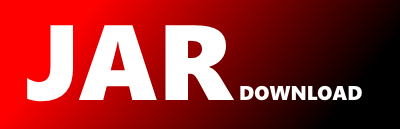
com.azure.resourcemanager.avs.fluent.WorkloadNetworksClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-avs Show documentation
Show all versions of azure-resourcemanager-avs Show documentation
This package contains Microsoft Azure SDK for Avs Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Azure VMware Solution API. Package tag package-2023-09-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.avs.fluent;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkDhcpInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkDnsServiceInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkDnsZoneInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkGatewayInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkPortMirroringInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkPublicIpInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkSegmentInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkVirtualMachineInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkVMGroupInner;
/**
* An instance of this class provides access to all the operations defined in WorkloadNetworksClient.
*/
public interface WorkloadNetworksClient {
/**
* List WorkloadNetwork resources by PrivateCloud.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetwork list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable list(String resourceGroupName, String privateCloudName);
/**
* List WorkloadNetwork resources by PrivateCloud.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetwork list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable list(String resourceGroupName, String privateCloudName, Context context);
/**
* Get a WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetwork along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getWithResponse(String resourceGroupName, String privateCloudName, Context context);
/**
* Get a WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetwork.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkInner get(String resourceGroupName, String privateCloudName);
/**
* List WorkloadNetworkDhcp resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkDhcp list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listDhcp(String resourceGroupName, String privateCloudName);
/**
* List WorkloadNetworkDhcp resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkDhcp list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listDhcp(String resourceGroupName, String privateCloudName,
Context context);
/**
* Get a WorkloadNetworkDhcp.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param dhcpId The ID of the DHCP configuration.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkDhcp along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getDhcpWithResponse(String resourceGroupName, String dhcpId,
String privateCloudName, Context context);
/**
* Get a WorkloadNetworkDhcp.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param dhcpId The ID of the DHCP configuration.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkDhcp.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDhcpInner getDhcp(String resourceGroupName, String dhcpId, String privateCloudName);
/**
* Create a WorkloadNetworkDhcp.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dhcpId The ID of the DHCP configuration.
* @param workloadNetworkDhcp Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX DHCP.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkDhcpInner> beginCreateDhcp(String resourceGroupName,
String privateCloudName, String dhcpId, WorkloadNetworkDhcpInner workloadNetworkDhcp);
/**
* Create a WorkloadNetworkDhcp.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dhcpId The ID of the DHCP configuration.
* @param workloadNetworkDhcp Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX DHCP.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkDhcpInner> beginCreateDhcp(String resourceGroupName,
String privateCloudName, String dhcpId, WorkloadNetworkDhcpInner workloadNetworkDhcp, Context context);
/**
* Create a WorkloadNetworkDhcp.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dhcpId The ID of the DHCP configuration.
* @param workloadNetworkDhcp Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX DHCP.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDhcpInner createDhcp(String resourceGroupName, String privateCloudName, String dhcpId,
WorkloadNetworkDhcpInner workloadNetworkDhcp);
/**
* Create a WorkloadNetworkDhcp.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dhcpId The ID of the DHCP configuration.
* @param workloadNetworkDhcp Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX DHCP.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDhcpInner createDhcp(String resourceGroupName, String privateCloudName, String dhcpId,
WorkloadNetworkDhcpInner workloadNetworkDhcp, Context context);
/**
* Update a WorkloadNetworkDhcp.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dhcpId The ID of the DHCP configuration.
* @param workloadNetworkDhcp The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX DHCP.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkDhcpInner> beginUpdateDhcp(String resourceGroupName,
String privateCloudName, String dhcpId, WorkloadNetworkDhcpInner workloadNetworkDhcp);
/**
* Update a WorkloadNetworkDhcp.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dhcpId The ID of the DHCP configuration.
* @param workloadNetworkDhcp The resource properties to be updated.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX DHCP.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkDhcpInner> beginUpdateDhcp(String resourceGroupName,
String privateCloudName, String dhcpId, WorkloadNetworkDhcpInner workloadNetworkDhcp, Context context);
/**
* Update a WorkloadNetworkDhcp.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dhcpId The ID of the DHCP configuration.
* @param workloadNetworkDhcp The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX DHCP.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDhcpInner updateDhcp(String resourceGroupName, String privateCloudName, String dhcpId,
WorkloadNetworkDhcpInner workloadNetworkDhcp);
/**
* Update a WorkloadNetworkDhcp.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dhcpId The ID of the DHCP configuration.
* @param workloadNetworkDhcp The resource properties to be updated.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX DHCP.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDhcpInner updateDhcp(String resourceGroupName, String privateCloudName, String dhcpId,
WorkloadNetworkDhcpInner workloadNetworkDhcp, Context context);
/**
* Delete a WorkloadNetworkDhcp.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dhcpId The ID of the DHCP configuration.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteDhcp(String resourceGroupName, String privateCloudName,
String dhcpId);
/**
* Delete a WorkloadNetworkDhcp.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dhcpId The ID of the DHCP configuration.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteDhcp(String resourceGroupName, String privateCloudName, String dhcpId,
Context context);
/**
* Delete a WorkloadNetworkDhcp.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dhcpId The ID of the DHCP configuration.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteDhcp(String resourceGroupName, String privateCloudName, String dhcpId);
/**
* Delete a WorkloadNetworkDhcp.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dhcpId The ID of the DHCP configuration.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteDhcp(String resourceGroupName, String privateCloudName, String dhcpId, Context context);
/**
* List WorkloadNetworkDnsService resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkDnsService list operation as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listDnsServices(String resourceGroupName, String privateCloudName);
/**
* List WorkloadNetworkDnsService resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkDnsService list operation as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listDnsServices(String resourceGroupName, String privateCloudName,
Context context);
/**
* Get a WorkloadNetworkDnsService.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsServiceId ID of the DNS service.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkDnsService along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getDnsServiceWithResponse(String resourceGroupName,
String privateCloudName, String dnsServiceId, Context context);
/**
* Get a WorkloadNetworkDnsService.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsServiceId ID of the DNS service.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkDnsService.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDnsServiceInner getDnsService(String resourceGroupName, String privateCloudName,
String dnsServiceId);
/**
* Create a WorkloadNetworkDnsService.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsServiceId ID of the DNS service.
* @param workloadNetworkDnsService Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX DNS Service.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkDnsServiceInner> beginCreateDnsService(
String resourceGroupName, String privateCloudName, String dnsServiceId,
WorkloadNetworkDnsServiceInner workloadNetworkDnsService);
/**
* Create a WorkloadNetworkDnsService.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsServiceId ID of the DNS service.
* @param workloadNetworkDnsService Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX DNS Service.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkDnsServiceInner> beginCreateDnsService(
String resourceGroupName, String privateCloudName, String dnsServiceId,
WorkloadNetworkDnsServiceInner workloadNetworkDnsService, Context context);
/**
* Create a WorkloadNetworkDnsService.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsServiceId ID of the DNS service.
* @param workloadNetworkDnsService Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX DNS Service.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDnsServiceInner createDnsService(String resourceGroupName, String privateCloudName,
String dnsServiceId, WorkloadNetworkDnsServiceInner workloadNetworkDnsService);
/**
* Create a WorkloadNetworkDnsService.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsServiceId ID of the DNS service.
* @param workloadNetworkDnsService Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX DNS Service.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDnsServiceInner createDnsService(String resourceGroupName, String privateCloudName,
String dnsServiceId, WorkloadNetworkDnsServiceInner workloadNetworkDnsService, Context context);
/**
* Update a WorkloadNetworkDnsService.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsServiceId ID of the DNS service.
* @param workloadNetworkDnsService The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX DNS Service.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkDnsServiceInner> beginUpdateDnsService(
String resourceGroupName, String privateCloudName, String dnsServiceId,
WorkloadNetworkDnsServiceInner workloadNetworkDnsService);
/**
* Update a WorkloadNetworkDnsService.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsServiceId ID of the DNS service.
* @param workloadNetworkDnsService The resource properties to be updated.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX DNS Service.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkDnsServiceInner> beginUpdateDnsService(
String resourceGroupName, String privateCloudName, String dnsServiceId,
WorkloadNetworkDnsServiceInner workloadNetworkDnsService, Context context);
/**
* Update a WorkloadNetworkDnsService.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsServiceId ID of the DNS service.
* @param workloadNetworkDnsService The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX DNS Service.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDnsServiceInner updateDnsService(String resourceGroupName, String privateCloudName,
String dnsServiceId, WorkloadNetworkDnsServiceInner workloadNetworkDnsService);
/**
* Update a WorkloadNetworkDnsService.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsServiceId ID of the DNS service.
* @param workloadNetworkDnsService The resource properties to be updated.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX DNS Service.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDnsServiceInner updateDnsService(String resourceGroupName, String privateCloudName,
String dnsServiceId, WorkloadNetworkDnsServiceInner workloadNetworkDnsService, Context context);
/**
* Delete a WorkloadNetworkDnsService.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param dnsServiceId ID of the DNS service.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteDnsService(String resourceGroupName, String dnsServiceId,
String privateCloudName);
/**
* Delete a WorkloadNetworkDnsService.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param dnsServiceId ID of the DNS service.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteDnsService(String resourceGroupName, String dnsServiceId,
String privateCloudName, Context context);
/**
* Delete a WorkloadNetworkDnsService.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param dnsServiceId ID of the DNS service.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteDnsService(String resourceGroupName, String dnsServiceId, String privateCloudName);
/**
* Delete a WorkloadNetworkDnsService.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param dnsServiceId ID of the DNS service.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteDnsService(String resourceGroupName, String dnsServiceId, String privateCloudName, Context context);
/**
* List WorkloadNetworkDnsZone resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkDnsZone list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listDnsZones(String resourceGroupName, String privateCloudName);
/**
* List WorkloadNetworkDnsZone resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkDnsZone list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listDnsZones(String resourceGroupName, String privateCloudName,
Context context);
/**
* Get a WorkloadNetworkDnsZone.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsZoneId ID of the DNS zone.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkDnsZone along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getDnsZoneWithResponse(String resourceGroupName, String privateCloudName,
String dnsZoneId, Context context);
/**
* Get a WorkloadNetworkDnsZone.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsZoneId ID of the DNS zone.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkDnsZone.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDnsZoneInner getDnsZone(String resourceGroupName, String privateCloudName, String dnsZoneId);
/**
* Create a WorkloadNetworkDnsZone.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsZoneId ID of the DNS zone.
* @param workloadNetworkDnsZone Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX DNS Zone.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkDnsZoneInner> beginCreateDnsZone(
String resourceGroupName, String privateCloudName, String dnsZoneId,
WorkloadNetworkDnsZoneInner workloadNetworkDnsZone);
/**
* Create a WorkloadNetworkDnsZone.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsZoneId ID of the DNS zone.
* @param workloadNetworkDnsZone Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX DNS Zone.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkDnsZoneInner> beginCreateDnsZone(
String resourceGroupName, String privateCloudName, String dnsZoneId,
WorkloadNetworkDnsZoneInner workloadNetworkDnsZone, Context context);
/**
* Create a WorkloadNetworkDnsZone.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsZoneId ID of the DNS zone.
* @param workloadNetworkDnsZone Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX DNS Zone.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDnsZoneInner createDnsZone(String resourceGroupName, String privateCloudName, String dnsZoneId,
WorkloadNetworkDnsZoneInner workloadNetworkDnsZone);
/**
* Create a WorkloadNetworkDnsZone.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsZoneId ID of the DNS zone.
* @param workloadNetworkDnsZone Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX DNS Zone.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDnsZoneInner createDnsZone(String resourceGroupName, String privateCloudName, String dnsZoneId,
WorkloadNetworkDnsZoneInner workloadNetworkDnsZone, Context context);
/**
* Update a WorkloadNetworkDnsZone.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsZoneId ID of the DNS zone.
* @param workloadNetworkDnsZone The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX DNS Zone.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkDnsZoneInner> beginUpdateDnsZone(
String resourceGroupName, String privateCloudName, String dnsZoneId,
WorkloadNetworkDnsZoneInner workloadNetworkDnsZone);
/**
* Update a WorkloadNetworkDnsZone.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsZoneId ID of the DNS zone.
* @param workloadNetworkDnsZone The resource properties to be updated.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX DNS Zone.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkDnsZoneInner> beginUpdateDnsZone(
String resourceGroupName, String privateCloudName, String dnsZoneId,
WorkloadNetworkDnsZoneInner workloadNetworkDnsZone, Context context);
/**
* Update a WorkloadNetworkDnsZone.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsZoneId ID of the DNS zone.
* @param workloadNetworkDnsZone The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX DNS Zone.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDnsZoneInner updateDnsZone(String resourceGroupName, String privateCloudName, String dnsZoneId,
WorkloadNetworkDnsZoneInner workloadNetworkDnsZone);
/**
* Update a WorkloadNetworkDnsZone.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param dnsZoneId ID of the DNS zone.
* @param workloadNetworkDnsZone The resource properties to be updated.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX DNS Zone.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkDnsZoneInner updateDnsZone(String resourceGroupName, String privateCloudName, String dnsZoneId,
WorkloadNetworkDnsZoneInner workloadNetworkDnsZone, Context context);
/**
* Delete a WorkloadNetworkDnsZone.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param dnsZoneId ID of the DNS zone.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteDnsZone(String resourceGroupName, String dnsZoneId,
String privateCloudName);
/**
* Delete a WorkloadNetworkDnsZone.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param dnsZoneId ID of the DNS zone.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteDnsZone(String resourceGroupName, String dnsZoneId,
String privateCloudName, Context context);
/**
* Delete a WorkloadNetworkDnsZone.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param dnsZoneId ID of the DNS zone.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteDnsZone(String resourceGroupName, String dnsZoneId, String privateCloudName);
/**
* Delete a WorkloadNetworkDnsZone.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param dnsZoneId ID of the DNS zone.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteDnsZone(String resourceGroupName, String dnsZoneId, String privateCloudName, Context context);
/**
* List WorkloadNetworkGateway resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkGateway list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listGateways(String resourceGroupName, String privateCloudName);
/**
* List WorkloadNetworkGateway resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkGateway list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listGateways(String resourceGroupName, String privateCloudName,
Context context);
/**
* Get a WorkloadNetworkGateway.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param gatewayId The ID of the NSX Gateway.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkGateway along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getGatewayWithResponse(String resourceGroupName, String privateCloudName,
String gatewayId, Context context);
/**
* Get a WorkloadNetworkGateway.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param gatewayId The ID of the NSX Gateway.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkGateway.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkGatewayInner getGateway(String resourceGroupName, String privateCloudName, String gatewayId);
/**
* List WorkloadNetworkPortMirroring resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkPortMirroring list operation as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listPortMirroring(String resourceGroupName,
String privateCloudName);
/**
* List WorkloadNetworkPortMirroring resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkPortMirroring list operation as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listPortMirroring(String resourceGroupName,
String privateCloudName, Context context);
/**
* Get a WorkloadNetworkPortMirroring.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param portMirroringId ID of the NSX port mirroring profile.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkPortMirroring along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getPortMirroringWithResponse(String resourceGroupName,
String privateCloudName, String portMirroringId, Context context);
/**
* Get a WorkloadNetworkPortMirroring.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param portMirroringId ID of the NSX port mirroring profile.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkPortMirroring.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkPortMirroringInner getPortMirroring(String resourceGroupName, String privateCloudName,
String portMirroringId);
/**
* Create a WorkloadNetworkPortMirroring.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param portMirroringId ID of the NSX port mirroring profile.
* @param workloadNetworkPortMirroring Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX Port Mirroring.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkPortMirroringInner>
beginCreatePortMirroring(String resourceGroupName, String privateCloudName, String portMirroringId,
WorkloadNetworkPortMirroringInner workloadNetworkPortMirroring);
/**
* Create a WorkloadNetworkPortMirroring.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param portMirroringId ID of the NSX port mirroring profile.
* @param workloadNetworkPortMirroring Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX Port Mirroring.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkPortMirroringInner>
beginCreatePortMirroring(String resourceGroupName, String privateCloudName, String portMirroringId,
WorkloadNetworkPortMirroringInner workloadNetworkPortMirroring, Context context);
/**
* Create a WorkloadNetworkPortMirroring.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param portMirroringId ID of the NSX port mirroring profile.
* @param workloadNetworkPortMirroring Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX Port Mirroring.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkPortMirroringInner createPortMirroring(String resourceGroupName, String privateCloudName,
String portMirroringId, WorkloadNetworkPortMirroringInner workloadNetworkPortMirroring);
/**
* Create a WorkloadNetworkPortMirroring.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param portMirroringId ID of the NSX port mirroring profile.
* @param workloadNetworkPortMirroring Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX Port Mirroring.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkPortMirroringInner createPortMirroring(String resourceGroupName, String privateCloudName,
String portMirroringId, WorkloadNetworkPortMirroringInner workloadNetworkPortMirroring, Context context);
/**
* Update a WorkloadNetworkPortMirroring.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param portMirroringId ID of the NSX port mirroring profile.
* @param workloadNetworkPortMirroring The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX Port Mirroring.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkPortMirroringInner>
beginUpdatePortMirroring(String resourceGroupName, String privateCloudName, String portMirroringId,
WorkloadNetworkPortMirroringInner workloadNetworkPortMirroring);
/**
* Update a WorkloadNetworkPortMirroring.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param portMirroringId ID of the NSX port mirroring profile.
* @param workloadNetworkPortMirroring The resource properties to be updated.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX Port Mirroring.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkPortMirroringInner>
beginUpdatePortMirroring(String resourceGroupName, String privateCloudName, String portMirroringId,
WorkloadNetworkPortMirroringInner workloadNetworkPortMirroring, Context context);
/**
* Update a WorkloadNetworkPortMirroring.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param portMirroringId ID of the NSX port mirroring profile.
* @param workloadNetworkPortMirroring The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX Port Mirroring.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkPortMirroringInner updatePortMirroring(String resourceGroupName, String privateCloudName,
String portMirroringId, WorkloadNetworkPortMirroringInner workloadNetworkPortMirroring);
/**
* Update a WorkloadNetworkPortMirroring.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param portMirroringId ID of the NSX port mirroring profile.
* @param workloadNetworkPortMirroring The resource properties to be updated.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX Port Mirroring.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkPortMirroringInner updatePortMirroring(String resourceGroupName, String privateCloudName,
String portMirroringId, WorkloadNetworkPortMirroringInner workloadNetworkPortMirroring, Context context);
/**
* Delete a WorkloadNetworkPortMirroring.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param portMirroringId ID of the NSX port mirroring profile.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeletePortMirroring(String resourceGroupName, String portMirroringId,
String privateCloudName);
/**
* Delete a WorkloadNetworkPortMirroring.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param portMirroringId ID of the NSX port mirroring profile.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeletePortMirroring(String resourceGroupName, String portMirroringId,
String privateCloudName, Context context);
/**
* Delete a WorkloadNetworkPortMirroring.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param portMirroringId ID of the NSX port mirroring profile.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deletePortMirroring(String resourceGroupName, String portMirroringId, String privateCloudName);
/**
* Delete a WorkloadNetworkPortMirroring.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param portMirroringId ID of the NSX port mirroring profile.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deletePortMirroring(String resourceGroupName, String portMirroringId, String privateCloudName,
Context context);
/**
* List WorkloadNetworkPublicIP resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkPublicIP list operation as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listPublicIPs(String resourceGroupName, String privateCloudName);
/**
* List WorkloadNetworkPublicIP resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkPublicIP list operation as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listPublicIPs(String resourceGroupName, String privateCloudName,
Context context);
/**
* Get a WorkloadNetworkPublicIP.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param publicIpId ID of the DNS zone.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkPublicIP along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getPublicIpWithResponse(String resourceGroupName, String privateCloudName,
String publicIpId, Context context);
/**
* Get a WorkloadNetworkPublicIP.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param publicIpId ID of the DNS zone.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkPublicIP.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkPublicIpInner getPublicIp(String resourceGroupName, String privateCloudName, String publicIpId);
/**
* Create a WorkloadNetworkPublicIP.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param publicIpId ID of the DNS zone.
* @param workloadNetworkPublicIp Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX Public IP Block.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkPublicIpInner> beginCreatePublicIp(
String resourceGroupName, String privateCloudName, String publicIpId,
WorkloadNetworkPublicIpInner workloadNetworkPublicIp);
/**
* Create a WorkloadNetworkPublicIP.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param publicIpId ID of the DNS zone.
* @param workloadNetworkPublicIp Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX Public IP Block.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkPublicIpInner> beginCreatePublicIp(
String resourceGroupName, String privateCloudName, String publicIpId,
WorkloadNetworkPublicIpInner workloadNetworkPublicIp, Context context);
/**
* Create a WorkloadNetworkPublicIP.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param publicIpId ID of the DNS zone.
* @param workloadNetworkPublicIp Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX Public IP Block.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkPublicIpInner createPublicIp(String resourceGroupName, String privateCloudName, String publicIpId,
WorkloadNetworkPublicIpInner workloadNetworkPublicIp);
/**
* Create a WorkloadNetworkPublicIP.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param publicIpId ID of the DNS zone.
* @param workloadNetworkPublicIp Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX Public IP Block.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkPublicIpInner createPublicIp(String resourceGroupName, String privateCloudName, String publicIpId,
WorkloadNetworkPublicIpInner workloadNetworkPublicIp, Context context);
/**
* Delete a WorkloadNetworkPublicIP.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param publicIpId ID of the DNS zone.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeletePublicIp(String resourceGroupName, String publicIpId,
String privateCloudName);
/**
* Delete a WorkloadNetworkPublicIP.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param publicIpId ID of the DNS zone.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeletePublicIp(String resourceGroupName, String publicIpId,
String privateCloudName, Context context);
/**
* Delete a WorkloadNetworkPublicIP.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param publicIpId ID of the DNS zone.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deletePublicIp(String resourceGroupName, String publicIpId, String privateCloudName);
/**
* Delete a WorkloadNetworkPublicIP.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param publicIpId ID of the DNS zone.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deletePublicIp(String resourceGroupName, String publicIpId, String privateCloudName, Context context);
/**
* List WorkloadNetworkSegment resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkSegment list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSegments(String resourceGroupName, String privateCloudName);
/**
* List WorkloadNetworkSegment resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkSegment list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSegments(String resourceGroupName, String privateCloudName,
Context context);
/**
* Get a WorkloadNetworkSegment.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param segmentId The ID of the NSX Segment.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkSegment along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getSegmentWithResponse(String resourceGroupName, String privateCloudName,
String segmentId, Context context);
/**
* Get a WorkloadNetworkSegment.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param segmentId The ID of the NSX Segment.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkSegment.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkSegmentInner getSegment(String resourceGroupName, String privateCloudName, String segmentId);
/**
* Create a WorkloadNetworkSegment.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param segmentId The ID of the NSX Segment.
* @param workloadNetworkSegment Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX Segment.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkSegmentInner> beginCreateSegments(
String resourceGroupName, String privateCloudName, String segmentId,
WorkloadNetworkSegmentInner workloadNetworkSegment);
/**
* Create a WorkloadNetworkSegment.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param segmentId The ID of the NSX Segment.
* @param workloadNetworkSegment Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX Segment.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkSegmentInner> beginCreateSegments(
String resourceGroupName, String privateCloudName, String segmentId,
WorkloadNetworkSegmentInner workloadNetworkSegment, Context context);
/**
* Create a WorkloadNetworkSegment.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param segmentId The ID of the NSX Segment.
* @param workloadNetworkSegment Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX Segment.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkSegmentInner createSegments(String resourceGroupName, String privateCloudName, String segmentId,
WorkloadNetworkSegmentInner workloadNetworkSegment);
/**
* Create a WorkloadNetworkSegment.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param segmentId The ID of the NSX Segment.
* @param workloadNetworkSegment Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX Segment.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkSegmentInner createSegments(String resourceGroupName, String privateCloudName, String segmentId,
WorkloadNetworkSegmentInner workloadNetworkSegment, Context context);
/**
* Update a WorkloadNetworkSegment.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param segmentId The ID of the NSX Segment.
* @param workloadNetworkSegment The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX Segment.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkSegmentInner> beginUpdateSegments(
String resourceGroupName, String privateCloudName, String segmentId,
WorkloadNetworkSegmentInner workloadNetworkSegment);
/**
* Update a WorkloadNetworkSegment.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param segmentId The ID of the NSX Segment.
* @param workloadNetworkSegment The resource properties to be updated.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX Segment.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkSegmentInner> beginUpdateSegments(
String resourceGroupName, String privateCloudName, String segmentId,
WorkloadNetworkSegmentInner workloadNetworkSegment, Context context);
/**
* Update a WorkloadNetworkSegment.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param segmentId The ID of the NSX Segment.
* @param workloadNetworkSegment The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX Segment.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkSegmentInner updateSegments(String resourceGroupName, String privateCloudName, String segmentId,
WorkloadNetworkSegmentInner workloadNetworkSegment);
/**
* Update a WorkloadNetworkSegment.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param segmentId The ID of the NSX Segment.
* @param workloadNetworkSegment The resource properties to be updated.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX Segment.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkSegmentInner updateSegments(String resourceGroupName, String privateCloudName, String segmentId,
WorkloadNetworkSegmentInner workloadNetworkSegment, Context context);
/**
* Delete a WorkloadNetworkSegment.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param segmentId The ID of the NSX Segment.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSegment(String resourceGroupName, String privateCloudName,
String segmentId);
/**
* Delete a WorkloadNetworkSegment.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param segmentId The ID of the NSX Segment.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSegment(String resourceGroupName, String privateCloudName,
String segmentId, Context context);
/**
* Delete a WorkloadNetworkSegment.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param segmentId The ID of the NSX Segment.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSegment(String resourceGroupName, String privateCloudName, String segmentId);
/**
* Delete a WorkloadNetworkSegment.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param segmentId The ID of the NSX Segment.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSegment(String resourceGroupName, String privateCloudName, String segmentId, Context context);
/**
* List WorkloadNetworkVirtualMachine resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkVirtualMachine list operation as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listVirtualMachines(String resourceGroupName,
String privateCloudName);
/**
* List WorkloadNetworkVirtualMachine resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkVirtualMachine list operation as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listVirtualMachines(String resourceGroupName,
String privateCloudName, Context context);
/**
* Get a WorkloadNetworkVirtualMachine.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param virtualMachineId ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkVirtualMachine along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getVirtualMachineWithResponse(String resourceGroupName,
String privateCloudName, String virtualMachineId, Context context);
/**
* Get a WorkloadNetworkVirtualMachine.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param virtualMachineId ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkVirtualMachine.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkVirtualMachineInner getVirtualMachine(String resourceGroupName, String privateCloudName,
String virtualMachineId);
/**
* List WorkloadNetworkVMGroup resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkVMGroup list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listVMGroups(String resourceGroupName, String privateCloudName);
/**
* List WorkloadNetworkVMGroup resources by WorkloadNetwork.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response of a WorkloadNetworkVMGroup list operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listVMGroups(String resourceGroupName, String privateCloudName,
Context context);
/**
* Get a WorkloadNetworkVMGroup.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param vmGroupId ID of the VM group.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkVMGroup along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getVMGroupWithResponse(String resourceGroupName, String privateCloudName,
String vmGroupId, Context context);
/**
* Get a WorkloadNetworkVMGroup.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param vmGroupId ID of the VM group.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a WorkloadNetworkVMGroup.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkVMGroupInner getVMGroup(String resourceGroupName, String privateCloudName, String vmGroupId);
/**
* Create a WorkloadNetworkVMGroup.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param vmGroupId ID of the VM group.
* @param workloadNetworkVMGroup Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX VM Group.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkVMGroupInner> beginCreateVMGroup(
String resourceGroupName, String privateCloudName, String vmGroupId,
WorkloadNetworkVMGroupInner workloadNetworkVMGroup);
/**
* Create a WorkloadNetworkVMGroup.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param vmGroupId ID of the VM group.
* @param workloadNetworkVMGroup Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX VM Group.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkVMGroupInner> beginCreateVMGroup(
String resourceGroupName, String privateCloudName, String vmGroupId,
WorkloadNetworkVMGroupInner workloadNetworkVMGroup, Context context);
/**
* Create a WorkloadNetworkVMGroup.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param vmGroupId ID of the VM group.
* @param workloadNetworkVMGroup Resource create parameters.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX VM Group.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkVMGroupInner createVMGroup(String resourceGroupName, String privateCloudName, String vmGroupId,
WorkloadNetworkVMGroupInner workloadNetworkVMGroup);
/**
* Create a WorkloadNetworkVMGroup.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param vmGroupId ID of the VM group.
* @param workloadNetworkVMGroup Resource create parameters.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX VM Group.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkVMGroupInner createVMGroup(String resourceGroupName, String privateCloudName, String vmGroupId,
WorkloadNetworkVMGroupInner workloadNetworkVMGroup, Context context);
/**
* Update a WorkloadNetworkVMGroup.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param vmGroupId ID of the VM group.
* @param workloadNetworkVMGroup The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX VM Group.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkVMGroupInner> beginUpdateVMGroup(
String resourceGroupName, String privateCloudName, String vmGroupId,
WorkloadNetworkVMGroupInner workloadNetworkVMGroup);
/**
* Update a WorkloadNetworkVMGroup.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param vmGroupId ID of the VM group.
* @param workloadNetworkVMGroup The resource properties to be updated.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of nSX VM Group.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, WorkloadNetworkVMGroupInner> beginUpdateVMGroup(
String resourceGroupName, String privateCloudName, String vmGroupId,
WorkloadNetworkVMGroupInner workloadNetworkVMGroup, Context context);
/**
* Update a WorkloadNetworkVMGroup.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param vmGroupId ID of the VM group.
* @param workloadNetworkVMGroup The resource properties to be updated.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX VM Group.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkVMGroupInner updateVMGroup(String resourceGroupName, String privateCloudName, String vmGroupId,
WorkloadNetworkVMGroupInner workloadNetworkVMGroup);
/**
* Update a WorkloadNetworkVMGroup.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @param vmGroupId ID of the VM group.
* @param workloadNetworkVMGroup The resource properties to be updated.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return nSX VM Group.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
WorkloadNetworkVMGroupInner updateVMGroup(String resourceGroupName, String privateCloudName, String vmGroupId,
WorkloadNetworkVMGroupInner workloadNetworkVMGroup, Context context);
/**
* Delete a WorkloadNetworkVMGroup.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param vmGroupId ID of the VM group.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteVMGroup(String resourceGroupName, String vmGroupId,
String privateCloudName);
/**
* Delete a WorkloadNetworkVMGroup.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param vmGroupId ID of the VM group.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteVMGroup(String resourceGroupName, String vmGroupId,
String privateCloudName, Context context);
/**
* Delete a WorkloadNetworkVMGroup.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param vmGroupId ID of the VM group.
* @param privateCloudName Name of the private cloud.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteVMGroup(String resourceGroupName, String vmGroupId, String privateCloudName);
/**
* Delete a WorkloadNetworkVMGroup.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param vmGroupId ID of the VM group.
* @param privateCloudName Name of the private cloud.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteVMGroup(String resourceGroupName, String vmGroupId, String privateCloudName, Context context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy