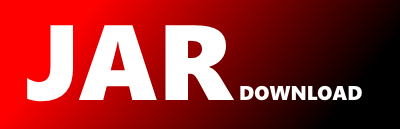
com.azure.resourcemanager.appcontainers.models.SpringCloudEurekaComponent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-appcontainers Show documentation
Show all versions of azure-resourcemanager-appcontainers Show documentation
This package contains Microsoft Azure SDK for ContainerAppsApi Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-03.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* Spring Cloud Eureka properties.
*/
@Fluent
public final class SpringCloudEurekaComponent extends JavaComponentProperties {
/*
* Type of the Java Component.
*/
private JavaComponentType componentType = JavaComponentType.SPRING_CLOUD_EUREKA;
/*
* Java Component Ingress configurations.
*/
private JavaComponentIngress ingress;
/*
* Provisioning state of the Java Component.
*/
private JavaComponentProvisioningState provisioningState;
/**
* Creates an instance of SpringCloudEurekaComponent class.
*/
public SpringCloudEurekaComponent() {
}
/**
* Get the componentType property: Type of the Java Component.
*
* @return the componentType value.
*/
@Override
public JavaComponentType componentType() {
return this.componentType;
}
/**
* Get the ingress property: Java Component Ingress configurations.
*
* @return the ingress value.
*/
public JavaComponentIngress ingress() {
return this.ingress;
}
/**
* Set the ingress property: Java Component Ingress configurations.
*
* @param ingress the ingress value to set.
* @return the SpringCloudEurekaComponent object itself.
*/
public SpringCloudEurekaComponent withIngress(JavaComponentIngress ingress) {
this.ingress = ingress;
return this;
}
/**
* Get the provisioningState property: Provisioning state of the Java Component.
*
* @return the provisioningState value.
*/
@Override
public JavaComponentProvisioningState provisioningState() {
return this.provisioningState;
}
/**
* {@inheritDoc}
*/
@Override
public SpringCloudEurekaComponent withConfigurations(List configurations) {
super.withConfigurations(configurations);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public SpringCloudEurekaComponent withScale(JavaComponentPropertiesScale scale) {
super.withScale(scale);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public SpringCloudEurekaComponent withServiceBinds(List serviceBinds) {
super.withServiceBinds(serviceBinds);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
if (ingress() != null) {
ingress().validate();
}
if (configurations() != null) {
configurations().forEach(e -> e.validate());
}
if (scale() != null) {
scale().validate();
}
if (serviceBinds() != null) {
serviceBinds().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeArrayField("configurations", configurations(), (writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("scale", scale());
jsonWriter.writeArrayField("serviceBinds", serviceBinds(), (writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("componentType", this.componentType == null ? null : this.componentType.toString());
jsonWriter.writeJsonField("ingress", this.ingress);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of SpringCloudEurekaComponent from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of SpringCloudEurekaComponent if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IOException If an error occurs while reading the SpringCloudEurekaComponent.
*/
public static SpringCloudEurekaComponent fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
SpringCloudEurekaComponent deserializedSpringCloudEurekaComponent = new SpringCloudEurekaComponent();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("provisioningState".equals(fieldName)) {
deserializedSpringCloudEurekaComponent.provisioningState
= JavaComponentProvisioningState.fromString(reader.getString());
} else if ("configurations".equals(fieldName)) {
List configurations
= reader.readArray(reader1 -> JavaComponentConfigurationProperty.fromJson(reader1));
deserializedSpringCloudEurekaComponent.withConfigurations(configurations);
} else if ("scale".equals(fieldName)) {
deserializedSpringCloudEurekaComponent.withScale(JavaComponentPropertiesScale.fromJson(reader));
} else if ("serviceBinds".equals(fieldName)) {
List serviceBinds
= reader.readArray(reader1 -> JavaComponentServiceBind.fromJson(reader1));
deserializedSpringCloudEurekaComponent.withServiceBinds(serviceBinds);
} else if ("componentType".equals(fieldName)) {
deserializedSpringCloudEurekaComponent.componentType
= JavaComponentType.fromString(reader.getString());
} else if ("ingress".equals(fieldName)) {
deserializedSpringCloudEurekaComponent.ingress = JavaComponentIngress.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedSpringCloudEurekaComponent;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy