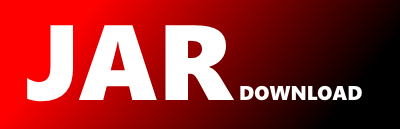
com.azure.resourcemanager.authorization.models.RoleAssignments Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.resourcemanager.authorization.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.resourcemanager.authorization.AuthorizationManager;
import com.azure.resourcemanager.resources.fluentcore.arm.collection.SupportsGettingById;
import com.azure.resourcemanager.resources.fluentcore.arm.models.HasManager;
import com.azure.resourcemanager.resources.fluentcore.collection.SupportsBatchCreation;
import com.azure.resourcemanager.resources.fluentcore.collection.SupportsCreating;
import com.azure.resourcemanager.resources.fluentcore.collection.SupportsDeletingById;
import reactor.core.publisher.Mono;
/** Entry point to role assignment management API. */
@Fluent
public interface RoleAssignments
extends SupportsGettingById,
SupportsCreating,
SupportsBatchCreation,
SupportsDeletingById,
HasManager {
/**
* Gets the information about a role assignment based on scope and name.
*
* @param scope the scope of the role assignment
* @param name the name of the role assignment
* @return an immutable representation of the role assignment
*/
Mono getByScopeAsync(String scope, String name);
/**
* Gets the information about a role assignment based on scope and name.
*
* @param scope the scope of the role assignment
* @param name the name of the role assignment
* @return an immutable representation of the role assignment
*/
RoleAssignment getByScope(String scope, String name);
/**
* List role assignments in a scope.
*
* @param scope the scope of the role assignments
* @return a list of role assignments
*/
PagedFlux listByScopeAsync(String scope);
/**
* List role assignments in a scope.
*
* @param scope the scope of the role assignments
* @return a list of role assignments
*/
PagedIterable listByScope(String scope);
/**
* List role assignments for a service principal.
*
* @param servicePrincipal the service principal
* @return a list of role assignments
*/
PagedFlux listByServicePrincipalAsync(ServicePrincipal servicePrincipal);
/**
* List role assignments for a service principal.
*
* @param servicePrincipal the service principal
* @return a list of role assignments
*/
PagedIterable listByServicePrincipal(ServicePrincipal servicePrincipal);
/**
* List role assignments for a service principal.
*
* @param principalId the ID of the service principal
* @return a list of role assignments
*/
PagedFlux listByServicePrincipalAsync(String principalId);
/**
* List role assignments for a service principal.
*
* @param principalId the ID of the service principal
* @return a list of role assignments
*/
PagedIterable listByServicePrincipal(String principalId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy