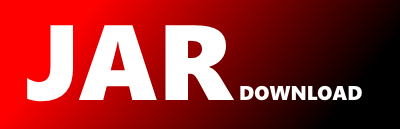
com.azure.resourcemanager.authorization.fluent.models.MicrosoftGraphEvent Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.authorization.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
/**
* event.
*/
@Fluent
public final class MicrosoftGraphEvent extends MicrosoftGraphOutlookItem {
/*
* True if the meeting organizer allows invitees to propose a new time when responding, false otherwise. Optional.
* Default is true.
*/
private Boolean allowNewTimeProposals;
/*
* The collection of attendees for the event.
*/
private List attendees;
/*
* itemBody
*/
private MicrosoftGraphItemBody body;
/*
* The preview of the message associated with the event. It is in text format.
*/
private String bodyPreview;
/*
* dateTimeTimeZone
*/
private MicrosoftGraphDateTimeZone end;
/*
* Set to true if the event has attachments.
*/
private Boolean hasAttachments;
/*
* The hideAttendees property.
*/
private Boolean hideAttendees;
/*
* A unique identifier for an event across calendars. This ID is different for each occurrence in a recurring
* series. Read-only.
*/
private String iCalUId;
/*
* importance
*/
private MicrosoftGraphImportance importance;
/*
* Set to true if the event lasts all day.
*/
private Boolean isAllDay;
/*
* Set to true if the event has been canceled.
*/
private Boolean isCancelled;
/*
* The isDraft property.
*/
private Boolean isDraft;
/*
* True if this event has online meeting information, false otherwise. Default is false. Optional.
*/
private Boolean isOnlineMeeting;
/*
* Set to true if the calendar owner (specified by the owner property of the calendar) is the organizer of the event
* (specified by the organizer property of the event). This also applies if a delegate organized the event on behalf
* of the owner.
*/
private Boolean isOrganizer;
/*
* Set to true if an alert is set to remind the user of the event.
*/
private Boolean isReminderOn;
/*
* location
*/
private MicrosoftGraphLocation location;
/*
* The locations where the event is held or attended from. The location and locations properties always correspond
* with each other. If you update the location property, any prior locations in the locations collection would be
* removed and replaced by the new location value.
*/
private List locations;
/*
* onlineMeetingInfo
*/
private MicrosoftGraphOnlineMeetingInfo onlineMeeting;
/*
* onlineMeetingProviderType
*/
private MicrosoftGraphOnlineMeetingProviderType onlineMeetingProvider;
/*
* A URL for an online meeting. The property is set only when an organizer specifies an event as an online meeting
* such as a Skype meeting. Read-only.
*/
private String onlineMeetingUrl;
/*
* recipient
*/
private MicrosoftGraphRecipient organizer;
/*
* The end time zone that was set when the event was created. A value of tzone://Microsoft/Custom indicates that a
* legacy custom time zone was set in desktop Outlook.
*/
private String originalEndTimeZone;
/*
* The Timestamp type represents date and time information using ISO 8601 format and is always in UTC time. For
* example, midnight UTC on Jan 1, 2014 would look like this: '2014-01-01T00:00:00Z'
*/
private OffsetDateTime originalStart;
/*
* The start time zone that was set when the event was created. A value of tzone://Microsoft/Custom indicates that a
* legacy custom time zone was set in desktop Outlook.
*/
private String originalStartTimeZone;
/*
* patternedRecurrence
*/
private MicrosoftGraphPatternedRecurrence recurrence;
/*
* The number of minutes before the event start time that the reminder alert occurs.
*/
private Integer reminderMinutesBeforeStart;
/*
* Default is true, which represents the organizer would like an invitee to send a response to the event.
*/
private Boolean responseRequested;
/*
* responseStatus
*/
private MicrosoftGraphResponseStatus responseStatus;
/*
* sensitivity
*/
private MicrosoftGraphSensitivity sensitivity;
/*
* The ID for the recurring series master item, if this event is part of a recurring series.
*/
private String seriesMasterId;
/*
* freeBusyStatus
*/
private MicrosoftGraphFreeBusyStatus showAs;
/*
* dateTimeTimeZone
*/
private MicrosoftGraphDateTimeZone start;
/*
* The text of the event's subject line.
*/
private String subject;
/*
* A custom identifier specified by a client app for the server to avoid redundant POST operations in case of client
* retries to create the same event. This is useful when low network connectivity causes the client to time out
* before receiving a response from the server for the client's prior create-event request. After you set
* transactionId when creating an event, you cannot change transactionId in a subsequent update. This property is
* only returned in a response payload if an app has set it. Optional.
*/
private String transactionId;
/*
* eventType
*/
private MicrosoftGraphEventType type;
/*
* The URL to open the event in Outlook on the web.Outlook on the web opens the event in the browser if you are
* signed in to your mailbox. Otherwise, Outlook on the web prompts you to sign in.This URL cannot be accessed from
* within an iFrame.
*/
private String webLink;
/*
* The collection of fileAttachment and itemAttachment attachments for the event. Navigation property. Read-only.
* Nullable.
*/
private List attachments;
/*
* calendar
*/
private MicrosoftGraphCalendar calendar;
/*
* The collection of open extensions defined for the event. Read-only. Nullable.
*/
private List extensions;
/*
* The instances of the event. Navigation property. Read-only. Nullable.
*/
private List instances;
/*
* The collection of multi-value extended properties defined for the event. Read-only. Nullable.
*/
private List multiValueExtendedProperties;
/*
* The collection of single-value extended properties defined for the event. Read-only. Nullable.
*/
private List singleValueExtendedProperties;
/*
* event
*/
private Map additionalProperties;
/**
* Creates an instance of MicrosoftGraphEvent class.
*/
public MicrosoftGraphEvent() {
}
/**
* Get the allowNewTimeProposals property: True if the meeting organizer allows invitees to propose a new time when
* responding, false otherwise. Optional. Default is true.
*
* @return the allowNewTimeProposals value.
*/
public Boolean allowNewTimeProposals() {
return this.allowNewTimeProposals;
}
/**
* Set the allowNewTimeProposals property: True if the meeting organizer allows invitees to propose a new time when
* responding, false otherwise. Optional. Default is true.
*
* @param allowNewTimeProposals the allowNewTimeProposals value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withAllowNewTimeProposals(Boolean allowNewTimeProposals) {
this.allowNewTimeProposals = allowNewTimeProposals;
return this;
}
/**
* Get the attendees property: The collection of attendees for the event.
*
* @return the attendees value.
*/
public List attendees() {
return this.attendees;
}
/**
* Set the attendees property: The collection of attendees for the event.
*
* @param attendees the attendees value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withAttendees(List attendees) {
this.attendees = attendees;
return this;
}
/**
* Get the body property: itemBody.
*
* @return the body value.
*/
public MicrosoftGraphItemBody body() {
return this.body;
}
/**
* Set the body property: itemBody.
*
* @param body the body value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withBody(MicrosoftGraphItemBody body) {
this.body = body;
return this;
}
/**
* Get the bodyPreview property: The preview of the message associated with the event. It is in text format.
*
* @return the bodyPreview value.
*/
public String bodyPreview() {
return this.bodyPreview;
}
/**
* Set the bodyPreview property: The preview of the message associated with the event. It is in text format.
*
* @param bodyPreview the bodyPreview value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withBodyPreview(String bodyPreview) {
this.bodyPreview = bodyPreview;
return this;
}
/**
* Get the end property: dateTimeTimeZone.
*
* @return the end value.
*/
public MicrosoftGraphDateTimeZone end() {
return this.end;
}
/**
* Set the end property: dateTimeTimeZone.
*
* @param end the end value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withEnd(MicrosoftGraphDateTimeZone end) {
this.end = end;
return this;
}
/**
* Get the hasAttachments property: Set to true if the event has attachments.
*
* @return the hasAttachments value.
*/
public Boolean hasAttachments() {
return this.hasAttachments;
}
/**
* Set the hasAttachments property: Set to true if the event has attachments.
*
* @param hasAttachments the hasAttachments value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withHasAttachments(Boolean hasAttachments) {
this.hasAttachments = hasAttachments;
return this;
}
/**
* Get the hideAttendees property: The hideAttendees property.
*
* @return the hideAttendees value.
*/
public Boolean hideAttendees() {
return this.hideAttendees;
}
/**
* Set the hideAttendees property: The hideAttendees property.
*
* @param hideAttendees the hideAttendees value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withHideAttendees(Boolean hideAttendees) {
this.hideAttendees = hideAttendees;
return this;
}
/**
* Get the iCalUId property: A unique identifier for an event across calendars. This ID is different for each
* occurrence in a recurring series. Read-only.
*
* @return the iCalUId value.
*/
public String iCalUId() {
return this.iCalUId;
}
/**
* Set the iCalUId property: A unique identifier for an event across calendars. This ID is different for each
* occurrence in a recurring series. Read-only.
*
* @param iCalUId the iCalUId value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withICalUId(String iCalUId) {
this.iCalUId = iCalUId;
return this;
}
/**
* Get the importance property: importance.
*
* @return the importance value.
*/
public MicrosoftGraphImportance importance() {
return this.importance;
}
/**
* Set the importance property: importance.
*
* @param importance the importance value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withImportance(MicrosoftGraphImportance importance) {
this.importance = importance;
return this;
}
/**
* Get the isAllDay property: Set to true if the event lasts all day.
*
* @return the isAllDay value.
*/
public Boolean isAllDay() {
return this.isAllDay;
}
/**
* Set the isAllDay property: Set to true if the event lasts all day.
*
* @param isAllDay the isAllDay value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withIsAllDay(Boolean isAllDay) {
this.isAllDay = isAllDay;
return this;
}
/**
* Get the isCancelled property: Set to true if the event has been canceled.
*
* @return the isCancelled value.
*/
public Boolean isCancelled() {
return this.isCancelled;
}
/**
* Set the isCancelled property: Set to true if the event has been canceled.
*
* @param isCancelled the isCancelled value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withIsCancelled(Boolean isCancelled) {
this.isCancelled = isCancelled;
return this;
}
/**
* Get the isDraft property: The isDraft property.
*
* @return the isDraft value.
*/
public Boolean isDraft() {
return this.isDraft;
}
/**
* Set the isDraft property: The isDraft property.
*
* @param isDraft the isDraft value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withIsDraft(Boolean isDraft) {
this.isDraft = isDraft;
return this;
}
/**
* Get the isOnlineMeeting property: True if this event has online meeting information, false otherwise. Default is
* false. Optional.
*
* @return the isOnlineMeeting value.
*/
public Boolean isOnlineMeeting() {
return this.isOnlineMeeting;
}
/**
* Set the isOnlineMeeting property: True if this event has online meeting information, false otherwise. Default is
* false. Optional.
*
* @param isOnlineMeeting the isOnlineMeeting value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withIsOnlineMeeting(Boolean isOnlineMeeting) {
this.isOnlineMeeting = isOnlineMeeting;
return this;
}
/**
* Get the isOrganizer property: Set to true if the calendar owner (specified by the owner property of the calendar)
* is the organizer of the event (specified by the organizer property of the event). This also applies if a delegate
* organized the event on behalf of the owner.
*
* @return the isOrganizer value.
*/
public Boolean isOrganizer() {
return this.isOrganizer;
}
/**
* Set the isOrganizer property: Set to true if the calendar owner (specified by the owner property of the calendar)
* is the organizer of the event (specified by the organizer property of the event). This also applies if a delegate
* organized the event on behalf of the owner.
*
* @param isOrganizer the isOrganizer value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withIsOrganizer(Boolean isOrganizer) {
this.isOrganizer = isOrganizer;
return this;
}
/**
* Get the isReminderOn property: Set to true if an alert is set to remind the user of the event.
*
* @return the isReminderOn value.
*/
public Boolean isReminderOn() {
return this.isReminderOn;
}
/**
* Set the isReminderOn property: Set to true if an alert is set to remind the user of the event.
*
* @param isReminderOn the isReminderOn value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withIsReminderOn(Boolean isReminderOn) {
this.isReminderOn = isReminderOn;
return this;
}
/**
* Get the location property: location.
*
* @return the location value.
*/
public MicrosoftGraphLocation location() {
return this.location;
}
/**
* Set the location property: location.
*
* @param location the location value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withLocation(MicrosoftGraphLocation location) {
this.location = location;
return this;
}
/**
* Get the locations property: The locations where the event is held or attended from. The location and locations
* properties always correspond with each other. If you update the location property, any prior locations in the
* locations collection would be removed and replaced by the new location value.
*
* @return the locations value.
*/
public List locations() {
return this.locations;
}
/**
* Set the locations property: The locations where the event is held or attended from. The location and locations
* properties always correspond with each other. If you update the location property, any prior locations in the
* locations collection would be removed and replaced by the new location value.
*
* @param locations the locations value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withLocations(List locations) {
this.locations = locations;
return this;
}
/**
* Get the onlineMeeting property: onlineMeetingInfo.
*
* @return the onlineMeeting value.
*/
public MicrosoftGraphOnlineMeetingInfo onlineMeeting() {
return this.onlineMeeting;
}
/**
* Set the onlineMeeting property: onlineMeetingInfo.
*
* @param onlineMeeting the onlineMeeting value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withOnlineMeeting(MicrosoftGraphOnlineMeetingInfo onlineMeeting) {
this.onlineMeeting = onlineMeeting;
return this;
}
/**
* Get the onlineMeetingProvider property: onlineMeetingProviderType.
*
* @return the onlineMeetingProvider value.
*/
public MicrosoftGraphOnlineMeetingProviderType onlineMeetingProvider() {
return this.onlineMeetingProvider;
}
/**
* Set the onlineMeetingProvider property: onlineMeetingProviderType.
*
* @param onlineMeetingProvider the onlineMeetingProvider value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent
withOnlineMeetingProvider(MicrosoftGraphOnlineMeetingProviderType onlineMeetingProvider) {
this.onlineMeetingProvider = onlineMeetingProvider;
return this;
}
/**
* Get the onlineMeetingUrl property: A URL for an online meeting. The property is set only when an organizer
* specifies an event as an online meeting such as a Skype meeting. Read-only.
*
* @return the onlineMeetingUrl value.
*/
public String onlineMeetingUrl() {
return this.onlineMeetingUrl;
}
/**
* Set the onlineMeetingUrl property: A URL for an online meeting. The property is set only when an organizer
* specifies an event as an online meeting such as a Skype meeting. Read-only.
*
* @param onlineMeetingUrl the onlineMeetingUrl value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withOnlineMeetingUrl(String onlineMeetingUrl) {
this.onlineMeetingUrl = onlineMeetingUrl;
return this;
}
/**
* Get the organizer property: recipient.
*
* @return the organizer value.
*/
public MicrosoftGraphRecipient organizer() {
return this.organizer;
}
/**
* Set the organizer property: recipient.
*
* @param organizer the organizer value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withOrganizer(MicrosoftGraphRecipient organizer) {
this.organizer = organizer;
return this;
}
/**
* Get the originalEndTimeZone property: The end time zone that was set when the event was created. A value of
* tzone://Microsoft/Custom indicates that a legacy custom time zone was set in desktop Outlook.
*
* @return the originalEndTimeZone value.
*/
public String originalEndTimeZone() {
return this.originalEndTimeZone;
}
/**
* Set the originalEndTimeZone property: The end time zone that was set when the event was created. A value of
* tzone://Microsoft/Custom indicates that a legacy custom time zone was set in desktop Outlook.
*
* @param originalEndTimeZone the originalEndTimeZone value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withOriginalEndTimeZone(String originalEndTimeZone) {
this.originalEndTimeZone = originalEndTimeZone;
return this;
}
/**
* Get the originalStart property: The Timestamp type represents date and time information using ISO 8601 format and
* is always in UTC time. For example, midnight UTC on Jan 1, 2014 would look like this: '2014-01-01T00:00:00Z'.
*
* @return the originalStart value.
*/
public OffsetDateTime originalStart() {
return this.originalStart;
}
/**
* Set the originalStart property: The Timestamp type represents date and time information using ISO 8601 format and
* is always in UTC time. For example, midnight UTC on Jan 1, 2014 would look like this: '2014-01-01T00:00:00Z'.
*
* @param originalStart the originalStart value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withOriginalStart(OffsetDateTime originalStart) {
this.originalStart = originalStart;
return this;
}
/**
* Get the originalStartTimeZone property: The start time zone that was set when the event was created. A value of
* tzone://Microsoft/Custom indicates that a legacy custom time zone was set in desktop Outlook.
*
* @return the originalStartTimeZone value.
*/
public String originalStartTimeZone() {
return this.originalStartTimeZone;
}
/**
* Set the originalStartTimeZone property: The start time zone that was set when the event was created. A value of
* tzone://Microsoft/Custom indicates that a legacy custom time zone was set in desktop Outlook.
*
* @param originalStartTimeZone the originalStartTimeZone value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withOriginalStartTimeZone(String originalStartTimeZone) {
this.originalStartTimeZone = originalStartTimeZone;
return this;
}
/**
* Get the recurrence property: patternedRecurrence.
*
* @return the recurrence value.
*/
public MicrosoftGraphPatternedRecurrence recurrence() {
return this.recurrence;
}
/**
* Set the recurrence property: patternedRecurrence.
*
* @param recurrence the recurrence value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withRecurrence(MicrosoftGraphPatternedRecurrence recurrence) {
this.recurrence = recurrence;
return this;
}
/**
* Get the reminderMinutesBeforeStart property: The number of minutes before the event start time that the reminder
* alert occurs.
*
* @return the reminderMinutesBeforeStart value.
*/
public Integer reminderMinutesBeforeStart() {
return this.reminderMinutesBeforeStart;
}
/**
* Set the reminderMinutesBeforeStart property: The number of minutes before the event start time that the reminder
* alert occurs.
*
* @param reminderMinutesBeforeStart the reminderMinutesBeforeStart value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withReminderMinutesBeforeStart(Integer reminderMinutesBeforeStart) {
this.reminderMinutesBeforeStart = reminderMinutesBeforeStart;
return this;
}
/**
* Get the responseRequested property: Default is true, which represents the organizer would like an invitee to send
* a response to the event.
*
* @return the responseRequested value.
*/
public Boolean responseRequested() {
return this.responseRequested;
}
/**
* Set the responseRequested property: Default is true, which represents the organizer would like an invitee to send
* a response to the event.
*
* @param responseRequested the responseRequested value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withResponseRequested(Boolean responseRequested) {
this.responseRequested = responseRequested;
return this;
}
/**
* Get the responseStatus property: responseStatus.
*
* @return the responseStatus value.
*/
public MicrosoftGraphResponseStatus responseStatus() {
return this.responseStatus;
}
/**
* Set the responseStatus property: responseStatus.
*
* @param responseStatus the responseStatus value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withResponseStatus(MicrosoftGraphResponseStatus responseStatus) {
this.responseStatus = responseStatus;
return this;
}
/**
* Get the sensitivity property: sensitivity.
*
* @return the sensitivity value.
*/
public MicrosoftGraphSensitivity sensitivity() {
return this.sensitivity;
}
/**
* Set the sensitivity property: sensitivity.
*
* @param sensitivity the sensitivity value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withSensitivity(MicrosoftGraphSensitivity sensitivity) {
this.sensitivity = sensitivity;
return this;
}
/**
* Get the seriesMasterId property: The ID for the recurring series master item, if this event is part of a
* recurring series.
*
* @return the seriesMasterId value.
*/
public String seriesMasterId() {
return this.seriesMasterId;
}
/**
* Set the seriesMasterId property: The ID for the recurring series master item, if this event is part of a
* recurring series.
*
* @param seriesMasterId the seriesMasterId value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withSeriesMasterId(String seriesMasterId) {
this.seriesMasterId = seriesMasterId;
return this;
}
/**
* Get the showAs property: freeBusyStatus.
*
* @return the showAs value.
*/
public MicrosoftGraphFreeBusyStatus showAs() {
return this.showAs;
}
/**
* Set the showAs property: freeBusyStatus.
*
* @param showAs the showAs value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withShowAs(MicrosoftGraphFreeBusyStatus showAs) {
this.showAs = showAs;
return this;
}
/**
* Get the start property: dateTimeTimeZone.
*
* @return the start value.
*/
public MicrosoftGraphDateTimeZone start() {
return this.start;
}
/**
* Set the start property: dateTimeTimeZone.
*
* @param start the start value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withStart(MicrosoftGraphDateTimeZone start) {
this.start = start;
return this;
}
/**
* Get the subject property: The text of the event's subject line.
*
* @return the subject value.
*/
public String subject() {
return this.subject;
}
/**
* Set the subject property: The text of the event's subject line.
*
* @param subject the subject value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withSubject(String subject) {
this.subject = subject;
return this;
}
/**
* Get the transactionId property: A custom identifier specified by a client app for the server to avoid redundant
* POST operations in case of client retries to create the same event. This is useful when low network connectivity
* causes the client to time out before receiving a response from the server for the client's prior create-event
* request. After you set transactionId when creating an event, you cannot change transactionId in a subsequent
* update. This property is only returned in a response payload if an app has set it. Optional.
*
* @return the transactionId value.
*/
public String transactionId() {
return this.transactionId;
}
/**
* Set the transactionId property: A custom identifier specified by a client app for the server to avoid redundant
* POST operations in case of client retries to create the same event. This is useful when low network connectivity
* causes the client to time out before receiving a response from the server for the client's prior create-event
* request. After you set transactionId when creating an event, you cannot change transactionId in a subsequent
* update. This property is only returned in a response payload if an app has set it. Optional.
*
* @param transactionId the transactionId value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withTransactionId(String transactionId) {
this.transactionId = transactionId;
return this;
}
/**
* Get the type property: eventType.
*
* @return the type value.
*/
public MicrosoftGraphEventType type() {
return this.type;
}
/**
* Set the type property: eventType.
*
* @param type the type value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withType(MicrosoftGraphEventType type) {
this.type = type;
return this;
}
/**
* Get the webLink property: The URL to open the event in Outlook on the web.Outlook on the web opens the event in
* the browser if you are signed in to your mailbox. Otherwise, Outlook on the web prompts you to sign in.This URL
* cannot be accessed from within an iFrame.
*
* @return the webLink value.
*/
public String webLink() {
return this.webLink;
}
/**
* Set the webLink property: The URL to open the event in Outlook on the web.Outlook on the web opens the event in
* the browser if you are signed in to your mailbox. Otherwise, Outlook on the web prompts you to sign in.This URL
* cannot be accessed from within an iFrame.
*
* @param webLink the webLink value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withWebLink(String webLink) {
this.webLink = webLink;
return this;
}
/**
* Get the attachments property: The collection of fileAttachment and itemAttachment attachments for the event.
* Navigation property. Read-only. Nullable.
*
* @return the attachments value.
*/
public List attachments() {
return this.attachments;
}
/**
* Set the attachments property: The collection of fileAttachment and itemAttachment attachments for the event.
* Navigation property. Read-only. Nullable.
*
* @param attachments the attachments value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withAttachments(List attachments) {
this.attachments = attachments;
return this;
}
/**
* Get the calendar property: calendar.
*
* @return the calendar value.
*/
public MicrosoftGraphCalendar calendar() {
return this.calendar;
}
/**
* Set the calendar property: calendar.
*
* @param calendar the calendar value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withCalendar(MicrosoftGraphCalendar calendar) {
this.calendar = calendar;
return this;
}
/**
* Get the extensions property: The collection of open extensions defined for the event. Read-only. Nullable.
*
* @return the extensions value.
*/
public List extensions() {
return this.extensions;
}
/**
* Set the extensions property: The collection of open extensions defined for the event. Read-only. Nullable.
*
* @param extensions the extensions value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withExtensions(List extensions) {
this.extensions = extensions;
return this;
}
/**
* Get the instances property: The instances of the event. Navigation property. Read-only. Nullable.
*
* @return the instances value.
*/
public List instances() {
return this.instances;
}
/**
* Set the instances property: The instances of the event. Navigation property. Read-only. Nullable.
*
* @param instances the instances value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withInstances(List instances) {
this.instances = instances;
return this;
}
/**
* Get the multiValueExtendedProperties property: The collection of multi-value extended properties defined for the
* event. Read-only. Nullable.
*
* @return the multiValueExtendedProperties value.
*/
public List multiValueExtendedProperties() {
return this.multiValueExtendedProperties;
}
/**
* Set the multiValueExtendedProperties property: The collection of multi-value extended properties defined for the
* event. Read-only. Nullable.
*
* @param multiValueExtendedProperties the multiValueExtendedProperties value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withMultiValueExtendedProperties(
List multiValueExtendedProperties) {
this.multiValueExtendedProperties = multiValueExtendedProperties;
return this;
}
/**
* Get the singleValueExtendedProperties property: The collection of single-value extended properties defined for
* the event. Read-only. Nullable.
*
* @return the singleValueExtendedProperties value.
*/
public List singleValueExtendedProperties() {
return this.singleValueExtendedProperties;
}
/**
* Set the singleValueExtendedProperties property: The collection of single-value extended properties defined for
* the event. Read-only. Nullable.
*
* @param singleValueExtendedProperties the singleValueExtendedProperties value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withSingleValueExtendedProperties(
List singleValueExtendedProperties) {
this.singleValueExtendedProperties = singleValueExtendedProperties;
return this;
}
/**
* Get the additionalProperties property: event.
*
* @return the additionalProperties value.
*/
public Map additionalProperties() {
return this.additionalProperties;
}
/**
* Set the additionalProperties property: event.
*
* @param additionalProperties the additionalProperties value to set.
* @return the MicrosoftGraphEvent object itself.
*/
public MicrosoftGraphEvent withAdditionalProperties(Map additionalProperties) {
this.additionalProperties = additionalProperties;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphEvent withCategories(List categories) {
super.withCategories(categories);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphEvent withChangeKey(String changeKey) {
super.withChangeKey(changeKey);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphEvent withCreatedDateTime(OffsetDateTime createdDateTime) {
super.withCreatedDateTime(createdDateTime);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphEvent withLastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
super.withLastModifiedDateTime(lastModifiedDateTime);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphEvent withId(String id) {
super.withId(id);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (attendees() != null) {
attendees().forEach(e -> e.validate());
}
if (body() != null) {
body().validate();
}
if (end() != null) {
end().validate();
}
if (location() != null) {
location().validate();
}
if (locations() != null) {
locations().forEach(e -> e.validate());
}
if (onlineMeeting() != null) {
onlineMeeting().validate();
}
if (organizer() != null) {
organizer().validate();
}
if (recurrence() != null) {
recurrence().validate();
}
if (responseStatus() != null) {
responseStatus().validate();
}
if (start() != null) {
start().validate();
}
if (attachments() != null) {
attachments().forEach(e -> e.validate());
}
if (calendar() != null) {
calendar().validate();
}
if (extensions() != null) {
extensions().forEach(e -> e.validate());
}
if (instances() != null) {
instances().forEach(e -> e.validate());
}
if (multiValueExtendedProperties() != null) {
multiValueExtendedProperties().forEach(e -> e.validate());
}
if (singleValueExtendedProperties() != null) {
singleValueExtendedProperties().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("id", id());
jsonWriter.writeArrayField("categories", categories(), (writer, element) -> writer.writeString(element));
jsonWriter.writeStringField("changeKey", changeKey());
jsonWriter.writeStringField("createdDateTime",
createdDateTime() == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(createdDateTime()));
jsonWriter.writeStringField("lastModifiedDateTime",
lastModifiedDateTime() == null
? null
: DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(lastModifiedDateTime()));
jsonWriter.writeBooleanField("allowNewTimeProposals", this.allowNewTimeProposals);
jsonWriter.writeArrayField("attendees", this.attendees, (writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("body", this.body);
jsonWriter.writeStringField("bodyPreview", this.bodyPreview);
jsonWriter.writeJsonField("end", this.end);
jsonWriter.writeBooleanField("hasAttachments", this.hasAttachments);
jsonWriter.writeBooleanField("hideAttendees", this.hideAttendees);
jsonWriter.writeStringField("iCalUId", this.iCalUId);
jsonWriter.writeStringField("importance", this.importance == null ? null : this.importance.toString());
jsonWriter.writeBooleanField("isAllDay", this.isAllDay);
jsonWriter.writeBooleanField("isCancelled", this.isCancelled);
jsonWriter.writeBooleanField("isDraft", this.isDraft);
jsonWriter.writeBooleanField("isOnlineMeeting", this.isOnlineMeeting);
jsonWriter.writeBooleanField("isOrganizer", this.isOrganizer);
jsonWriter.writeBooleanField("isReminderOn", this.isReminderOn);
jsonWriter.writeJsonField("location", this.location);
jsonWriter.writeArrayField("locations", this.locations, (writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("onlineMeeting", this.onlineMeeting);
jsonWriter.writeStringField("onlineMeetingProvider",
this.onlineMeetingProvider == null ? null : this.onlineMeetingProvider.toString());
jsonWriter.writeStringField("onlineMeetingUrl", this.onlineMeetingUrl);
jsonWriter.writeJsonField("organizer", this.organizer);
jsonWriter.writeStringField("originalEndTimeZone", this.originalEndTimeZone);
jsonWriter.writeStringField("originalStart",
this.originalStart == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.originalStart));
jsonWriter.writeStringField("originalStartTimeZone", this.originalStartTimeZone);
jsonWriter.writeJsonField("recurrence", this.recurrence);
jsonWriter.writeNumberField("reminderMinutesBeforeStart", this.reminderMinutesBeforeStart);
jsonWriter.writeBooleanField("responseRequested", this.responseRequested);
jsonWriter.writeJsonField("responseStatus", this.responseStatus);
jsonWriter.writeStringField("sensitivity", this.sensitivity == null ? null : this.sensitivity.toString());
jsonWriter.writeStringField("seriesMasterId", this.seriesMasterId);
jsonWriter.writeStringField("showAs", this.showAs == null ? null : this.showAs.toString());
jsonWriter.writeJsonField("start", this.start);
jsonWriter.writeStringField("subject", this.subject);
jsonWriter.writeStringField("transactionId", this.transactionId);
jsonWriter.writeStringField("type", this.type == null ? null : this.type.toString());
jsonWriter.writeStringField("webLink", this.webLink);
jsonWriter.writeArrayField("attachments", this.attachments, (writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("calendar", this.calendar);
jsonWriter.writeArrayField("extensions", this.extensions, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("instances", this.instances, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("multiValueExtendedProperties", this.multiValueExtendedProperties,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("singleValueExtendedProperties", this.singleValueExtendedProperties,
(writer, element) -> writer.writeJson(element));
if (additionalProperties != null) {
for (Map.Entry additionalProperty : additionalProperties.entrySet()) {
jsonWriter.writeUntypedField(additionalProperty.getKey(), additionalProperty.getValue());
}
}
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MicrosoftGraphEvent from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MicrosoftGraphEvent if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the MicrosoftGraphEvent.
*/
public static MicrosoftGraphEvent fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
MicrosoftGraphEvent deserializedMicrosoftGraphEvent = new MicrosoftGraphEvent();
Map additionalProperties = null;
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedMicrosoftGraphEvent.withId(reader.getString());
} else if ("categories".equals(fieldName)) {
List categories = reader.readArray(reader1 -> reader1.getString());
deserializedMicrosoftGraphEvent.withCategories(categories);
} else if ("changeKey".equals(fieldName)) {
deserializedMicrosoftGraphEvent.withChangeKey(reader.getString());
} else if ("createdDateTime".equals(fieldName)) {
deserializedMicrosoftGraphEvent.withCreatedDateTime(reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString())));
} else if ("lastModifiedDateTime".equals(fieldName)) {
deserializedMicrosoftGraphEvent.withLastModifiedDateTime(reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString())));
} else if ("allowNewTimeProposals".equals(fieldName)) {
deserializedMicrosoftGraphEvent.allowNewTimeProposals = reader.getNullable(JsonReader::getBoolean);
} else if ("attendees".equals(fieldName)) {
List attendees
= reader.readArray(reader1 -> MicrosoftGraphAttendee.fromJson(reader1));
deserializedMicrosoftGraphEvent.attendees = attendees;
} else if ("body".equals(fieldName)) {
deserializedMicrosoftGraphEvent.body = MicrosoftGraphItemBody.fromJson(reader);
} else if ("bodyPreview".equals(fieldName)) {
deserializedMicrosoftGraphEvent.bodyPreview = reader.getString();
} else if ("end".equals(fieldName)) {
deserializedMicrosoftGraphEvent.end = MicrosoftGraphDateTimeZone.fromJson(reader);
} else if ("hasAttachments".equals(fieldName)) {
deserializedMicrosoftGraphEvent.hasAttachments = reader.getNullable(JsonReader::getBoolean);
} else if ("hideAttendees".equals(fieldName)) {
deserializedMicrosoftGraphEvent.hideAttendees = reader.getNullable(JsonReader::getBoolean);
} else if ("iCalUId".equals(fieldName)) {
deserializedMicrosoftGraphEvent.iCalUId = reader.getString();
} else if ("importance".equals(fieldName)) {
deserializedMicrosoftGraphEvent.importance
= MicrosoftGraphImportance.fromString(reader.getString());
} else if ("isAllDay".equals(fieldName)) {
deserializedMicrosoftGraphEvent.isAllDay = reader.getNullable(JsonReader::getBoolean);
} else if ("isCancelled".equals(fieldName)) {
deserializedMicrosoftGraphEvent.isCancelled = reader.getNullable(JsonReader::getBoolean);
} else if ("isDraft".equals(fieldName)) {
deserializedMicrosoftGraphEvent.isDraft = reader.getNullable(JsonReader::getBoolean);
} else if ("isOnlineMeeting".equals(fieldName)) {
deserializedMicrosoftGraphEvent.isOnlineMeeting = reader.getNullable(JsonReader::getBoolean);
} else if ("isOrganizer".equals(fieldName)) {
deserializedMicrosoftGraphEvent.isOrganizer = reader.getNullable(JsonReader::getBoolean);
} else if ("isReminderOn".equals(fieldName)) {
deserializedMicrosoftGraphEvent.isReminderOn = reader.getNullable(JsonReader::getBoolean);
} else if ("location".equals(fieldName)) {
deserializedMicrosoftGraphEvent.location = MicrosoftGraphLocation.fromJson(reader);
} else if ("locations".equals(fieldName)) {
List locations
= reader.readArray(reader1 -> MicrosoftGraphLocation.fromJson(reader1));
deserializedMicrosoftGraphEvent.locations = locations;
} else if ("onlineMeeting".equals(fieldName)) {
deserializedMicrosoftGraphEvent.onlineMeeting = MicrosoftGraphOnlineMeetingInfo.fromJson(reader);
} else if ("onlineMeetingProvider".equals(fieldName)) {
deserializedMicrosoftGraphEvent.onlineMeetingProvider
= MicrosoftGraphOnlineMeetingProviderType.fromString(reader.getString());
} else if ("onlineMeetingUrl".equals(fieldName)) {
deserializedMicrosoftGraphEvent.onlineMeetingUrl = reader.getString();
} else if ("organizer".equals(fieldName)) {
deserializedMicrosoftGraphEvent.organizer = MicrosoftGraphRecipient.fromJson(reader);
} else if ("originalEndTimeZone".equals(fieldName)) {
deserializedMicrosoftGraphEvent.originalEndTimeZone = reader.getString();
} else if ("originalStart".equals(fieldName)) {
deserializedMicrosoftGraphEvent.originalStart = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("originalStartTimeZone".equals(fieldName)) {
deserializedMicrosoftGraphEvent.originalStartTimeZone = reader.getString();
} else if ("recurrence".equals(fieldName)) {
deserializedMicrosoftGraphEvent.recurrence = MicrosoftGraphPatternedRecurrence.fromJson(reader);
} else if ("reminderMinutesBeforeStart".equals(fieldName)) {
deserializedMicrosoftGraphEvent.reminderMinutesBeforeStart = reader.getNullable(JsonReader::getInt);
} else if ("responseRequested".equals(fieldName)) {
deserializedMicrosoftGraphEvent.responseRequested = reader.getNullable(JsonReader::getBoolean);
} else if ("responseStatus".equals(fieldName)) {
deserializedMicrosoftGraphEvent.responseStatus = MicrosoftGraphResponseStatus.fromJson(reader);
} else if ("sensitivity".equals(fieldName)) {
deserializedMicrosoftGraphEvent.sensitivity
= MicrosoftGraphSensitivity.fromString(reader.getString());
} else if ("seriesMasterId".equals(fieldName)) {
deserializedMicrosoftGraphEvent.seriesMasterId = reader.getString();
} else if ("showAs".equals(fieldName)) {
deserializedMicrosoftGraphEvent.showAs
= MicrosoftGraphFreeBusyStatus.fromString(reader.getString());
} else if ("start".equals(fieldName)) {
deserializedMicrosoftGraphEvent.start = MicrosoftGraphDateTimeZone.fromJson(reader);
} else if ("subject".equals(fieldName)) {
deserializedMicrosoftGraphEvent.subject = reader.getString();
} else if ("transactionId".equals(fieldName)) {
deserializedMicrosoftGraphEvent.transactionId = reader.getString();
} else if ("type".equals(fieldName)) {
deserializedMicrosoftGraphEvent.type = MicrosoftGraphEventType.fromString(reader.getString());
} else if ("webLink".equals(fieldName)) {
deserializedMicrosoftGraphEvent.webLink = reader.getString();
} else if ("attachments".equals(fieldName)) {
List attachments
= reader.readArray(reader1 -> MicrosoftGraphAttachment.fromJson(reader1));
deserializedMicrosoftGraphEvent.attachments = attachments;
} else if ("calendar".equals(fieldName)) {
deserializedMicrosoftGraphEvent.calendar = MicrosoftGraphCalendar.fromJson(reader);
} else if ("extensions".equals(fieldName)) {
List extensions
= reader.readArray(reader1 -> MicrosoftGraphExtension.fromJson(reader1));
deserializedMicrosoftGraphEvent.extensions = extensions;
} else if ("instances".equals(fieldName)) {
List instances
= reader.readArray(reader1 -> MicrosoftGraphEvent.fromJson(reader1));
deserializedMicrosoftGraphEvent.instances = instances;
} else if ("multiValueExtendedProperties".equals(fieldName)) {
List multiValueExtendedProperties
= reader.readArray(reader1 -> MicrosoftGraphMultiValueLegacyExtendedProperty.fromJson(reader1));
deserializedMicrosoftGraphEvent.multiValueExtendedProperties = multiValueExtendedProperties;
} else if ("singleValueExtendedProperties".equals(fieldName)) {
List singleValueExtendedProperties = reader
.readArray(reader1 -> MicrosoftGraphSingleValueLegacyExtendedProperty.fromJson(reader1));
deserializedMicrosoftGraphEvent.singleValueExtendedProperties = singleValueExtendedProperties;
} else {
if (additionalProperties == null) {
additionalProperties = new LinkedHashMap<>();
}
additionalProperties.put(fieldName, reader.readUntyped());
}
}
deserializedMicrosoftGraphEvent.additionalProperties = additionalProperties;
return deserializedMicrosoftGraphEvent;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy