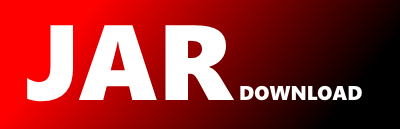
com.azure.resourcemanager.appcontainers.implementation.LogicAppsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-appcontainers Show documentation
Show all versions of azure-resourcemanager-appcontainers Show documentation
This package contains Microsoft Azure SDK for ContainerAppsApi Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-03.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.implementation;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.SimpleResponse;
import com.azure.core.util.Context;
import com.azure.core.util.logging.ClientLogger;
import com.azure.resourcemanager.appcontainers.fluent.LogicAppsClient;
import com.azure.resourcemanager.appcontainers.fluent.models.LogicAppInner;
import com.azure.resourcemanager.appcontainers.fluent.models.WorkflowEnvelopeInner;
import com.azure.resourcemanager.appcontainers.models.LogicApp;
import com.azure.resourcemanager.appcontainers.models.LogicApps;
import com.azure.resourcemanager.appcontainers.models.LogicAppsProxyMethod;
import com.azure.resourcemanager.appcontainers.models.WorkflowArtifacts;
import com.azure.resourcemanager.appcontainers.models.WorkflowEnvelope;
public final class LogicAppsImpl implements LogicApps {
private static final ClientLogger LOGGER = new ClientLogger(LogicAppsImpl.class);
private final LogicAppsClient innerClient;
private final com.azure.resourcemanager.appcontainers.ContainerAppsApiManager serviceManager;
public LogicAppsImpl(LogicAppsClient innerClient,
com.azure.resourcemanager.appcontainers.ContainerAppsApiManager serviceManager) {
this.innerClient = innerClient;
this.serviceManager = serviceManager;
}
public Response getWithResponse(String resourceGroupName, String containerAppName, String logicAppName,
Context context) {
Response inner
= this.serviceClient().getWithResponse(resourceGroupName, containerAppName, logicAppName, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new LogicAppImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public LogicApp get(String resourceGroupName, String containerAppName, String logicAppName) {
LogicAppInner inner = this.serviceClient().get(resourceGroupName, containerAppName, logicAppName);
if (inner != null) {
return new LogicAppImpl(inner, this.manager());
} else {
return null;
}
}
public Response deleteWithResponse(String resourceGroupName, String containerAppName, String logicAppName,
Context context) {
return this.serviceClient().deleteWithResponse(resourceGroupName, containerAppName, logicAppName, context);
}
public void delete(String resourceGroupName, String containerAppName, String logicAppName) {
this.serviceClient().delete(resourceGroupName, containerAppName, logicAppName);
}
public PagedIterable listWorkflows(String resourceGroupName, String containerAppName,
String logicAppName) {
PagedIterable inner
= this.serviceClient().listWorkflows(resourceGroupName, containerAppName, logicAppName);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkflowEnvelopeImpl(inner1, this.manager()));
}
public PagedIterable listWorkflows(String resourceGroupName, String containerAppName,
String logicAppName, Context context) {
PagedIterable inner
= this.serviceClient().listWorkflows(resourceGroupName, containerAppName, logicAppName, context);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkflowEnvelopeImpl(inner1, this.manager()));
}
public Response getWorkflowWithResponse(String resourceGroupName, String containerAppName,
String logicAppName, String workflowName, Context context) {
Response inner = this.serviceClient()
.getWorkflowWithResponse(resourceGroupName, containerAppName, logicAppName, workflowName, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new WorkflowEnvelopeImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public WorkflowEnvelope getWorkflow(String resourceGroupName, String containerAppName, String logicAppName,
String workflowName) {
WorkflowEnvelopeInner inner
= this.serviceClient().getWorkflow(resourceGroupName, containerAppName, logicAppName, workflowName);
if (inner != null) {
return new WorkflowEnvelopeImpl(inner, this.manager());
} else {
return null;
}
}
public Response deployWorkflowArtifactsWithResponse(String resourceGroupName, String containerAppName,
String logicAppName, WorkflowArtifacts workflowArtifacts, Context context) {
return this.serviceClient()
.deployWorkflowArtifactsWithResponse(resourceGroupName, containerAppName, logicAppName, workflowArtifacts,
context);
}
public void deployWorkflowArtifacts(String resourceGroupName, String containerAppName, String logicAppName) {
this.serviceClient().deployWorkflowArtifacts(resourceGroupName, containerAppName, logicAppName);
}
public Response listWorkflowsConnectionsWithResponse(String resourceGroupName,
String containerAppName, String logicAppName, Context context) {
Response inner = this.serviceClient()
.listWorkflowsConnectionsWithResponse(resourceGroupName, containerAppName, logicAppName, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new WorkflowEnvelopeImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public WorkflowEnvelope listWorkflowsConnections(String resourceGroupName, String containerAppName,
String logicAppName) {
WorkflowEnvelopeInner inner
= this.serviceClient().listWorkflowsConnections(resourceGroupName, containerAppName, logicAppName);
if (inner != null) {
return new WorkflowEnvelopeImpl(inner, this.manager());
} else {
return null;
}
}
public Response
© 2015 - 2025 Weber Informatics LLC | Privacy Policy