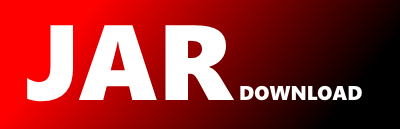
com.azure.resourcemanager.appcontainers.models.Template Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-appcontainers Show documentation
Show all versions of azure-resourcemanager-appcontainers Show documentation
This package contains Microsoft Azure SDK for ContainerAppsApi Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Package tag package-2024-03.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.appcontainers.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* Container App versioned application definition.
* Defines the desired state of an immutable revision.
* Any changes to this section Will result in a new revision being created.
*/
@Fluent
public final class Template implements JsonSerializable {
/*
* User friendly suffix that is appended to the revision name
*/
private String revisionSuffix;
/*
* Optional duration in seconds the Container App Instance needs to terminate gracefully. Value must be non-negative
* integer. The value zero indicates stop immediately via the kill signal (no opportunity to shut down). If this
* value is nil, the default grace period will be used instead. Set this value longer than the expected cleanup time
* for your process. Defaults to 30 seconds.
*/
private Long terminationGracePeriodSeconds;
/*
* List of specialized containers that run before app containers.
*/
private List initContainers;
/*
* List of container definitions for the Container App.
*/
private List containers;
/*
* Scaling properties for the Container App.
*/
private Scale scale;
/*
* List of volume definitions for the Container App.
*/
private List volumes;
/*
* List of container app services bound to the app
*/
private List serviceBinds;
/**
* Creates an instance of Template class.
*/
public Template() {
}
/**
* Get the revisionSuffix property: User friendly suffix that is appended to the revision name.
*
* @return the revisionSuffix value.
*/
public String revisionSuffix() {
return this.revisionSuffix;
}
/**
* Set the revisionSuffix property: User friendly suffix that is appended to the revision name.
*
* @param revisionSuffix the revisionSuffix value to set.
* @return the Template object itself.
*/
public Template withRevisionSuffix(String revisionSuffix) {
this.revisionSuffix = revisionSuffix;
return this;
}
/**
* Get the terminationGracePeriodSeconds property: Optional duration in seconds the Container App Instance needs to
* terminate gracefully. Value must be non-negative integer. The value zero indicates stop immediately via the kill
* signal (no opportunity to shut down). If this value is nil, the default grace period will be used instead. Set
* this value longer than the expected cleanup time for your process. Defaults to 30 seconds.
*
* @return the terminationGracePeriodSeconds value.
*/
public Long terminationGracePeriodSeconds() {
return this.terminationGracePeriodSeconds;
}
/**
* Set the terminationGracePeriodSeconds property: Optional duration in seconds the Container App Instance needs to
* terminate gracefully. Value must be non-negative integer. The value zero indicates stop immediately via the kill
* signal (no opportunity to shut down). If this value is nil, the default grace period will be used instead. Set
* this value longer than the expected cleanup time for your process. Defaults to 30 seconds.
*
* @param terminationGracePeriodSeconds the terminationGracePeriodSeconds value to set.
* @return the Template object itself.
*/
public Template withTerminationGracePeriodSeconds(Long terminationGracePeriodSeconds) {
this.terminationGracePeriodSeconds = terminationGracePeriodSeconds;
return this;
}
/**
* Get the initContainers property: List of specialized containers that run before app containers.
*
* @return the initContainers value.
*/
public List initContainers() {
return this.initContainers;
}
/**
* Set the initContainers property: List of specialized containers that run before app containers.
*
* @param initContainers the initContainers value to set.
* @return the Template object itself.
*/
public Template withInitContainers(List initContainers) {
this.initContainers = initContainers;
return this;
}
/**
* Get the containers property: List of container definitions for the Container App.
*
* @return the containers value.
*/
public List containers() {
return this.containers;
}
/**
* Set the containers property: List of container definitions for the Container App.
*
* @param containers the containers value to set.
* @return the Template object itself.
*/
public Template withContainers(List containers) {
this.containers = containers;
return this;
}
/**
* Get the scale property: Scaling properties for the Container App.
*
* @return the scale value.
*/
public Scale scale() {
return this.scale;
}
/**
* Set the scale property: Scaling properties for the Container App.
*
* @param scale the scale value to set.
* @return the Template object itself.
*/
public Template withScale(Scale scale) {
this.scale = scale;
return this;
}
/**
* Get the volumes property: List of volume definitions for the Container App.
*
* @return the volumes value.
*/
public List volumes() {
return this.volumes;
}
/**
* Set the volumes property: List of volume definitions for the Container App.
*
* @param volumes the volumes value to set.
* @return the Template object itself.
*/
public Template withVolumes(List volumes) {
this.volumes = volumes;
return this;
}
/**
* Get the serviceBinds property: List of container app services bound to the app.
*
* @return the serviceBinds value.
*/
public List serviceBinds() {
return this.serviceBinds;
}
/**
* Set the serviceBinds property: List of container app services bound to the app.
*
* @param serviceBinds the serviceBinds value to set.
* @return the Template object itself.
*/
public Template withServiceBinds(List serviceBinds) {
this.serviceBinds = serviceBinds;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (initContainers() != null) {
initContainers().forEach(e -> e.validate());
}
if (containers() != null) {
containers().forEach(e -> e.validate());
}
if (scale() != null) {
scale().validate();
}
if (volumes() != null) {
volumes().forEach(e -> e.validate());
}
if (serviceBinds() != null) {
serviceBinds().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("revisionSuffix", this.revisionSuffix);
jsonWriter.writeNumberField("terminationGracePeriodSeconds", this.terminationGracePeriodSeconds);
jsonWriter.writeArrayField("initContainers", this.initContainers,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("containers", this.containers, (writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("scale", this.scale);
jsonWriter.writeArrayField("volumes", this.volumes, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("serviceBinds", this.serviceBinds, (writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of Template from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of Template if the JsonReader was pointing to an instance of it, or null if it was pointing
* to JSON null.
* @throws IOException If an error occurs while reading the Template.
*/
public static Template fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
Template deserializedTemplate = new Template();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("revisionSuffix".equals(fieldName)) {
deserializedTemplate.revisionSuffix = reader.getString();
} else if ("terminationGracePeriodSeconds".equals(fieldName)) {
deserializedTemplate.terminationGracePeriodSeconds = reader.getNullable(JsonReader::getLong);
} else if ("initContainers".equals(fieldName)) {
List initContainers = reader.readArray(reader1 -> InitContainer.fromJson(reader1));
deserializedTemplate.initContainers = initContainers;
} else if ("containers".equals(fieldName)) {
List containers = reader.readArray(reader1 -> Container.fromJson(reader1));
deserializedTemplate.containers = containers;
} else if ("scale".equals(fieldName)) {
deserializedTemplate.scale = Scale.fromJson(reader);
} else if ("volumes".equals(fieldName)) {
List volumes = reader.readArray(reader1 -> Volume.fromJson(reader1));
deserializedTemplate.volumes = volumes;
} else if ("serviceBinds".equals(fieldName)) {
List serviceBinds = reader.readArray(reader1 -> ServiceBind.fromJson(reader1));
deserializedTemplate.serviceBinds = serviceBinds;
} else {
reader.skipChildren();
}
}
return deserializedTemplate;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy