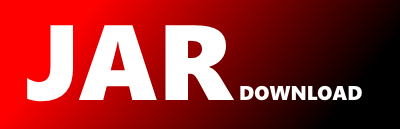
com.azure.resourcemanager.automation.models.DscCompilationJobCreateParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-automation Show documentation
Show all versions of azure-resourcemanager-automation Show documentation
This package contains Microsoft Azure SDK for Automation Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Automation Client. Package tag package-2019-06.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.automation.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.annotation.JsonFlatten;
import com.azure.core.util.logging.ClientLogger;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Map;
/** The parameters supplied to the create compilation job operation. */
@JsonFlatten
@Fluent
public class DscCompilationJobCreateParameters {
@JsonIgnore private final ClientLogger logger = new ClientLogger(DscCompilationJobCreateParameters.class);
/*
* Gets or sets name of the resource.
*/
@JsonProperty(value = "name")
private String name;
/*
* Gets or sets the location of the resource.
*/
@JsonProperty(value = "location")
private String location;
/*
* Gets or sets the tags attached to the resource.
*/
@JsonProperty(value = "tags")
private Map tags;
/*
* Gets or sets the configuration.
*/
@JsonProperty(value = "properties.configuration", required = true)
private DscConfigurationAssociationProperty configuration;
/*
* Gets or sets the parameters of the job.
*/
@JsonProperty(value = "properties.parameters")
private Map parameters;
/*
* If a new build version of NodeConfiguration is required.
*/
@JsonProperty(value = "properties.incrementNodeConfigurationBuild")
private Boolean incrementNodeConfigurationBuild;
/**
* Get the name property: Gets or sets name of the resource.
*
* @return the name value.
*/
public String name() {
return this.name;
}
/**
* Set the name property: Gets or sets name of the resource.
*
* @param name the name value to set.
* @return the DscCompilationJobCreateParameters object itself.
*/
public DscCompilationJobCreateParameters withName(String name) {
this.name = name;
return this;
}
/**
* Get the location property: Gets or sets the location of the resource.
*
* @return the location value.
*/
public String location() {
return this.location;
}
/**
* Set the location property: Gets or sets the location of the resource.
*
* @param location the location value to set.
* @return the DscCompilationJobCreateParameters object itself.
*/
public DscCompilationJobCreateParameters withLocation(String location) {
this.location = location;
return this;
}
/**
* Get the tags property: Gets or sets the tags attached to the resource.
*
* @return the tags value.
*/
public Map tags() {
return this.tags;
}
/**
* Set the tags property: Gets or sets the tags attached to the resource.
*
* @param tags the tags value to set.
* @return the DscCompilationJobCreateParameters object itself.
*/
public DscCompilationJobCreateParameters withTags(Map tags) {
this.tags = tags;
return this;
}
/**
* Get the configuration property: Gets or sets the configuration.
*
* @return the configuration value.
*/
public DscConfigurationAssociationProperty configuration() {
return this.configuration;
}
/**
* Set the configuration property: Gets or sets the configuration.
*
* @param configuration the configuration value to set.
* @return the DscCompilationJobCreateParameters object itself.
*/
public DscCompilationJobCreateParameters withConfiguration(DscConfigurationAssociationProperty configuration) {
this.configuration = configuration;
return this;
}
/**
* Get the parameters property: Gets or sets the parameters of the job.
*
* @return the parameters value.
*/
public Map parameters() {
return this.parameters;
}
/**
* Set the parameters property: Gets or sets the parameters of the job.
*
* @param parameters the parameters value to set.
* @return the DscCompilationJobCreateParameters object itself.
*/
public DscCompilationJobCreateParameters withParameters(Map parameters) {
this.parameters = parameters;
return this;
}
/**
* Get the incrementNodeConfigurationBuild property: If a new build version of NodeConfiguration is required.
*
* @return the incrementNodeConfigurationBuild value.
*/
public Boolean incrementNodeConfigurationBuild() {
return this.incrementNodeConfigurationBuild;
}
/**
* Set the incrementNodeConfigurationBuild property: If a new build version of NodeConfiguration is required.
*
* @param incrementNodeConfigurationBuild the incrementNodeConfigurationBuild value to set.
* @return the DscCompilationJobCreateParameters object itself.
*/
public DscCompilationJobCreateParameters withIncrementNodeConfigurationBuild(
Boolean incrementNodeConfigurationBuild) {
this.incrementNodeConfigurationBuild = incrementNodeConfigurationBuild;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (configuration() == null) {
throw logger
.logExceptionAsError(
new IllegalArgumentException(
"Missing required property configuration in model DscCompilationJobCreateParameters"));
} else {
configuration().validate();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy