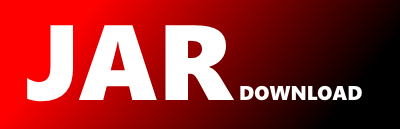
com.azure.resourcemanager.appcontainers.models.AzureActiveDirectoryRegistration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-appcontainers Show documentation
Show all versions of azure-resourcemanager-appcontainers Show documentation
This package contains Microsoft Azure SDK for ContainerAppsApi Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-03.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* The configuration settings of the Azure Active Directory app registration.
*/
@Fluent
public final class AzureActiveDirectoryRegistration implements JsonSerializable {
/*
* The OpenID Connect Issuer URI that represents the entity which issues access tokens for this application.
* When using Azure Active Directory, this value is the URI of the directory tenant, e.g.
* https://login.microsoftonline.com/v2.0/{tenant-guid}/.
* This URI is a case-sensitive identifier for the token issuer.
* More information on OpenID Connect Discovery: http://openid.net/specs/openid-connect-discovery-1_0.html
*/
private String openIdIssuer;
/*
* The Client ID of this relying party application, known as the client_id.
* This setting is required for enabling OpenID Connection authentication with Azure Active Directory or
* other 3rd party OpenID Connect providers.
* More information on OpenID Connect: http://openid.net/specs/openid-connect-core-1_0.html
*/
private String clientId;
/*
* The app setting name that contains the client secret of the relying party application.
*/
private String clientSecretSettingName;
/*
* An alternative to the client secret, that is the thumbprint of a certificate used for signing purposes. This
* property acts as
* a replacement for the Client Secret. It is also optional.
*/
private String clientSecretCertificateThumbprint;
/*
* An alternative to the client secret thumbprint, that is the subject alternative name of a certificate used for
* signing purposes. This property acts as
* a replacement for the Client Secret Certificate Thumbprint. It is also optional.
*/
private String clientSecretCertificateSubjectAlternativeName;
/*
* An alternative to the client secret thumbprint, that is the issuer of a certificate used for signing purposes.
* This property acts as
* a replacement for the Client Secret Certificate Thumbprint. It is also optional.
*/
private String clientSecretCertificateIssuer;
/**
* Creates an instance of AzureActiveDirectoryRegistration class.
*/
public AzureActiveDirectoryRegistration() {
}
/**
* Get the openIdIssuer property: The OpenID Connect Issuer URI that represents the entity which issues access
* tokens for this application.
* When using Azure Active Directory, this value is the URI of the directory tenant, e.g.
* https://login.microsoftonline.com/v2.0/{tenant-guid}/.
* This URI is a case-sensitive identifier for the token issuer.
* More information on OpenID Connect Discovery: http://openid.net/specs/openid-connect-discovery-1_0.html.
*
* @return the openIdIssuer value.
*/
public String openIdIssuer() {
return this.openIdIssuer;
}
/**
* Set the openIdIssuer property: The OpenID Connect Issuer URI that represents the entity which issues access
* tokens for this application.
* When using Azure Active Directory, this value is the URI of the directory tenant, e.g.
* https://login.microsoftonline.com/v2.0/{tenant-guid}/.
* This URI is a case-sensitive identifier for the token issuer.
* More information on OpenID Connect Discovery: http://openid.net/specs/openid-connect-discovery-1_0.html.
*
* @param openIdIssuer the openIdIssuer value to set.
* @return the AzureActiveDirectoryRegistration object itself.
*/
public AzureActiveDirectoryRegistration withOpenIdIssuer(String openIdIssuer) {
this.openIdIssuer = openIdIssuer;
return this;
}
/**
* Get the clientId property: The Client ID of this relying party application, known as the client_id.
* This setting is required for enabling OpenID Connection authentication with Azure Active Directory or
* other 3rd party OpenID Connect providers.
* More information on OpenID Connect: http://openid.net/specs/openid-connect-core-1_0.html.
*
* @return the clientId value.
*/
public String clientId() {
return this.clientId;
}
/**
* Set the clientId property: The Client ID of this relying party application, known as the client_id.
* This setting is required for enabling OpenID Connection authentication with Azure Active Directory or
* other 3rd party OpenID Connect providers.
* More information on OpenID Connect: http://openid.net/specs/openid-connect-core-1_0.html.
*
* @param clientId the clientId value to set.
* @return the AzureActiveDirectoryRegistration object itself.
*/
public AzureActiveDirectoryRegistration withClientId(String clientId) {
this.clientId = clientId;
return this;
}
/**
* Get the clientSecretSettingName property: The app setting name that contains the client secret of the relying
* party application.
*
* @return the clientSecretSettingName value.
*/
public String clientSecretSettingName() {
return this.clientSecretSettingName;
}
/**
* Set the clientSecretSettingName property: The app setting name that contains the client secret of the relying
* party application.
*
* @param clientSecretSettingName the clientSecretSettingName value to set.
* @return the AzureActiveDirectoryRegistration object itself.
*/
public AzureActiveDirectoryRegistration withClientSecretSettingName(String clientSecretSettingName) {
this.clientSecretSettingName = clientSecretSettingName;
return this;
}
/**
* Get the clientSecretCertificateThumbprint property: An alternative to the client secret, that is the thumbprint
* of a certificate used for signing purposes. This property acts as
* a replacement for the Client Secret. It is also optional.
*
* @return the clientSecretCertificateThumbprint value.
*/
public String clientSecretCertificateThumbprint() {
return this.clientSecretCertificateThumbprint;
}
/**
* Set the clientSecretCertificateThumbprint property: An alternative to the client secret, that is the thumbprint
* of a certificate used for signing purposes. This property acts as
* a replacement for the Client Secret. It is also optional.
*
* @param clientSecretCertificateThumbprint the clientSecretCertificateThumbprint value to set.
* @return the AzureActiveDirectoryRegistration object itself.
*/
public AzureActiveDirectoryRegistration
withClientSecretCertificateThumbprint(String clientSecretCertificateThumbprint) {
this.clientSecretCertificateThumbprint = clientSecretCertificateThumbprint;
return this;
}
/**
* Get the clientSecretCertificateSubjectAlternativeName property: An alternative to the client secret thumbprint,
* that is the subject alternative name of a certificate used for signing purposes. This property acts as
* a replacement for the Client Secret Certificate Thumbprint. It is also optional.
*
* @return the clientSecretCertificateSubjectAlternativeName value.
*/
public String clientSecretCertificateSubjectAlternativeName() {
return this.clientSecretCertificateSubjectAlternativeName;
}
/**
* Set the clientSecretCertificateSubjectAlternativeName property: An alternative to the client secret thumbprint,
* that is the subject alternative name of a certificate used for signing purposes. This property acts as
* a replacement for the Client Secret Certificate Thumbprint. It is also optional.
*
* @param clientSecretCertificateSubjectAlternativeName the clientSecretCertificateSubjectAlternativeName value to
* set.
* @return the AzureActiveDirectoryRegistration object itself.
*/
public AzureActiveDirectoryRegistration
withClientSecretCertificateSubjectAlternativeName(String clientSecretCertificateSubjectAlternativeName) {
this.clientSecretCertificateSubjectAlternativeName = clientSecretCertificateSubjectAlternativeName;
return this;
}
/**
* Get the clientSecretCertificateIssuer property: An alternative to the client secret thumbprint, that is the
* issuer of a certificate used for signing purposes. This property acts as
* a replacement for the Client Secret Certificate Thumbprint. It is also optional.
*
* @return the clientSecretCertificateIssuer value.
*/
public String clientSecretCertificateIssuer() {
return this.clientSecretCertificateIssuer;
}
/**
* Set the clientSecretCertificateIssuer property: An alternative to the client secret thumbprint, that is the
* issuer of a certificate used for signing purposes. This property acts as
* a replacement for the Client Secret Certificate Thumbprint. It is also optional.
*
* @param clientSecretCertificateIssuer the clientSecretCertificateIssuer value to set.
* @return the AzureActiveDirectoryRegistration object itself.
*/
public AzureActiveDirectoryRegistration withClientSecretCertificateIssuer(String clientSecretCertificateIssuer) {
this.clientSecretCertificateIssuer = clientSecretCertificateIssuer;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("openIdIssuer", this.openIdIssuer);
jsonWriter.writeStringField("clientId", this.clientId);
jsonWriter.writeStringField("clientSecretSettingName", this.clientSecretSettingName);
jsonWriter.writeStringField("clientSecretCertificateThumbprint", this.clientSecretCertificateThumbprint);
jsonWriter.writeStringField("clientSecretCertificateSubjectAlternativeName",
this.clientSecretCertificateSubjectAlternativeName);
jsonWriter.writeStringField("clientSecretCertificateIssuer", this.clientSecretCertificateIssuer);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AzureActiveDirectoryRegistration from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AzureActiveDirectoryRegistration if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the AzureActiveDirectoryRegistration.
*/
public static AzureActiveDirectoryRegistration fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AzureActiveDirectoryRegistration deserializedAzureActiveDirectoryRegistration
= new AzureActiveDirectoryRegistration();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("openIdIssuer".equals(fieldName)) {
deserializedAzureActiveDirectoryRegistration.openIdIssuer = reader.getString();
} else if ("clientId".equals(fieldName)) {
deserializedAzureActiveDirectoryRegistration.clientId = reader.getString();
} else if ("clientSecretSettingName".equals(fieldName)) {
deserializedAzureActiveDirectoryRegistration.clientSecretSettingName = reader.getString();
} else if ("clientSecretCertificateThumbprint".equals(fieldName)) {
deserializedAzureActiveDirectoryRegistration.clientSecretCertificateThumbprint = reader.getString();
} else if ("clientSecretCertificateSubjectAlternativeName".equals(fieldName)) {
deserializedAzureActiveDirectoryRegistration.clientSecretCertificateSubjectAlternativeName
= reader.getString();
} else if ("clientSecretCertificateIssuer".equals(fieldName)) {
deserializedAzureActiveDirectoryRegistration.clientSecretCertificateIssuer = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedAzureActiveDirectoryRegistration;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy