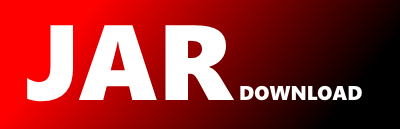
com.azure.resourcemanager.authorization.fluent.models.MicrosoftGraphServicePrincipalInner Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.authorization.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.UUID;
/**
* servicePrincipal
*
* Represents an Azure Active Directory object. The directoryObject type is the base type for many other directory
* entity types.
*/
@Fluent
public final class MicrosoftGraphServicePrincipalInner extends MicrosoftGraphDirectoryObjectInner {
/*
* true if the service principal account is enabled; otherwise, false.
*/
private Boolean accountEnabled;
/*
* Defines custom behavior that a consuming service can use to call an app in specific contexts. For example,
* applications that can render file streams may set the addIns property for its 'FileHandler' functionality. This
* will let services like Microsoft 365 call the application in the context of a document the user is working on.
*/
private List addIns;
/*
* Used to retrieve service principals by subscription, identify resource group and full resource ids for managed
* identities.
*/
private List alternativeNames;
/*
* The appDescription property.
*/
private String appDescription;
/*
* The display name exposed by the associated application.
*/
private String appDisplayName;
/*
* The unique identifier for the associated application (its appId property).
*/
private String appId;
/*
* Unique identifier of the applicationTemplate that the servicePrincipal was created from. Read-only.
*/
private String applicationTemplateId;
/*
* Contains the tenant id where the application is registered. This is applicable only to service principals backed
* by applications.
*/
private UUID appOwnerOrganizationId;
/*
* Specifies whether users or other service principals need to be granted an app role assignment for this service
* principal before users can sign in or apps can get tokens. The default value is false. Not nullable.
*/
private Boolean appRoleAssignmentRequired;
/*
* The roles exposed by the application which this service principal represents. For more information see the
* appRoles property definition on the application entity. Not nullable.
*/
private List appRoles;
/*
* The description property.
*/
private String description;
/*
* The display name for the service principal.
*/
private String displayName;
/*
* Home page or landing page of the application.
*/
private String homepage;
/*
* informationalUrl
*/
private MicrosoftGraphInformationalUrl info;
/*
* The collection of key credentials associated with the service principal. Not nullable.
*/
private List keyCredentials;
/*
* Specifies the URL where the service provider redirects the user to Azure AD to authenticate. Azure AD uses the
* URL to launch the application from Microsoft 365 or the Azure AD My Apps. When blank, Azure AD performs
* IdP-initiated sign-on for applications configured with SAML-based single sign-on. The user launches the
* application from Microsoft 365, the Azure AD My Apps, or the Azure AD SSO URL.
*/
private String loginUrl;
/*
* Specifies the URL that will be used by Microsoft's authorization service to logout an user using OpenId Connect
* front-channel, back-channel or SAML logout protocols.
*/
private String logoutUrl;
/*
* The notes property.
*/
private String notes;
/*
* Specifies the list of email addresses where Azure AD sends a notification when the active certificate is near the
* expiration date. This is only for the certificates used to sign the SAML token issued for Azure AD Gallery
* applications.
*/
private List notificationEmailAddresses;
/*
* The delegated permissions exposed by the application. For more information see the oauth2PermissionScopes
* property on the application entity's api property. Not nullable.
*/
private List oauth2PermissionScopes;
/*
* The collection of password credentials associated with the service principal. Not nullable.
*/
private List passwordCredentials;
/*
* Specifies the single sign-on mode configured for this application. Azure AD uses the preferred single sign-on
* mode to launch the application from Microsoft 365 or the Azure AD My Apps. The supported values are password,
* saml, external, and oidc.
*/
private String preferredSingleSignOnMode;
/*
* The preferredTokenSigningKeyThumbprint property.
*/
private String preferredTokenSigningKeyThumbprint;
/*
* The URLs that user tokens are sent to for sign in with the associated application, or the redirect URIs that
* OAuth 2.0 authorization codes and access tokens are sent to for the associated application. Not nullable.
*/
private List replyUrls;
/*
* samlSingleSignOnSettings
*/
private MicrosoftGraphSamlSingleSignOnSettings samlSingleSignOnSettings;
/*
* Contains the list of identifiersUris, copied over from the associated application. Additional values can be added
* to hybrid applications. These values can be used to identify the permissions exposed by this app within Azure AD.
* For example,Client apps can specify a resource URI which is based on the values of this property to acquire an
* access token, which is the URI returned in the 'aud' claim.The any operator is required for filter expressions on
* multi-valued properties. Not nullable.
*/
private List servicePrincipalNames;
/*
* Identifies if the service principal represents an application or a managed identity. This is set by Azure AD
* internally. For a service principal that represents an application this is set as Application. For a service
* principal that represent a managed identity this is set as ManagedIdentity.
*/
private String servicePrincipalType;
/*
* The signInAudience property.
*/
private String signInAudience;
/*
* Custom strings that can be used to categorize and identify the service principal. Not nullable.
*/
private List tags;
/*
* Specifies the keyId of a public key from the keyCredentials collection. When configured, Azure AD issues tokens
* for this application encrypted using the key specified by this property. The application code that receives the
* encrypted token must use the matching private key to decrypt the token before it can be used for the signed-in
* user.
*/
private UUID tokenEncryptionKeyId;
/*
* Principals (users, groups, and service principals) that are assigned to this service principal. Read-only.
*/
private List appRoleAssignedTo;
/*
* Applications that this service principal is assigned to. Read-only. Nullable.
*/
private List appRoleAssignments;
/*
* The claimsMappingPolicies assigned to this service principal.
*/
private List claimsMappingPolicies;
/*
* Directory objects created by this service principal. Read-only. Nullable.
*/
private List createdObjects;
/*
* The delegatedPermissionClassifications property.
*/
private List delegatedPermissionClassifications;
/*
* Endpoints available for discovery. Services like Sharepoint populate this property with a tenant specific
* SharePoint endpoints that other applications can discover and use in their experiences.
*/
private List endpoints;
/*
* The homeRealmDiscoveryPolicies assigned to this service principal.
*/
private List homeRealmDiscoveryPolicies;
/*
* Roles that this service principal is a member of. HTTP Methods: GET Read-only. Nullable.
*/
private List memberOf;
/*
* Delegated permission grants authorizing this service principal to access an API on behalf of a signed-in user.
* Read-only. Nullable.
*/
private List oauth2PermissionGrants;
/*
* Directory objects that are owned by this service principal. Read-only. Nullable.
*/
private List ownedObjects;
/*
* Directory objects that are owners of this servicePrincipal. The owners are a set of non-admin users or
* servicePrincipals who are allowed to modify this object. Read-only. Nullable.
*/
private List owners;
/*
* The tokenIssuancePolicies assigned to this service principal.
*/
private List tokenIssuancePolicies;
/*
* The tokenLifetimePolicies assigned to this service principal.
*/
private List tokenLifetimePolicies;
/*
* The transitiveMemberOf property.
*/
private List transitiveMemberOf;
/*
* Represents an Azure Active Directory object. The directoryObject type is the base type for many other directory
* entity types.
*/
private Map additionalProperties;
/**
* Creates an instance of MicrosoftGraphServicePrincipalInner class.
*/
public MicrosoftGraphServicePrincipalInner() {
}
/**
* Get the accountEnabled property: true if the service principal account is enabled; otherwise, false.
*
* @return the accountEnabled value.
*/
public Boolean accountEnabled() {
return this.accountEnabled;
}
/**
* Set the accountEnabled property: true if the service principal account is enabled; otherwise, false.
*
* @param accountEnabled the accountEnabled value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withAccountEnabled(Boolean accountEnabled) {
this.accountEnabled = accountEnabled;
return this;
}
/**
* Get the addIns property: Defines custom behavior that a consuming service can use to call an app in specific
* contexts. For example, applications that can render file streams may set the addIns property for its
* 'FileHandler' functionality. This will let services like Microsoft 365 call the application in the context of a
* document the user is working on.
*
* @return the addIns value.
*/
public List addIns() {
return this.addIns;
}
/**
* Set the addIns property: Defines custom behavior that a consuming service can use to call an app in specific
* contexts. For example, applications that can render file streams may set the addIns property for its
* 'FileHandler' functionality. This will let services like Microsoft 365 call the application in the context of a
* document the user is working on.
*
* @param addIns the addIns value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withAddIns(List addIns) {
this.addIns = addIns;
return this;
}
/**
* Get the alternativeNames property: Used to retrieve service principals by subscription, identify resource group
* and full resource ids for managed identities.
*
* @return the alternativeNames value.
*/
public List alternativeNames() {
return this.alternativeNames;
}
/**
* Set the alternativeNames property: Used to retrieve service principals by subscription, identify resource group
* and full resource ids for managed identities.
*
* @param alternativeNames the alternativeNames value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withAlternativeNames(List alternativeNames) {
this.alternativeNames = alternativeNames;
return this;
}
/**
* Get the appDescription property: The appDescription property.
*
* @return the appDescription value.
*/
public String appDescription() {
return this.appDescription;
}
/**
* Set the appDescription property: The appDescription property.
*
* @param appDescription the appDescription value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withAppDescription(String appDescription) {
this.appDescription = appDescription;
return this;
}
/**
* Get the appDisplayName property: The display name exposed by the associated application.
*
* @return the appDisplayName value.
*/
public String appDisplayName() {
return this.appDisplayName;
}
/**
* Set the appDisplayName property: The display name exposed by the associated application.
*
* @param appDisplayName the appDisplayName value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withAppDisplayName(String appDisplayName) {
this.appDisplayName = appDisplayName;
return this;
}
/**
* Get the appId property: The unique identifier for the associated application (its appId property).
*
* @return the appId value.
*/
public String appId() {
return this.appId;
}
/**
* Set the appId property: The unique identifier for the associated application (its appId property).
*
* @param appId the appId value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withAppId(String appId) {
this.appId = appId;
return this;
}
/**
* Get the applicationTemplateId property: Unique identifier of the applicationTemplate that the servicePrincipal
* was created from. Read-only.
*
* @return the applicationTemplateId value.
*/
public String applicationTemplateId() {
return this.applicationTemplateId;
}
/**
* Set the applicationTemplateId property: Unique identifier of the applicationTemplate that the servicePrincipal
* was created from. Read-only.
*
* @param applicationTemplateId the applicationTemplateId value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withApplicationTemplateId(String applicationTemplateId) {
this.applicationTemplateId = applicationTemplateId;
return this;
}
/**
* Get the appOwnerOrganizationId property: Contains the tenant id where the application is registered. This is
* applicable only to service principals backed by applications.
*
* @return the appOwnerOrganizationId value.
*/
public UUID appOwnerOrganizationId() {
return this.appOwnerOrganizationId;
}
/**
* Set the appOwnerOrganizationId property: Contains the tenant id where the application is registered. This is
* applicable only to service principals backed by applications.
*
* @param appOwnerOrganizationId the appOwnerOrganizationId value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withAppOwnerOrganizationId(UUID appOwnerOrganizationId) {
this.appOwnerOrganizationId = appOwnerOrganizationId;
return this;
}
/**
* Get the appRoleAssignmentRequired property: Specifies whether users or other service principals need to be
* granted an app role assignment for this service principal before users can sign in or apps can get tokens. The
* default value is false. Not nullable.
*
* @return the appRoleAssignmentRequired value.
*/
public Boolean appRoleAssignmentRequired() {
return this.appRoleAssignmentRequired;
}
/**
* Set the appRoleAssignmentRequired property: Specifies whether users or other service principals need to be
* granted an app role assignment for this service principal before users can sign in or apps can get tokens. The
* default value is false. Not nullable.
*
* @param appRoleAssignmentRequired the appRoleAssignmentRequired value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withAppRoleAssignmentRequired(Boolean appRoleAssignmentRequired) {
this.appRoleAssignmentRequired = appRoleAssignmentRequired;
return this;
}
/**
* Get the appRoles property: The roles exposed by the application which this service principal represents. For more
* information see the appRoles property definition on the application entity. Not nullable.
*
* @return the appRoles value.
*/
public List appRoles() {
return this.appRoles;
}
/**
* Set the appRoles property: The roles exposed by the application which this service principal represents. For more
* information see the appRoles property definition on the application entity. Not nullable.
*
* @param appRoles the appRoles value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withAppRoles(List appRoles) {
this.appRoles = appRoles;
return this;
}
/**
* Get the description property: The description property.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Set the description property: The description property.
*
* @param description the description value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withDescription(String description) {
this.description = description;
return this;
}
/**
* Get the displayName property: The display name for the service principal.
*
* @return the displayName value.
*/
public String displayName() {
return this.displayName;
}
/**
* Set the displayName property: The display name for the service principal.
*
* @param displayName the displayName value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withDisplayName(String displayName) {
this.displayName = displayName;
return this;
}
/**
* Get the homepage property: Home page or landing page of the application.
*
* @return the homepage value.
*/
public String homepage() {
return this.homepage;
}
/**
* Set the homepage property: Home page or landing page of the application.
*
* @param homepage the homepage value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withHomepage(String homepage) {
this.homepage = homepage;
return this;
}
/**
* Get the info property: informationalUrl.
*
* @return the info value.
*/
public MicrosoftGraphInformationalUrl info() {
return this.info;
}
/**
* Set the info property: informationalUrl.
*
* @param info the info value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withInfo(MicrosoftGraphInformationalUrl info) {
this.info = info;
return this;
}
/**
* Get the keyCredentials property: The collection of key credentials associated with the service principal. Not
* nullable.
*
* @return the keyCredentials value.
*/
public List keyCredentials() {
return this.keyCredentials;
}
/**
* Set the keyCredentials property: The collection of key credentials associated with the service principal. Not
* nullable.
*
* @param keyCredentials the keyCredentials value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner
withKeyCredentials(List keyCredentials) {
this.keyCredentials = keyCredentials;
return this;
}
/**
* Get the loginUrl property: Specifies the URL where the service provider redirects the user to Azure AD to
* authenticate. Azure AD uses the URL to launch the application from Microsoft 365 or the Azure AD My Apps. When
* blank, Azure AD performs IdP-initiated sign-on for applications configured with SAML-based single sign-on. The
* user launches the application from Microsoft 365, the Azure AD My Apps, or the Azure AD SSO URL.
*
* @return the loginUrl value.
*/
public String loginUrl() {
return this.loginUrl;
}
/**
* Set the loginUrl property: Specifies the URL where the service provider redirects the user to Azure AD to
* authenticate. Azure AD uses the URL to launch the application from Microsoft 365 or the Azure AD My Apps. When
* blank, Azure AD performs IdP-initiated sign-on for applications configured with SAML-based single sign-on. The
* user launches the application from Microsoft 365, the Azure AD My Apps, or the Azure AD SSO URL.
*
* @param loginUrl the loginUrl value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withLoginUrl(String loginUrl) {
this.loginUrl = loginUrl;
return this;
}
/**
* Get the logoutUrl property: Specifies the URL that will be used by Microsoft's authorization service to logout an
* user using OpenId Connect front-channel, back-channel or SAML logout protocols.
*
* @return the logoutUrl value.
*/
public String logoutUrl() {
return this.logoutUrl;
}
/**
* Set the logoutUrl property: Specifies the URL that will be used by Microsoft's authorization service to logout an
* user using OpenId Connect front-channel, back-channel or SAML logout protocols.
*
* @param logoutUrl the logoutUrl value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withLogoutUrl(String logoutUrl) {
this.logoutUrl = logoutUrl;
return this;
}
/**
* Get the notes property: The notes property.
*
* @return the notes value.
*/
public String notes() {
return this.notes;
}
/**
* Set the notes property: The notes property.
*
* @param notes the notes value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withNotes(String notes) {
this.notes = notes;
return this;
}
/**
* Get the notificationEmailAddresses property: Specifies the list of email addresses where Azure AD sends a
* notification when the active certificate is near the expiration date. This is only for the certificates used to
* sign the SAML token issued for Azure AD Gallery applications.
*
* @return the notificationEmailAddresses value.
*/
public List notificationEmailAddresses() {
return this.notificationEmailAddresses;
}
/**
* Set the notificationEmailAddresses property: Specifies the list of email addresses where Azure AD sends a
* notification when the active certificate is near the expiration date. This is only for the certificates used to
* sign the SAML token issued for Azure AD Gallery applications.
*
* @param notificationEmailAddresses the notificationEmailAddresses value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withNotificationEmailAddresses(List notificationEmailAddresses) {
this.notificationEmailAddresses = notificationEmailAddresses;
return this;
}
/**
* Get the oauth2PermissionScopes property: The delegated permissions exposed by the application. For more
* information see the oauth2PermissionScopes property on the application entity's api property. Not nullable.
*
* @return the oauth2PermissionScopes value.
*/
public List oauth2PermissionScopes() {
return this.oauth2PermissionScopes;
}
/**
* Set the oauth2PermissionScopes property: The delegated permissions exposed by the application. For more
* information see the oauth2PermissionScopes property on the application entity's api property. Not nullable.
*
* @param oauth2PermissionScopes the oauth2PermissionScopes value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner
withOauth2PermissionScopes(List oauth2PermissionScopes) {
this.oauth2PermissionScopes = oauth2PermissionScopes;
return this;
}
/**
* Get the passwordCredentials property: The collection of password credentials associated with the service
* principal. Not nullable.
*
* @return the passwordCredentials value.
*/
public List passwordCredentials() {
return this.passwordCredentials;
}
/**
* Set the passwordCredentials property: The collection of password credentials associated with the service
* principal. Not nullable.
*
* @param passwordCredentials the passwordCredentials value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner
withPasswordCredentials(List passwordCredentials) {
this.passwordCredentials = passwordCredentials;
return this;
}
/**
* Get the preferredSingleSignOnMode property: Specifies the single sign-on mode configured for this application.
* Azure AD uses the preferred single sign-on mode to launch the application from Microsoft 365 or the Azure AD My
* Apps. The supported values are password, saml, external, and oidc.
*
* @return the preferredSingleSignOnMode value.
*/
public String preferredSingleSignOnMode() {
return this.preferredSingleSignOnMode;
}
/**
* Set the preferredSingleSignOnMode property: Specifies the single sign-on mode configured for this application.
* Azure AD uses the preferred single sign-on mode to launch the application from Microsoft 365 or the Azure AD My
* Apps. The supported values are password, saml, external, and oidc.
*
* @param preferredSingleSignOnMode the preferredSingleSignOnMode value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withPreferredSingleSignOnMode(String preferredSingleSignOnMode) {
this.preferredSingleSignOnMode = preferredSingleSignOnMode;
return this;
}
/**
* Get the preferredTokenSigningKeyThumbprint property: The preferredTokenSigningKeyThumbprint property.
*
* @return the preferredTokenSigningKeyThumbprint value.
*/
public String preferredTokenSigningKeyThumbprint() {
return this.preferredTokenSigningKeyThumbprint;
}
/**
* Set the preferredTokenSigningKeyThumbprint property: The preferredTokenSigningKeyThumbprint property.
*
* @param preferredTokenSigningKeyThumbprint the preferredTokenSigningKeyThumbprint value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner
withPreferredTokenSigningKeyThumbprint(String preferredTokenSigningKeyThumbprint) {
this.preferredTokenSigningKeyThumbprint = preferredTokenSigningKeyThumbprint;
return this;
}
/**
* Get the replyUrls property: The URLs that user tokens are sent to for sign in with the associated application, or
* the redirect URIs that OAuth 2.0 authorization codes and access tokens are sent to for the associated
* application. Not nullable.
*
* @return the replyUrls value.
*/
public List replyUrls() {
return this.replyUrls;
}
/**
* Set the replyUrls property: The URLs that user tokens are sent to for sign in with the associated application, or
* the redirect URIs that OAuth 2.0 authorization codes and access tokens are sent to for the associated
* application. Not nullable.
*
* @param replyUrls the replyUrls value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withReplyUrls(List replyUrls) {
this.replyUrls = replyUrls;
return this;
}
/**
* Get the samlSingleSignOnSettings property: samlSingleSignOnSettings.
*
* @return the samlSingleSignOnSettings value.
*/
public MicrosoftGraphSamlSingleSignOnSettings samlSingleSignOnSettings() {
return this.samlSingleSignOnSettings;
}
/**
* Set the samlSingleSignOnSettings property: samlSingleSignOnSettings.
*
* @param samlSingleSignOnSettings the samlSingleSignOnSettings value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner
withSamlSingleSignOnSettings(MicrosoftGraphSamlSingleSignOnSettings samlSingleSignOnSettings) {
this.samlSingleSignOnSettings = samlSingleSignOnSettings;
return this;
}
/**
* Get the servicePrincipalNames property: Contains the list of identifiersUris, copied over from the associated
* application. Additional values can be added to hybrid applications. These values can be used to identify the
* permissions exposed by this app within Azure AD. For example,Client apps can specify a resource URI which is
* based on the values of this property to acquire an access token, which is the URI returned in the 'aud' claim.The
* any operator is required for filter expressions on multi-valued properties. Not nullable.
*
* @return the servicePrincipalNames value.
*/
public List servicePrincipalNames() {
return this.servicePrincipalNames;
}
/**
* Set the servicePrincipalNames property: Contains the list of identifiersUris, copied over from the associated
* application. Additional values can be added to hybrid applications. These values can be used to identify the
* permissions exposed by this app within Azure AD. For example,Client apps can specify a resource URI which is
* based on the values of this property to acquire an access token, which is the URI returned in the 'aud' claim.The
* any operator is required for filter expressions on multi-valued properties. Not nullable.
*
* @param servicePrincipalNames the servicePrincipalNames value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withServicePrincipalNames(List servicePrincipalNames) {
this.servicePrincipalNames = servicePrincipalNames;
return this;
}
/**
* Get the servicePrincipalType property: Identifies if the service principal represents an application or a managed
* identity. This is set by Azure AD internally. For a service principal that represents an application this is set
* as Application. For a service principal that represent a managed identity this is set as ManagedIdentity.
*
* @return the servicePrincipalType value.
*/
public String servicePrincipalType() {
return this.servicePrincipalType;
}
/**
* Set the servicePrincipalType property: Identifies if the service principal represents an application or a managed
* identity. This is set by Azure AD internally. For a service principal that represents an application this is set
* as Application. For a service principal that represent a managed identity this is set as ManagedIdentity.
*
* @param servicePrincipalType the servicePrincipalType value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withServicePrincipalType(String servicePrincipalType) {
this.servicePrincipalType = servicePrincipalType;
return this;
}
/**
* Get the signInAudience property: The signInAudience property.
*
* @return the signInAudience value.
*/
public String signInAudience() {
return this.signInAudience;
}
/**
* Set the signInAudience property: The signInAudience property.
*
* @param signInAudience the signInAudience value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withSignInAudience(String signInAudience) {
this.signInAudience = signInAudience;
return this;
}
/**
* Get the tags property: Custom strings that can be used to categorize and identify the service principal. Not
* nullable.
*
* @return the tags value.
*/
public List tags() {
return this.tags;
}
/**
* Set the tags property: Custom strings that can be used to categorize and identify the service principal. Not
* nullable.
*
* @param tags the tags value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withTags(List tags) {
this.tags = tags;
return this;
}
/**
* Get the tokenEncryptionKeyId property: Specifies the keyId of a public key from the keyCredentials collection.
* When configured, Azure AD issues tokens for this application encrypted using the key specified by this property.
* The application code that receives the encrypted token must use the matching private key to decrypt the token
* before it can be used for the signed-in user.
*
* @return the tokenEncryptionKeyId value.
*/
public UUID tokenEncryptionKeyId() {
return this.tokenEncryptionKeyId;
}
/**
* Set the tokenEncryptionKeyId property: Specifies the keyId of a public key from the keyCredentials collection.
* When configured, Azure AD issues tokens for this application encrypted using the key specified by this property.
* The application code that receives the encrypted token must use the matching private key to decrypt the token
* before it can be used for the signed-in user.
*
* @param tokenEncryptionKeyId the tokenEncryptionKeyId value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withTokenEncryptionKeyId(UUID tokenEncryptionKeyId) {
this.tokenEncryptionKeyId = tokenEncryptionKeyId;
return this;
}
/**
* Get the appRoleAssignedTo property: Principals (users, groups, and service principals) that are assigned to this
* service principal. Read-only.
*
* @return the appRoleAssignedTo value.
*/
public List appRoleAssignedTo() {
return this.appRoleAssignedTo;
}
/**
* Set the appRoleAssignedTo property: Principals (users, groups, and service principals) that are assigned to this
* service principal. Read-only.
*
* @param appRoleAssignedTo the appRoleAssignedTo value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner
withAppRoleAssignedTo(List appRoleAssignedTo) {
this.appRoleAssignedTo = appRoleAssignedTo;
return this;
}
/**
* Get the appRoleAssignments property: Applications that this service principal is assigned to. Read-only.
* Nullable.
*
* @return the appRoleAssignments value.
*/
public List appRoleAssignments() {
return this.appRoleAssignments;
}
/**
* Set the appRoleAssignments property: Applications that this service principal is assigned to. Read-only.
* Nullable.
*
* @param appRoleAssignments the appRoleAssignments value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner
withAppRoleAssignments(List appRoleAssignments) {
this.appRoleAssignments = appRoleAssignments;
return this;
}
/**
* Get the claimsMappingPolicies property: The claimsMappingPolicies assigned to this service principal.
*
* @return the claimsMappingPolicies value.
*/
public List claimsMappingPolicies() {
return this.claimsMappingPolicies;
}
/**
* Set the claimsMappingPolicies property: The claimsMappingPolicies assigned to this service principal.
*
* @param claimsMappingPolicies the claimsMappingPolicies value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner
withClaimsMappingPolicies(List claimsMappingPolicies) {
this.claimsMappingPolicies = claimsMappingPolicies;
return this;
}
/**
* Get the createdObjects property: Directory objects created by this service principal. Read-only. Nullable.
*
* @return the createdObjects value.
*/
public List createdObjects() {
return this.createdObjects;
}
/**
* Set the createdObjects property: Directory objects created by this service principal. Read-only. Nullable.
*
* @param createdObjects the createdObjects value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner
withCreatedObjects(List createdObjects) {
this.createdObjects = createdObjects;
return this;
}
/**
* Get the delegatedPermissionClassifications property: The delegatedPermissionClassifications property.
*
* @return the delegatedPermissionClassifications value.
*/
public List delegatedPermissionClassifications() {
return this.delegatedPermissionClassifications;
}
/**
* Set the delegatedPermissionClassifications property: The delegatedPermissionClassifications property.
*
* @param delegatedPermissionClassifications the delegatedPermissionClassifications value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withDelegatedPermissionClassifications(
List delegatedPermissionClassifications) {
this.delegatedPermissionClassifications = delegatedPermissionClassifications;
return this;
}
/**
* Get the endpoints property: Endpoints available for discovery. Services like Sharepoint populate this property
* with a tenant specific SharePoint endpoints that other applications can discover and use in their experiences.
*
* @return the endpoints value.
*/
public List endpoints() {
return this.endpoints;
}
/**
* Set the endpoints property: Endpoints available for discovery. Services like Sharepoint populate this property
* with a tenant specific SharePoint endpoints that other applications can discover and use in their experiences.
*
* @param endpoints the endpoints value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withEndpoints(List endpoints) {
this.endpoints = endpoints;
return this;
}
/**
* Get the homeRealmDiscoveryPolicies property: The homeRealmDiscoveryPolicies assigned to this service principal.
*
* @return the homeRealmDiscoveryPolicies value.
*/
public List homeRealmDiscoveryPolicies() {
return this.homeRealmDiscoveryPolicies;
}
/**
* Set the homeRealmDiscoveryPolicies property: The homeRealmDiscoveryPolicies assigned to this service principal.
*
* @param homeRealmDiscoveryPolicies the homeRealmDiscoveryPolicies value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner
withHomeRealmDiscoveryPolicies(List homeRealmDiscoveryPolicies) {
this.homeRealmDiscoveryPolicies = homeRealmDiscoveryPolicies;
return this;
}
/**
* Get the memberOf property: Roles that this service principal is a member of. HTTP Methods: GET Read-only.
* Nullable.
*
* @return the memberOf value.
*/
public List memberOf() {
return this.memberOf;
}
/**
* Set the memberOf property: Roles that this service principal is a member of. HTTP Methods: GET Read-only.
* Nullable.
*
* @param memberOf the memberOf value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withMemberOf(List memberOf) {
this.memberOf = memberOf;
return this;
}
/**
* Get the oauth2PermissionGrants property: Delegated permission grants authorizing this service principal to access
* an API on behalf of a signed-in user. Read-only. Nullable.
*
* @return the oauth2PermissionGrants value.
*/
public List oauth2PermissionGrants() {
return this.oauth2PermissionGrants;
}
/**
* Set the oauth2PermissionGrants property: Delegated permission grants authorizing this service principal to access
* an API on behalf of a signed-in user. Read-only. Nullable.
*
* @param oauth2PermissionGrants the oauth2PermissionGrants value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner
withOauth2PermissionGrants(List oauth2PermissionGrants) {
this.oauth2PermissionGrants = oauth2PermissionGrants;
return this;
}
/**
* Get the ownedObjects property: Directory objects that are owned by this service principal. Read-only. Nullable.
*
* @return the ownedObjects value.
*/
public List ownedObjects() {
return this.ownedObjects;
}
/**
* Set the ownedObjects property: Directory objects that are owned by this service principal. Read-only. Nullable.
*
* @param ownedObjects the ownedObjects value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withOwnedObjects(List ownedObjects) {
this.ownedObjects = ownedObjects;
return this;
}
/**
* Get the owners property: Directory objects that are owners of this servicePrincipal. The owners are a set of
* non-admin users or servicePrincipals who are allowed to modify this object. Read-only. Nullable.
*
* @return the owners value.
*/
public List owners() {
return this.owners;
}
/**
* Set the owners property: Directory objects that are owners of this servicePrincipal. The owners are a set of
* non-admin users or servicePrincipals who are allowed to modify this object. Read-only. Nullable.
*
* @param owners the owners value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withOwners(List owners) {
this.owners = owners;
return this;
}
/**
* Get the tokenIssuancePolicies property: The tokenIssuancePolicies assigned to this service principal.
*
* @return the tokenIssuancePolicies value.
*/
public List tokenIssuancePolicies() {
return this.tokenIssuancePolicies;
}
/**
* Set the tokenIssuancePolicies property: The tokenIssuancePolicies assigned to this service principal.
*
* @param tokenIssuancePolicies the tokenIssuancePolicies value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner
withTokenIssuancePolicies(List tokenIssuancePolicies) {
this.tokenIssuancePolicies = tokenIssuancePolicies;
return this;
}
/**
* Get the tokenLifetimePolicies property: The tokenLifetimePolicies assigned to this service principal.
*
* @return the tokenLifetimePolicies value.
*/
public List tokenLifetimePolicies() {
return this.tokenLifetimePolicies;
}
/**
* Set the tokenLifetimePolicies property: The tokenLifetimePolicies assigned to this service principal.
*
* @param tokenLifetimePolicies the tokenLifetimePolicies value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner
withTokenLifetimePolicies(List tokenLifetimePolicies) {
this.tokenLifetimePolicies = tokenLifetimePolicies;
return this;
}
/**
* Get the transitiveMemberOf property: The transitiveMemberOf property.
*
* @return the transitiveMemberOf value.
*/
public List transitiveMemberOf() {
return this.transitiveMemberOf;
}
/**
* Set the transitiveMemberOf property: The transitiveMemberOf property.
*
* @param transitiveMemberOf the transitiveMemberOf value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner
withTransitiveMemberOf(List transitiveMemberOf) {
this.transitiveMemberOf = transitiveMemberOf;
return this;
}
/**
* Get the additionalProperties property: Represents an Azure Active Directory object. The directoryObject type is
* the base type for many other directory entity types.
*
* @return the additionalProperties value.
*/
public Map additionalProperties() {
return this.additionalProperties;
}
/**
* Set the additionalProperties property: Represents an Azure Active Directory object. The directoryObject type is
* the base type for many other directory entity types.
*
* @param additionalProperties the additionalProperties value to set.
* @return the MicrosoftGraphServicePrincipalInner object itself.
*/
public MicrosoftGraphServicePrincipalInner withAdditionalProperties(Map additionalProperties) {
this.additionalProperties = additionalProperties;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphServicePrincipalInner withDeletedDateTime(OffsetDateTime deletedDateTime) {
super.withDeletedDateTime(deletedDateTime);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphServicePrincipalInner withId(String id) {
super.withId(id);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (addIns() != null) {
addIns().forEach(e -> e.validate());
}
if (appRoles() != null) {
appRoles().forEach(e -> e.validate());
}
if (info() != null) {
info().validate();
}
if (keyCredentials() != null) {
keyCredentials().forEach(e -> e.validate());
}
if (oauth2PermissionScopes() != null) {
oauth2PermissionScopes().forEach(e -> e.validate());
}
if (passwordCredentials() != null) {
passwordCredentials().forEach(e -> e.validate());
}
if (samlSingleSignOnSettings() != null) {
samlSingleSignOnSettings().validate();
}
if (appRoleAssignedTo() != null) {
appRoleAssignedTo().forEach(e -> e.validate());
}
if (appRoleAssignments() != null) {
appRoleAssignments().forEach(e -> e.validate());
}
if (claimsMappingPolicies() != null) {
claimsMappingPolicies().forEach(e -> e.validate());
}
if (createdObjects() != null) {
createdObjects().forEach(e -> e.validate());
}
if (delegatedPermissionClassifications() != null) {
delegatedPermissionClassifications().forEach(e -> e.validate());
}
if (endpoints() != null) {
endpoints().forEach(e -> e.validate());
}
if (homeRealmDiscoveryPolicies() != null) {
homeRealmDiscoveryPolicies().forEach(e -> e.validate());
}
if (memberOf() != null) {
memberOf().forEach(e -> e.validate());
}
if (oauth2PermissionGrants() != null) {
oauth2PermissionGrants().forEach(e -> e.validate());
}
if (ownedObjects() != null) {
ownedObjects().forEach(e -> e.validate());
}
if (owners() != null) {
owners().forEach(e -> e.validate());
}
if (tokenIssuancePolicies() != null) {
tokenIssuancePolicies().forEach(e -> e.validate());
}
if (tokenLifetimePolicies() != null) {
tokenLifetimePolicies().forEach(e -> e.validate());
}
if (transitiveMemberOf() != null) {
transitiveMemberOf().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("id", id());
jsonWriter.writeStringField("deletedDateTime",
deletedDateTime() == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(deletedDateTime()));
jsonWriter.writeBooleanField("accountEnabled", this.accountEnabled);
jsonWriter.writeArrayField("addIns", this.addIns, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("alternativeNames", this.alternativeNames,
(writer, element) -> writer.writeString(element));
jsonWriter.writeStringField("appDescription", this.appDescription);
jsonWriter.writeStringField("appDisplayName", this.appDisplayName);
jsonWriter.writeStringField("appId", this.appId);
jsonWriter.writeStringField("applicationTemplateId", this.applicationTemplateId);
jsonWriter.writeStringField("appOwnerOrganizationId", Objects.toString(this.appOwnerOrganizationId, null));
jsonWriter.writeBooleanField("appRoleAssignmentRequired", this.appRoleAssignmentRequired);
jsonWriter.writeArrayField("appRoles", this.appRoles, (writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("description", this.description);
jsonWriter.writeStringField("displayName", this.displayName);
jsonWriter.writeStringField("homepage", this.homepage);
jsonWriter.writeJsonField("info", this.info);
jsonWriter.writeArrayField("keyCredentials", this.keyCredentials,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("loginUrl", this.loginUrl);
jsonWriter.writeStringField("logoutUrl", this.logoutUrl);
jsonWriter.writeStringField("notes", this.notes);
jsonWriter.writeArrayField("notificationEmailAddresses", this.notificationEmailAddresses,
(writer, element) -> writer.writeString(element));
jsonWriter.writeArrayField("oauth2PermissionScopes", this.oauth2PermissionScopes,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("passwordCredentials", this.passwordCredentials,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("preferredSingleSignOnMode", this.preferredSingleSignOnMode);
jsonWriter.writeStringField("preferredTokenSigningKeyThumbprint", this.preferredTokenSigningKeyThumbprint);
jsonWriter.writeArrayField("replyUrls", this.replyUrls, (writer, element) -> writer.writeString(element));
jsonWriter.writeJsonField("samlSingleSignOnSettings", this.samlSingleSignOnSettings);
jsonWriter.writeArrayField("servicePrincipalNames", this.servicePrincipalNames,
(writer, element) -> writer.writeString(element));
jsonWriter.writeStringField("servicePrincipalType", this.servicePrincipalType);
jsonWriter.writeStringField("signInAudience", this.signInAudience);
jsonWriter.writeArrayField("tags", this.tags, (writer, element) -> writer.writeString(element));
jsonWriter.writeStringField("tokenEncryptionKeyId", Objects.toString(this.tokenEncryptionKeyId, null));
jsonWriter.writeArrayField("appRoleAssignedTo", this.appRoleAssignedTo,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("appRoleAssignments", this.appRoleAssignments,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("claimsMappingPolicies", this.claimsMappingPolicies,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("createdObjects", this.createdObjects,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("delegatedPermissionClassifications", this.delegatedPermissionClassifications,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("endpoints", this.endpoints, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("homeRealmDiscoveryPolicies", this.homeRealmDiscoveryPolicies,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("memberOf", this.memberOf, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("oauth2PermissionGrants", this.oauth2PermissionGrants,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("ownedObjects", this.ownedObjects, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("owners", this.owners, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("tokenIssuancePolicies", this.tokenIssuancePolicies,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("tokenLifetimePolicies", this.tokenLifetimePolicies,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("transitiveMemberOf", this.transitiveMemberOf,
(writer, element) -> writer.writeJson(element));
if (additionalProperties != null) {
for (Map.Entry additionalProperty : additionalProperties.entrySet()) {
jsonWriter.writeUntypedField(additionalProperty.getKey(), additionalProperty.getValue());
}
}
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MicrosoftGraphServicePrincipalInner from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MicrosoftGraphServicePrincipalInner if the JsonReader was pointing to an instance of it,
* or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the MicrosoftGraphServicePrincipalInner.
*/
public static MicrosoftGraphServicePrincipalInner fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
MicrosoftGraphServicePrincipalInner deserializedMicrosoftGraphServicePrincipalInner
= new MicrosoftGraphServicePrincipalInner();
Map additionalProperties = null;
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.withId(reader.getString());
} else if ("deletedDateTime".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.withDeletedDateTime(reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString())));
} else if ("accountEnabled".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.accountEnabled
= reader.getNullable(JsonReader::getBoolean);
} else if ("addIns".equals(fieldName)) {
List addIns
= reader.readArray(reader1 -> MicrosoftGraphAddIn.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.addIns = addIns;
} else if ("alternativeNames".equals(fieldName)) {
List alternativeNames = reader.readArray(reader1 -> reader1.getString());
deserializedMicrosoftGraphServicePrincipalInner.alternativeNames = alternativeNames;
} else if ("appDescription".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.appDescription = reader.getString();
} else if ("appDisplayName".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.appDisplayName = reader.getString();
} else if ("appId".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.appId = reader.getString();
} else if ("applicationTemplateId".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.applicationTemplateId = reader.getString();
} else if ("appOwnerOrganizationId".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.appOwnerOrganizationId
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else if ("appRoleAssignmentRequired".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.appRoleAssignmentRequired
= reader.getNullable(JsonReader::getBoolean);
} else if ("appRoles".equals(fieldName)) {
List appRoles
= reader.readArray(reader1 -> MicrosoftGraphAppRole.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.appRoles = appRoles;
} else if ("description".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.description = reader.getString();
} else if ("displayName".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.displayName = reader.getString();
} else if ("homepage".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.homepage = reader.getString();
} else if ("info".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.info
= MicrosoftGraphInformationalUrl.fromJson(reader);
} else if ("keyCredentials".equals(fieldName)) {
List keyCredentials
= reader.readArray(reader1 -> MicrosoftGraphKeyCredentialInner.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.keyCredentials = keyCredentials;
} else if ("loginUrl".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.loginUrl = reader.getString();
} else if ("logoutUrl".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.logoutUrl = reader.getString();
} else if ("notes".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.notes = reader.getString();
} else if ("notificationEmailAddresses".equals(fieldName)) {
List notificationEmailAddresses = reader.readArray(reader1 -> reader1.getString());
deserializedMicrosoftGraphServicePrincipalInner.notificationEmailAddresses
= notificationEmailAddresses;
} else if ("oauth2PermissionScopes".equals(fieldName)) {
List oauth2PermissionScopes
= reader.readArray(reader1 -> MicrosoftGraphPermissionScope.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.oauth2PermissionScopes = oauth2PermissionScopes;
} else if ("passwordCredentials".equals(fieldName)) {
List passwordCredentials
= reader.readArray(reader1 -> MicrosoftGraphPasswordCredentialInner.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.passwordCredentials = passwordCredentials;
} else if ("preferredSingleSignOnMode".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.preferredSingleSignOnMode = reader.getString();
} else if ("preferredTokenSigningKeyThumbprint".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.preferredTokenSigningKeyThumbprint
= reader.getString();
} else if ("replyUrls".equals(fieldName)) {
List replyUrls = reader.readArray(reader1 -> reader1.getString());
deserializedMicrosoftGraphServicePrincipalInner.replyUrls = replyUrls;
} else if ("samlSingleSignOnSettings".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.samlSingleSignOnSettings
= MicrosoftGraphSamlSingleSignOnSettings.fromJson(reader);
} else if ("servicePrincipalNames".equals(fieldName)) {
List servicePrincipalNames = reader.readArray(reader1 -> reader1.getString());
deserializedMicrosoftGraphServicePrincipalInner.servicePrincipalNames = servicePrincipalNames;
} else if ("servicePrincipalType".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.servicePrincipalType = reader.getString();
} else if ("signInAudience".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.signInAudience = reader.getString();
} else if ("tags".equals(fieldName)) {
List tags = reader.readArray(reader1 -> reader1.getString());
deserializedMicrosoftGraphServicePrincipalInner.tags = tags;
} else if ("tokenEncryptionKeyId".equals(fieldName)) {
deserializedMicrosoftGraphServicePrincipalInner.tokenEncryptionKeyId
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else if ("appRoleAssignedTo".equals(fieldName)) {
List appRoleAssignedTo
= reader.readArray(reader1 -> MicrosoftGraphAppRoleAssignment.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.appRoleAssignedTo = appRoleAssignedTo;
} else if ("appRoleAssignments".equals(fieldName)) {
List appRoleAssignments
= reader.readArray(reader1 -> MicrosoftGraphAppRoleAssignment.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.appRoleAssignments = appRoleAssignments;
} else if ("claimsMappingPolicies".equals(fieldName)) {
List claimsMappingPolicies
= reader.readArray(reader1 -> MicrosoftGraphClaimsMappingPolicy.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.claimsMappingPolicies = claimsMappingPolicies;
} else if ("createdObjects".equals(fieldName)) {
List createdObjects
= reader.readArray(reader1 -> MicrosoftGraphDirectoryObjectInner.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.createdObjects = createdObjects;
} else if ("delegatedPermissionClassifications".equals(fieldName)) {
List delegatedPermissionClassifications = reader
.readArray(reader1 -> MicrosoftGraphDelegatedPermissionClassification.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.delegatedPermissionClassifications
= delegatedPermissionClassifications;
} else if ("endpoints".equals(fieldName)) {
List endpoints
= reader.readArray(reader1 -> MicrosoftGraphEndpoint.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.endpoints = endpoints;
} else if ("homeRealmDiscoveryPolicies".equals(fieldName)) {
List homeRealmDiscoveryPolicies
= reader.readArray(reader1 -> MicrosoftGraphHomeRealmDiscoveryPolicyInner.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.homeRealmDiscoveryPolicies
= homeRealmDiscoveryPolicies;
} else if ("memberOf".equals(fieldName)) {
List memberOf
= reader.readArray(reader1 -> MicrosoftGraphDirectoryObjectInner.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.memberOf = memberOf;
} else if ("oauth2PermissionGrants".equals(fieldName)) {
List oauth2PermissionGrants
= reader.readArray(reader1 -> MicrosoftGraphOAuth2PermissionGrant.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.oauth2PermissionGrants = oauth2PermissionGrants;
} else if ("ownedObjects".equals(fieldName)) {
List ownedObjects
= reader.readArray(reader1 -> MicrosoftGraphDirectoryObjectInner.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.ownedObjects = ownedObjects;
} else if ("owners".equals(fieldName)) {
List owners
= reader.readArray(reader1 -> MicrosoftGraphDirectoryObjectInner.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.owners = owners;
} else if ("tokenIssuancePolicies".equals(fieldName)) {
List tokenIssuancePolicies
= reader.readArray(reader1 -> MicrosoftGraphTokenIssuancePolicy.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.tokenIssuancePolicies = tokenIssuancePolicies;
} else if ("tokenLifetimePolicies".equals(fieldName)) {
List tokenLifetimePolicies
= reader.readArray(reader1 -> MicrosoftGraphTokenLifetimePolicy.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.tokenLifetimePolicies = tokenLifetimePolicies;
} else if ("transitiveMemberOf".equals(fieldName)) {
List transitiveMemberOf
= reader.readArray(reader1 -> MicrosoftGraphDirectoryObjectInner.fromJson(reader1));
deserializedMicrosoftGraphServicePrincipalInner.transitiveMemberOf = transitiveMemberOf;
} else {
if (additionalProperties == null) {
additionalProperties = new LinkedHashMap<>();
}
additionalProperties.put(fieldName, reader.readUntyped());
}
}
deserializedMicrosoftGraphServicePrincipalInner.additionalProperties = additionalProperties;
return deserializedMicrosoftGraphServicePrincipalInner;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy