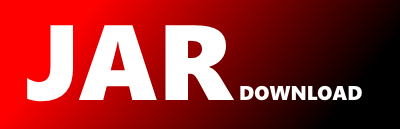
com.azure.resourcemanager.automation.fluent.models.UpdateConfigurationMachineRunProperties Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.automation.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.exception.ManagementError;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.automation.models.JobNavigation;
import com.azure.resourcemanager.automation.models.UpdateConfigurationNavigation;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.UUID;
/**
* Software update configuration machine run properties.
*/
@Fluent
public final class UpdateConfigurationMachineRunProperties
implements JsonSerializable {
/*
* name of the updated computer
*/
private String targetComputer;
/*
* type of the updated computer.
*/
private String targetComputerType;
/*
* software update configuration triggered this run
*/
private UpdateConfigurationNavigation softwareUpdateConfiguration;
/*
* Status of the software update configuration machine run.
*/
private String status;
/*
* Operating system target of the software update configuration triggered this run
*/
private String osType;
/*
* correlation id of the software update configuration machine run
*/
private UUID correlationId;
/*
* source computer id of the software update configuration machine run
*/
private UUID sourceComputerId;
/*
* Start time of the software update configuration machine run.
*/
private OffsetDateTime startTime;
/*
* End time of the software update configuration machine run.
*/
private OffsetDateTime endTime;
/*
* configured duration for the software update configuration run.
*/
private String configuredDuration;
/*
* Job associated with the software update configuration machine run
*/
private JobNavigation job;
/*
* Creation time of the resource, which only appears in the response.
*/
private OffsetDateTime creationTime;
/*
* createdBy property, which only appears in the response.
*/
private String createdBy;
/*
* Last time resource was modified, which only appears in the response.
*/
private OffsetDateTime lastModifiedTime;
/*
* lastModifiedBy property, which only appears in the response.
*/
private String lastModifiedBy;
/*
* Details of provisioning error
*/
private ManagementError error;
/**
* Creates an instance of UpdateConfigurationMachineRunProperties class.
*/
public UpdateConfigurationMachineRunProperties() {
}
/**
* Get the targetComputer property: name of the updated computer.
*
* @return the targetComputer value.
*/
public String targetComputer() {
return this.targetComputer;
}
/**
* Get the targetComputerType property: type of the updated computer.
*
* @return the targetComputerType value.
*/
public String targetComputerType() {
return this.targetComputerType;
}
/**
* Get the softwareUpdateConfiguration property: software update configuration triggered this run.
*
* @return the softwareUpdateConfiguration value.
*/
public UpdateConfigurationNavigation softwareUpdateConfiguration() {
return this.softwareUpdateConfiguration;
}
/**
* Set the softwareUpdateConfiguration property: software update configuration triggered this run.
*
* @param softwareUpdateConfiguration the softwareUpdateConfiguration value to set.
* @return the UpdateConfigurationMachineRunProperties object itself.
*/
public UpdateConfigurationMachineRunProperties
withSoftwareUpdateConfiguration(UpdateConfigurationNavigation softwareUpdateConfiguration) {
this.softwareUpdateConfiguration = softwareUpdateConfiguration;
return this;
}
/**
* Get the status property: Status of the software update configuration machine run.
*
* @return the status value.
*/
public String status() {
return this.status;
}
/**
* Get the osType property: Operating system target of the software update configuration triggered this run.
*
* @return the osType value.
*/
public String osType() {
return this.osType;
}
/**
* Get the correlationId property: correlation id of the software update configuration machine run.
*
* @return the correlationId value.
*/
public UUID correlationId() {
return this.correlationId;
}
/**
* Get the sourceComputerId property: source computer id of the software update configuration machine run.
*
* @return the sourceComputerId value.
*/
public UUID sourceComputerId() {
return this.sourceComputerId;
}
/**
* Get the startTime property: Start time of the software update configuration machine run.
*
* @return the startTime value.
*/
public OffsetDateTime startTime() {
return this.startTime;
}
/**
* Get the endTime property: End time of the software update configuration machine run.
*
* @return the endTime value.
*/
public OffsetDateTime endTime() {
return this.endTime;
}
/**
* Get the configuredDuration property: configured duration for the software update configuration run.
*
* @return the configuredDuration value.
*/
public String configuredDuration() {
return this.configuredDuration;
}
/**
* Get the job property: Job associated with the software update configuration machine run.
*
* @return the job value.
*/
public JobNavigation job() {
return this.job;
}
/**
* Set the job property: Job associated with the software update configuration machine run.
*
* @param job the job value to set.
* @return the UpdateConfigurationMachineRunProperties object itself.
*/
public UpdateConfigurationMachineRunProperties withJob(JobNavigation job) {
this.job = job;
return this;
}
/**
* Get the creationTime property: Creation time of the resource, which only appears in the response.
*
* @return the creationTime value.
*/
public OffsetDateTime creationTime() {
return this.creationTime;
}
/**
* Get the createdBy property: createdBy property, which only appears in the response.
*
* @return the createdBy value.
*/
public String createdBy() {
return this.createdBy;
}
/**
* Get the lastModifiedTime property: Last time resource was modified, which only appears in the response.
*
* @return the lastModifiedTime value.
*/
public OffsetDateTime lastModifiedTime() {
return this.lastModifiedTime;
}
/**
* Get the lastModifiedBy property: lastModifiedBy property, which only appears in the response.
*
* @return the lastModifiedBy value.
*/
public String lastModifiedBy() {
return this.lastModifiedBy;
}
/**
* Get the error property: Details of provisioning error.
*
* @return the error value.
*/
public ManagementError error() {
return this.error;
}
/**
* Set the error property: Details of provisioning error.
*
* @param error the error value to set.
* @return the UpdateConfigurationMachineRunProperties object itself.
*/
public UpdateConfigurationMachineRunProperties withError(ManagementError error) {
this.error = error;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (softwareUpdateConfiguration() != null) {
softwareUpdateConfiguration().validate();
}
if (job() != null) {
job().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("softwareUpdateConfiguration", this.softwareUpdateConfiguration);
jsonWriter.writeJsonField("job", this.job);
jsonWriter.writeJsonField("error", this.error);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of UpdateConfigurationMachineRunProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of UpdateConfigurationMachineRunProperties if the JsonReader was pointing to an instance of
* it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the UpdateConfigurationMachineRunProperties.
*/
public static UpdateConfigurationMachineRunProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
UpdateConfigurationMachineRunProperties deserializedUpdateConfigurationMachineRunProperties
= new UpdateConfigurationMachineRunProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("targetComputer".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.targetComputer = reader.getString();
} else if ("targetComputerType".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.targetComputerType = reader.getString();
} else if ("softwareUpdateConfiguration".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.softwareUpdateConfiguration
= UpdateConfigurationNavigation.fromJson(reader);
} else if ("status".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.status = reader.getString();
} else if ("osType".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.osType = reader.getString();
} else if ("correlationId".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.correlationId
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else if ("sourceComputerId".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.sourceComputerId
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else if ("startTime".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.startTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("endTime".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.endTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("configuredDuration".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.configuredDuration = reader.getString();
} else if ("job".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.job = JobNavigation.fromJson(reader);
} else if ("creationTime".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.creationTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("createdBy".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.createdBy = reader.getString();
} else if ("lastModifiedTime".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.lastModifiedTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("lastModifiedBy".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.lastModifiedBy = reader.getString();
} else if ("error".equals(fieldName)) {
deserializedUpdateConfigurationMachineRunProperties.error = ManagementError.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedUpdateConfigurationMachineRunProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy