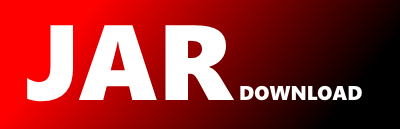
com.azure.resourcemanager.appcontainers.models.HeaderMatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-appcontainers Show documentation
Show all versions of azure-resourcemanager-appcontainers Show documentation
This package contains Microsoft Azure SDK for ContainerAppsApi Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-03.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.appcontainers.fluent.models.HeaderMatchMatch;
import java.io.IOException;
/**
* Conditions required to match a header.
*/
@Fluent
public final class HeaderMatch implements JsonSerializable {
/*
* Name of the header
*/
private String headerProperty;
/*
* Type of match to perform
*/
private HeaderMatchMatch innerMatch;
/**
* Creates an instance of HeaderMatch class.
*/
public HeaderMatch() {
}
/**
* Get the headerProperty property: Name of the header.
*
* @return the headerProperty value.
*/
public String headerProperty() {
return this.headerProperty;
}
/**
* Set the headerProperty property: Name of the header.
*
* @param headerProperty the headerProperty value to set.
* @return the HeaderMatch object itself.
*/
public HeaderMatch withHeaderProperty(String headerProperty) {
this.headerProperty = headerProperty;
return this;
}
/**
* Get the innerMatch property: Type of match to perform.
*
* @return the innerMatch value.
*/
private HeaderMatchMatch innerMatch() {
return this.innerMatch;
}
/**
* Get the exactMatch property: Exact value of the header.
*
* @return the exactMatch value.
*/
public String exactMatch() {
return this.innerMatch() == null ? null : this.innerMatch().exactMatch();
}
/**
* Set the exactMatch property: Exact value of the header.
*
* @param exactMatch the exactMatch value to set.
* @return the HeaderMatch object itself.
*/
public HeaderMatch withExactMatch(String exactMatch) {
if (this.innerMatch() == null) {
this.innerMatch = new HeaderMatchMatch();
}
this.innerMatch().withExactMatch(exactMatch);
return this;
}
/**
* Get the prefixMatch property: Prefix value of the header.
*
* @return the prefixMatch value.
*/
public String prefixMatch() {
return this.innerMatch() == null ? null : this.innerMatch().prefixMatch();
}
/**
* Set the prefixMatch property: Prefix value of the header.
*
* @param prefixMatch the prefixMatch value to set.
* @return the HeaderMatch object itself.
*/
public HeaderMatch withPrefixMatch(String prefixMatch) {
if (this.innerMatch() == null) {
this.innerMatch = new HeaderMatchMatch();
}
this.innerMatch().withPrefixMatch(prefixMatch);
return this;
}
/**
* Get the suffixMatch property: Suffix value of the header.
*
* @return the suffixMatch value.
*/
public String suffixMatch() {
return this.innerMatch() == null ? null : this.innerMatch().suffixMatch();
}
/**
* Set the suffixMatch property: Suffix value of the header.
*
* @param suffixMatch the suffixMatch value to set.
* @return the HeaderMatch object itself.
*/
public HeaderMatch withSuffixMatch(String suffixMatch) {
if (this.innerMatch() == null) {
this.innerMatch = new HeaderMatchMatch();
}
this.innerMatch().withSuffixMatch(suffixMatch);
return this;
}
/**
* Get the regexMatch property: Regex value of the header.
*
* @return the regexMatch value.
*/
public String regexMatch() {
return this.innerMatch() == null ? null : this.innerMatch().regexMatch();
}
/**
* Set the regexMatch property: Regex value of the header.
*
* @param regexMatch the regexMatch value to set.
* @return the HeaderMatch object itself.
*/
public HeaderMatch withRegexMatch(String regexMatch) {
if (this.innerMatch() == null) {
this.innerMatch = new HeaderMatchMatch();
}
this.innerMatch().withRegexMatch(regexMatch);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (innerMatch() != null) {
innerMatch().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("header", this.headerProperty);
jsonWriter.writeJsonField("match", this.innerMatch);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of HeaderMatch from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of HeaderMatch if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the HeaderMatch.
*/
public static HeaderMatch fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
HeaderMatch deserializedHeaderMatch = new HeaderMatch();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("header".equals(fieldName)) {
deserializedHeaderMatch.headerProperty = reader.getString();
} else if ("match".equals(fieldName)) {
deserializedHeaderMatch.innerMatch = HeaderMatchMatch.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedHeaderMatch;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy