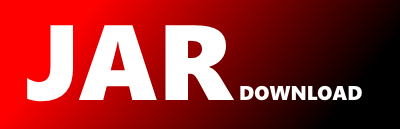
com.azure.resourcemanager.authorization.fluent.models.MicrosoftGraphContact Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.authorization.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
/**
* contact.
*/
@Fluent
public final class MicrosoftGraphContact extends MicrosoftGraphOutlookItem {
/*
* The name of the contact's assistant.
*/
private String assistantName;
/*
* The contact's birthday. The Timestamp type represents date and time information using ISO 8601 format and is
* always in UTC time. For example, midnight UTC on Jan 1, 2014 would look like this: '2014-01-01T00:00:00Z'
*/
private OffsetDateTime birthday;
/*
* physicalAddress
*/
private MicrosoftGraphPhysicalAddress businessAddress;
/*
* The business home page of the contact.
*/
private String businessHomePage;
/*
* The contact's business phone numbers.
*/
private List businessPhones;
/*
* The names of the contact's children.
*/
private List children;
/*
* The name of the contact's company.
*/
private String companyName;
/*
* The contact's department.
*/
private String department;
/*
* The contact's display name. You can specify the display name in a create or update operation. Note that later
* updates to other properties may cause an automatically generated value to overwrite the displayName value you
* have specified. To preserve a pre-existing value, always include it as displayName in an update operation.
*/
private String displayName;
/*
* The contact's email addresses.
*/
private List emailAddresses;
/*
* The name the contact is filed under.
*/
private String fileAs;
/*
* The contact's generation.
*/
private String generation;
/*
* The contact's given name.
*/
private String givenName;
/*
* physicalAddress
*/
private MicrosoftGraphPhysicalAddress homeAddress;
/*
* The contact's home phone numbers.
*/
private List homePhones;
/*
* The contact's instant messaging (IM) addresses.
*/
private List imAddresses;
/*
* The contact's initials.
*/
private String initials;
/*
* The contact’s job title.
*/
private String jobTitle;
/*
* The name of the contact's manager.
*/
private String manager;
/*
* The contact's middle name.
*/
private String middleName;
/*
* The contact's mobile phone number.
*/
private String mobilePhone;
/*
* The contact's nickname.
*/
private String nickName;
/*
* The location of the contact's office.
*/
private String officeLocation;
/*
* physicalAddress
*/
private MicrosoftGraphPhysicalAddress otherAddress;
/*
* The ID of the contact's parent folder.
*/
private String parentFolderId;
/*
* The user's notes about the contact.
*/
private String personalNotes;
/*
* The contact's profession.
*/
private String profession;
/*
* The name of the contact's spouse/partner.
*/
private String spouseName;
/*
* The contact's surname.
*/
private String surname;
/*
* The contact's title.
*/
private String title;
/*
* The phonetic Japanese company name of the contact.
*/
private String yomiCompanyName;
/*
* The phonetic Japanese given name (first name) of the contact.
*/
private String yomiGivenName;
/*
* The phonetic Japanese surname (last name) of the contact.
*/
private String yomiSurname;
/*
* The collection of open extensions defined for the contact. Read-only. Nullable.
*/
private List extensions;
/*
* The collection of multi-value extended properties defined for the contact. Read-only. Nullable.
*/
private List multiValueExtendedProperties;
/*
* profilePhoto
*/
private MicrosoftGraphProfilePhoto photo;
/*
* The collection of single-value extended properties defined for the contact. Read-only. Nullable.
*/
private List singleValueExtendedProperties;
/*
* contact
*/
private Map additionalProperties;
/**
* Creates an instance of MicrosoftGraphContact class.
*/
public MicrosoftGraphContact() {
}
/**
* Get the assistantName property: The name of the contact's assistant.
*
* @return the assistantName value.
*/
public String assistantName() {
return this.assistantName;
}
/**
* Set the assistantName property: The name of the contact's assistant.
*
* @param assistantName the assistantName value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withAssistantName(String assistantName) {
this.assistantName = assistantName;
return this;
}
/**
* Get the birthday property: The contact's birthday. The Timestamp type represents date and time information using
* ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 would look like this:
* '2014-01-01T00:00:00Z'.
*
* @return the birthday value.
*/
public OffsetDateTime birthday() {
return this.birthday;
}
/**
* Set the birthday property: The contact's birthday. The Timestamp type represents date and time information using
* ISO 8601 format and is always in UTC time. For example, midnight UTC on Jan 1, 2014 would look like this:
* '2014-01-01T00:00:00Z'.
*
* @param birthday the birthday value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withBirthday(OffsetDateTime birthday) {
this.birthday = birthday;
return this;
}
/**
* Get the businessAddress property: physicalAddress.
*
* @return the businessAddress value.
*/
public MicrosoftGraphPhysicalAddress businessAddress() {
return this.businessAddress;
}
/**
* Set the businessAddress property: physicalAddress.
*
* @param businessAddress the businessAddress value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withBusinessAddress(MicrosoftGraphPhysicalAddress businessAddress) {
this.businessAddress = businessAddress;
return this;
}
/**
* Get the businessHomePage property: The business home page of the contact.
*
* @return the businessHomePage value.
*/
public String businessHomePage() {
return this.businessHomePage;
}
/**
* Set the businessHomePage property: The business home page of the contact.
*
* @param businessHomePage the businessHomePage value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withBusinessHomePage(String businessHomePage) {
this.businessHomePage = businessHomePage;
return this;
}
/**
* Get the businessPhones property: The contact's business phone numbers.
*
* @return the businessPhones value.
*/
public List businessPhones() {
return this.businessPhones;
}
/**
* Set the businessPhones property: The contact's business phone numbers.
*
* @param businessPhones the businessPhones value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withBusinessPhones(List businessPhones) {
this.businessPhones = businessPhones;
return this;
}
/**
* Get the children property: The names of the contact's children.
*
* @return the children value.
*/
public List children() {
return this.children;
}
/**
* Set the children property: The names of the contact's children.
*
* @param children the children value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withChildren(List children) {
this.children = children;
return this;
}
/**
* Get the companyName property: The name of the contact's company.
*
* @return the companyName value.
*/
public String companyName() {
return this.companyName;
}
/**
* Set the companyName property: The name of the contact's company.
*
* @param companyName the companyName value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withCompanyName(String companyName) {
this.companyName = companyName;
return this;
}
/**
* Get the department property: The contact's department.
*
* @return the department value.
*/
public String department() {
return this.department;
}
/**
* Set the department property: The contact's department.
*
* @param department the department value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withDepartment(String department) {
this.department = department;
return this;
}
/**
* Get the displayName property: The contact's display name. You can specify the display name in a create or update
* operation. Note that later updates to other properties may cause an automatically generated value to overwrite
* the displayName value you have specified. To preserve a pre-existing value, always include it as displayName in
* an update operation.
*
* @return the displayName value.
*/
public String displayName() {
return this.displayName;
}
/**
* Set the displayName property: The contact's display name. You can specify the display name in a create or update
* operation. Note that later updates to other properties may cause an automatically generated value to overwrite
* the displayName value you have specified. To preserve a pre-existing value, always include it as displayName in
* an update operation.
*
* @param displayName the displayName value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withDisplayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Get the emailAddresses property: The contact's email addresses.
*
* @return the emailAddresses value.
*/
public List emailAddresses() {
return this.emailAddresses;
}
/**
* Set the emailAddresses property: The contact's email addresses.
*
* @param emailAddresses the emailAddresses value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withEmailAddresses(List emailAddresses) {
this.emailAddresses = emailAddresses;
return this;
}
/**
* Get the fileAs property: The name the contact is filed under.
*
* @return the fileAs value.
*/
public String fileAs() {
return this.fileAs;
}
/**
* Set the fileAs property: The name the contact is filed under.
*
* @param fileAs the fileAs value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withFileAs(String fileAs) {
this.fileAs = fileAs;
return this;
}
/**
* Get the generation property: The contact's generation.
*
* @return the generation value.
*/
public String generation() {
return this.generation;
}
/**
* Set the generation property: The contact's generation.
*
* @param generation the generation value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withGeneration(String generation) {
this.generation = generation;
return this;
}
/**
* Get the givenName property: The contact's given name.
*
* @return the givenName value.
*/
public String givenName() {
return this.givenName;
}
/**
* Set the givenName property: The contact's given name.
*
* @param givenName the givenName value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withGivenName(String givenName) {
this.givenName = givenName;
return this;
}
/**
* Get the homeAddress property: physicalAddress.
*
* @return the homeAddress value.
*/
public MicrosoftGraphPhysicalAddress homeAddress() {
return this.homeAddress;
}
/**
* Set the homeAddress property: physicalAddress.
*
* @param homeAddress the homeAddress value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withHomeAddress(MicrosoftGraphPhysicalAddress homeAddress) {
this.homeAddress = homeAddress;
return this;
}
/**
* Get the homePhones property: The contact's home phone numbers.
*
* @return the homePhones value.
*/
public List homePhones() {
return this.homePhones;
}
/**
* Set the homePhones property: The contact's home phone numbers.
*
* @param homePhones the homePhones value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withHomePhones(List homePhones) {
this.homePhones = homePhones;
return this;
}
/**
* Get the imAddresses property: The contact's instant messaging (IM) addresses.
*
* @return the imAddresses value.
*/
public List imAddresses() {
return this.imAddresses;
}
/**
* Set the imAddresses property: The contact's instant messaging (IM) addresses.
*
* @param imAddresses the imAddresses value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withImAddresses(List imAddresses) {
this.imAddresses = imAddresses;
return this;
}
/**
* Get the initials property: The contact's initials.
*
* @return the initials value.
*/
public String initials() {
return this.initials;
}
/**
* Set the initials property: The contact's initials.
*
* @param initials the initials value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withInitials(String initials) {
this.initials = initials;
return this;
}
/**
* Get the jobTitle property: The contact’s job title.
*
* @return the jobTitle value.
*/
public String jobTitle() {
return this.jobTitle;
}
/**
* Set the jobTitle property: The contact’s job title.
*
* @param jobTitle the jobTitle value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withJobTitle(String jobTitle) {
this.jobTitle = jobTitle;
return this;
}
/**
* Get the manager property: The name of the contact's manager.
*
* @return the manager value.
*/
public String manager() {
return this.manager;
}
/**
* Set the manager property: The name of the contact's manager.
*
* @param manager the manager value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withManager(String manager) {
this.manager = manager;
return this;
}
/**
* Get the middleName property: The contact's middle name.
*
* @return the middleName value.
*/
public String middleName() {
return this.middleName;
}
/**
* Set the middleName property: The contact's middle name.
*
* @param middleName the middleName value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withMiddleName(String middleName) {
this.middleName = middleName;
return this;
}
/**
* Get the mobilePhone property: The contact's mobile phone number.
*
* @return the mobilePhone value.
*/
public String mobilePhone() {
return this.mobilePhone;
}
/**
* Set the mobilePhone property: The contact's mobile phone number.
*
* @param mobilePhone the mobilePhone value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withMobilePhone(String mobilePhone) {
this.mobilePhone = mobilePhone;
return this;
}
/**
* Get the nickName property: The contact's nickname.
*
* @return the nickName value.
*/
public String nickName() {
return this.nickName;
}
/**
* Set the nickName property: The contact's nickname.
*
* @param nickName the nickName value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withNickName(String nickName) {
this.nickName = nickName;
return this;
}
/**
* Get the officeLocation property: The location of the contact's office.
*
* @return the officeLocation value.
*/
public String officeLocation() {
return this.officeLocation;
}
/**
* Set the officeLocation property: The location of the contact's office.
*
* @param officeLocation the officeLocation value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withOfficeLocation(String officeLocation) {
this.officeLocation = officeLocation;
return this;
}
/**
* Get the otherAddress property: physicalAddress.
*
* @return the otherAddress value.
*/
public MicrosoftGraphPhysicalAddress otherAddress() {
return this.otherAddress;
}
/**
* Set the otherAddress property: physicalAddress.
*
* @param otherAddress the otherAddress value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withOtherAddress(MicrosoftGraphPhysicalAddress otherAddress) {
this.otherAddress = otherAddress;
return this;
}
/**
* Get the parentFolderId property: The ID of the contact's parent folder.
*
* @return the parentFolderId value.
*/
public String parentFolderId() {
return this.parentFolderId;
}
/**
* Set the parentFolderId property: The ID of the contact's parent folder.
*
* @param parentFolderId the parentFolderId value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withParentFolderId(String parentFolderId) {
this.parentFolderId = parentFolderId;
return this;
}
/**
* Get the personalNotes property: The user's notes about the contact.
*
* @return the personalNotes value.
*/
public String personalNotes() {
return this.personalNotes;
}
/**
* Set the personalNotes property: The user's notes about the contact.
*
* @param personalNotes the personalNotes value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withPersonalNotes(String personalNotes) {
this.personalNotes = personalNotes;
return this;
}
/**
* Get the profession property: The contact's profession.
*
* @return the profession value.
*/
public String profession() {
return this.profession;
}
/**
* Set the profession property: The contact's profession.
*
* @param profession the profession value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withProfession(String profession) {
this.profession = profession;
return this;
}
/**
* Get the spouseName property: The name of the contact's spouse/partner.
*
* @return the spouseName value.
*/
public String spouseName() {
return this.spouseName;
}
/**
* Set the spouseName property: The name of the contact's spouse/partner.
*
* @param spouseName the spouseName value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withSpouseName(String spouseName) {
this.spouseName = spouseName;
return this;
}
/**
* Get the surname property: The contact's surname.
*
* @return the surname value.
*/
public String surname() {
return this.surname;
}
/**
* Set the surname property: The contact's surname.
*
* @param surname the surname value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withSurname(String surname) {
this.surname = surname;
return this;
}
/**
* Get the title property: The contact's title.
*
* @return the title value.
*/
public String title() {
return this.title;
}
/**
* Set the title property: The contact's title.
*
* @param title the title value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withTitle(String title) {
this.title = title;
return this;
}
/**
* Get the yomiCompanyName property: The phonetic Japanese company name of the contact.
*
* @return the yomiCompanyName value.
*/
public String yomiCompanyName() {
return this.yomiCompanyName;
}
/**
* Set the yomiCompanyName property: The phonetic Japanese company name of the contact.
*
* @param yomiCompanyName the yomiCompanyName value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withYomiCompanyName(String yomiCompanyName) {
this.yomiCompanyName = yomiCompanyName;
return this;
}
/**
* Get the yomiGivenName property: The phonetic Japanese given name (first name) of the contact.
*
* @return the yomiGivenName value.
*/
public String yomiGivenName() {
return this.yomiGivenName;
}
/**
* Set the yomiGivenName property: The phonetic Japanese given name (first name) of the contact.
*
* @param yomiGivenName the yomiGivenName value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withYomiGivenName(String yomiGivenName) {
this.yomiGivenName = yomiGivenName;
return this;
}
/**
* Get the yomiSurname property: The phonetic Japanese surname (last name) of the contact.
*
* @return the yomiSurname value.
*/
public String yomiSurname() {
return this.yomiSurname;
}
/**
* Set the yomiSurname property: The phonetic Japanese surname (last name) of the contact.
*
* @param yomiSurname the yomiSurname value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withYomiSurname(String yomiSurname) {
this.yomiSurname = yomiSurname;
return this;
}
/**
* Get the extensions property: The collection of open extensions defined for the contact. Read-only. Nullable.
*
* @return the extensions value.
*/
public List extensions() {
return this.extensions;
}
/**
* Set the extensions property: The collection of open extensions defined for the contact. Read-only. Nullable.
*
* @param extensions the extensions value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withExtensions(List extensions) {
this.extensions = extensions;
return this;
}
/**
* Get the multiValueExtendedProperties property: The collection of multi-value extended properties defined for the
* contact. Read-only. Nullable.
*
* @return the multiValueExtendedProperties value.
*/
public List multiValueExtendedProperties() {
return this.multiValueExtendedProperties;
}
/**
* Set the multiValueExtendedProperties property: The collection of multi-value extended properties defined for the
* contact. Read-only. Nullable.
*
* @param multiValueExtendedProperties the multiValueExtendedProperties value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withMultiValueExtendedProperties(
List multiValueExtendedProperties) {
this.multiValueExtendedProperties = multiValueExtendedProperties;
return this;
}
/**
* Get the photo property: profilePhoto.
*
* @return the photo value.
*/
public MicrosoftGraphProfilePhoto photo() {
return this.photo;
}
/**
* Set the photo property: profilePhoto.
*
* @param photo the photo value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withPhoto(MicrosoftGraphProfilePhoto photo) {
this.photo = photo;
return this;
}
/**
* Get the singleValueExtendedProperties property: The collection of single-value extended properties defined for
* the contact. Read-only. Nullable.
*
* @return the singleValueExtendedProperties value.
*/
public List singleValueExtendedProperties() {
return this.singleValueExtendedProperties;
}
/**
* Set the singleValueExtendedProperties property: The collection of single-value extended properties defined for
* the contact. Read-only. Nullable.
*
* @param singleValueExtendedProperties the singleValueExtendedProperties value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withSingleValueExtendedProperties(
List singleValueExtendedProperties) {
this.singleValueExtendedProperties = singleValueExtendedProperties;
return this;
}
/**
* Get the additionalProperties property: contact.
*
* @return the additionalProperties value.
*/
public Map additionalProperties() {
return this.additionalProperties;
}
/**
* Set the additionalProperties property: contact.
*
* @param additionalProperties the additionalProperties value to set.
* @return the MicrosoftGraphContact object itself.
*/
public MicrosoftGraphContact withAdditionalProperties(Map additionalProperties) {
this.additionalProperties = additionalProperties;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphContact withCategories(List categories) {
super.withCategories(categories);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphContact withChangeKey(String changeKey) {
super.withChangeKey(changeKey);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphContact withCreatedDateTime(OffsetDateTime createdDateTime) {
super.withCreatedDateTime(createdDateTime);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphContact withLastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
super.withLastModifiedDateTime(lastModifiedDateTime);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphContact withId(String id) {
super.withId(id);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (businessAddress() != null) {
businessAddress().validate();
}
if (emailAddresses() != null) {
emailAddresses().forEach(e -> e.validate());
}
if (homeAddress() != null) {
homeAddress().validate();
}
if (otherAddress() != null) {
otherAddress().validate();
}
if (extensions() != null) {
extensions().forEach(e -> e.validate());
}
if (multiValueExtendedProperties() != null) {
multiValueExtendedProperties().forEach(e -> e.validate());
}
if (photo() != null) {
photo().validate();
}
if (singleValueExtendedProperties() != null) {
singleValueExtendedProperties().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("id", id());
jsonWriter.writeArrayField("categories", categories(), (writer, element) -> writer.writeString(element));
jsonWriter.writeStringField("changeKey", changeKey());
jsonWriter.writeStringField("createdDateTime",
createdDateTime() == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(createdDateTime()));
jsonWriter.writeStringField("lastModifiedDateTime",
lastModifiedDateTime() == null
? null
: DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(lastModifiedDateTime()));
jsonWriter.writeStringField("assistantName", this.assistantName);
jsonWriter.writeStringField("birthday",
this.birthday == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.birthday));
jsonWriter.writeJsonField("businessAddress", this.businessAddress);
jsonWriter.writeStringField("businessHomePage", this.businessHomePage);
jsonWriter.writeArrayField("businessPhones", this.businessPhones,
(writer, element) -> writer.writeString(element));
jsonWriter.writeArrayField("children", this.children, (writer, element) -> writer.writeString(element));
jsonWriter.writeStringField("companyName", this.companyName);
jsonWriter.writeStringField("department", this.department);
jsonWriter.writeStringField("displayName", this.displayName);
jsonWriter.writeArrayField("emailAddresses", this.emailAddresses,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("fileAs", this.fileAs);
jsonWriter.writeStringField("generation", this.generation);
jsonWriter.writeStringField("givenName", this.givenName);
jsonWriter.writeJsonField("homeAddress", this.homeAddress);
jsonWriter.writeArrayField("homePhones", this.homePhones, (writer, element) -> writer.writeString(element));
jsonWriter.writeArrayField("imAddresses", this.imAddresses, (writer, element) -> writer.writeString(element));
jsonWriter.writeStringField("initials", this.initials);
jsonWriter.writeStringField("jobTitle", this.jobTitle);
jsonWriter.writeStringField("manager", this.manager);
jsonWriter.writeStringField("middleName", this.middleName);
jsonWriter.writeStringField("mobilePhone", this.mobilePhone);
jsonWriter.writeStringField("nickName", this.nickName);
jsonWriter.writeStringField("officeLocation", this.officeLocation);
jsonWriter.writeJsonField("otherAddress", this.otherAddress);
jsonWriter.writeStringField("parentFolderId", this.parentFolderId);
jsonWriter.writeStringField("personalNotes", this.personalNotes);
jsonWriter.writeStringField("profession", this.profession);
jsonWriter.writeStringField("spouseName", this.spouseName);
jsonWriter.writeStringField("surname", this.surname);
jsonWriter.writeStringField("title", this.title);
jsonWriter.writeStringField("yomiCompanyName", this.yomiCompanyName);
jsonWriter.writeStringField("yomiGivenName", this.yomiGivenName);
jsonWriter.writeStringField("yomiSurname", this.yomiSurname);
jsonWriter.writeArrayField("extensions", this.extensions, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("multiValueExtendedProperties", this.multiValueExtendedProperties,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("photo", this.photo);
jsonWriter.writeArrayField("singleValueExtendedProperties", this.singleValueExtendedProperties,
(writer, element) -> writer.writeJson(element));
if (additionalProperties != null) {
for (Map.Entry additionalProperty : additionalProperties.entrySet()) {
jsonWriter.writeUntypedField(additionalProperty.getKey(), additionalProperty.getValue());
}
}
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MicrosoftGraphContact from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MicrosoftGraphContact if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the MicrosoftGraphContact.
*/
public static MicrosoftGraphContact fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
MicrosoftGraphContact deserializedMicrosoftGraphContact = new MicrosoftGraphContact();
Map additionalProperties = null;
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedMicrosoftGraphContact.withId(reader.getString());
} else if ("categories".equals(fieldName)) {
List categories = reader.readArray(reader1 -> reader1.getString());
deserializedMicrosoftGraphContact.withCategories(categories);
} else if ("changeKey".equals(fieldName)) {
deserializedMicrosoftGraphContact.withChangeKey(reader.getString());
} else if ("createdDateTime".equals(fieldName)) {
deserializedMicrosoftGraphContact.withCreatedDateTime(reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString())));
} else if ("lastModifiedDateTime".equals(fieldName)) {
deserializedMicrosoftGraphContact.withLastModifiedDateTime(reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString())));
} else if ("assistantName".equals(fieldName)) {
deserializedMicrosoftGraphContact.assistantName = reader.getString();
} else if ("birthday".equals(fieldName)) {
deserializedMicrosoftGraphContact.birthday = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("businessAddress".equals(fieldName)) {
deserializedMicrosoftGraphContact.businessAddress = MicrosoftGraphPhysicalAddress.fromJson(reader);
} else if ("businessHomePage".equals(fieldName)) {
deserializedMicrosoftGraphContact.businessHomePage = reader.getString();
} else if ("businessPhones".equals(fieldName)) {
List businessPhones = reader.readArray(reader1 -> reader1.getString());
deserializedMicrosoftGraphContact.businessPhones = businessPhones;
} else if ("children".equals(fieldName)) {
List children = reader.readArray(reader1 -> reader1.getString());
deserializedMicrosoftGraphContact.children = children;
} else if ("companyName".equals(fieldName)) {
deserializedMicrosoftGraphContact.companyName = reader.getString();
} else if ("department".equals(fieldName)) {
deserializedMicrosoftGraphContact.department = reader.getString();
} else if ("displayName".equals(fieldName)) {
deserializedMicrosoftGraphContact.displayName = reader.getString();
} else if ("emailAddresses".equals(fieldName)) {
List emailAddresses
= reader.readArray(reader1 -> MicrosoftGraphEmailAddress.fromJson(reader1));
deserializedMicrosoftGraphContact.emailAddresses = emailAddresses;
} else if ("fileAs".equals(fieldName)) {
deserializedMicrosoftGraphContact.fileAs = reader.getString();
} else if ("generation".equals(fieldName)) {
deserializedMicrosoftGraphContact.generation = reader.getString();
} else if ("givenName".equals(fieldName)) {
deserializedMicrosoftGraphContact.givenName = reader.getString();
} else if ("homeAddress".equals(fieldName)) {
deserializedMicrosoftGraphContact.homeAddress = MicrosoftGraphPhysicalAddress.fromJson(reader);
} else if ("homePhones".equals(fieldName)) {
List homePhones = reader.readArray(reader1 -> reader1.getString());
deserializedMicrosoftGraphContact.homePhones = homePhones;
} else if ("imAddresses".equals(fieldName)) {
List imAddresses = reader.readArray(reader1 -> reader1.getString());
deserializedMicrosoftGraphContact.imAddresses = imAddresses;
} else if ("initials".equals(fieldName)) {
deserializedMicrosoftGraphContact.initials = reader.getString();
} else if ("jobTitle".equals(fieldName)) {
deserializedMicrosoftGraphContact.jobTitle = reader.getString();
} else if ("manager".equals(fieldName)) {
deserializedMicrosoftGraphContact.manager = reader.getString();
} else if ("middleName".equals(fieldName)) {
deserializedMicrosoftGraphContact.middleName = reader.getString();
} else if ("mobilePhone".equals(fieldName)) {
deserializedMicrosoftGraphContact.mobilePhone = reader.getString();
} else if ("nickName".equals(fieldName)) {
deserializedMicrosoftGraphContact.nickName = reader.getString();
} else if ("officeLocation".equals(fieldName)) {
deserializedMicrosoftGraphContact.officeLocation = reader.getString();
} else if ("otherAddress".equals(fieldName)) {
deserializedMicrosoftGraphContact.otherAddress = MicrosoftGraphPhysicalAddress.fromJson(reader);
} else if ("parentFolderId".equals(fieldName)) {
deserializedMicrosoftGraphContact.parentFolderId = reader.getString();
} else if ("personalNotes".equals(fieldName)) {
deserializedMicrosoftGraphContact.personalNotes = reader.getString();
} else if ("profession".equals(fieldName)) {
deserializedMicrosoftGraphContact.profession = reader.getString();
} else if ("spouseName".equals(fieldName)) {
deserializedMicrosoftGraphContact.spouseName = reader.getString();
} else if ("surname".equals(fieldName)) {
deserializedMicrosoftGraphContact.surname = reader.getString();
} else if ("title".equals(fieldName)) {
deserializedMicrosoftGraphContact.title = reader.getString();
} else if ("yomiCompanyName".equals(fieldName)) {
deserializedMicrosoftGraphContact.yomiCompanyName = reader.getString();
} else if ("yomiGivenName".equals(fieldName)) {
deserializedMicrosoftGraphContact.yomiGivenName = reader.getString();
} else if ("yomiSurname".equals(fieldName)) {
deserializedMicrosoftGraphContact.yomiSurname = reader.getString();
} else if ("extensions".equals(fieldName)) {
List extensions
= reader.readArray(reader1 -> MicrosoftGraphExtension.fromJson(reader1));
deserializedMicrosoftGraphContact.extensions = extensions;
} else if ("multiValueExtendedProperties".equals(fieldName)) {
List multiValueExtendedProperties
= reader.readArray(reader1 -> MicrosoftGraphMultiValueLegacyExtendedProperty.fromJson(reader1));
deserializedMicrosoftGraphContact.multiValueExtendedProperties = multiValueExtendedProperties;
} else if ("photo".equals(fieldName)) {
deserializedMicrosoftGraphContact.photo = MicrosoftGraphProfilePhoto.fromJson(reader);
} else if ("singleValueExtendedProperties".equals(fieldName)) {
List singleValueExtendedProperties = reader
.readArray(reader1 -> MicrosoftGraphSingleValueLegacyExtendedProperty.fromJson(reader1));
deserializedMicrosoftGraphContact.singleValueExtendedProperties = singleValueExtendedProperties;
} else {
if (additionalProperties == null) {
additionalProperties = new LinkedHashMap<>();
}
additionalProperties.put(fieldName, reader.readUntyped());
}
}
deserializedMicrosoftGraphContact.additionalProperties = additionalProperties;
return deserializedMicrosoftGraphContact;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy