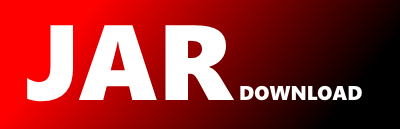
com.azure.resourcemanager.authorization.fluent.models.MicrosoftGraphApplicationInner Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.authorization.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.Base64Url;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.UUID;
/**
* application
*
* Represents an Azure Active Directory object. The directoryObject type is the base type for many other directory
* entity types.
*/
@Fluent
public final class MicrosoftGraphApplicationInner extends MicrosoftGraphDirectoryObjectInner {
private static final byte[] EMPTY_BYTE_ARRAY = new byte[0];
/*
* Defines custom behavior that a consuming service can use to call an app in specific contexts. For example,
* applications that can render file streams may set the addIns property for its 'FileHandler' functionality. This
* will let services like Microsoft 365 call the application in the context of a document the user is working on.
*/
private List addIns;
/*
* apiApplication
*/
private MicrosoftGraphApiApplication api;
/*
* The unique identifier for the application that is assigned to an application by Azure AD. Not nullable.
* Read-only.
*/
private String appId;
/*
* The applicationTemplateId property.
*/
private String applicationTemplateId;
/*
* The collection of roles the application declares. With app role assignments, these roles can be assigned to
* users, groups, or other applications' service principals. Not nullable.
*/
private List appRoles;
/*
* The date and time the application was registered. Read-only.
*/
private OffsetDateTime createdDateTime;
/*
* The description property.
*/
private String description;
/*
* The display name for the application.
*/
private String displayName;
/*
* Configures the groups claim issued in a user or OAuth 2.0 access token that the application expects. To set this
* attribute, use one of the following valid string values:NoneSecurityGroup: For security groups and Azure AD
* rolesAll: This will get all of the security groups, distribution groups, and Azure AD directory roles that the
* signed-in user is a member of
*/
private String groupMembershipClaims;
/*
* The URIs that identify the application within its Azure AD tenant, or within a verified custom domain if the
* application is multi-tenant. For more information see Application Objects and Service Principal Objects. The any
* operator is required for filter expressions on multi-valued properties. Not nullable.
*/
private List identifierUris;
/*
* informationalUrl
*/
private MicrosoftGraphInformationalUrl info;
/*
* The isDeviceOnlyAuthSupported property.
*/
private Boolean isDeviceOnlyAuthSupported;
/*
* Specifies the fallback application type as public client, such as an installed application running on a mobile
* device. The default value is false which means the fallback application type is confidential client such as web
* app. There are certain scenarios where Azure AD cannot determine the client application type (e.g. ROPC flow
* where it is configured without specifying a redirect URI). In those cases Azure AD will interpret the application
* type based on the value of this property.
*/
private Boolean isFallbackPublicClient;
/*
* The collection of key credentials associated with the application Not nullable.
*/
private List keyCredentials;
/*
* The main logo for the application. Not nullable.
*/
private Base64Url logo;
/*
* The notes property.
*/
private String notes;
/*
* The oauth2RequirePostResponse property.
*/
private Boolean oauth2RequirePostResponse;
/*
* optionalClaims
*/
private MicrosoftGraphOptionalClaims optionalClaims;
/*
* parentalControlSettings
*/
private MicrosoftGraphParentalControlSettings parentalControlSettings;
/*
* The collection of password credentials associated with the application. Not nullable.
*/
private List passwordCredentials;
/*
* publicClientApplication
*/
private MicrosoftGraphPublicClientApplication publicClient;
/*
* The verified publisher domain for the application. Read-only.
*/
private String publisherDomain;
/*
* Specifies resources that this application requires access to and the set of OAuth permission scopes and
* application roles that it needs under each of those resources. This pre-configuration of required resource access
* drives the consent experience. Not nullable.
*/
private List requiredResourceAccess;
/*
* Specifies the Microsoft accounts that are supported for the current application. Supported values
* are:AzureADMyOrg: Users with a Microsoft work or school account in my organization’s Azure AD tenant (single
* tenant)AzureADMultipleOrgs: Users with a Microsoft work or school account in any organization’s Azure AD tenant
* (multi-tenant).AzureADandPersonalMicrosoftAccount: Users with a personal Microsoft account, or a work or school
* account in any organization’s Azure AD tenant.PersonalMicrosoftAccount: Users with a personal Microsoft account
* only.For authenticating users with Azure AD B2C user flows, use AzureADandPersonalMicrosoftAccount. This value
* allows for the widest set of user identities including local accounts and user identities from Microsoft,
* Facebook, Google, Twitter, or any OpenID Connect provider.
*/
private String signInAudience;
/*
* Custom strings that can be used to categorize and identify the application. Not nullable.
*/
private List tags;
/*
* Specifies the keyId of a public key from the keyCredentials collection. When configured, Azure AD encrypts all
* the tokens it emits by using the key this property points to. The application code that receives the encrypted
* token must use the matching private key to decrypt the token before it can be used for the signed-in user.
*/
private UUID tokenEncryptionKeyId;
/*
* webApplication
*/
private MicrosoftGraphWebApplication web;
/*
* Represents an Azure Active Directory object. The directoryObject type is the base type for many other directory
* entity types.
*/
private MicrosoftGraphDirectoryObjectInner createdOnBehalfOf;
/*
* Read-only. Nullable.
*/
private List extensionProperties;
/*
* The homeRealmDiscoveryPolicies property.
*/
private List homeRealmDiscoveryPolicies;
/*
* Directory objects that are owners of the application. The owners are a set of non-admin users who are allowed to
* modify this object. Requires version 2013-11-08 or newer. Read-only. Nullable.
*/
private List owners;
/*
* The tokenIssuancePolicies property.
*/
private List tokenIssuancePolicies;
/*
* The tokenLifetimePolicies property.
*/
private List tokenLifetimePolicies;
/*
* Represents an Azure Active Directory object. The directoryObject type is the base type for many other directory
* entity types.
*/
private Map additionalProperties;
/**
* Creates an instance of MicrosoftGraphApplicationInner class.
*/
public MicrosoftGraphApplicationInner() {
}
/**
* Get the addIns property: Defines custom behavior that a consuming service can use to call an app in specific
* contexts. For example, applications that can render file streams may set the addIns property for its
* 'FileHandler' functionality. This will let services like Microsoft 365 call the application in the context of a
* document the user is working on.
*
* @return the addIns value.
*/
public List addIns() {
return this.addIns;
}
/**
* Set the addIns property: Defines custom behavior that a consuming service can use to call an app in specific
* contexts. For example, applications that can render file streams may set the addIns property for its
* 'FileHandler' functionality. This will let services like Microsoft 365 call the application in the context of a
* document the user is working on.
*
* @param addIns the addIns value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withAddIns(List addIns) {
this.addIns = addIns;
return this;
}
/**
* Get the api property: apiApplication.
*
* @return the api value.
*/
public MicrosoftGraphApiApplication api() {
return this.api;
}
/**
* Set the api property: apiApplication.
*
* @param api the api value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withApi(MicrosoftGraphApiApplication api) {
this.api = api;
return this;
}
/**
* Get the appId property: The unique identifier for the application that is assigned to an application by Azure AD.
* Not nullable. Read-only.
*
* @return the appId value.
*/
public String appId() {
return this.appId;
}
/**
* Set the appId property: The unique identifier for the application that is assigned to an application by Azure AD.
* Not nullable. Read-only.
*
* @param appId the appId value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withAppId(String appId) {
this.appId = appId;
return this;
}
/**
* Get the applicationTemplateId property: The applicationTemplateId property.
*
* @return the applicationTemplateId value.
*/
public String applicationTemplateId() {
return this.applicationTemplateId;
}
/**
* Set the applicationTemplateId property: The applicationTemplateId property.
*
* @param applicationTemplateId the applicationTemplateId value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withApplicationTemplateId(String applicationTemplateId) {
this.applicationTemplateId = applicationTemplateId;
return this;
}
/**
* Get the appRoles property: The collection of roles the application declares. With app role assignments, these
* roles can be assigned to users, groups, or other applications' service principals. Not nullable.
*
* @return the appRoles value.
*/
public List appRoles() {
return this.appRoles;
}
/**
* Set the appRoles property: The collection of roles the application declares. With app role assignments, these
* roles can be assigned to users, groups, or other applications' service principals. Not nullable.
*
* @param appRoles the appRoles value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withAppRoles(List appRoles) {
this.appRoles = appRoles;
return this;
}
/**
* Get the createdDateTime property: The date and time the application was registered. Read-only.
*
* @return the createdDateTime value.
*/
public OffsetDateTime createdDateTime() {
return this.createdDateTime;
}
/**
* Set the createdDateTime property: The date and time the application was registered. Read-only.
*
* @param createdDateTime the createdDateTime value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withCreatedDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
return this;
}
/**
* Get the description property: The description property.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Set the description property: The description property.
*
* @param description the description value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withDescription(String description) {
this.description = description;
return this;
}
/**
* Get the displayName property: The display name for the application.
*
* @return the displayName value.
*/
public String displayName() {
return this.displayName;
}
/**
* Set the displayName property: The display name for the application.
*
* @param displayName the displayName value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withDisplayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Get the groupMembershipClaims property: Configures the groups claim issued in a user or OAuth 2.0 access token
* that the application expects. To set this attribute, use one of the following valid string
* values:NoneSecurityGroup: For security groups and Azure AD rolesAll: This will get all of the security groups,
* distribution groups, and Azure AD directory roles that the signed-in user is a member of.
*
* @return the groupMembershipClaims value.
*/
public String groupMembershipClaims() {
return this.groupMembershipClaims;
}
/**
* Set the groupMembershipClaims property: Configures the groups claim issued in a user or OAuth 2.0 access token
* that the application expects. To set this attribute, use one of the following valid string
* values:NoneSecurityGroup: For security groups and Azure AD rolesAll: This will get all of the security groups,
* distribution groups, and Azure AD directory roles that the signed-in user is a member of.
*
* @param groupMembershipClaims the groupMembershipClaims value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withGroupMembershipClaims(String groupMembershipClaims) {
this.groupMembershipClaims = groupMembershipClaims;
return this;
}
/**
* Get the identifierUris property: The URIs that identify the application within its Azure AD tenant, or within a
* verified custom domain if the application is multi-tenant. For more information see Application Objects and
* Service Principal Objects. The any operator is required for filter expressions on multi-valued properties. Not
* nullable.
*
* @return the identifierUris value.
*/
public List identifierUris() {
return this.identifierUris;
}
/**
* Set the identifierUris property: The URIs that identify the application within its Azure AD tenant, or within a
* verified custom domain if the application is multi-tenant. For more information see Application Objects and
* Service Principal Objects. The any operator is required for filter expressions on multi-valued properties. Not
* nullable.
*
* @param identifierUris the identifierUris value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withIdentifierUris(List identifierUris) {
this.identifierUris = identifierUris;
return this;
}
/**
* Get the info property: informationalUrl.
*
* @return the info value.
*/
public MicrosoftGraphInformationalUrl info() {
return this.info;
}
/**
* Set the info property: informationalUrl.
*
* @param info the info value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withInfo(MicrosoftGraphInformationalUrl info) {
this.info = info;
return this;
}
/**
* Get the isDeviceOnlyAuthSupported property: The isDeviceOnlyAuthSupported property.
*
* @return the isDeviceOnlyAuthSupported value.
*/
public Boolean isDeviceOnlyAuthSupported() {
return this.isDeviceOnlyAuthSupported;
}
/**
* Set the isDeviceOnlyAuthSupported property: The isDeviceOnlyAuthSupported property.
*
* @param isDeviceOnlyAuthSupported the isDeviceOnlyAuthSupported value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withIsDeviceOnlyAuthSupported(Boolean isDeviceOnlyAuthSupported) {
this.isDeviceOnlyAuthSupported = isDeviceOnlyAuthSupported;
return this;
}
/**
* Get the isFallbackPublicClient property: Specifies the fallback application type as public client, such as an
* installed application running on a mobile device. The default value is false which means the fallback application
* type is confidential client such as web app. There are certain scenarios where Azure AD cannot determine the
* client application type (e.g. ROPC flow where it is configured without specifying a redirect URI). In those cases
* Azure AD will interpret the application type based on the value of this property.
*
* @return the isFallbackPublicClient value.
*/
public Boolean isFallbackPublicClient() {
return this.isFallbackPublicClient;
}
/**
* Set the isFallbackPublicClient property: Specifies the fallback application type as public client, such as an
* installed application running on a mobile device. The default value is false which means the fallback application
* type is confidential client such as web app. There are certain scenarios where Azure AD cannot determine the
* client application type (e.g. ROPC flow where it is configured without specifying a redirect URI). In those cases
* Azure AD will interpret the application type based on the value of this property.
*
* @param isFallbackPublicClient the isFallbackPublicClient value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withIsFallbackPublicClient(Boolean isFallbackPublicClient) {
this.isFallbackPublicClient = isFallbackPublicClient;
return this;
}
/**
* Get the keyCredentials property: The collection of key credentials associated with the application Not nullable.
*
* @return the keyCredentials value.
*/
public List keyCredentials() {
return this.keyCredentials;
}
/**
* Set the keyCredentials property: The collection of key credentials associated with the application Not nullable.
*
* @param keyCredentials the keyCredentials value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withKeyCredentials(List keyCredentials) {
this.keyCredentials = keyCredentials;
return this;
}
/**
* Get the logo property: The main logo for the application. Not nullable.
*
* @return the logo value.
*/
public byte[] logo() {
if (this.logo == null) {
return EMPTY_BYTE_ARRAY;
}
return this.logo.decodedBytes();
}
/**
* Set the logo property: The main logo for the application. Not nullable.
*
* @param logo the logo value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withLogo(byte[] logo) {
if (logo == null) {
this.logo = null;
} else {
this.logo = Base64Url.encode(CoreUtils.clone(logo));
}
return this;
}
/**
* Get the notes property: The notes property.
*
* @return the notes value.
*/
public String notes() {
return this.notes;
}
/**
* Set the notes property: The notes property.
*
* @param notes the notes value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withNotes(String notes) {
this.notes = notes;
return this;
}
/**
* Get the oauth2RequirePostResponse property: The oauth2RequirePostResponse property.
*
* @return the oauth2RequirePostResponse value.
*/
public Boolean oauth2RequirePostResponse() {
return this.oauth2RequirePostResponse;
}
/**
* Set the oauth2RequirePostResponse property: The oauth2RequirePostResponse property.
*
* @param oauth2RequirePostResponse the oauth2RequirePostResponse value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withOauth2RequirePostResponse(Boolean oauth2RequirePostResponse) {
this.oauth2RequirePostResponse = oauth2RequirePostResponse;
return this;
}
/**
* Get the optionalClaims property: optionalClaims.
*
* @return the optionalClaims value.
*/
public MicrosoftGraphOptionalClaims optionalClaims() {
return this.optionalClaims;
}
/**
* Set the optionalClaims property: optionalClaims.
*
* @param optionalClaims the optionalClaims value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withOptionalClaims(MicrosoftGraphOptionalClaims optionalClaims) {
this.optionalClaims = optionalClaims;
return this;
}
/**
* Get the parentalControlSettings property: parentalControlSettings.
*
* @return the parentalControlSettings value.
*/
public MicrosoftGraphParentalControlSettings parentalControlSettings() {
return this.parentalControlSettings;
}
/**
* Set the parentalControlSettings property: parentalControlSettings.
*
* @param parentalControlSettings the parentalControlSettings value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner
withParentalControlSettings(MicrosoftGraphParentalControlSettings parentalControlSettings) {
this.parentalControlSettings = parentalControlSettings;
return this;
}
/**
* Get the passwordCredentials property: The collection of password credentials associated with the application. Not
* nullable.
*
* @return the passwordCredentials value.
*/
public List passwordCredentials() {
return this.passwordCredentials;
}
/**
* Set the passwordCredentials property: The collection of password credentials associated with the application. Not
* nullable.
*
* @param passwordCredentials the passwordCredentials value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner
withPasswordCredentials(List passwordCredentials) {
this.passwordCredentials = passwordCredentials;
return this;
}
/**
* Get the publicClient property: publicClientApplication.
*
* @return the publicClient value.
*/
public MicrosoftGraphPublicClientApplication publicClient() {
return this.publicClient;
}
/**
* Set the publicClient property: publicClientApplication.
*
* @param publicClient the publicClient value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withPublicClient(MicrosoftGraphPublicClientApplication publicClient) {
this.publicClient = publicClient;
return this;
}
/**
* Get the publisherDomain property: The verified publisher domain for the application. Read-only.
*
* @return the publisherDomain value.
*/
public String publisherDomain() {
return this.publisherDomain;
}
/**
* Set the publisherDomain property: The verified publisher domain for the application. Read-only.
*
* @param publisherDomain the publisherDomain value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withPublisherDomain(String publisherDomain) {
this.publisherDomain = publisherDomain;
return this;
}
/**
* Get the requiredResourceAccess property: Specifies resources that this application requires access to and the set
* of OAuth permission scopes and application roles that it needs under each of those resources. This
* pre-configuration of required resource access drives the consent experience. Not nullable.
*
* @return the requiredResourceAccess value.
*/
public List requiredResourceAccess() {
return this.requiredResourceAccess;
}
/**
* Set the requiredResourceAccess property: Specifies resources that this application requires access to and the set
* of OAuth permission scopes and application roles that it needs under each of those resources. This
* pre-configuration of required resource access drives the consent experience. Not nullable.
*
* @param requiredResourceAccess the requiredResourceAccess value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner
withRequiredResourceAccess(List requiredResourceAccess) {
this.requiredResourceAccess = requiredResourceAccess;
return this;
}
/**
* Get the signInAudience property: Specifies the Microsoft accounts that are supported for the current application.
* Supported values are:AzureADMyOrg: Users with a Microsoft work or school account in my organization’s Azure AD
* tenant (single tenant)AzureADMultipleOrgs: Users with a Microsoft work or school account in any organization’s
* Azure AD tenant (multi-tenant).AzureADandPersonalMicrosoftAccount: Users with a personal Microsoft account, or a
* work or school account in any organization’s Azure AD tenant.PersonalMicrosoftAccount: Users with a personal
* Microsoft account only.For authenticating users with Azure AD B2C user flows, use
* AzureADandPersonalMicrosoftAccount. This value allows for the widest set of user identities including local
* accounts and user identities from Microsoft, Facebook, Google, Twitter, or any OpenID Connect provider.
*
* @return the signInAudience value.
*/
public String signInAudience() {
return this.signInAudience;
}
/**
* Set the signInAudience property: Specifies the Microsoft accounts that are supported for the current application.
* Supported values are:AzureADMyOrg: Users with a Microsoft work or school account in my organization’s Azure AD
* tenant (single tenant)AzureADMultipleOrgs: Users with a Microsoft work or school account in any organization’s
* Azure AD tenant (multi-tenant).AzureADandPersonalMicrosoftAccount: Users with a personal Microsoft account, or a
* work or school account in any organization’s Azure AD tenant.PersonalMicrosoftAccount: Users with a personal
* Microsoft account only.For authenticating users with Azure AD B2C user flows, use
* AzureADandPersonalMicrosoftAccount. This value allows for the widest set of user identities including local
* accounts and user identities from Microsoft, Facebook, Google, Twitter, or any OpenID Connect provider.
*
* @param signInAudience the signInAudience value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withSignInAudience(String signInAudience) {
this.signInAudience = signInAudience;
return this;
}
/**
* Get the tags property: Custom strings that can be used to categorize and identify the application. Not nullable.
*
* @return the tags value.
*/
public List tags() {
return this.tags;
}
/**
* Set the tags property: Custom strings that can be used to categorize and identify the application. Not nullable.
*
* @param tags the tags value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withTags(List tags) {
this.tags = tags;
return this;
}
/**
* Get the tokenEncryptionKeyId property: Specifies the keyId of a public key from the keyCredentials collection.
* When configured, Azure AD encrypts all the tokens it emits by using the key this property points to. The
* application code that receives the encrypted token must use the matching private key to decrypt the token before
* it can be used for the signed-in user.
*
* @return the tokenEncryptionKeyId value.
*/
public UUID tokenEncryptionKeyId() {
return this.tokenEncryptionKeyId;
}
/**
* Set the tokenEncryptionKeyId property: Specifies the keyId of a public key from the keyCredentials collection.
* When configured, Azure AD encrypts all the tokens it emits by using the key this property points to. The
* application code that receives the encrypted token must use the matching private key to decrypt the token before
* it can be used for the signed-in user.
*
* @param tokenEncryptionKeyId the tokenEncryptionKeyId value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withTokenEncryptionKeyId(UUID tokenEncryptionKeyId) {
this.tokenEncryptionKeyId = tokenEncryptionKeyId;
return this;
}
/**
* Get the web property: webApplication.
*
* @return the web value.
*/
public MicrosoftGraphWebApplication web() {
return this.web;
}
/**
* Set the web property: webApplication.
*
* @param web the web value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withWeb(MicrosoftGraphWebApplication web) {
this.web = web;
return this;
}
/**
* Get the createdOnBehalfOf property: Represents an Azure Active Directory object. The directoryObject type is the
* base type for many other directory entity types.
*
* @return the createdOnBehalfOf value.
*/
public MicrosoftGraphDirectoryObjectInner createdOnBehalfOf() {
return this.createdOnBehalfOf;
}
/**
* Set the createdOnBehalfOf property: Represents an Azure Active Directory object. The directoryObject type is the
* base type for many other directory entity types.
*
* @param createdOnBehalfOf the createdOnBehalfOf value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withCreatedOnBehalfOf(MicrosoftGraphDirectoryObjectInner createdOnBehalfOf) {
this.createdOnBehalfOf = createdOnBehalfOf;
return this;
}
/**
* Get the extensionProperties property: Read-only. Nullable.
*
* @return the extensionProperties value.
*/
public List extensionProperties() {
return this.extensionProperties;
}
/**
* Set the extensionProperties property: Read-only. Nullable.
*
* @param extensionProperties the extensionProperties value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner
withExtensionProperties(List extensionProperties) {
this.extensionProperties = extensionProperties;
return this;
}
/**
* Get the homeRealmDiscoveryPolicies property: The homeRealmDiscoveryPolicies property.
*
* @return the homeRealmDiscoveryPolicies value.
*/
public List homeRealmDiscoveryPolicies() {
return this.homeRealmDiscoveryPolicies;
}
/**
* Set the homeRealmDiscoveryPolicies property: The homeRealmDiscoveryPolicies property.
*
* @param homeRealmDiscoveryPolicies the homeRealmDiscoveryPolicies value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner
withHomeRealmDiscoveryPolicies(List homeRealmDiscoveryPolicies) {
this.homeRealmDiscoveryPolicies = homeRealmDiscoveryPolicies;
return this;
}
/**
* Get the owners property: Directory objects that are owners of the application. The owners are a set of non-admin
* users who are allowed to modify this object. Requires version 2013-11-08 or newer. Read-only. Nullable.
*
* @return the owners value.
*/
public List owners() {
return this.owners;
}
/**
* Set the owners property: Directory objects that are owners of the application. The owners are a set of non-admin
* users who are allowed to modify this object. Requires version 2013-11-08 or newer. Read-only. Nullable.
*
* @param owners the owners value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withOwners(List owners) {
this.owners = owners;
return this;
}
/**
* Get the tokenIssuancePolicies property: The tokenIssuancePolicies property.
*
* @return the tokenIssuancePolicies value.
*/
public List tokenIssuancePolicies() {
return this.tokenIssuancePolicies;
}
/**
* Set the tokenIssuancePolicies property: The tokenIssuancePolicies property.
*
* @param tokenIssuancePolicies the tokenIssuancePolicies value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner
withTokenIssuancePolicies(List tokenIssuancePolicies) {
this.tokenIssuancePolicies = tokenIssuancePolicies;
return this;
}
/**
* Get the tokenLifetimePolicies property: The tokenLifetimePolicies property.
*
* @return the tokenLifetimePolicies value.
*/
public List tokenLifetimePolicies() {
return this.tokenLifetimePolicies;
}
/**
* Set the tokenLifetimePolicies property: The tokenLifetimePolicies property.
*
* @param tokenLifetimePolicies the tokenLifetimePolicies value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner
withTokenLifetimePolicies(List tokenLifetimePolicies) {
this.tokenLifetimePolicies = tokenLifetimePolicies;
return this;
}
/**
* Get the additionalProperties property: Represents an Azure Active Directory object. The directoryObject type is
* the base type for many other directory entity types.
*
* @return the additionalProperties value.
*/
public Map additionalProperties() {
return this.additionalProperties;
}
/**
* Set the additionalProperties property: Represents an Azure Active Directory object. The directoryObject type is
* the base type for many other directory entity types.
*
* @param additionalProperties the additionalProperties value to set.
* @return the MicrosoftGraphApplicationInner object itself.
*/
public MicrosoftGraphApplicationInner withAdditionalProperties(Map additionalProperties) {
this.additionalProperties = additionalProperties;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphApplicationInner withDeletedDateTime(OffsetDateTime deletedDateTime) {
super.withDeletedDateTime(deletedDateTime);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphApplicationInner withId(String id) {
super.withId(id);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (addIns() != null) {
addIns().forEach(e -> e.validate());
}
if (api() != null) {
api().validate();
}
if (appRoles() != null) {
appRoles().forEach(e -> e.validate());
}
if (info() != null) {
info().validate();
}
if (keyCredentials() != null) {
keyCredentials().forEach(e -> e.validate());
}
if (optionalClaims() != null) {
optionalClaims().validate();
}
if (parentalControlSettings() != null) {
parentalControlSettings().validate();
}
if (passwordCredentials() != null) {
passwordCredentials().forEach(e -> e.validate());
}
if (publicClient() != null) {
publicClient().validate();
}
if (requiredResourceAccess() != null) {
requiredResourceAccess().forEach(e -> e.validate());
}
if (web() != null) {
web().validate();
}
if (createdOnBehalfOf() != null) {
createdOnBehalfOf().validate();
}
if (extensionProperties() != null) {
extensionProperties().forEach(e -> e.validate());
}
if (homeRealmDiscoveryPolicies() != null) {
homeRealmDiscoveryPolicies().forEach(e -> e.validate());
}
if (owners() != null) {
owners().forEach(e -> e.validate());
}
if (tokenIssuancePolicies() != null) {
tokenIssuancePolicies().forEach(e -> e.validate());
}
if (tokenLifetimePolicies() != null) {
tokenLifetimePolicies().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("id", id());
jsonWriter.writeStringField("deletedDateTime",
deletedDateTime() == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(deletedDateTime()));
jsonWriter.writeArrayField("addIns", this.addIns, (writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("api", this.api);
jsonWriter.writeStringField("appId", this.appId);
jsonWriter.writeStringField("applicationTemplateId", this.applicationTemplateId);
jsonWriter.writeArrayField("appRoles", this.appRoles, (writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("createdDateTime",
this.createdDateTime == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.createdDateTime));
jsonWriter.writeStringField("description", this.description);
jsonWriter.writeStringField("displayName", this.displayName);
jsonWriter.writeStringField("groupMembershipClaims", this.groupMembershipClaims);
jsonWriter.writeArrayField("identifierUris", this.identifierUris,
(writer, element) -> writer.writeString(element));
jsonWriter.writeJsonField("info", this.info);
jsonWriter.writeBooleanField("isDeviceOnlyAuthSupported", this.isDeviceOnlyAuthSupported);
jsonWriter.writeBooleanField("isFallbackPublicClient", this.isFallbackPublicClient);
jsonWriter.writeArrayField("keyCredentials", this.keyCredentials,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("logo", Objects.toString(this.logo, null));
jsonWriter.writeStringField("notes", this.notes);
jsonWriter.writeBooleanField("oauth2RequirePostResponse", this.oauth2RequirePostResponse);
jsonWriter.writeJsonField("optionalClaims", this.optionalClaims);
jsonWriter.writeJsonField("parentalControlSettings", this.parentalControlSettings);
jsonWriter.writeArrayField("passwordCredentials", this.passwordCredentials,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("publicClient", this.publicClient);
jsonWriter.writeStringField("publisherDomain", this.publisherDomain);
jsonWriter.writeArrayField("requiredResourceAccess", this.requiredResourceAccess,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("signInAudience", this.signInAudience);
jsonWriter.writeArrayField("tags", this.tags, (writer, element) -> writer.writeString(element));
jsonWriter.writeStringField("tokenEncryptionKeyId", Objects.toString(this.tokenEncryptionKeyId, null));
jsonWriter.writeJsonField("web", this.web);
jsonWriter.writeJsonField("createdOnBehalfOf", this.createdOnBehalfOf);
jsonWriter.writeArrayField("extensionProperties", this.extensionProperties,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("homeRealmDiscoveryPolicies", this.homeRealmDiscoveryPolicies,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("owners", this.owners, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("tokenIssuancePolicies", this.tokenIssuancePolicies,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("tokenLifetimePolicies", this.tokenLifetimePolicies,
(writer, element) -> writer.writeJson(element));
if (additionalProperties != null) {
for (Map.Entry additionalProperty : additionalProperties.entrySet()) {
jsonWriter.writeUntypedField(additionalProperty.getKey(), additionalProperty.getValue());
}
}
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MicrosoftGraphApplicationInner from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MicrosoftGraphApplicationInner if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the MicrosoftGraphApplicationInner.
*/
public static MicrosoftGraphApplicationInner fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
MicrosoftGraphApplicationInner deserializedMicrosoftGraphApplicationInner
= new MicrosoftGraphApplicationInner();
Map additionalProperties = null;
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.withId(reader.getString());
} else if ("deletedDateTime".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.withDeletedDateTime(reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString())));
} else if ("addIns".equals(fieldName)) {
List addIns
= reader.readArray(reader1 -> MicrosoftGraphAddIn.fromJson(reader1));
deserializedMicrosoftGraphApplicationInner.addIns = addIns;
} else if ("api".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.api = MicrosoftGraphApiApplication.fromJson(reader);
} else if ("appId".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.appId = reader.getString();
} else if ("applicationTemplateId".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.applicationTemplateId = reader.getString();
} else if ("appRoles".equals(fieldName)) {
List appRoles
= reader.readArray(reader1 -> MicrosoftGraphAppRole.fromJson(reader1));
deserializedMicrosoftGraphApplicationInner.appRoles = appRoles;
} else if ("createdDateTime".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.createdDateTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("description".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.description = reader.getString();
} else if ("displayName".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.displayName = reader.getString();
} else if ("groupMembershipClaims".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.groupMembershipClaims = reader.getString();
} else if ("identifierUris".equals(fieldName)) {
List identifierUris = reader.readArray(reader1 -> reader1.getString());
deserializedMicrosoftGraphApplicationInner.identifierUris = identifierUris;
} else if ("info".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.info = MicrosoftGraphInformationalUrl.fromJson(reader);
} else if ("isDeviceOnlyAuthSupported".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.isDeviceOnlyAuthSupported
= reader.getNullable(JsonReader::getBoolean);
} else if ("isFallbackPublicClient".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.isFallbackPublicClient
= reader.getNullable(JsonReader::getBoolean);
} else if ("keyCredentials".equals(fieldName)) {
List keyCredentials
= reader.readArray(reader1 -> MicrosoftGraphKeyCredentialInner.fromJson(reader1));
deserializedMicrosoftGraphApplicationInner.keyCredentials = keyCredentials;
} else if ("logo".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.logo
= reader.getNullable(nonNullReader -> new Base64Url(nonNullReader.getString()));
} else if ("notes".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.notes = reader.getString();
} else if ("oauth2RequirePostResponse".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.oauth2RequirePostResponse
= reader.getNullable(JsonReader::getBoolean);
} else if ("optionalClaims".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.optionalClaims
= MicrosoftGraphOptionalClaims.fromJson(reader);
} else if ("parentalControlSettings".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.parentalControlSettings
= MicrosoftGraphParentalControlSettings.fromJson(reader);
} else if ("passwordCredentials".equals(fieldName)) {
List passwordCredentials
= reader.readArray(reader1 -> MicrosoftGraphPasswordCredentialInner.fromJson(reader1));
deserializedMicrosoftGraphApplicationInner.passwordCredentials = passwordCredentials;
} else if ("publicClient".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.publicClient
= MicrosoftGraphPublicClientApplication.fromJson(reader);
} else if ("publisherDomain".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.publisherDomain = reader.getString();
} else if ("requiredResourceAccess".equals(fieldName)) {
List requiredResourceAccess
= reader.readArray(reader1 -> MicrosoftGraphRequiredResourceAccess.fromJson(reader1));
deserializedMicrosoftGraphApplicationInner.requiredResourceAccess = requiredResourceAccess;
} else if ("signInAudience".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.signInAudience = reader.getString();
} else if ("tags".equals(fieldName)) {
List tags = reader.readArray(reader1 -> reader1.getString());
deserializedMicrosoftGraphApplicationInner.tags = tags;
} else if ("tokenEncryptionKeyId".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.tokenEncryptionKeyId
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else if ("web".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.web = MicrosoftGraphWebApplication.fromJson(reader);
} else if ("createdOnBehalfOf".equals(fieldName)) {
deserializedMicrosoftGraphApplicationInner.createdOnBehalfOf
= MicrosoftGraphDirectoryObjectInner.fromJson(reader);
} else if ("extensionProperties".equals(fieldName)) {
List extensionProperties
= reader.readArray(reader1 -> MicrosoftGraphExtensionPropertyInner.fromJson(reader1));
deserializedMicrosoftGraphApplicationInner.extensionProperties = extensionProperties;
} else if ("homeRealmDiscoveryPolicies".equals(fieldName)) {
List homeRealmDiscoveryPolicies
= reader.readArray(reader1 -> MicrosoftGraphHomeRealmDiscoveryPolicyInner.fromJson(reader1));
deserializedMicrosoftGraphApplicationInner.homeRealmDiscoveryPolicies = homeRealmDiscoveryPolicies;
} else if ("owners".equals(fieldName)) {
List owners
= reader.readArray(reader1 -> MicrosoftGraphDirectoryObjectInner.fromJson(reader1));
deserializedMicrosoftGraphApplicationInner.owners = owners;
} else if ("tokenIssuancePolicies".equals(fieldName)) {
List tokenIssuancePolicies
= reader.readArray(reader1 -> MicrosoftGraphTokenIssuancePolicy.fromJson(reader1));
deserializedMicrosoftGraphApplicationInner.tokenIssuancePolicies = tokenIssuancePolicies;
} else if ("tokenLifetimePolicies".equals(fieldName)) {
List tokenLifetimePolicies
= reader.readArray(reader1 -> MicrosoftGraphTokenLifetimePolicy.fromJson(reader1));
deserializedMicrosoftGraphApplicationInner.tokenLifetimePolicies = tokenLifetimePolicies;
} else {
if (additionalProperties == null) {
additionalProperties = new LinkedHashMap<>();
}
additionalProperties.put(fieldName, reader.readUntyped());
}
}
deserializedMicrosoftGraphApplicationInner.additionalProperties = additionalProperties;
return deserializedMicrosoftGraphApplicationInner;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy