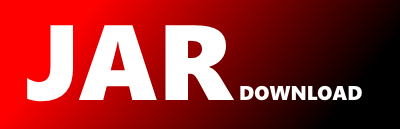
com.azure.resourcemanager.automation.models.SourceControlUpdateParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-automation Show documentation
Show all versions of azure-resourcemanager-automation Show documentation
This package contains Microsoft Azure SDK for Automation Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Automation Client. Package tag package-2019-06.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.automation.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.annotation.JsonFlatten;
import com.azure.core.util.logging.ClientLogger;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
/** The parameters supplied to the update source control operation. */
@JsonFlatten
@Fluent
public class SourceControlUpdateParameters {
@JsonIgnore private final ClientLogger logger = new ClientLogger(SourceControlUpdateParameters.class);
/*
* The repo branch of the source control.
*/
@JsonProperty(value = "properties.branch")
private String branch;
/*
* The folder path of the source control. Path must be relative.
*/
@JsonProperty(value = "properties.folderPath")
private String folderPath;
/*
* The auto sync of the source control. Default is false.
*/
@JsonProperty(value = "properties.autoSync")
private Boolean autoSync;
/*
* The auto publish of the source control. Default is true.
*/
@JsonProperty(value = "properties.publishRunbook")
private Boolean publishRunbook;
/*
* The authorization token for the repo of the source control.
*/
@JsonProperty(value = "properties.securityToken")
private SourceControlSecurityTokenProperties securityToken;
/*
* The user description of the source control.
*/
@JsonProperty(value = "properties.description")
private String description;
/**
* Get the branch property: The repo branch of the source control.
*
* @return the branch value.
*/
public String branch() {
return this.branch;
}
/**
* Set the branch property: The repo branch of the source control.
*
* @param branch the branch value to set.
* @return the SourceControlUpdateParameters object itself.
*/
public SourceControlUpdateParameters withBranch(String branch) {
this.branch = branch;
return this;
}
/**
* Get the folderPath property: The folder path of the source control. Path must be relative.
*
* @return the folderPath value.
*/
public String folderPath() {
return this.folderPath;
}
/**
* Set the folderPath property: The folder path of the source control. Path must be relative.
*
* @param folderPath the folderPath value to set.
* @return the SourceControlUpdateParameters object itself.
*/
public SourceControlUpdateParameters withFolderPath(String folderPath) {
this.folderPath = folderPath;
return this;
}
/**
* Get the autoSync property: The auto sync of the source control. Default is false.
*
* @return the autoSync value.
*/
public Boolean autoSync() {
return this.autoSync;
}
/**
* Set the autoSync property: The auto sync of the source control. Default is false.
*
* @param autoSync the autoSync value to set.
* @return the SourceControlUpdateParameters object itself.
*/
public SourceControlUpdateParameters withAutoSync(Boolean autoSync) {
this.autoSync = autoSync;
return this;
}
/**
* Get the publishRunbook property: The auto publish of the source control. Default is true.
*
* @return the publishRunbook value.
*/
public Boolean publishRunbook() {
return this.publishRunbook;
}
/**
* Set the publishRunbook property: The auto publish of the source control. Default is true.
*
* @param publishRunbook the publishRunbook value to set.
* @return the SourceControlUpdateParameters object itself.
*/
public SourceControlUpdateParameters withPublishRunbook(Boolean publishRunbook) {
this.publishRunbook = publishRunbook;
return this;
}
/**
* Get the securityToken property: The authorization token for the repo of the source control.
*
* @return the securityToken value.
*/
public SourceControlSecurityTokenProperties securityToken() {
return this.securityToken;
}
/**
* Set the securityToken property: The authorization token for the repo of the source control.
*
* @param securityToken the securityToken value to set.
* @return the SourceControlUpdateParameters object itself.
*/
public SourceControlUpdateParameters withSecurityToken(SourceControlSecurityTokenProperties securityToken) {
this.securityToken = securityToken;
return this;
}
/**
* Get the description property: The user description of the source control.
*
* @return the description value.
*/
public String description() {
return this.description;
}
/**
* Set the description property: The user description of the source control.
*
* @param description the description value to set.
* @return the SourceControlUpdateParameters object itself.
*/
public SourceControlUpdateParameters withDescription(String description) {
this.description = description;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (securityToken() != null) {
securityToken().validate();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy