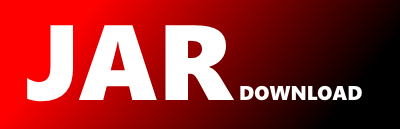
com.azure.resourcemanager.appcontainers.models.JobConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-appcontainers Show documentation
Show all versions of azure-resourcemanager-appcontainers Show documentation
This package contains Microsoft Azure SDK for ContainerAppsApi Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-03.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* Non versioned Container Apps Job configuration properties.
*/
@Fluent
public final class JobConfiguration implements JsonSerializable {
/*
* Collection of secrets used by a Container Apps Job
*/
private List secrets;
/*
* Trigger type of the job
*/
private TriggerType triggerType;
/*
* Maximum number of seconds a replica is allowed to run.
*/
private int replicaTimeout;
/*
* Maximum number of retries before failing the job.
*/
private Integer replicaRetryLimit;
/*
* Manual trigger configuration for a single execution job. Properties replicaCompletionCount and parallelism would
* be set to 1 by default
*/
private JobConfigurationManualTriggerConfig manualTriggerConfig;
/*
* Cron formatted repeating trigger schedule ("* * * * *") for cronjobs. Properties completions and parallelism
* would be set to 1 by default
*/
private JobConfigurationScheduleTriggerConfig scheduleTriggerConfig;
/*
* Trigger configuration of an event driven job.
*/
private JobConfigurationEventTriggerConfig eventTriggerConfig;
/*
* Collection of private container registry credentials used by a Container apps job
*/
private List registries;
/*
* Optional settings for Managed Identities that are assigned to the Container App Job. If a Managed Identity is not
* specified here, default settings will be used.
*/
private List identitySettings;
/**
* Creates an instance of JobConfiguration class.
*/
public JobConfiguration() {
}
/**
* Get the secrets property: Collection of secrets used by a Container Apps Job.
*
* @return the secrets value.
*/
public List secrets() {
return this.secrets;
}
/**
* Set the secrets property: Collection of secrets used by a Container Apps Job.
*
* @param secrets the secrets value to set.
* @return the JobConfiguration object itself.
*/
public JobConfiguration withSecrets(List secrets) {
this.secrets = secrets;
return this;
}
/**
* Get the triggerType property: Trigger type of the job.
*
* @return the triggerType value.
*/
public TriggerType triggerType() {
return this.triggerType;
}
/**
* Set the triggerType property: Trigger type of the job.
*
* @param triggerType the triggerType value to set.
* @return the JobConfiguration object itself.
*/
public JobConfiguration withTriggerType(TriggerType triggerType) {
this.triggerType = triggerType;
return this;
}
/**
* Get the replicaTimeout property: Maximum number of seconds a replica is allowed to run.
*
* @return the replicaTimeout value.
*/
public int replicaTimeout() {
return this.replicaTimeout;
}
/**
* Set the replicaTimeout property: Maximum number of seconds a replica is allowed to run.
*
* @param replicaTimeout the replicaTimeout value to set.
* @return the JobConfiguration object itself.
*/
public JobConfiguration withReplicaTimeout(int replicaTimeout) {
this.replicaTimeout = replicaTimeout;
return this;
}
/**
* Get the replicaRetryLimit property: Maximum number of retries before failing the job.
*
* @return the replicaRetryLimit value.
*/
public Integer replicaRetryLimit() {
return this.replicaRetryLimit;
}
/**
* Set the replicaRetryLimit property: Maximum number of retries before failing the job.
*
* @param replicaRetryLimit the replicaRetryLimit value to set.
* @return the JobConfiguration object itself.
*/
public JobConfiguration withReplicaRetryLimit(Integer replicaRetryLimit) {
this.replicaRetryLimit = replicaRetryLimit;
return this;
}
/**
* Get the manualTriggerConfig property: Manual trigger configuration for a single execution job. Properties
* replicaCompletionCount and parallelism would be set to 1 by default.
*
* @return the manualTriggerConfig value.
*/
public JobConfigurationManualTriggerConfig manualTriggerConfig() {
return this.manualTriggerConfig;
}
/**
* Set the manualTriggerConfig property: Manual trigger configuration for a single execution job. Properties
* replicaCompletionCount and parallelism would be set to 1 by default.
*
* @param manualTriggerConfig the manualTriggerConfig value to set.
* @return the JobConfiguration object itself.
*/
public JobConfiguration withManualTriggerConfig(JobConfigurationManualTriggerConfig manualTriggerConfig) {
this.manualTriggerConfig = manualTriggerConfig;
return this;
}
/**
* Get the scheduleTriggerConfig property: Cron formatted repeating trigger schedule ("* * * * *") for cronjobs.
* Properties completions and parallelism would be set to 1 by default.
*
* @return the scheduleTriggerConfig value.
*/
public JobConfigurationScheduleTriggerConfig scheduleTriggerConfig() {
return this.scheduleTriggerConfig;
}
/**
* Set the scheduleTriggerConfig property: Cron formatted repeating trigger schedule ("* * * * *") for cronjobs.
* Properties completions and parallelism would be set to 1 by default.
*
* @param scheduleTriggerConfig the scheduleTriggerConfig value to set.
* @return the JobConfiguration object itself.
*/
public JobConfiguration withScheduleTriggerConfig(JobConfigurationScheduleTriggerConfig scheduleTriggerConfig) {
this.scheduleTriggerConfig = scheduleTriggerConfig;
return this;
}
/**
* Get the eventTriggerConfig property: Trigger configuration of an event driven job.
*
* @return the eventTriggerConfig value.
*/
public JobConfigurationEventTriggerConfig eventTriggerConfig() {
return this.eventTriggerConfig;
}
/**
* Set the eventTriggerConfig property: Trigger configuration of an event driven job.
*
* @param eventTriggerConfig the eventTriggerConfig value to set.
* @return the JobConfiguration object itself.
*/
public JobConfiguration withEventTriggerConfig(JobConfigurationEventTriggerConfig eventTriggerConfig) {
this.eventTriggerConfig = eventTriggerConfig;
return this;
}
/**
* Get the registries property: Collection of private container registry credentials used by a Container apps job.
*
* @return the registries value.
*/
public List registries() {
return this.registries;
}
/**
* Set the registries property: Collection of private container registry credentials used by a Container apps job.
*
* @param registries the registries value to set.
* @return the JobConfiguration object itself.
*/
public JobConfiguration withRegistries(List registries) {
this.registries = registries;
return this;
}
/**
* Get the identitySettings property: Optional settings for Managed Identities that are assigned to the Container
* App Job. If a Managed Identity is not specified here, default settings will be used.
*
* @return the identitySettings value.
*/
public List identitySettings() {
return this.identitySettings;
}
/**
* Set the identitySettings property: Optional settings for Managed Identities that are assigned to the Container
* App Job. If a Managed Identity is not specified here, default settings will be used.
*
* @param identitySettings the identitySettings value to set.
* @return the JobConfiguration object itself.
*/
public JobConfiguration withIdentitySettings(List identitySettings) {
this.identitySettings = identitySettings;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (secrets() != null) {
secrets().forEach(e -> e.validate());
}
if (triggerType() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException("Missing required property triggerType in model JobConfiguration"));
}
if (manualTriggerConfig() != null) {
manualTriggerConfig().validate();
}
if (scheduleTriggerConfig() != null) {
scheduleTriggerConfig().validate();
}
if (eventTriggerConfig() != null) {
eventTriggerConfig().validate();
}
if (registries() != null) {
registries().forEach(e -> e.validate());
}
if (identitySettings() != null) {
identitySettings().forEach(e -> e.validate());
}
}
private static final ClientLogger LOGGER = new ClientLogger(JobConfiguration.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("triggerType", this.triggerType == null ? null : this.triggerType.toString());
jsonWriter.writeIntField("replicaTimeout", this.replicaTimeout);
jsonWriter.writeArrayField("secrets", this.secrets, (writer, element) -> writer.writeJson(element));
jsonWriter.writeNumberField("replicaRetryLimit", this.replicaRetryLimit);
jsonWriter.writeJsonField("manualTriggerConfig", this.manualTriggerConfig);
jsonWriter.writeJsonField("scheduleTriggerConfig", this.scheduleTriggerConfig);
jsonWriter.writeJsonField("eventTriggerConfig", this.eventTriggerConfig);
jsonWriter.writeArrayField("registries", this.registries, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("identitySettings", this.identitySettings,
(writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of JobConfiguration from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of JobConfiguration if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the JobConfiguration.
*/
public static JobConfiguration fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
JobConfiguration deserializedJobConfiguration = new JobConfiguration();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("triggerType".equals(fieldName)) {
deserializedJobConfiguration.triggerType = TriggerType.fromString(reader.getString());
} else if ("replicaTimeout".equals(fieldName)) {
deserializedJobConfiguration.replicaTimeout = reader.getInt();
} else if ("secrets".equals(fieldName)) {
List secrets = reader.readArray(reader1 -> Secret.fromJson(reader1));
deserializedJobConfiguration.secrets = secrets;
} else if ("replicaRetryLimit".equals(fieldName)) {
deserializedJobConfiguration.replicaRetryLimit = reader.getNullable(JsonReader::getInt);
} else if ("manualTriggerConfig".equals(fieldName)) {
deserializedJobConfiguration.manualTriggerConfig
= JobConfigurationManualTriggerConfig.fromJson(reader);
} else if ("scheduleTriggerConfig".equals(fieldName)) {
deserializedJobConfiguration.scheduleTriggerConfig
= JobConfigurationScheduleTriggerConfig.fromJson(reader);
} else if ("eventTriggerConfig".equals(fieldName)) {
deserializedJobConfiguration.eventTriggerConfig
= JobConfigurationEventTriggerConfig.fromJson(reader);
} else if ("registries".equals(fieldName)) {
List registries
= reader.readArray(reader1 -> RegistryCredentials.fromJson(reader1));
deserializedJobConfiguration.registries = registries;
} else if ("identitySettings".equals(fieldName)) {
List identitySettings
= reader.readArray(reader1 -> IdentitySettings.fromJson(reader1));
deserializedJobConfiguration.identitySettings = identitySettings;
} else {
reader.skipChildren();
}
}
return deserializedJobConfiguration;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy