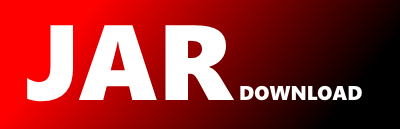
com.azure.resourcemanager.appcontainers.models.HttpRetryPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-appcontainers Show documentation
Show all versions of azure-resourcemanager-appcontainers Show documentation
This package contains Microsoft Azure SDK for ContainerAppsApi Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-03.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.appcontainers.fluent.models.HttpRetryPolicyMatches;
import com.azure.resourcemanager.appcontainers.fluent.models.HttpRetryPolicyRetryBackOff;
import java.io.IOException;
import java.util.List;
/**
* Policy that defines http request retry conditions.
*/
@Fluent
public final class HttpRetryPolicy implements JsonSerializable {
/*
* Maximum number of times a request will retry
*/
private Integer maxRetries;
/*
* Settings for retry backoff characteristics
*/
private HttpRetryPolicyRetryBackOff innerRetryBackOff;
/*
* Conditions that must be met for a request to be retried
*/
private HttpRetryPolicyMatches innerMatches;
/**
* Creates an instance of HttpRetryPolicy class.
*/
public HttpRetryPolicy() {
}
/**
* Get the maxRetries property: Maximum number of times a request will retry.
*
* @return the maxRetries value.
*/
public Integer maxRetries() {
return this.maxRetries;
}
/**
* Set the maxRetries property: Maximum number of times a request will retry.
*
* @param maxRetries the maxRetries value to set.
* @return the HttpRetryPolicy object itself.
*/
public HttpRetryPolicy withMaxRetries(Integer maxRetries) {
this.maxRetries = maxRetries;
return this;
}
/**
* Get the innerRetryBackOff property: Settings for retry backoff characteristics.
*
* @return the innerRetryBackOff value.
*/
private HttpRetryPolicyRetryBackOff innerRetryBackOff() {
return this.innerRetryBackOff;
}
/**
* Get the innerMatches property: Conditions that must be met for a request to be retried.
*
* @return the innerMatches value.
*/
private HttpRetryPolicyMatches innerMatches() {
return this.innerMatches;
}
/**
* Get the initialDelayInMilliseconds property: Initial delay, in milliseconds, before retrying a request.
*
* @return the initialDelayInMilliseconds value.
*/
public Long initialDelayInMilliseconds() {
return this.innerRetryBackOff() == null ? null : this.innerRetryBackOff().initialDelayInMilliseconds();
}
/**
* Set the initialDelayInMilliseconds property: Initial delay, in milliseconds, before retrying a request.
*
* @param initialDelayInMilliseconds the initialDelayInMilliseconds value to set.
* @return the HttpRetryPolicy object itself.
*/
public HttpRetryPolicy withInitialDelayInMilliseconds(Long initialDelayInMilliseconds) {
if (this.innerRetryBackOff() == null) {
this.innerRetryBackOff = new HttpRetryPolicyRetryBackOff();
}
this.innerRetryBackOff().withInitialDelayInMilliseconds(initialDelayInMilliseconds);
return this;
}
/**
* Get the maxIntervalInMilliseconds property: Maximum interval, in milliseconds, between retries.
*
* @return the maxIntervalInMilliseconds value.
*/
public Long maxIntervalInMilliseconds() {
return this.innerRetryBackOff() == null ? null : this.innerRetryBackOff().maxIntervalInMilliseconds();
}
/**
* Set the maxIntervalInMilliseconds property: Maximum interval, in milliseconds, between retries.
*
* @param maxIntervalInMilliseconds the maxIntervalInMilliseconds value to set.
* @return the HttpRetryPolicy object itself.
*/
public HttpRetryPolicy withMaxIntervalInMilliseconds(Long maxIntervalInMilliseconds) {
if (this.innerRetryBackOff() == null) {
this.innerRetryBackOff = new HttpRetryPolicyRetryBackOff();
}
this.innerRetryBackOff().withMaxIntervalInMilliseconds(maxIntervalInMilliseconds);
return this;
}
/**
* Get the headers property: Headers that must be present for a request to be retried.
*
* @return the headers value.
*/
public List headers() {
return this.innerMatches() == null ? null : this.innerMatches().headers();
}
/**
* Set the headers property: Headers that must be present for a request to be retried.
*
* @param headers the headers value to set.
* @return the HttpRetryPolicy object itself.
*/
public HttpRetryPolicy withHeaders(List headers) {
if (this.innerMatches() == null) {
this.innerMatches = new HttpRetryPolicyMatches();
}
this.innerMatches().withHeaders(headers);
return this;
}
/**
* Get the httpStatusCodes property: Additional http status codes that can trigger a retry.
*
* @return the httpStatusCodes value.
*/
public List httpStatusCodes() {
return this.innerMatches() == null ? null : this.innerMatches().httpStatusCodes();
}
/**
* Set the httpStatusCodes property: Additional http status codes that can trigger a retry.
*
* @param httpStatusCodes the httpStatusCodes value to set.
* @return the HttpRetryPolicy object itself.
*/
public HttpRetryPolicy withHttpStatusCodes(List httpStatusCodes) {
if (this.innerMatches() == null) {
this.innerMatches = new HttpRetryPolicyMatches();
}
this.innerMatches().withHttpStatusCodes(httpStatusCodes);
return this;
}
/**
* Get the errors property: Errors that can trigger a retry.
*
* @return the errors value.
*/
public List errors() {
return this.innerMatches() == null ? null : this.innerMatches().errors();
}
/**
* Set the errors property: Errors that can trigger a retry.
*
* @param errors the errors value to set.
* @return the HttpRetryPolicy object itself.
*/
public HttpRetryPolicy withErrors(List errors) {
if (this.innerMatches() == null) {
this.innerMatches = new HttpRetryPolicyMatches();
}
this.innerMatches().withErrors(errors);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (innerRetryBackOff() != null) {
innerRetryBackOff().validate();
}
if (innerMatches() != null) {
innerMatches().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeNumberField("maxRetries", this.maxRetries);
jsonWriter.writeJsonField("retryBackOff", this.innerRetryBackOff);
jsonWriter.writeJsonField("matches", this.innerMatches);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of HttpRetryPolicy from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of HttpRetryPolicy if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the HttpRetryPolicy.
*/
public static HttpRetryPolicy fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
HttpRetryPolicy deserializedHttpRetryPolicy = new HttpRetryPolicy();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("maxRetries".equals(fieldName)) {
deserializedHttpRetryPolicy.maxRetries = reader.getNullable(JsonReader::getInt);
} else if ("retryBackOff".equals(fieldName)) {
deserializedHttpRetryPolicy.innerRetryBackOff = HttpRetryPolicyRetryBackOff.fromJson(reader);
} else if ("matches".equals(fieldName)) {
deserializedHttpRetryPolicy.innerMatches = HttpRetryPolicyMatches.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedHttpRetryPolicy;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy