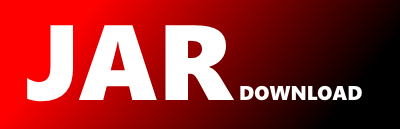
com.azure.resourcemanager.automation.fluent.models.HybridRunbookWorkerGroupInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-automation Show documentation
Show all versions of azure-resourcemanager-automation Show documentation
This package contains Microsoft Azure SDK for Automation Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Automation Client. Package tag package-2019-06.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.automation.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.resourcemanager.automation.models.GroupTypeEnum;
import com.azure.resourcemanager.automation.models.HybridRunbookWorker;
import com.azure.resourcemanager.automation.models.RunAsCredentialAssociationProperty;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
/** Definition of hybrid runbook worker group. */
@Fluent
public final class HybridRunbookWorkerGroupInner {
@JsonIgnore private final ClientLogger logger = new ClientLogger(HybridRunbookWorkerGroupInner.class);
/*
* Gets or sets the id of the resource.
*/
@JsonProperty(value = "id")
private String id;
/*
* Gets or sets the name of the group.
*/
@JsonProperty(value = "name")
private String name;
/*
* Gets or sets the list of hybrid runbook workers.
*/
@JsonProperty(value = "hybridRunbookWorkers")
private List hybridRunbookWorkers;
/*
* Sets the credential of a worker group.
*/
@JsonProperty(value = "credential")
private RunAsCredentialAssociationProperty credential;
/*
* Type of the HybridWorkerGroup.
*/
@JsonProperty(value = "groupType")
private GroupTypeEnum groupType;
/**
* Get the id property: Gets or sets the id of the resource.
*
* @return the id value.
*/
public String id() {
return this.id;
}
/**
* Set the id property: Gets or sets the id of the resource.
*
* @param id the id value to set.
* @return the HybridRunbookWorkerGroupInner object itself.
*/
public HybridRunbookWorkerGroupInner withId(String id) {
this.id = id;
return this;
}
/**
* Get the name property: Gets or sets the name of the group.
*
* @return the name value.
*/
public String name() {
return this.name;
}
/**
* Set the name property: Gets or sets the name of the group.
*
* @param name the name value to set.
* @return the HybridRunbookWorkerGroupInner object itself.
*/
public HybridRunbookWorkerGroupInner withName(String name) {
this.name = name;
return this;
}
/**
* Get the hybridRunbookWorkers property: Gets or sets the list of hybrid runbook workers.
*
* @return the hybridRunbookWorkers value.
*/
public List hybridRunbookWorkers() {
return this.hybridRunbookWorkers;
}
/**
* Set the hybridRunbookWorkers property: Gets or sets the list of hybrid runbook workers.
*
* @param hybridRunbookWorkers the hybridRunbookWorkers value to set.
* @return the HybridRunbookWorkerGroupInner object itself.
*/
public HybridRunbookWorkerGroupInner withHybridRunbookWorkers(List hybridRunbookWorkers) {
this.hybridRunbookWorkers = hybridRunbookWorkers;
return this;
}
/**
* Get the credential property: Sets the credential of a worker group.
*
* @return the credential value.
*/
public RunAsCredentialAssociationProperty credential() {
return this.credential;
}
/**
* Set the credential property: Sets the credential of a worker group.
*
* @param credential the credential value to set.
* @return the HybridRunbookWorkerGroupInner object itself.
*/
public HybridRunbookWorkerGroupInner withCredential(RunAsCredentialAssociationProperty credential) {
this.credential = credential;
return this;
}
/**
* Get the groupType property: Type of the HybridWorkerGroup.
*
* @return the groupType value.
*/
public GroupTypeEnum groupType() {
return this.groupType;
}
/**
* Set the groupType property: Type of the HybridWorkerGroup.
*
* @param groupType the groupType value to set.
* @return the HybridRunbookWorkerGroupInner object itself.
*/
public HybridRunbookWorkerGroupInner withGroupType(GroupTypeEnum groupType) {
this.groupType = groupType;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (hybridRunbookWorkers() != null) {
hybridRunbookWorkers().forEach(e -> e.validate());
}
if (credential() != null) {
credential().validate();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy