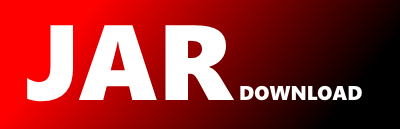
com.azure.resourcemanager.authorization.fluent.models.MicrosoftGraphTodoTask Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.authorization.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
/**
* todoTask.
*/
@Fluent
public final class MicrosoftGraphTodoTask extends MicrosoftGraphEntity {
/*
* itemBody
*/
private MicrosoftGraphItemBody body;
/*
* The date and time when the task was last modified. By default, it is in UTC. You can provide a custom time zone
* in the request header. The property value uses ISO 8601 format and is always in UTC time. For example, midnight
* UTC on Jan 1, 2020 would look like this: '2020-01-01T00:00:00Z'.
*/
private OffsetDateTime bodyLastModifiedDateTime;
/*
* dateTimeTimeZone
*/
private MicrosoftGraphDateTimeZone completedDateTime;
/*
* The date and time when the task was created. By default, it is in UTC. You can provide a custom time zone in the
* request header. The property value uses ISO 8601 format. For example, midnight UTC on Jan 1, 2020 would look like
* this: '2020-01-01T00:00:00Z'.
*/
private OffsetDateTime createdDateTime;
/*
* dateTimeTimeZone
*/
private MicrosoftGraphDateTimeZone dueDateTime;
/*
* importance
*/
private MicrosoftGraphImportance importance;
/*
* Set to true if an alert is set to remind the user of the task.
*/
private Boolean isReminderOn;
/*
* The date and time when the task was last modified. By default, it is in UTC. You can provide a custom time zone
* in the request header. The property value uses ISO 8601 format and is always in UTC time. For example, midnight
* UTC on Jan 1, 2020 would look like this: '2020-01-01T00:00:00Z'.
*/
private OffsetDateTime lastModifiedDateTime;
/*
* patternedRecurrence
*/
private MicrosoftGraphPatternedRecurrence recurrence;
/*
* dateTimeTimeZone
*/
private MicrosoftGraphDateTimeZone reminderDateTime;
/*
* taskStatus
*/
private MicrosoftGraphTaskStatus status;
/*
* A brief description of the task.
*/
private String title;
/*
* The collection of open extensions defined for the task. Nullable.
*/
private List extensions;
/*
* A collection of resources linked to the task.
*/
private List linkedResources;
/*
* todoTask
*/
private Map additionalProperties;
/**
* Creates an instance of MicrosoftGraphTodoTask class.
*/
public MicrosoftGraphTodoTask() {
}
/**
* Get the body property: itemBody.
*
* @return the body value.
*/
public MicrosoftGraphItemBody body() {
return this.body;
}
/**
* Set the body property: itemBody.
*
* @param body the body value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withBody(MicrosoftGraphItemBody body) {
this.body = body;
return this;
}
/**
* Get the bodyLastModifiedDateTime property: The date and time when the task was last modified. By default, it is
* in UTC. You can provide a custom time zone in the request header. The property value uses ISO 8601 format and is
* always in UTC time. For example, midnight UTC on Jan 1, 2020 would look like this: '2020-01-01T00:00:00Z'.
*
* @return the bodyLastModifiedDateTime value.
*/
public OffsetDateTime bodyLastModifiedDateTime() {
return this.bodyLastModifiedDateTime;
}
/**
* Set the bodyLastModifiedDateTime property: The date and time when the task was last modified. By default, it is
* in UTC. You can provide a custom time zone in the request header. The property value uses ISO 8601 format and is
* always in UTC time. For example, midnight UTC on Jan 1, 2020 would look like this: '2020-01-01T00:00:00Z'.
*
* @param bodyLastModifiedDateTime the bodyLastModifiedDateTime value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withBodyLastModifiedDateTime(OffsetDateTime bodyLastModifiedDateTime) {
this.bodyLastModifiedDateTime = bodyLastModifiedDateTime;
return this;
}
/**
* Get the completedDateTime property: dateTimeTimeZone.
*
* @return the completedDateTime value.
*/
public MicrosoftGraphDateTimeZone completedDateTime() {
return this.completedDateTime;
}
/**
* Set the completedDateTime property: dateTimeTimeZone.
*
* @param completedDateTime the completedDateTime value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withCompletedDateTime(MicrosoftGraphDateTimeZone completedDateTime) {
this.completedDateTime = completedDateTime;
return this;
}
/**
* Get the createdDateTime property: The date and time when the task was created. By default, it is in UTC. You can
* provide a custom time zone in the request header. The property value uses ISO 8601 format. For example, midnight
* UTC on Jan 1, 2020 would look like this: '2020-01-01T00:00:00Z'.
*
* @return the createdDateTime value.
*/
public OffsetDateTime createdDateTime() {
return this.createdDateTime;
}
/**
* Set the createdDateTime property: The date and time when the task was created. By default, it is in UTC. You can
* provide a custom time zone in the request header. The property value uses ISO 8601 format. For example, midnight
* UTC on Jan 1, 2020 would look like this: '2020-01-01T00:00:00Z'.
*
* @param createdDateTime the createdDateTime value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withCreatedDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
return this;
}
/**
* Get the dueDateTime property: dateTimeTimeZone.
*
* @return the dueDateTime value.
*/
public MicrosoftGraphDateTimeZone dueDateTime() {
return this.dueDateTime;
}
/**
* Set the dueDateTime property: dateTimeTimeZone.
*
* @param dueDateTime the dueDateTime value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withDueDateTime(MicrosoftGraphDateTimeZone dueDateTime) {
this.dueDateTime = dueDateTime;
return this;
}
/**
* Get the importance property: importance.
*
* @return the importance value.
*/
public MicrosoftGraphImportance importance() {
return this.importance;
}
/**
* Set the importance property: importance.
*
* @param importance the importance value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withImportance(MicrosoftGraphImportance importance) {
this.importance = importance;
return this;
}
/**
* Get the isReminderOn property: Set to true if an alert is set to remind the user of the task.
*
* @return the isReminderOn value.
*/
public Boolean isReminderOn() {
return this.isReminderOn;
}
/**
* Set the isReminderOn property: Set to true if an alert is set to remind the user of the task.
*
* @param isReminderOn the isReminderOn value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withIsReminderOn(Boolean isReminderOn) {
this.isReminderOn = isReminderOn;
return this;
}
/**
* Get the lastModifiedDateTime property: The date and time when the task was last modified. By default, it is in
* UTC. You can provide a custom time zone in the request header. The property value uses ISO 8601 format and is
* always in UTC time. For example, midnight UTC on Jan 1, 2020 would look like this: '2020-01-01T00:00:00Z'.
*
* @return the lastModifiedDateTime value.
*/
public OffsetDateTime lastModifiedDateTime() {
return this.lastModifiedDateTime;
}
/**
* Set the lastModifiedDateTime property: The date and time when the task was last modified. By default, it is in
* UTC. You can provide a custom time zone in the request header. The property value uses ISO 8601 format and is
* always in UTC time. For example, midnight UTC on Jan 1, 2020 would look like this: '2020-01-01T00:00:00Z'.
*
* @param lastModifiedDateTime the lastModifiedDateTime value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withLastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
return this;
}
/**
* Get the recurrence property: patternedRecurrence.
*
* @return the recurrence value.
*/
public MicrosoftGraphPatternedRecurrence recurrence() {
return this.recurrence;
}
/**
* Set the recurrence property: patternedRecurrence.
*
* @param recurrence the recurrence value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withRecurrence(MicrosoftGraphPatternedRecurrence recurrence) {
this.recurrence = recurrence;
return this;
}
/**
* Get the reminderDateTime property: dateTimeTimeZone.
*
* @return the reminderDateTime value.
*/
public MicrosoftGraphDateTimeZone reminderDateTime() {
return this.reminderDateTime;
}
/**
* Set the reminderDateTime property: dateTimeTimeZone.
*
* @param reminderDateTime the reminderDateTime value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withReminderDateTime(MicrosoftGraphDateTimeZone reminderDateTime) {
this.reminderDateTime = reminderDateTime;
return this;
}
/**
* Get the status property: taskStatus.
*
* @return the status value.
*/
public MicrosoftGraphTaskStatus status() {
return this.status;
}
/**
* Set the status property: taskStatus.
*
* @param status the status value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withStatus(MicrosoftGraphTaskStatus status) {
this.status = status;
return this;
}
/**
* Get the title property: A brief description of the task.
*
* @return the title value.
*/
public String title() {
return this.title;
}
/**
* Set the title property: A brief description of the task.
*
* @param title the title value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withTitle(String title) {
this.title = title;
return this;
}
/**
* Get the extensions property: The collection of open extensions defined for the task. Nullable.
*
* @return the extensions value.
*/
public List extensions() {
return this.extensions;
}
/**
* Set the extensions property: The collection of open extensions defined for the task. Nullable.
*
* @param extensions the extensions value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withExtensions(List extensions) {
this.extensions = extensions;
return this;
}
/**
* Get the linkedResources property: A collection of resources linked to the task.
*
* @return the linkedResources value.
*/
public List linkedResources() {
return this.linkedResources;
}
/**
* Set the linkedResources property: A collection of resources linked to the task.
*
* @param linkedResources the linkedResources value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withLinkedResources(List linkedResources) {
this.linkedResources = linkedResources;
return this;
}
/**
* Get the additionalProperties property: todoTask.
*
* @return the additionalProperties value.
*/
public Map additionalProperties() {
return this.additionalProperties;
}
/**
* Set the additionalProperties property: todoTask.
*
* @param additionalProperties the additionalProperties value to set.
* @return the MicrosoftGraphTodoTask object itself.
*/
public MicrosoftGraphTodoTask withAdditionalProperties(Map additionalProperties) {
this.additionalProperties = additionalProperties;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public MicrosoftGraphTodoTask withId(String id) {
super.withId(id);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (body() != null) {
body().validate();
}
if (completedDateTime() != null) {
completedDateTime().validate();
}
if (dueDateTime() != null) {
dueDateTime().validate();
}
if (recurrence() != null) {
recurrence().validate();
}
if (reminderDateTime() != null) {
reminderDateTime().validate();
}
if (extensions() != null) {
extensions().forEach(e -> e.validate());
}
if (linkedResources() != null) {
linkedResources().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("id", id());
jsonWriter.writeJsonField("body", this.body);
jsonWriter.writeStringField("bodyLastModifiedDateTime",
this.bodyLastModifiedDateTime == null
? null
: DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.bodyLastModifiedDateTime));
jsonWriter.writeJsonField("completedDateTime", this.completedDateTime);
jsonWriter.writeStringField("createdDateTime",
this.createdDateTime == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.createdDateTime));
jsonWriter.writeJsonField("dueDateTime", this.dueDateTime);
jsonWriter.writeStringField("importance", this.importance == null ? null : this.importance.toString());
jsonWriter.writeBooleanField("isReminderOn", this.isReminderOn);
jsonWriter.writeStringField("lastModifiedDateTime",
this.lastModifiedDateTime == null
? null
: DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.lastModifiedDateTime));
jsonWriter.writeJsonField("recurrence", this.recurrence);
jsonWriter.writeJsonField("reminderDateTime", this.reminderDateTime);
jsonWriter.writeStringField("status", this.status == null ? null : this.status.toString());
jsonWriter.writeStringField("title", this.title);
jsonWriter.writeArrayField("extensions", this.extensions, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("linkedResources", this.linkedResources,
(writer, element) -> writer.writeJson(element));
if (additionalProperties != null) {
for (Map.Entry additionalProperty : additionalProperties.entrySet()) {
jsonWriter.writeUntypedField(additionalProperty.getKey(), additionalProperty.getValue());
}
}
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MicrosoftGraphTodoTask from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MicrosoftGraphTodoTask if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the MicrosoftGraphTodoTask.
*/
public static MicrosoftGraphTodoTask fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
MicrosoftGraphTodoTask deserializedMicrosoftGraphTodoTask = new MicrosoftGraphTodoTask();
Map additionalProperties = null;
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedMicrosoftGraphTodoTask.withId(reader.getString());
} else if ("body".equals(fieldName)) {
deserializedMicrosoftGraphTodoTask.body = MicrosoftGraphItemBody.fromJson(reader);
} else if ("bodyLastModifiedDateTime".equals(fieldName)) {
deserializedMicrosoftGraphTodoTask.bodyLastModifiedDateTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("completedDateTime".equals(fieldName)) {
deserializedMicrosoftGraphTodoTask.completedDateTime = MicrosoftGraphDateTimeZone.fromJson(reader);
} else if ("createdDateTime".equals(fieldName)) {
deserializedMicrosoftGraphTodoTask.createdDateTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("dueDateTime".equals(fieldName)) {
deserializedMicrosoftGraphTodoTask.dueDateTime = MicrosoftGraphDateTimeZone.fromJson(reader);
} else if ("importance".equals(fieldName)) {
deserializedMicrosoftGraphTodoTask.importance
= MicrosoftGraphImportance.fromString(reader.getString());
} else if ("isReminderOn".equals(fieldName)) {
deserializedMicrosoftGraphTodoTask.isReminderOn = reader.getNullable(JsonReader::getBoolean);
} else if ("lastModifiedDateTime".equals(fieldName)) {
deserializedMicrosoftGraphTodoTask.lastModifiedDateTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("recurrence".equals(fieldName)) {
deserializedMicrosoftGraphTodoTask.recurrence = MicrosoftGraphPatternedRecurrence.fromJson(reader);
} else if ("reminderDateTime".equals(fieldName)) {
deserializedMicrosoftGraphTodoTask.reminderDateTime = MicrosoftGraphDateTimeZone.fromJson(reader);
} else if ("status".equals(fieldName)) {
deserializedMicrosoftGraphTodoTask.status = MicrosoftGraphTaskStatus.fromString(reader.getString());
} else if ("title".equals(fieldName)) {
deserializedMicrosoftGraphTodoTask.title = reader.getString();
} else if ("extensions".equals(fieldName)) {
List extensions
= reader.readArray(reader1 -> MicrosoftGraphExtension.fromJson(reader1));
deserializedMicrosoftGraphTodoTask.extensions = extensions;
} else if ("linkedResources".equals(fieldName)) {
List linkedResources
= reader.readArray(reader1 -> MicrosoftGraphLinkedResource.fromJson(reader1));
deserializedMicrosoftGraphTodoTask.linkedResources = linkedResources;
} else {
if (additionalProperties == null) {
additionalProperties = new LinkedHashMap<>();
}
additionalProperties.put(fieldName, reader.readUntyped());
}
}
deserializedMicrosoftGraphTodoTask.additionalProperties = additionalProperties;
return deserializedMicrosoftGraphTodoTask;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy