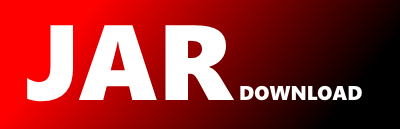
com.azure.resourcemanager.avs.implementation.WorkloadNetworksImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-avs Show documentation
Show all versions of azure-resourcemanager-avs Show documentation
This package contains Microsoft Azure SDK for Avs Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Azure VMware Solution API. Package tag package-2023-09-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.avs.implementation;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.SimpleResponse;
import com.azure.core.util.Context;
import com.azure.core.util.logging.ClientLogger;
import com.azure.resourcemanager.avs.fluent.WorkloadNetworksClient;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkDhcpInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkDnsServiceInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkDnsZoneInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkGatewayInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkPortMirroringInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkPublicIpInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkSegmentInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkVirtualMachineInner;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkVMGroupInner;
import com.azure.resourcemanager.avs.models.WorkloadNetwork;
import com.azure.resourcemanager.avs.models.WorkloadNetworkDhcp;
import com.azure.resourcemanager.avs.models.WorkloadNetworkDnsService;
import com.azure.resourcemanager.avs.models.WorkloadNetworkDnsZone;
import com.azure.resourcemanager.avs.models.WorkloadNetworkGateway;
import com.azure.resourcemanager.avs.models.WorkloadNetworkPortMirroring;
import com.azure.resourcemanager.avs.models.WorkloadNetworkPublicIp;
import com.azure.resourcemanager.avs.models.WorkloadNetworks;
import com.azure.resourcemanager.avs.models.WorkloadNetworkSegment;
import com.azure.resourcemanager.avs.models.WorkloadNetworkVirtualMachine;
import com.azure.resourcemanager.avs.models.WorkloadNetworkVMGroup;
public final class WorkloadNetworksImpl implements WorkloadNetworks {
private static final ClientLogger LOGGER = new ClientLogger(WorkloadNetworksImpl.class);
private final WorkloadNetworksClient innerClient;
private final com.azure.resourcemanager.avs.AvsManager serviceManager;
public WorkloadNetworksImpl(WorkloadNetworksClient innerClient,
com.azure.resourcemanager.avs.AvsManager serviceManager) {
this.innerClient = innerClient;
this.serviceManager = serviceManager;
}
public PagedIterable list(String resourceGroupName, String privateCloudName) {
PagedIterable inner = this.serviceClient().list(resourceGroupName, privateCloudName);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkImpl(inner1, this.manager()));
}
public PagedIterable list(String resourceGroupName, String privateCloudName, Context context) {
PagedIterable inner
= this.serviceClient().list(resourceGroupName, privateCloudName, context);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkImpl(inner1, this.manager()));
}
public Response getWithResponse(String resourceGroupName, String privateCloudName,
Context context) {
Response inner
= this.serviceClient().getWithResponse(resourceGroupName, privateCloudName, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new WorkloadNetworkImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public WorkloadNetwork get(String resourceGroupName, String privateCloudName) {
WorkloadNetworkInner inner = this.serviceClient().get(resourceGroupName, privateCloudName);
if (inner != null) {
return new WorkloadNetworkImpl(inner, this.manager());
} else {
return null;
}
}
public PagedIterable listDhcp(String resourceGroupName, String privateCloudName) {
PagedIterable inner
= this.serviceClient().listDhcp(resourceGroupName, privateCloudName);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkDhcpImpl(inner1, this.manager()));
}
public PagedIterable listDhcp(String resourceGroupName, String privateCloudName,
Context context) {
PagedIterable inner
= this.serviceClient().listDhcp(resourceGroupName, privateCloudName, context);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkDhcpImpl(inner1, this.manager()));
}
public Response getDhcpWithResponse(String resourceGroupName, String dhcpId,
String privateCloudName, Context context) {
Response inner
= this.serviceClient().getDhcpWithResponse(resourceGroupName, dhcpId, privateCloudName, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new WorkloadNetworkDhcpImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public WorkloadNetworkDhcp getDhcp(String resourceGroupName, String dhcpId, String privateCloudName) {
WorkloadNetworkDhcpInner inner = this.serviceClient().getDhcp(resourceGroupName, dhcpId, privateCloudName);
if (inner != null) {
return new WorkloadNetworkDhcpImpl(inner, this.manager());
} else {
return null;
}
}
public void deleteDhcp(String resourceGroupName, String privateCloudName, String dhcpId) {
this.serviceClient().deleteDhcp(resourceGroupName, privateCloudName, dhcpId);
}
public void deleteDhcp(String resourceGroupName, String privateCloudName, String dhcpId, Context context) {
this.serviceClient().deleteDhcp(resourceGroupName, privateCloudName, dhcpId, context);
}
public PagedIterable listDnsServices(String resourceGroupName, String privateCloudName) {
PagedIterable inner
= this.serviceClient().listDnsServices(resourceGroupName, privateCloudName);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkDnsServiceImpl(inner1, this.manager()));
}
public PagedIterable listDnsServices(String resourceGroupName, String privateCloudName,
Context context) {
PagedIterable inner
= this.serviceClient().listDnsServices(resourceGroupName, privateCloudName, context);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkDnsServiceImpl(inner1, this.manager()));
}
public Response getDnsServiceWithResponse(String resourceGroupName,
String privateCloudName, String dnsServiceId, Context context) {
Response inner = this.serviceClient()
.getDnsServiceWithResponse(resourceGroupName, privateCloudName, dnsServiceId, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new WorkloadNetworkDnsServiceImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public WorkloadNetworkDnsService getDnsService(String resourceGroupName, String privateCloudName,
String dnsServiceId) {
WorkloadNetworkDnsServiceInner inner
= this.serviceClient().getDnsService(resourceGroupName, privateCloudName, dnsServiceId);
if (inner != null) {
return new WorkloadNetworkDnsServiceImpl(inner, this.manager());
} else {
return null;
}
}
public void deleteDnsService(String resourceGroupName, String dnsServiceId, String privateCloudName) {
this.serviceClient().deleteDnsService(resourceGroupName, dnsServiceId, privateCloudName);
}
public void deleteDnsService(String resourceGroupName, String dnsServiceId, String privateCloudName,
Context context) {
this.serviceClient().deleteDnsService(resourceGroupName, dnsServiceId, privateCloudName, context);
}
public PagedIterable listDnsZones(String resourceGroupName, String privateCloudName) {
PagedIterable inner
= this.serviceClient().listDnsZones(resourceGroupName, privateCloudName);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkDnsZoneImpl(inner1, this.manager()));
}
public PagedIterable listDnsZones(String resourceGroupName, String privateCloudName,
Context context) {
PagedIterable inner
= this.serviceClient().listDnsZones(resourceGroupName, privateCloudName, context);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkDnsZoneImpl(inner1, this.manager()));
}
public Response getDnsZoneWithResponse(String resourceGroupName, String privateCloudName,
String dnsZoneId, Context context) {
Response inner
= this.serviceClient().getDnsZoneWithResponse(resourceGroupName, privateCloudName, dnsZoneId, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new WorkloadNetworkDnsZoneImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public WorkloadNetworkDnsZone getDnsZone(String resourceGroupName, String privateCloudName, String dnsZoneId) {
WorkloadNetworkDnsZoneInner inner
= this.serviceClient().getDnsZone(resourceGroupName, privateCloudName, dnsZoneId);
if (inner != null) {
return new WorkloadNetworkDnsZoneImpl(inner, this.manager());
} else {
return null;
}
}
public void deleteDnsZone(String resourceGroupName, String dnsZoneId, String privateCloudName) {
this.serviceClient().deleteDnsZone(resourceGroupName, dnsZoneId, privateCloudName);
}
public void deleteDnsZone(String resourceGroupName, String dnsZoneId, String privateCloudName, Context context) {
this.serviceClient().deleteDnsZone(resourceGroupName, dnsZoneId, privateCloudName, context);
}
public PagedIterable listGateways(String resourceGroupName, String privateCloudName) {
PagedIterable inner
= this.serviceClient().listGateways(resourceGroupName, privateCloudName);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkGatewayImpl(inner1, this.manager()));
}
public PagedIterable listGateways(String resourceGroupName, String privateCloudName,
Context context) {
PagedIterable inner
= this.serviceClient().listGateways(resourceGroupName, privateCloudName, context);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkGatewayImpl(inner1, this.manager()));
}
public Response getGatewayWithResponse(String resourceGroupName, String privateCloudName,
String gatewayId, Context context) {
Response inner
= this.serviceClient().getGatewayWithResponse(resourceGroupName, privateCloudName, gatewayId, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new WorkloadNetworkGatewayImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public WorkloadNetworkGateway getGateway(String resourceGroupName, String privateCloudName, String gatewayId) {
WorkloadNetworkGatewayInner inner
= this.serviceClient().getGateway(resourceGroupName, privateCloudName, gatewayId);
if (inner != null) {
return new WorkloadNetworkGatewayImpl(inner, this.manager());
} else {
return null;
}
}
public PagedIterable listPortMirroring(String resourceGroupName,
String privateCloudName) {
PagedIterable inner
= this.serviceClient().listPortMirroring(resourceGroupName, privateCloudName);
return ResourceManagerUtils.mapPage(inner,
inner1 -> new WorkloadNetworkPortMirroringImpl(inner1, this.manager()));
}
public PagedIterable listPortMirroring(String resourceGroupName,
String privateCloudName, Context context) {
PagedIterable inner
= this.serviceClient().listPortMirroring(resourceGroupName, privateCloudName, context);
return ResourceManagerUtils.mapPage(inner,
inner1 -> new WorkloadNetworkPortMirroringImpl(inner1, this.manager()));
}
public Response getPortMirroringWithResponse(String resourceGroupName,
String privateCloudName, String portMirroringId, Context context) {
Response inner = this.serviceClient()
.getPortMirroringWithResponse(resourceGroupName, privateCloudName, portMirroringId, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new WorkloadNetworkPortMirroringImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public WorkloadNetworkPortMirroring getPortMirroring(String resourceGroupName, String privateCloudName,
String portMirroringId) {
WorkloadNetworkPortMirroringInner inner
= this.serviceClient().getPortMirroring(resourceGroupName, privateCloudName, portMirroringId);
if (inner != null) {
return new WorkloadNetworkPortMirroringImpl(inner, this.manager());
} else {
return null;
}
}
public void deletePortMirroring(String resourceGroupName, String portMirroringId, String privateCloudName) {
this.serviceClient().deletePortMirroring(resourceGroupName, portMirroringId, privateCloudName);
}
public void deletePortMirroring(String resourceGroupName, String portMirroringId, String privateCloudName,
Context context) {
this.serviceClient().deletePortMirroring(resourceGroupName, portMirroringId, privateCloudName, context);
}
public PagedIterable listPublicIPs(String resourceGroupName, String privateCloudName) {
PagedIterable inner
= this.serviceClient().listPublicIPs(resourceGroupName, privateCloudName);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkPublicIpImpl(inner1, this.manager()));
}
public PagedIterable listPublicIPs(String resourceGroupName, String privateCloudName,
Context context) {
PagedIterable inner
= this.serviceClient().listPublicIPs(resourceGroupName, privateCloudName, context);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkPublicIpImpl(inner1, this.manager()));
}
public Response getPublicIpWithResponse(String resourceGroupName, String privateCloudName,
String publicIpId, Context context) {
Response inner
= this.serviceClient().getPublicIpWithResponse(resourceGroupName, privateCloudName, publicIpId, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new WorkloadNetworkPublicIpImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public WorkloadNetworkPublicIp getPublicIp(String resourceGroupName, String privateCloudName, String publicIpId) {
WorkloadNetworkPublicIpInner inner
= this.serviceClient().getPublicIp(resourceGroupName, privateCloudName, publicIpId);
if (inner != null) {
return new WorkloadNetworkPublicIpImpl(inner, this.manager());
} else {
return null;
}
}
public void deletePublicIp(String resourceGroupName, String publicIpId, String privateCloudName) {
this.serviceClient().deletePublicIp(resourceGroupName, publicIpId, privateCloudName);
}
public void deletePublicIp(String resourceGroupName, String publicIpId, String privateCloudName, Context context) {
this.serviceClient().deletePublicIp(resourceGroupName, publicIpId, privateCloudName, context);
}
public PagedIterable listSegments(String resourceGroupName, String privateCloudName) {
PagedIterable inner
= this.serviceClient().listSegments(resourceGroupName, privateCloudName);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkSegmentImpl(inner1, this.manager()));
}
public PagedIterable listSegments(String resourceGroupName, String privateCloudName,
Context context) {
PagedIterable inner
= this.serviceClient().listSegments(resourceGroupName, privateCloudName, context);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkSegmentImpl(inner1, this.manager()));
}
public Response getSegmentWithResponse(String resourceGroupName, String privateCloudName,
String segmentId, Context context) {
Response inner
= this.serviceClient().getSegmentWithResponse(resourceGroupName, privateCloudName, segmentId, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new WorkloadNetworkSegmentImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public WorkloadNetworkSegment getSegment(String resourceGroupName, String privateCloudName, String segmentId) {
WorkloadNetworkSegmentInner inner
= this.serviceClient().getSegment(resourceGroupName, privateCloudName, segmentId);
if (inner != null) {
return new WorkloadNetworkSegmentImpl(inner, this.manager());
} else {
return null;
}
}
public void deleteSegment(String resourceGroupName, String privateCloudName, String segmentId) {
this.serviceClient().deleteSegment(resourceGroupName, privateCloudName, segmentId);
}
public void deleteSegment(String resourceGroupName, String privateCloudName, String segmentId, Context context) {
this.serviceClient().deleteSegment(resourceGroupName, privateCloudName, segmentId, context);
}
public PagedIterable listVirtualMachines(String resourceGroupName,
String privateCloudName) {
PagedIterable inner
= this.serviceClient().listVirtualMachines(resourceGroupName, privateCloudName);
return ResourceManagerUtils.mapPage(inner,
inner1 -> new WorkloadNetworkVirtualMachineImpl(inner1, this.manager()));
}
public PagedIterable listVirtualMachines(String resourceGroupName,
String privateCloudName, Context context) {
PagedIterable inner
= this.serviceClient().listVirtualMachines(resourceGroupName, privateCloudName, context);
return ResourceManagerUtils.mapPage(inner,
inner1 -> new WorkloadNetworkVirtualMachineImpl(inner1, this.manager()));
}
public Response getVirtualMachineWithResponse(String resourceGroupName,
String privateCloudName, String virtualMachineId, Context context) {
Response inner = this.serviceClient()
.getVirtualMachineWithResponse(resourceGroupName, privateCloudName, virtualMachineId, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new WorkloadNetworkVirtualMachineImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public WorkloadNetworkVirtualMachine getVirtualMachine(String resourceGroupName, String privateCloudName,
String virtualMachineId) {
WorkloadNetworkVirtualMachineInner inner
= this.serviceClient().getVirtualMachine(resourceGroupName, privateCloudName, virtualMachineId);
if (inner != null) {
return new WorkloadNetworkVirtualMachineImpl(inner, this.manager());
} else {
return null;
}
}
public PagedIterable listVMGroups(String resourceGroupName, String privateCloudName) {
PagedIterable inner
= this.serviceClient().listVMGroups(resourceGroupName, privateCloudName);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkVMGroupImpl(inner1, this.manager()));
}
public PagedIterable listVMGroups(String resourceGroupName, String privateCloudName,
Context context) {
PagedIterable inner
= this.serviceClient().listVMGroups(resourceGroupName, privateCloudName, context);
return ResourceManagerUtils.mapPage(inner, inner1 -> new WorkloadNetworkVMGroupImpl(inner1, this.manager()));
}
public Response getVMGroupWithResponse(String resourceGroupName, String privateCloudName,
String vmGroupId, Context context) {
Response inner
= this.serviceClient().getVMGroupWithResponse(resourceGroupName, privateCloudName, vmGroupId, context);
if (inner != null) {
return new SimpleResponse<>(inner.getRequest(), inner.getStatusCode(), inner.getHeaders(),
new WorkloadNetworkVMGroupImpl(inner.getValue(), this.manager()));
} else {
return null;
}
}
public WorkloadNetworkVMGroup getVMGroup(String resourceGroupName, String privateCloudName, String vmGroupId) {
WorkloadNetworkVMGroupInner inner
= this.serviceClient().getVMGroup(resourceGroupName, privateCloudName, vmGroupId);
if (inner != null) {
return new WorkloadNetworkVMGroupImpl(inner, this.manager());
} else {
return null;
}
}
public void deleteVMGroup(String resourceGroupName, String vmGroupId, String privateCloudName) {
this.serviceClient().deleteVMGroup(resourceGroupName, vmGroupId, privateCloudName);
}
public void deleteVMGroup(String resourceGroupName, String vmGroupId, String privateCloudName, Context context) {
this.serviceClient().deleteVMGroup(resourceGroupName, vmGroupId, privateCloudName, context);
}
public WorkloadNetworkDhcp getDhcpById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String dhcpId = ResourceManagerUtils.getValueFromIdByName(id, "dhcpConfigurations");
if (dhcpId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'dhcpConfigurations'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
return this.getDhcpWithResponse(resourceGroupName, dhcpId, privateCloudName, Context.NONE).getValue();
}
public Response getDhcpByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String dhcpId = ResourceManagerUtils.getValueFromIdByName(id, "dhcpConfigurations");
if (dhcpId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'dhcpConfigurations'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
return this.getDhcpWithResponse(resourceGroupName, dhcpId, privateCloudName, context);
}
public WorkloadNetworkDnsService getDnsServiceById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String dnsServiceId = ResourceManagerUtils.getValueFromIdByName(id, "dnsServices");
if (dnsServiceId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'dnsServices'.", id)));
}
return this.getDnsServiceWithResponse(resourceGroupName, privateCloudName, dnsServiceId, Context.NONE)
.getValue();
}
public Response getDnsServiceByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String dnsServiceId = ResourceManagerUtils.getValueFromIdByName(id, "dnsServices");
if (dnsServiceId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'dnsServices'.", id)));
}
return this.getDnsServiceWithResponse(resourceGroupName, privateCloudName, dnsServiceId, context);
}
public WorkloadNetworkDnsZone getDnsZoneById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String dnsZoneId = ResourceManagerUtils.getValueFromIdByName(id, "dnsZones");
if (dnsZoneId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'dnsZones'.", id)));
}
return this.getDnsZoneWithResponse(resourceGroupName, privateCloudName, dnsZoneId, Context.NONE).getValue();
}
public Response getDnsZoneByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String dnsZoneId = ResourceManagerUtils.getValueFromIdByName(id, "dnsZones");
if (dnsZoneId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'dnsZones'.", id)));
}
return this.getDnsZoneWithResponse(resourceGroupName, privateCloudName, dnsZoneId, context);
}
public WorkloadNetworkPortMirroring getPortMirroringById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String portMirroringId = ResourceManagerUtils.getValueFromIdByName(id, "portMirroringProfiles");
if (portMirroringId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'portMirroringProfiles'.", id)));
}
return this.getPortMirroringWithResponse(resourceGroupName, privateCloudName, portMirroringId, Context.NONE)
.getValue();
}
public Response getPortMirroringByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String portMirroringId = ResourceManagerUtils.getValueFromIdByName(id, "portMirroringProfiles");
if (portMirroringId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'portMirroringProfiles'.", id)));
}
return this.getPortMirroringWithResponse(resourceGroupName, privateCloudName, portMirroringId, context);
}
public WorkloadNetworkPublicIp getPublicIpById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String publicIpId = ResourceManagerUtils.getValueFromIdByName(id, "publicIPs");
if (publicIpId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'publicIPs'.", id)));
}
return this.getPublicIpWithResponse(resourceGroupName, privateCloudName, publicIpId, Context.NONE).getValue();
}
public Response getPublicIpByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String publicIpId = ResourceManagerUtils.getValueFromIdByName(id, "publicIPs");
if (publicIpId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'publicIPs'.", id)));
}
return this.getPublicIpWithResponse(resourceGroupName, privateCloudName, publicIpId, context);
}
public WorkloadNetworkSegment getSegmentById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String segmentId = ResourceManagerUtils.getValueFromIdByName(id, "segments");
if (segmentId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'segments'.", id)));
}
return this.getSegmentWithResponse(resourceGroupName, privateCloudName, segmentId, Context.NONE).getValue();
}
public Response getSegmentByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String segmentId = ResourceManagerUtils.getValueFromIdByName(id, "segments");
if (segmentId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'segments'.", id)));
}
return this.getSegmentWithResponse(resourceGroupName, privateCloudName, segmentId, context);
}
public WorkloadNetworkVMGroup getVMGroupById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String vmGroupId = ResourceManagerUtils.getValueFromIdByName(id, "vmGroups");
if (vmGroupId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'vmGroups'.", id)));
}
return this.getVMGroupWithResponse(resourceGroupName, privateCloudName, vmGroupId, Context.NONE).getValue();
}
public Response getVMGroupByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String vmGroupId = ResourceManagerUtils.getValueFromIdByName(id, "vmGroups");
if (vmGroupId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'vmGroups'.", id)));
}
return this.getVMGroupWithResponse(resourceGroupName, privateCloudName, vmGroupId, context);
}
public void deleteDhcpById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String dhcpId = ResourceManagerUtils.getValueFromIdByName(id, "dhcpConfigurations");
if (dhcpId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'dhcpConfigurations'.", id)));
}
this.deleteDhcp(resourceGroupName, privateCloudName, dhcpId, Context.NONE);
}
public void deleteDhcpByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String dhcpId = ResourceManagerUtils.getValueFromIdByName(id, "dhcpConfigurations");
if (dhcpId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'dhcpConfigurations'.", id)));
}
this.deleteDhcp(resourceGroupName, privateCloudName, dhcpId, context);
}
public void deleteDnsServiceById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String dnsServiceId = ResourceManagerUtils.getValueFromIdByName(id, "dnsServices");
if (dnsServiceId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'dnsServices'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
this.deleteDnsService(resourceGroupName, dnsServiceId, privateCloudName, Context.NONE);
}
public void deleteDnsServiceByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String dnsServiceId = ResourceManagerUtils.getValueFromIdByName(id, "dnsServices");
if (dnsServiceId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'dnsServices'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
this.deleteDnsService(resourceGroupName, dnsServiceId, privateCloudName, context);
}
public void deleteDnsZoneById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String dnsZoneId = ResourceManagerUtils.getValueFromIdByName(id, "dnsZones");
if (dnsZoneId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'dnsZones'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
this.deleteDnsZone(resourceGroupName, dnsZoneId, privateCloudName, Context.NONE);
}
public void deleteDnsZoneByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String dnsZoneId = ResourceManagerUtils.getValueFromIdByName(id, "dnsZones");
if (dnsZoneId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'dnsZones'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
this.deleteDnsZone(resourceGroupName, dnsZoneId, privateCloudName, context);
}
public void deletePortMirroringById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String portMirroringId = ResourceManagerUtils.getValueFromIdByName(id, "portMirroringProfiles");
if (portMirroringId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'portMirroringProfiles'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
this.deletePortMirroring(resourceGroupName, portMirroringId, privateCloudName, Context.NONE);
}
public void deletePortMirroringByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String portMirroringId = ResourceManagerUtils.getValueFromIdByName(id, "portMirroringProfiles");
if (portMirroringId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'portMirroringProfiles'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
this.deletePortMirroring(resourceGroupName, portMirroringId, privateCloudName, context);
}
public void deletePublicIpById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String publicIpId = ResourceManagerUtils.getValueFromIdByName(id, "publicIPs");
if (publicIpId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'publicIPs'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
this.deletePublicIp(resourceGroupName, publicIpId, privateCloudName, Context.NONE);
}
public void deletePublicIpByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String publicIpId = ResourceManagerUtils.getValueFromIdByName(id, "publicIPs");
if (publicIpId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'publicIPs'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
this.deletePublicIp(resourceGroupName, publicIpId, privateCloudName, context);
}
public void deleteSegmentById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String segmentId = ResourceManagerUtils.getValueFromIdByName(id, "segments");
if (segmentId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'segments'.", id)));
}
this.deleteSegment(resourceGroupName, privateCloudName, segmentId, Context.NONE);
}
public void deleteSegmentByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
String segmentId = ResourceManagerUtils.getValueFromIdByName(id, "segments");
if (segmentId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'segments'.", id)));
}
this.deleteSegment(resourceGroupName, privateCloudName, segmentId, context);
}
public void deleteVMGroupById(String id) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String vmGroupId = ResourceManagerUtils.getValueFromIdByName(id, "vmGroups");
if (vmGroupId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'vmGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
this.deleteVMGroup(resourceGroupName, vmGroupId, privateCloudName, Context.NONE);
}
public void deleteVMGroupByIdWithResponse(String id, Context context) {
String resourceGroupName = ResourceManagerUtils.getValueFromIdByName(id, "resourceGroups");
if (resourceGroupName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'resourceGroups'.", id)));
}
String vmGroupId = ResourceManagerUtils.getValueFromIdByName(id, "vmGroups");
if (vmGroupId == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'vmGroups'.", id)));
}
String privateCloudName = ResourceManagerUtils.getValueFromIdByName(id, "privateClouds");
if (privateCloudName == null) {
throw LOGGER.logExceptionAsError(new IllegalArgumentException(
String.format("The resource ID '%s' is not valid. Missing path segment 'privateClouds'.", id)));
}
this.deleteVMGroup(resourceGroupName, vmGroupId, privateCloudName, context);
}
private WorkloadNetworksClient serviceClient() {
return this.innerClient;
}
private com.azure.resourcemanager.avs.AvsManager manager() {
return this.serviceManager;
}
public WorkloadNetworkDhcpImpl defineDhcp(String name) {
return new WorkloadNetworkDhcpImpl(name, this.manager());
}
public WorkloadNetworkDnsServiceImpl defineDnsService(String name) {
return new WorkloadNetworkDnsServiceImpl(name, this.manager());
}
public WorkloadNetworkDnsZoneImpl defineDnsZone(String name) {
return new WorkloadNetworkDnsZoneImpl(name, this.manager());
}
public WorkloadNetworkPortMirroringImpl definePortMirroring(String name) {
return new WorkloadNetworkPortMirroringImpl(name, this.manager());
}
public WorkloadNetworkPublicIpImpl definePublicIp(String name) {
return new WorkloadNetworkPublicIpImpl(name, this.manager());
}
public WorkloadNetworkSegmentImpl defineSegments(String name) {
return new WorkloadNetworkSegmentImpl(name, this.manager());
}
public WorkloadNetworkVMGroupImpl defineVMGroup(String name) {
return new WorkloadNetworkVMGroupImpl(name, this.manager());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy