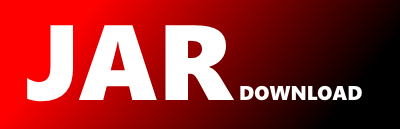
com.azure.resourcemanager.automation.fluent.models.RunbookCreateOrUpdateParametersInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-automation Show documentation
Show all versions of azure-resourcemanager-automation Show documentation
This package contains Microsoft Azure SDK for Automation Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Automation Client. Package tag package-2019-06.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.automation.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.annotation.JsonFlatten;
import com.azure.core.util.logging.ClientLogger;
import com.azure.resourcemanager.automation.models.ContentLink;
import com.azure.resourcemanager.automation.models.RunbookTypeEnum;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Map;
/** The parameters supplied to the create or update runbook operation. */
@JsonFlatten
@Fluent
public class RunbookCreateOrUpdateParametersInner {
@JsonIgnore private final ClientLogger logger = new ClientLogger(RunbookCreateOrUpdateParametersInner.class);
/*
* Gets or sets the name of the resource.
*/
@JsonProperty(value = "name")
private String name;
/*
* Gets or sets the location of the resource.
*/
@JsonProperty(value = "location")
private String location;
/*
* Gets or sets the tags attached to the resource.
*/
@JsonProperty(value = "tags")
private Map tags;
/*
* Gets or sets verbose log option.
*/
@JsonProperty(value = "properties.logVerbose")
private Boolean logVerbose;
/*
* Gets or sets progress log option.
*/
@JsonProperty(value = "properties.logProgress")
private Boolean logProgress;
/*
* Gets or sets the type of the runbook.
*/
@JsonProperty(value = "properties.runbookType", required = true)
private RunbookTypeEnum runbookType;
/*
* Gets or sets the draft runbook properties.
*/
@JsonProperty(value = "properties.draft")
private RunbookDraftInner draft;
/*
* Gets or sets the published runbook content link.
*/
@JsonProperty(value = "properties.publishContentLink")
private ContentLink publishContentLink;
/*
* Gets or sets the description of the runbook.
*/
@JsonProperty(value = "properties.description")
private String description;
/*
* Gets or sets the activity-level tracing options of the runbook.
*/
@JsonProperty(value = "properties.logActivityTrace")
private Integer logActivityTrace;
/**
* Get the name property: Gets or sets the name of the resource.
*
* @return the name value.
*/
public String name() {
return this.name;
}
/**
* Set the name property: Gets or sets the name of the resource.
*
* @param name the name value to set.
* @return the RunbookCreateOrUpdateParametersInner object itself.
*/
public RunbookCreateOrUpdateParametersInner withName(String name) {
this.name = name;
return this;
}
/**
* Get the location property: Gets or sets the location of the resource.
*
* @return the location value.
*/
public String location() {
return this.location;
}
/**
* Set the location property: Gets or sets the location of the resource.
*
* @param location the location value to set.
* @return the RunbookCreateOrUpdateParametersInner object itself.
*/
public RunbookCreateOrUpdateParametersInner withLocation(String location) {
this.location = location;
return this;
}
/**
* Get the tags property: Gets or sets the tags attached to the resource.
*
* @return the tags value.
*/
public Map tags() {
return this.tags;
}
/**
* Set the tags property: Gets or sets the tags attached to the resource.
*
* @param tags the tags value to set.
* @return the RunbookCreateOrUpdateParametersInner object itself.
*/
public RunbookCreateOrUpdateParametersInner withTags(Map tags) {
this.tags = tags;
return this;
}
/**
* Get the logVerbose property: Gets or sets verbose log option.
*
* @return the logVerbose value.
*/
public Boolean logVerbose() {
return this.logVerbose;
}
/**
* Set the logVerbose property: Gets or sets verbose log option.
*
* @param logVerbose the logVerbose value to set.
* @return the RunbookCreateOrUpdateParametersInner object itself.
*/
public RunbookCreateOrUpdateParametersInner withLogVerbose(Boolean logVerbose) {
this.logVerbose = logVerbose;
return this;
}
/**
* Get the logProgress property: Gets or sets progress log option.
*
* @return the logProgress value.
*/
public Boolean logProgress() {
return this.logProgress;
}
/**
* Set the logProgress property: Gets or sets progress log option.
*
* @param logProgress the logProgress value to set.
* @return the RunbookCreateOrUpdateParametersInner object itself.
*/
public RunbookCreateOrUpdateParametersInner withLogProgress(Boolean logProgress) {
this.logProgress = logProgress;
return this;
}
/**
* Get the runbookType property: Gets or sets the type of the runbook.
*
* @return the runbookType value.
*/
public RunbookTypeEnum runbookType() {
return this.runbookType;
}
/**
* Set the runbookType property: Gets or sets the type of the runbook.
*
* @param runbookType the runbookType value to set.
* @return the RunbookCreateOrUpdateParametersInner object itself.
*/
public RunbookCreateOrUpdateParametersInner withRunbookType(RunbookTypeEnum runbookType) {
this.runbookType = runbookType;
return this;
}
/**
* Get the draft property: Gets or sets the draft runbook properties.
*
* @return the draft value.
*/
public RunbookDraftInner draft() {
return this.draft;
}
/**
* Set the draft property: Gets or sets the draft runbook properties.
*
* @param draft the draft value to set.
* @return the RunbookCreateOrUpdateParametersInner object itself.
*/
public RunbookCreateOrUpdateParametersInner withDraft(RunbookDraftInner draft) {
this.draft = draft;
return this;
}
/**
* Get the publishContentLink property: Gets or sets the published runbook content link.
*
* @return the publishContentLink value.
*/
public ContentLink publishContentLink() {
return this.publishContentLink;
}
/**
* Set the publishContentLink property: Gets or sets the published runbook content link.
*
* @param publishContentLink the publishContentLink value to set.
* @return the RunbookCreateOrUpdateParametersInner object itself.
*/
public RunbookCreateOrUpdateParametersInner withPublishContentLink(ContentLink publishContentLink) {
this.publishContentLink = publishContentLink;
return this;
}
/**
* Get the description property: Gets or sets the description of the runbook.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Set the description property: Gets or sets the description of the runbook.
*
* @param description the description value to set.
* @return the RunbookCreateOrUpdateParametersInner object itself.
*/
public RunbookCreateOrUpdateParametersInner withDescription(String description) {
this.description = description;
return this;
}
/**
* Get the logActivityTrace property: Gets or sets the activity-level tracing options of the runbook.
*
* @return the logActivityTrace value.
*/
public Integer logActivityTrace() {
return this.logActivityTrace;
}
/**
* Set the logActivityTrace property: Gets or sets the activity-level tracing options of the runbook.
*
* @param logActivityTrace the logActivityTrace value to set.
* @return the RunbookCreateOrUpdateParametersInner object itself.
*/
public RunbookCreateOrUpdateParametersInner withLogActivityTrace(Integer logActivityTrace) {
this.logActivityTrace = logActivityTrace;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (runbookType() == null) {
throw logger
.logExceptionAsError(
new IllegalArgumentException(
"Missing required property runbookType in model RunbookCreateOrUpdateParametersInner"));
}
if (draft() != null) {
draft().validate();
}
if (publishContentLink() != null) {
publishContentLink().validate();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy