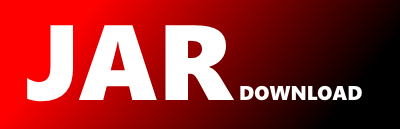
com.azure.resourcemanager.avs.models.WorkloadNetworkDnsService Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.avs.models;
import com.azure.core.management.SystemData;
import com.azure.core.util.Context;
import com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkDnsServiceInner;
import java.util.List;
/**
* An immutable client-side representation of WorkloadNetworkDnsService.
*/
public interface WorkloadNetworkDnsService {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the systemData property: Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
* @return the systemData value.
*/
SystemData systemData();
/**
* Gets the displayName property: Display name of the DNS Service.
*
* @return the displayName value.
*/
String displayName();
/**
* Gets the dnsServiceIp property: DNS service IP of the DNS Service.
*
* @return the dnsServiceIp value.
*/
String dnsServiceIp();
/**
* Gets the defaultDnsZone property: Default DNS zone of the DNS Service.
*
* @return the defaultDnsZone value.
*/
String defaultDnsZone();
/**
* Gets the fqdnZones property: FQDN zones of the DNS Service.
*
* @return the fqdnZones value.
*/
List fqdnZones();
/**
* Gets the logLevel property: DNS Service log level.
*
* @return the logLevel value.
*/
DnsServiceLogLevelEnum logLevel();
/**
* Gets the status property: DNS Service status.
*
* @return the status value.
*/
DnsServiceStatusEnum status();
/**
* Gets the provisioningState property: The provisioning state.
*
* @return the provisioningState value.
*/
WorkloadNetworkDnsServiceProvisioningState provisioningState();
/**
* Gets the revision property: NSX revision number.
*
* @return the revision value.
*/
Long revision();
/**
* Gets the name of the resource group.
*
* @return the name of the resource group.
*/
String resourceGroupName();
/**
* Gets the inner com.azure.resourcemanager.avs.fluent.models.WorkloadNetworkDnsServiceInner object.
*
* @return the inner object.
*/
WorkloadNetworkDnsServiceInner innerModel();
/**
* The entirety of the WorkloadNetworkDnsService definition.
*/
interface Definition
extends DefinitionStages.Blank, DefinitionStages.WithParentResource, DefinitionStages.WithCreate {
}
/**
* The WorkloadNetworkDnsService definition stages.
*/
interface DefinitionStages {
/**
* The first stage of the WorkloadNetworkDnsService definition.
*/
interface Blank extends WithParentResource {
}
/**
* The stage of the WorkloadNetworkDnsService definition allowing to specify parent resource.
*/
interface WithParentResource {
/**
* Specifies resourceGroupName, privateCloudName.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param privateCloudName Name of the private cloud.
* @return the next definition stage.
*/
WithCreate withExistingPrivateCloud(String resourceGroupName, String privateCloudName);
}
/**
* The stage of the WorkloadNetworkDnsService definition which contains all the minimum required properties for
* the resource to be created, but also allows for any other optional properties to be specified.
*/
interface WithCreate extends DefinitionStages.WithDisplayName, DefinitionStages.WithDnsServiceIp,
DefinitionStages.WithDefaultDnsZone, DefinitionStages.WithFqdnZones, DefinitionStages.WithLogLevel,
DefinitionStages.WithRevision {
/**
* Executes the create request.
*
* @return the created resource.
*/
WorkloadNetworkDnsService create();
/**
* Executes the create request.
*
* @param context The context to associate with this operation.
* @return the created resource.
*/
WorkloadNetworkDnsService create(Context context);
}
/**
* The stage of the WorkloadNetworkDnsService definition allowing to specify displayName.
*/
interface WithDisplayName {
/**
* Specifies the displayName property: Display name of the DNS Service..
*
* @param displayName Display name of the DNS Service.
* @return the next definition stage.
*/
WithCreate withDisplayName(String displayName);
}
/**
* The stage of the WorkloadNetworkDnsService definition allowing to specify dnsServiceIp.
*/
interface WithDnsServiceIp {
/**
* Specifies the dnsServiceIp property: DNS service IP of the DNS Service..
*
* @param dnsServiceIp DNS service IP of the DNS Service.
* @return the next definition stage.
*/
WithCreate withDnsServiceIp(String dnsServiceIp);
}
/**
* The stage of the WorkloadNetworkDnsService definition allowing to specify defaultDnsZone.
*/
interface WithDefaultDnsZone {
/**
* Specifies the defaultDnsZone property: Default DNS zone of the DNS Service..
*
* @param defaultDnsZone Default DNS zone of the DNS Service.
* @return the next definition stage.
*/
WithCreate withDefaultDnsZone(String defaultDnsZone);
}
/**
* The stage of the WorkloadNetworkDnsService definition allowing to specify fqdnZones.
*/
interface WithFqdnZones {
/**
* Specifies the fqdnZones property: FQDN zones of the DNS Service..
*
* @param fqdnZones FQDN zones of the DNS Service.
* @return the next definition stage.
*/
WithCreate withFqdnZones(List fqdnZones);
}
/**
* The stage of the WorkloadNetworkDnsService definition allowing to specify logLevel.
*/
interface WithLogLevel {
/**
* Specifies the logLevel property: DNS Service log level..
*
* @param logLevel DNS Service log level.
* @return the next definition stage.
*/
WithCreate withLogLevel(DnsServiceLogLevelEnum logLevel);
}
/**
* The stage of the WorkloadNetworkDnsService definition allowing to specify revision.
*/
interface WithRevision {
/**
* Specifies the revision property: NSX revision number..
*
* @param revision NSX revision number.
* @return the next definition stage.
*/
WithCreate withRevision(Long revision);
}
}
/**
* Begins update for the WorkloadNetworkDnsService resource.
*
* @return the stage of resource update.
*/
WorkloadNetworkDnsService.Update update();
/**
* The template for WorkloadNetworkDnsService update.
*/
interface Update
extends UpdateStages.WithDisplayName, UpdateStages.WithDnsServiceIp, UpdateStages.WithDefaultDnsZone,
UpdateStages.WithFqdnZones, UpdateStages.WithLogLevel, UpdateStages.WithRevision {
/**
* Executes the update request.
*
* @return the updated resource.
*/
WorkloadNetworkDnsService apply();
/**
* Executes the update request.
*
* @param context The context to associate with this operation.
* @return the updated resource.
*/
WorkloadNetworkDnsService apply(Context context);
}
/**
* The WorkloadNetworkDnsService update stages.
*/
interface UpdateStages {
/**
* The stage of the WorkloadNetworkDnsService update allowing to specify displayName.
*/
interface WithDisplayName {
/**
* Specifies the displayName property: Display name of the DNS Service..
*
* @param displayName Display name of the DNS Service.
* @return the next definition stage.
*/
Update withDisplayName(String displayName);
}
/**
* The stage of the WorkloadNetworkDnsService update allowing to specify dnsServiceIp.
*/
interface WithDnsServiceIp {
/**
* Specifies the dnsServiceIp property: DNS service IP of the DNS Service..
*
* @param dnsServiceIp DNS service IP of the DNS Service.
* @return the next definition stage.
*/
Update withDnsServiceIp(String dnsServiceIp);
}
/**
* The stage of the WorkloadNetworkDnsService update allowing to specify defaultDnsZone.
*/
interface WithDefaultDnsZone {
/**
* Specifies the defaultDnsZone property: Default DNS zone of the DNS Service..
*
* @param defaultDnsZone Default DNS zone of the DNS Service.
* @return the next definition stage.
*/
Update withDefaultDnsZone(String defaultDnsZone);
}
/**
* The stage of the WorkloadNetworkDnsService update allowing to specify fqdnZones.
*/
interface WithFqdnZones {
/**
* Specifies the fqdnZones property: FQDN zones of the DNS Service..
*
* @param fqdnZones FQDN zones of the DNS Service.
* @return the next definition stage.
*/
Update withFqdnZones(List fqdnZones);
}
/**
* The stage of the WorkloadNetworkDnsService update allowing to specify logLevel.
*/
interface WithLogLevel {
/**
* Specifies the logLevel property: DNS Service log level..
*
* @param logLevel DNS Service log level.
* @return the next definition stage.
*/
Update withLogLevel(DnsServiceLogLevelEnum logLevel);
}
/**
* The stage of the WorkloadNetworkDnsService update allowing to specify revision.
*/
interface WithRevision {
/**
* Specifies the revision property: NSX revision number..
*
* @param revision NSX revision number.
* @return the next definition stage.
*/
Update withRevision(Long revision);
}
}
/**
* Refreshes the resource to sync with Azure.
*
* @return the refreshed resource.
*/
WorkloadNetworkDnsService refresh();
/**
* Refreshes the resource to sync with Azure.
*
* @param context The context to associate with this operation.
* @return the refreshed resource.
*/
WorkloadNetworkDnsService refresh(Context context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy