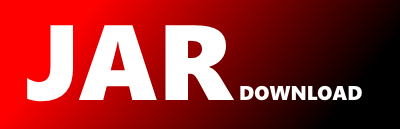
com.azure.resourcemanager.automation.fluent.AutomationClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-automation Show documentation
Show all versions of azure-resourcemanager-automation Show documentation
This package contains Microsoft Azure SDK for Automation Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Automation Client. Package tag package-2019-06.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.automation.fluent;
import com.azure.core.http.HttpPipeline;
import java.time.Duration;
/** The interface for AutomationClient class. */
public interface AutomationClient {
/**
* Gets Gets subscription credentials which uniquely identify Microsoft Azure subscription. The subscription ID
* forms part of the URI for every service call.
*
* @return the subscriptionId value.
*/
String getSubscriptionId();
/**
* Gets server parameter.
*
* @return the endpoint value.
*/
String getEndpoint();
/**
* Gets The HTTP pipeline to send requests through.
*
* @return the httpPipeline value.
*/
HttpPipeline getHttpPipeline();
/**
* Gets The default poll interval for long-running operation.
*
* @return the defaultPollInterval value.
*/
Duration getDefaultPollInterval();
/**
* Gets the RunbookDraftsClient object to access its operations.
*
* @return the RunbookDraftsClient object.
*/
RunbookDraftsClient getRunbookDrafts();
/**
* Gets the RunbooksClient object to access its operations.
*
* @return the RunbooksClient object.
*/
RunbooksClient getRunbooks();
/**
* Gets the TestJobStreamsClient object to access its operations.
*
* @return the TestJobStreamsClient object.
*/
TestJobStreamsClient getTestJobStreams();
/**
* Gets the TestJobsClient object to access its operations.
*
* @return the TestJobsClient object.
*/
TestJobsClient getTestJobs();
/**
* Gets the Python2PackagesClient object to access its operations.
*
* @return the Python2PackagesClient object.
*/
Python2PackagesClient getPython2Packages();
/**
* Gets the AgentRegistrationInformationsClient object to access its operations.
*
* @return the AgentRegistrationInformationsClient object.
*/
AgentRegistrationInformationsClient getAgentRegistrationInformations();
/**
* Gets the DscNodesClient object to access its operations.
*
* @return the DscNodesClient object.
*/
DscNodesClient getDscNodes();
/**
* Gets the NodeReportsClient object to access its operations.
*
* @return the NodeReportsClient object.
*/
NodeReportsClient getNodeReports();
/**
* Gets the DscCompilationJobsClient object to access its operations.
*
* @return the DscCompilationJobsClient object.
*/
DscCompilationJobsClient getDscCompilationJobs();
/**
* Gets the DscCompilationJobStreamsClient object to access its operations.
*
* @return the DscCompilationJobStreamsClient object.
*/
DscCompilationJobStreamsClient getDscCompilationJobStreams();
/**
* Gets the DscNodeConfigurationsClient object to access its operations.
*
* @return the DscNodeConfigurationsClient object.
*/
DscNodeConfigurationsClient getDscNodeConfigurations();
/**
* Gets the NodeCountInformationsClient object to access its operations.
*
* @return the NodeCountInformationsClient object.
*/
NodeCountInformationsClient getNodeCountInformations();
/**
* Gets the SoftwareUpdateConfigurationRunsClient object to access its operations.
*
* @return the SoftwareUpdateConfigurationRunsClient object.
*/
SoftwareUpdateConfigurationRunsClient getSoftwareUpdateConfigurationRuns();
/**
* Gets the SoftwareUpdateConfigurationMachineRunsClient object to access its operations.
*
* @return the SoftwareUpdateConfigurationMachineRunsClient object.
*/
SoftwareUpdateConfigurationMachineRunsClient getSoftwareUpdateConfigurationMachineRuns();
/**
* Gets the SourceControlsClient object to access its operations.
*
* @return the SourceControlsClient object.
*/
SourceControlsClient getSourceControls();
/**
* Gets the SourceControlSyncJobsClient object to access its operations.
*
* @return the SourceControlSyncJobsClient object.
*/
SourceControlSyncJobsClient getSourceControlSyncJobs();
/**
* Gets the SourceControlSyncJobStreamsClient object to access its operations.
*
* @return the SourceControlSyncJobStreamsClient object.
*/
SourceControlSyncJobStreamsClient getSourceControlSyncJobStreams();
/**
* Gets the JobsClient object to access its operations.
*
* @return the JobsClient object.
*/
JobsClient getJobs();
/**
* Gets the JobStreamsClient object to access its operations.
*
* @return the JobStreamsClient object.
*/
JobStreamsClient getJobStreams();
/**
* Gets the AutomationAccountsClient object to access its operations.
*
* @return the AutomationAccountsClient object.
*/
AutomationAccountsClient getAutomationAccounts();
/**
* Gets the StatisticsOperationsClient object to access its operations.
*
* @return the StatisticsOperationsClient object.
*/
StatisticsOperationsClient getStatisticsOperations();
/**
* Gets the UsagesClient object to access its operations.
*
* @return the UsagesClient object.
*/
UsagesClient getUsages();
/**
* Gets the KeysClient object to access its operations.
*
* @return the KeysClient object.
*/
KeysClient getKeys();
/**
* Gets the CertificatesClient object to access its operations.
*
* @return the CertificatesClient object.
*/
CertificatesClient getCertificates();
/**
* Gets the ConnectionsClient object to access its operations.
*
* @return the ConnectionsClient object.
*/
ConnectionsClient getConnections();
/**
* Gets the ConnectionTypesClient object to access its operations.
*
* @return the ConnectionTypesClient object.
*/
ConnectionTypesClient getConnectionTypes();
/**
* Gets the CredentialsClient object to access its operations.
*
* @return the CredentialsClient object.
*/
CredentialsClient getCredentials();
/**
* Gets the DscConfigurationsClient object to access its operations.
*
* @return the DscConfigurationsClient object.
*/
DscConfigurationsClient getDscConfigurations();
/**
* Gets the SoftwareUpdateConfigurationsClient object to access its operations.
*
* @return the SoftwareUpdateConfigurationsClient object.
*/
SoftwareUpdateConfigurationsClient getSoftwareUpdateConfigurations();
/**
* Gets the HybridRunbookWorkerGroupsClient object to access its operations.
*
* @return the HybridRunbookWorkerGroupsClient object.
*/
HybridRunbookWorkerGroupsClient getHybridRunbookWorkerGroups();
/**
* Gets the JobSchedulesClient object to access its operations.
*
* @return the JobSchedulesClient object.
*/
JobSchedulesClient getJobSchedules();
/**
* Gets the LinkedWorkspacesClient object to access its operations.
*
* @return the LinkedWorkspacesClient object.
*/
LinkedWorkspacesClient getLinkedWorkspaces();
/**
* Gets the ActivitiesClient object to access its operations.
*
* @return the ActivitiesClient object.
*/
ActivitiesClient getActivities();
/**
* Gets the ModulesClient object to access its operations.
*
* @return the ModulesClient object.
*/
ModulesClient getModules();
/**
* Gets the ObjectDataTypesClient object to access its operations.
*
* @return the ObjectDataTypesClient object.
*/
ObjectDataTypesClient getObjectDataTypes();
/**
* Gets the FieldsClient object to access its operations.
*
* @return the FieldsClient object.
*/
FieldsClient getFields();
/**
* Gets the OperationsClient object to access its operations.
*
* @return the OperationsClient object.
*/
OperationsClient getOperations();
/**
* Gets the SchedulesClient object to access its operations.
*
* @return the SchedulesClient object.
*/
SchedulesClient getSchedules();
/**
* Gets the VariablesClient object to access its operations.
*
* @return the VariablesClient object.
*/
VariablesClient getVariables();
/**
* Gets the WatchersClient object to access its operations.
*
* @return the WatchersClient object.
*/
WatchersClient getWatchers();
/**
* Gets the WebhooksClient object to access its operations.
*
* @return the WebhooksClient object.
*/
WebhooksClient getWebhooks();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy