httpSettings().validate();
}
if (encryptionSettings() != null) {
encryptionSettings().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("platform", this.platform);
jsonWriter.writeJsonField("globalValidation", this.globalValidation);
jsonWriter.writeJsonField("identityProviders", this.identityProviders);
jsonWriter.writeJsonField("login", this.login);
jsonWriter.writeJsonField("httpSettings", this.httpSettings);
jsonWriter.writeJsonField("encryptionSettings", this.encryptionSettings);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AuthConfigProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AuthConfigProperties if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the AuthConfigProperties.
*/
public static AuthConfigProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AuthConfigProperties deserializedAuthConfigProperties = new AuthConfigProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("platform".equals(fieldName)) {
deserializedAuthConfigProperties.platform = AuthPlatform.fromJson(reader);
} else if ("globalValidation".equals(fieldName)) {
deserializedAuthConfigProperties.globalValidation = GlobalValidation.fromJson(reader);
} else if ("identityProviders".equals(fieldName)) {
deserializedAuthConfigProperties.identityProviders = IdentityProviders.fromJson(reader);
} else if ("login".equals(fieldName)) {
deserializedAuthConfigProperties.login = Login.fromJson(reader);
} else if ("httpSettings".equals(fieldName)) {
deserializedAuthConfigProperties.httpSettings = HttpSettings.fromJson(reader);
} else if ("encryptionSettings".equals(fieldName)) {
deserializedAuthConfigProperties.encryptionSettings = EncryptionSettings.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedAuthConfigProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy