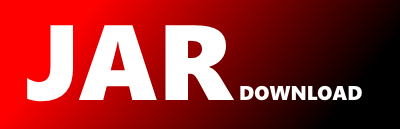
com.azure.resourcemanager.authorization.fluent.models.MicrosoftGraphManagedAppRegistration Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.authorization.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
/**
* managedAppRegistration
*
* The ManagedAppEntity is the base entity type for all other entity types under app management workflow.
*/
@Fluent
public final class MicrosoftGraphManagedAppRegistration extends MicrosoftGraphEntity {
/*
* The identifier for a mobile app.
*/
private Map appIdentifier;
/*
* App version
*/
private String applicationVersion;
/*
* Date and time of creation
*/
private OffsetDateTime createdDateTime;
/*
* Host device name
*/
private String deviceName;
/*
* App management SDK generated tag, which helps relate apps hosted on the same device. Not guaranteed to relate
* apps in all conditions.
*/
private String deviceTag;
/*
* Host device type
*/
private String deviceType;
/*
* Zero or more reasons an app registration is flagged. E.g. app running on rooted device
*/
private List flaggedReasons;
/*
* Date and time of last the app synced with management service.
*/
private OffsetDateTime lastSyncDateTime;
/*
* App management SDK version
*/
private String managementSdkVersion;
/*
* Operating System version
*/
private String platformVersion;
/*
* The user Id to who this app registration belongs.
*/
private String userId;
/*
* Version of the entity.
*/
private String version;
/*
* Zero or more policys already applied on the registered app when it last synchronized with managment service.
*/
private List appliedPolicies;
/*
* Zero or more policies admin intended for the app as of now.
*/
private List intendedPolicies;
/*
* Zero or more long running operations triggered on the app registration.
*/
private List operations;
/*
* The ManagedAppEntity is the base entity type for all other entity types under app management workflow.
*/
private Map additionalProperties;
/**
* Creates an instance of MicrosoftGraphManagedAppRegistration class.
*/
public MicrosoftGraphManagedAppRegistration() {
}
/**
* Get the appIdentifier property: The identifier for a mobile app.
*
* @return the appIdentifier value.
*/
public Map appIdentifier() {
return this.appIdentifier;
}
/**
* Set the appIdentifier property: The identifier for a mobile app.
*
* @param appIdentifier the appIdentifier value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration withAppIdentifier(Map appIdentifier) {
this.appIdentifier = appIdentifier;
return this;
}
/**
* Get the applicationVersion property: App version.
*
* @return the applicationVersion value.
*/
public String applicationVersion() {
return this.applicationVersion;
}
/**
* Set the applicationVersion property: App version.
*
* @param applicationVersion the applicationVersion value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration withApplicationVersion(String applicationVersion) {
this.applicationVersion = applicationVersion;
return this;
}
/**
* Get the createdDateTime property: Date and time of creation.
*
* @return the createdDateTime value.
*/
public OffsetDateTime createdDateTime() {
return this.createdDateTime;
}
/**
* Set the createdDateTime property: Date and time of creation.
*
* @param createdDateTime the createdDateTime value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration withCreatedDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
return this;
}
/**
* Get the deviceName property: Host device name.
*
* @return the deviceName value.
*/
public String deviceName() {
return this.deviceName;
}
/**
* Set the deviceName property: Host device name.
*
* @param deviceName the deviceName value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration withDeviceName(String deviceName) {
this.deviceName = deviceName;
return this;
}
/**
* Get the deviceTag property: App management SDK generated tag, which helps relate apps hosted on the same device.
* Not guaranteed to relate apps in all conditions.
*
* @return the deviceTag value.
*/
public String deviceTag() {
return this.deviceTag;
}
/**
* Set the deviceTag property: App management SDK generated tag, which helps relate apps hosted on the same device.
* Not guaranteed to relate apps in all conditions.
*
* @param deviceTag the deviceTag value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration withDeviceTag(String deviceTag) {
this.deviceTag = deviceTag;
return this;
}
/**
* Get the deviceType property: Host device type.
*
* @return the deviceType value.
*/
public String deviceType() {
return this.deviceType;
}
/**
* Set the deviceType property: Host device type.
*
* @param deviceType the deviceType value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration withDeviceType(String deviceType) {
this.deviceType = deviceType;
return this;
}
/**
* Get the flaggedReasons property: Zero or more reasons an app registration is flagged. E.g. app running on rooted
* device.
*
* @return the flaggedReasons value.
*/
public List flaggedReasons() {
return this.flaggedReasons;
}
/**
* Set the flaggedReasons property: Zero or more reasons an app registration is flagged. E.g. app running on rooted
* device.
*
* @param flaggedReasons the flaggedReasons value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration
withFlaggedReasons(List flaggedReasons) {
this.flaggedReasons = flaggedReasons;
return this;
}
/**
* Get the lastSyncDateTime property: Date and time of last the app synced with management service.
*
* @return the lastSyncDateTime value.
*/
public OffsetDateTime lastSyncDateTime() {
return this.lastSyncDateTime;
}
/**
* Set the lastSyncDateTime property: Date and time of last the app synced with management service.
*
* @param lastSyncDateTime the lastSyncDateTime value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration withLastSyncDateTime(OffsetDateTime lastSyncDateTime) {
this.lastSyncDateTime = lastSyncDateTime;
return this;
}
/**
* Get the managementSdkVersion property: App management SDK version.
*
* @return the managementSdkVersion value.
*/
public String managementSdkVersion() {
return this.managementSdkVersion;
}
/**
* Set the managementSdkVersion property: App management SDK version.
*
* @param managementSdkVersion the managementSdkVersion value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration withManagementSdkVersion(String managementSdkVersion) {
this.managementSdkVersion = managementSdkVersion;
return this;
}
/**
* Get the platformVersion property: Operating System version.
*
* @return the platformVersion value.
*/
public String platformVersion() {
return this.platformVersion;
}
/**
* Set the platformVersion property: Operating System version.
*
* @param platformVersion the platformVersion value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration withPlatformVersion(String platformVersion) {
this.platformVersion = platformVersion;
return this;
}
/**
* Get the userId property: The user Id to who this app registration belongs.
*
* @return the userId value.
*/
public String userId() {
return this.userId;
}
/**
* Set the userId property: The user Id to who this app registration belongs.
*
* @param userId the userId value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration withUserId(String userId) {
this.userId = userId;
return this;
}
/**
* Get the version property: Version of the entity.
*
* @return the version value.
*/
public String version() {
return this.version;
}
/**
* Set the version property: Version of the entity.
*
* @param version the version value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration withVersion(String version) {
this.version = version;
return this;
}
/**
* Get the appliedPolicies property: Zero or more policys already applied on the registered app when it last
* synchronized with managment service.
*
* @return the appliedPolicies value.
*/
public List appliedPolicies() {
return this.appliedPolicies;
}
/**
* Set the appliedPolicies property: Zero or more policys already applied on the registered app when it last
* synchronized with managment service.
*
* @param appliedPolicies the appliedPolicies value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration
withAppliedPolicies(List appliedPolicies) {
this.appliedPolicies = appliedPolicies;
return this;
}
/**
* Get the intendedPolicies property: Zero or more policies admin intended for the app as of now.
*
* @return the intendedPolicies value.
*/
public List intendedPolicies() {
return this.intendedPolicies;
}
/**
* Set the intendedPolicies property: Zero or more policies admin intended for the app as of now.
*
* @param intendedPolicies the intendedPolicies value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration
withIntendedPolicies(List intendedPolicies) {
this.intendedPolicies = intendedPolicies;
return this;
}
/**
* Get the operations property: Zero or more long running operations triggered on the app registration.
*
* @return the operations value.
*/
public List operations() {
return this.operations;
}
/**
* Set the operations property: Zero or more long running operations triggered on the app registration.
*
* @param operations the operations value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration withOperations(List operations) {
this.operations = operations;
return this;
}
/**
* Get the additionalProperties property: The ManagedAppEntity is the base entity type for all other entity types
* under app management workflow.
*
* @return the additionalProperties value.
*/
public Map additionalProperties() {
return this.additionalProperties;
}
/**
* Set the additionalProperties property: The ManagedAppEntity is the base entity type for all other entity types
* under app management workflow.
*
* @param additionalProperties the additionalProperties value to set.
* @return the MicrosoftGraphManagedAppRegistration object itself.
*/
public MicrosoftGraphManagedAppRegistration withAdditionalProperties(Map additionalProperties) {
this.additionalProperties = additionalProperties;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphManagedAppRegistration withId(String id) {
super.withId(id);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (appliedPolicies() != null) {
appliedPolicies().forEach(e -> e.validate());
}
if (intendedPolicies() != null) {
intendedPolicies().forEach(e -> e.validate());
}
if (operations() != null) {
operations().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("id", id());
jsonWriter.writeMapField("appIdentifier", this.appIdentifier,
(writer, element) -> writer.writeUntyped(element));
jsonWriter.writeStringField("applicationVersion", this.applicationVersion);
jsonWriter.writeStringField("createdDateTime",
this.createdDateTime == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.createdDateTime));
jsonWriter.writeStringField("deviceName", this.deviceName);
jsonWriter.writeStringField("deviceTag", this.deviceTag);
jsonWriter.writeStringField("deviceType", this.deviceType);
jsonWriter.writeArrayField("flaggedReasons", this.flaggedReasons,
(writer, element) -> writer.writeString(element == null ? null : element.toString()));
jsonWriter.writeStringField("lastSyncDateTime",
this.lastSyncDateTime == null
? null
: DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.lastSyncDateTime));
jsonWriter.writeStringField("managementSdkVersion", this.managementSdkVersion);
jsonWriter.writeStringField("platformVersion", this.platformVersion);
jsonWriter.writeStringField("userId", this.userId);
jsonWriter.writeStringField("version", this.version);
jsonWriter.writeArrayField("appliedPolicies", this.appliedPolicies,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("intendedPolicies", this.intendedPolicies,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("operations", this.operations, (writer, element) -> writer.writeJson(element));
if (additionalProperties != null) {
for (Map.Entry additionalProperty : additionalProperties.entrySet()) {
jsonWriter.writeUntypedField(additionalProperty.getKey(), additionalProperty.getValue());
}
}
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MicrosoftGraphManagedAppRegistration from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MicrosoftGraphManagedAppRegistration if the JsonReader was pointing to an instance of it,
* or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the MicrosoftGraphManagedAppRegistration.
*/
public static MicrosoftGraphManagedAppRegistration fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
MicrosoftGraphManagedAppRegistration deserializedMicrosoftGraphManagedAppRegistration
= new MicrosoftGraphManagedAppRegistration();
Map additionalProperties = null;
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedMicrosoftGraphManagedAppRegistration.withId(reader.getString());
} else if ("appIdentifier".equals(fieldName)) {
Map appIdentifier = reader.readMap(reader1 -> reader1.readUntyped());
deserializedMicrosoftGraphManagedAppRegistration.appIdentifier = appIdentifier;
} else if ("applicationVersion".equals(fieldName)) {
deserializedMicrosoftGraphManagedAppRegistration.applicationVersion = reader.getString();
} else if ("createdDateTime".equals(fieldName)) {
deserializedMicrosoftGraphManagedAppRegistration.createdDateTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("deviceName".equals(fieldName)) {
deserializedMicrosoftGraphManagedAppRegistration.deviceName = reader.getString();
} else if ("deviceTag".equals(fieldName)) {
deserializedMicrosoftGraphManagedAppRegistration.deviceTag = reader.getString();
} else if ("deviceType".equals(fieldName)) {
deserializedMicrosoftGraphManagedAppRegistration.deviceType = reader.getString();
} else if ("flaggedReasons".equals(fieldName)) {
List flaggedReasons = reader
.readArray(reader1 -> MicrosoftGraphManagedAppFlaggedReason.fromString(reader1.getString()));
deserializedMicrosoftGraphManagedAppRegistration.flaggedReasons = flaggedReasons;
} else if ("lastSyncDateTime".equals(fieldName)) {
deserializedMicrosoftGraphManagedAppRegistration.lastSyncDateTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("managementSdkVersion".equals(fieldName)) {
deserializedMicrosoftGraphManagedAppRegistration.managementSdkVersion = reader.getString();
} else if ("platformVersion".equals(fieldName)) {
deserializedMicrosoftGraphManagedAppRegistration.platformVersion = reader.getString();
} else if ("userId".equals(fieldName)) {
deserializedMicrosoftGraphManagedAppRegistration.userId = reader.getString();
} else if ("version".equals(fieldName)) {
deserializedMicrosoftGraphManagedAppRegistration.version = reader.getString();
} else if ("appliedPolicies".equals(fieldName)) {
List appliedPolicies
= reader.readArray(reader1 -> MicrosoftGraphManagedAppPolicy.fromJson(reader1));
deserializedMicrosoftGraphManagedAppRegistration.appliedPolicies = appliedPolicies;
} else if ("intendedPolicies".equals(fieldName)) {
List intendedPolicies
= reader.readArray(reader1 -> MicrosoftGraphManagedAppPolicy.fromJson(reader1));
deserializedMicrosoftGraphManagedAppRegistration.intendedPolicies = intendedPolicies;
} else if ("operations".equals(fieldName)) {
List operations
= reader.readArray(reader1 -> MicrosoftGraphManagedAppOperation.fromJson(reader1));
deserializedMicrosoftGraphManagedAppRegistration.operations = operations;
} else {
if (additionalProperties == null) {
additionalProperties = new LinkedHashMap<>();
}
additionalProperties.put(fieldName, reader.readUntyped());
}
}
deserializedMicrosoftGraphManagedAppRegistration.additionalProperties = additionalProperties;
return deserializedMicrosoftGraphManagedAppRegistration;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy