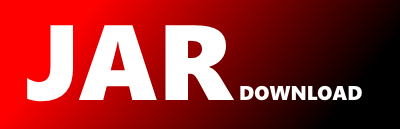
com.azure.resourcemanager.compute.fluent.VirtualMachineScaleSetVMsClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-compute Show documentation
Show all versions of azure-resourcemanager-compute Show documentation
This package contains Microsoft Azure Compute Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.compute.fluent;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.polling.PollerFlux;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.compute.fluent.models.RetrieveBootDiagnosticsDataResultInner;
import com.azure.resourcemanager.compute.fluent.models.RunCommandResultInner;
import com.azure.resourcemanager.compute.fluent.models.VirtualMachineScaleSetVMInner;
import com.azure.resourcemanager.compute.fluent.models.VirtualMachineScaleSetVMInstanceViewInner;
import com.azure.resourcemanager.compute.models.AttachDetachDataDisksRequest;
import com.azure.resourcemanager.compute.models.InstanceViewTypes;
import com.azure.resourcemanager.compute.models.RunCommandInput;
import com.azure.resourcemanager.compute.models.StorageProfile;
import com.azure.resourcemanager.compute.models.VirtualMachineScaleSetVMReimageParameters;
import java.nio.ByteBuffer;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* An instance of this class provides access to all the operations defined in VirtualMachineScaleSetVMsClient.
*/
public interface VirtualMachineScaleSetVMsClient {
/**
* Reimages (upgrade the operating system) a specific virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param vmScaleSetVMReimageInput Parameters for the Reimaging Virtual machine in ScaleSet.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> reimageWithResponseAsync(String resourceGroupName, String vmScaleSetName,
String instanceId, VirtualMachineScaleSetVMReimageParameters vmScaleSetVMReimageInput);
/**
* Reimages (upgrade the operating system) a specific virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param vmScaleSetVMReimageInput Parameters for the Reimaging Virtual machine in ScaleSet.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginReimageAsync(String resourceGroupName, String vmScaleSetName,
String instanceId, VirtualMachineScaleSetVMReimageParameters vmScaleSetVMReimageInput);
/**
* Reimages (upgrade the operating system) a specific virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginReimageAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Reimages (upgrade the operating system) a specific virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginReimage(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Reimages (upgrade the operating system) a specific virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param vmScaleSetVMReimageInput Parameters for the Reimaging Virtual machine in ScaleSet.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginReimage(String resourceGroupName, String vmScaleSetName, String instanceId,
VirtualMachineScaleSetVMReimageParameters vmScaleSetVMReimageInput, Context context);
/**
* Reimages (upgrade the operating system) a specific virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param vmScaleSetVMReimageInput Parameters for the Reimaging Virtual machine in ScaleSet.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono reimageAsync(String resourceGroupName, String vmScaleSetName, String instanceId,
VirtualMachineScaleSetVMReimageParameters vmScaleSetVMReimageInput);
/**
* Reimages (upgrade the operating system) a specific virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono reimageAsync(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Reimages (upgrade the operating system) a specific virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void reimage(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Reimages (upgrade the operating system) a specific virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param vmScaleSetVMReimageInput Parameters for the Reimaging Virtual machine in ScaleSet.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void reimage(String resourceGroupName, String vmScaleSetName, String instanceId,
VirtualMachineScaleSetVMReimageParameters vmScaleSetVMReimageInput, Context context);
/**
* Allows you to re-image all the disks ( including data disks ) in the a VM scale set instance. This operation is
* only supported for managed disks.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> reimageAllWithResponseAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Allows you to re-image all the disks ( including data disks ) in the a VM scale set instance. This operation is
* only supported for managed disks.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginReimageAllAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Allows you to re-image all the disks ( including data disks ) in the a VM scale set instance. This operation is
* only supported for managed disks.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginReimageAll(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Allows you to re-image all the disks ( including data disks ) in the a VM scale set instance. This operation is
* only supported for managed disks.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginReimageAll(String resourceGroupName, String vmScaleSetName,
String instanceId, Context context);
/**
* Allows you to re-image all the disks ( including data disks ) in the a VM scale set instance. This operation is
* only supported for managed disks.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono reimageAllAsync(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Allows you to re-image all the disks ( including data disks ) in the a VM scale set instance. This operation is
* only supported for managed disks.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void reimageAll(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Allows you to re-image all the disks ( including data disks ) in the a VM scale set instance. This operation is
* only supported for managed disks.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void reimageAll(String resourceGroupName, String vmScaleSetName, String instanceId, Context context);
/**
* Approve upgrade on deferred rolling upgrade for OS disk on a VM scale set instance.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> approveRollingUpgradeWithResponseAsync(String resourceGroupName,
String vmScaleSetName, String instanceId);
/**
* Approve upgrade on deferred rolling upgrade for OS disk on a VM scale set instance.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginApproveRollingUpgradeAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Approve upgrade on deferred rolling upgrade for OS disk on a VM scale set instance.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginApproveRollingUpgrade(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Approve upgrade on deferred rolling upgrade for OS disk on a VM scale set instance.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginApproveRollingUpgrade(String resourceGroupName, String vmScaleSetName,
String instanceId, Context context);
/**
* Approve upgrade on deferred rolling upgrade for OS disk on a VM scale set instance.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono approveRollingUpgradeAsync(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Approve upgrade on deferred rolling upgrade for OS disk on a VM scale set instance.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void approveRollingUpgrade(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Approve upgrade on deferred rolling upgrade for OS disk on a VM scale set instance.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void approveRollingUpgrade(String resourceGroupName, String vmScaleSetName, String instanceId, Context context);
/**
* Deallocates a specific virtual machine in a VM scale set. Shuts down the virtual machine and releases the compute
* resources it uses. You are not billed for the compute resources of this virtual machine once it is deallocated.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deallocateWithResponseAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Deallocates a specific virtual machine in a VM scale set. Shuts down the virtual machine and releases the compute
* resources it uses. You are not billed for the compute resources of this virtual machine once it is deallocated.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeallocateAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Deallocates a specific virtual machine in a VM scale set. Shuts down the virtual machine and releases the compute
* resources it uses. You are not billed for the compute resources of this virtual machine once it is deallocated.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeallocate(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Deallocates a specific virtual machine in a VM scale set. Shuts down the virtual machine and releases the compute
* resources it uses. You are not billed for the compute resources of this virtual machine once it is deallocated.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeallocate(String resourceGroupName, String vmScaleSetName,
String instanceId, Context context);
/**
* Deallocates a specific virtual machine in a VM scale set. Shuts down the virtual machine and releases the compute
* resources it uses. You are not billed for the compute resources of this virtual machine once it is deallocated.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deallocateAsync(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Deallocates a specific virtual machine in a VM scale set. Shuts down the virtual machine and releases the compute
* resources it uses. You are not billed for the compute resources of this virtual machine once it is deallocated.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deallocate(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Deallocates a specific virtual machine in a VM scale set. Shuts down the virtual machine and releases the compute
* resources it uses. You are not billed for the compute resources of this virtual machine once it is deallocated.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deallocate(String resourceGroupName, String vmScaleSetName, String instanceId, Context context);
/**
* Updates a virtual machine of a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set where the extension should be create or updated.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine Scale Sets VM operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a virtual machine scale set virtual machine along with {@link Response} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> updateWithResponseAsync(String resourceGroupName, String vmScaleSetName,
String instanceId, VirtualMachineScaleSetVMInner parameters, String ifMatch, String ifNoneMatch);
/**
* Updates a virtual machine of a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set where the extension should be create or updated.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine Scale Sets VM operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of describes a virtual machine scale set virtual machine.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, VirtualMachineScaleSetVMInner> beginUpdateAsync(
String resourceGroupName, String vmScaleSetName, String instanceId, VirtualMachineScaleSetVMInner parameters,
String ifMatch, String ifNoneMatch);
/**
* Updates a virtual machine of a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set where the extension should be create or updated.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine Scale Sets VM operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of describes a virtual machine scale set virtual machine.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, VirtualMachineScaleSetVMInner> beginUpdateAsync(
String resourceGroupName, String vmScaleSetName, String instanceId, VirtualMachineScaleSetVMInner parameters);
/**
* Updates a virtual machine of a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set where the extension should be create or updated.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine Scale Sets VM operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of describes a virtual machine scale set virtual machine.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, VirtualMachineScaleSetVMInner> beginUpdate(
String resourceGroupName, String vmScaleSetName, String instanceId, VirtualMachineScaleSetVMInner parameters);
/**
* Updates a virtual machine of a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set where the extension should be create or updated.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine Scale Sets VM operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of describes a virtual machine scale set virtual machine.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, VirtualMachineScaleSetVMInner> beginUpdate(
String resourceGroupName, String vmScaleSetName, String instanceId, VirtualMachineScaleSetVMInner parameters,
String ifMatch, String ifNoneMatch, Context context);
/**
* Updates a virtual machine of a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set where the extension should be create or updated.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine Scale Sets VM operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a virtual machine scale set virtual machine on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono updateAsync(String resourceGroupName, String vmScaleSetName, String instanceId,
VirtualMachineScaleSetVMInner parameters, String ifMatch, String ifNoneMatch);
/**
* Updates a virtual machine of a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set where the extension should be create or updated.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine Scale Sets VM operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a virtual machine scale set virtual machine on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono updateAsync(String resourceGroupName, String vmScaleSetName, String instanceId,
VirtualMachineScaleSetVMInner parameters);
/**
* Updates a virtual machine of a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set where the extension should be create or updated.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine Scale Sets VM operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a virtual machine scale set virtual machine.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
VirtualMachineScaleSetVMInner update(String resourceGroupName, String vmScaleSetName, String instanceId,
VirtualMachineScaleSetVMInner parameters);
/**
* Updates a virtual machine of a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set where the extension should be create or updated.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine Scale Sets VM operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a virtual machine scale set virtual machine.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
VirtualMachineScaleSetVMInner update(String resourceGroupName, String vmScaleSetName, String instanceId,
VirtualMachineScaleSetVMInner parameters, String ifMatch, String ifNoneMatch, Context context);
/**
* Deletes a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param forceDeletion Optional parameter to force delete a virtual machine from a VM scale set. (Feature in
* Preview).
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deleteWithResponseAsync(String resourceGroupName, String vmScaleSetName,
String instanceId, Boolean forceDeletion);
/**
* Deletes a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param forceDeletion Optional parameter to force delete a virtual machine from a VM scale set. (Feature in
* Preview).
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteAsync(String resourceGroupName, String vmScaleSetName,
String instanceId, Boolean forceDeletion);
/**
* Deletes a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Deletes a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDelete(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Deletes a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param forceDeletion Optional parameter to force delete a virtual machine from a VM scale set. (Feature in
* Preview).
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDelete(String resourceGroupName, String vmScaleSetName, String instanceId,
Boolean forceDeletion, Context context);
/**
* Deletes a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param forceDeletion Optional parameter to force delete a virtual machine from a VM scale set. (Feature in
* Preview).
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteAsync(String resourceGroupName, String vmScaleSetName, String instanceId, Boolean forceDeletion);
/**
* Deletes a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteAsync(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Deletes a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void delete(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Deletes a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param forceDeletion Optional parameter to force delete a virtual machine from a VM scale set. (Feature in
* Preview).
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void delete(String resourceGroupName, String vmScaleSetName, String instanceId, Boolean forceDeletion,
Context context);
/**
* Gets a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param expand The expand expression to apply on the operation. 'InstanceView' will retrieve the instance view of
* the virtual machine. 'UserData' will retrieve the UserData of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a virtual machine from a VM scale set along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getWithResponseAsync(String resourceGroupName, String vmScaleSetName,
String instanceId, InstanceViewTypes expand);
/**
* Gets a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a virtual machine from a VM scale set on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getAsync(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Gets a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param expand The expand expression to apply on the operation. 'InstanceView' will retrieve the instance view of
* the virtual machine. 'UserData' will retrieve the UserData of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a virtual machine from a VM scale set along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getWithResponse(String resourceGroupName, String vmScaleSetName,
String instanceId, InstanceViewTypes expand, Context context);
/**
* Gets a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a virtual machine from a VM scale set.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
VirtualMachineScaleSetVMInner get(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Gets the status of a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the status of a virtual machine from a VM scale set along with {@link Response} on successful completion
* of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getInstanceViewWithResponseAsync(String resourceGroupName,
String vmScaleSetName, String instanceId);
/**
* Gets the status of a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the status of a virtual machine from a VM scale set on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getInstanceViewAsync(String resourceGroupName,
String vmScaleSetName, String instanceId);
/**
* Gets the status of a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the status of a virtual machine from a VM scale set along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getInstanceViewWithResponse(String resourceGroupName,
String vmScaleSetName, String instanceId, Context context);
/**
* Gets the status of a virtual machine from a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the status of a virtual machine from a VM scale set.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
VirtualMachineScaleSetVMInstanceViewInner getInstanceView(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Gets a list of all virtual machines in a VM scale sets.
*
* @param resourceGroupName The name of the resource group.
* @param virtualMachineScaleSetName The name of the VM scale set.
* @param filter The filter to apply to the operation. Allowed values are 'startswith(instanceView/statuses/code,
* 'PowerState') eq true', 'properties/latestModelApplied eq true', 'properties/latestModelApplied eq false'.
* @param select The list parameters. Allowed values are 'instanceView', 'instanceView/statuses'.
* @param expand The expand expression to apply to the operation. Allowed values are 'instanceView'.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of all virtual machines in a VM scale sets as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listAsync(String resourceGroupName, String virtualMachineScaleSetName,
String filter, String select, String expand);
/**
* Gets a list of all virtual machines in a VM scale sets.
*
* @param resourceGroupName The name of the resource group.
* @param virtualMachineScaleSetName The name of the VM scale set.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of all virtual machines in a VM scale sets as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listAsync(String resourceGroupName, String virtualMachineScaleSetName);
/**
* Gets a list of all virtual machines in a VM scale sets.
*
* @param resourceGroupName The name of the resource group.
* @param virtualMachineScaleSetName The name of the VM scale set.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of all virtual machines in a VM scale sets as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable list(String resourceGroupName, String virtualMachineScaleSetName);
/**
* Gets a list of all virtual machines in a VM scale sets.
*
* @param resourceGroupName The name of the resource group.
* @param virtualMachineScaleSetName The name of the VM scale set.
* @param filter The filter to apply to the operation. Allowed values are 'startswith(instanceView/statuses/code,
* 'PowerState') eq true', 'properties/latestModelApplied eq true', 'properties/latestModelApplied eq false'.
* @param select The list parameters. Allowed values are 'instanceView', 'instanceView/statuses'.
* @param expand The expand expression to apply to the operation. Allowed values are 'instanceView'.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of all virtual machines in a VM scale sets as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable list(String resourceGroupName, String virtualMachineScaleSetName,
String filter, String select, String expand, Context context);
/**
* Power off (stop) a virtual machine in a VM scale set. Note that resources are still attached and you are getting
* charged for the resources. Instead, use deallocate to release resources and avoid charges.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param skipShutdown The parameter to request non-graceful VM shutdown. True value for this flag indicates
* non-graceful shutdown whereas false indicates otherwise. Default value for this flag is false if not specified.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> powerOffWithResponseAsync(String resourceGroupName, String vmScaleSetName,
String instanceId, Boolean skipShutdown);
/**
* Power off (stop) a virtual machine in a VM scale set. Note that resources are still attached and you are getting
* charged for the resources. Instead, use deallocate to release resources and avoid charges.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param skipShutdown The parameter to request non-graceful VM shutdown. True value for this flag indicates
* non-graceful shutdown whereas false indicates otherwise. Default value for this flag is false if not specified.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginPowerOffAsync(String resourceGroupName, String vmScaleSetName,
String instanceId, Boolean skipShutdown);
/**
* Power off (stop) a virtual machine in a VM scale set. Note that resources are still attached and you are getting
* charged for the resources. Instead, use deallocate to release resources and avoid charges.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginPowerOffAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Power off (stop) a virtual machine in a VM scale set. Note that resources are still attached and you are getting
* charged for the resources. Instead, use deallocate to release resources and avoid charges.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginPowerOff(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Power off (stop) a virtual machine in a VM scale set. Note that resources are still attached and you are getting
* charged for the resources. Instead, use deallocate to release resources and avoid charges.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param skipShutdown The parameter to request non-graceful VM shutdown. True value for this flag indicates
* non-graceful shutdown whereas false indicates otherwise. Default value for this flag is false if not specified.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginPowerOff(String resourceGroupName, String vmScaleSetName, String instanceId,
Boolean skipShutdown, Context context);
/**
* Power off (stop) a virtual machine in a VM scale set. Note that resources are still attached and you are getting
* charged for the resources. Instead, use deallocate to release resources and avoid charges.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param skipShutdown The parameter to request non-graceful VM shutdown. True value for this flag indicates
* non-graceful shutdown whereas false indicates otherwise. Default value for this flag is false if not specified.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono powerOffAsync(String resourceGroupName, String vmScaleSetName, String instanceId, Boolean skipShutdown);
/**
* Power off (stop) a virtual machine in a VM scale set. Note that resources are still attached and you are getting
* charged for the resources. Instead, use deallocate to release resources and avoid charges.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono powerOffAsync(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Power off (stop) a virtual machine in a VM scale set. Note that resources are still attached and you are getting
* charged for the resources. Instead, use deallocate to release resources and avoid charges.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void powerOff(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Power off (stop) a virtual machine in a VM scale set. Note that resources are still attached and you are getting
* charged for the resources. Instead, use deallocate to release resources and avoid charges.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param skipShutdown The parameter to request non-graceful VM shutdown. True value for this flag indicates
* non-graceful shutdown whereas false indicates otherwise. Default value for this flag is false if not specified.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void powerOff(String resourceGroupName, String vmScaleSetName, String instanceId, Boolean skipShutdown,
Context context);
/**
* Restarts a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> restartWithResponseAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Restarts a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginRestartAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Restarts a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginRestart(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Restarts a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginRestart(String resourceGroupName, String vmScaleSetName, String instanceId,
Context context);
/**
* Restarts a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono restartAsync(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Restarts a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void restart(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Restarts a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void restart(String resourceGroupName, String vmScaleSetName, String instanceId, Context context);
/**
* Starts a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> startWithResponseAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Starts a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginStartAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Starts a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginStart(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Starts a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginStart(String resourceGroupName, String vmScaleSetName, String instanceId,
Context context);
/**
* Starts a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono startAsync(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Starts a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void start(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Starts a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void start(String resourceGroupName, String vmScaleSetName, String instanceId, Context context);
/**
* Shuts down the virtual machine in the virtual machine scale set, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> redeployWithResponseAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Shuts down the virtual machine in the virtual machine scale set, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginRedeployAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Shuts down the virtual machine in the virtual machine scale set, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginRedeploy(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Shuts down the virtual machine in the virtual machine scale set, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginRedeploy(String resourceGroupName, String vmScaleSetName, String instanceId,
Context context);
/**
* Shuts down the virtual machine in the virtual machine scale set, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono redeployAsync(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Shuts down the virtual machine in the virtual machine scale set, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void redeploy(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Shuts down the virtual machine in the virtual machine scale set, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void redeploy(String resourceGroupName, String vmScaleSetName, String instanceId, Context context);
/**
* The operation to retrieve SAS URIs of boot diagnostic logs for a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param sasUriExpirationTimeInMinutes Expiration duration in minutes for the SAS URIs with a value between 1 to
* 1440 minutes. **Note:** If not specified, SAS URIs will be generated with a default expiration duration of 120
* minutes.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SAS URIs of the console screenshot and serial log blobs along with {@link Response} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> retrieveBootDiagnosticsDataWithResponseAsync(
String resourceGroupName, String vmScaleSetName, String instanceId, Integer sasUriExpirationTimeInMinutes);
/**
* The operation to retrieve SAS URIs of boot diagnostic logs for a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SAS URIs of the console screenshot and serial log blobs on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono retrieveBootDiagnosticsDataAsync(String resourceGroupName,
String vmScaleSetName, String instanceId);
/**
* The operation to retrieve SAS URIs of boot diagnostic logs for a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param sasUriExpirationTimeInMinutes Expiration duration in minutes for the SAS URIs with a value between 1 to
* 1440 minutes. **Note:** If not specified, SAS URIs will be generated with a default expiration duration of 120
* minutes.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SAS URIs of the console screenshot and serial log blobs along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response retrieveBootDiagnosticsDataWithResponse(String resourceGroupName,
String vmScaleSetName, String instanceId, Integer sasUriExpirationTimeInMinutes, Context context);
/**
* The operation to retrieve SAS URIs of boot diagnostic logs for a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SAS URIs of the console screenshot and serial log blobs.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
RetrieveBootDiagnosticsDataResultInner retrieveBootDiagnosticsData(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Performs maintenance on a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> performMaintenanceWithResponseAsync(String resourceGroupName,
String vmScaleSetName, String instanceId);
/**
* Performs maintenance on a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginPerformMaintenanceAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Performs maintenance on a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginPerformMaintenance(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* Performs maintenance on a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginPerformMaintenance(String resourceGroupName, String vmScaleSetName,
String instanceId, Context context);
/**
* Performs maintenance on a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono performMaintenanceAsync(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Performs maintenance on a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void performMaintenance(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Performs maintenance on a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void performMaintenance(String resourceGroupName, String vmScaleSetName, String instanceId, Context context);
/**
* The operation to simulate the eviction of spot virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> simulateEvictionWithResponseAsync(String resourceGroupName, String vmScaleSetName,
String instanceId);
/**
* The operation to simulate the eviction of spot virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono simulateEvictionAsync(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* The operation to simulate the eviction of spot virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response simulateEvictionWithResponse(String resourceGroupName, String vmScaleSetName, String instanceId,
Context context);
/**
* The operation to simulate the eviction of spot virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void simulateEviction(String resourceGroupName, String vmScaleSetName, String instanceId);
/**
* Attach and detach data disks to/from a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the attach and detach data disks operation on a Virtual Machine Scale
* Sets VM.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return specifies the storage settings for the virtual machine disks along with {@link Response} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> attachDetachDataDisksWithResponseAsync(String resourceGroupName,
String vmScaleSetName, String instanceId, AttachDetachDataDisksRequest parameters);
/**
* Attach and detach data disks to/from a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the attach and detach data disks operation on a Virtual Machine Scale
* Sets VM.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of specifies the storage settings for the virtual machine disks.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, StorageProfile> beginAttachDetachDataDisksAsync(String resourceGroupName,
String vmScaleSetName, String instanceId, AttachDetachDataDisksRequest parameters);
/**
* Attach and detach data disks to/from a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the attach and detach data disks operation on a Virtual Machine Scale
* Sets VM.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of specifies the storage settings for the virtual machine disks.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, StorageProfile> beginAttachDetachDataDisks(String resourceGroupName,
String vmScaleSetName, String instanceId, AttachDetachDataDisksRequest parameters);
/**
* Attach and detach data disks to/from a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the attach and detach data disks operation on a Virtual Machine Scale
* Sets VM.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of specifies the storage settings for the virtual machine disks.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, StorageProfile> beginAttachDetachDataDisks(String resourceGroupName,
String vmScaleSetName, String instanceId, AttachDetachDataDisksRequest parameters, Context context);
/**
* Attach and detach data disks to/from a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the attach and detach data disks operation on a Virtual Machine Scale
* Sets VM.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return specifies the storage settings for the virtual machine disks on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono attachDetachDataDisksAsync(String resourceGroupName, String vmScaleSetName, String instanceId,
AttachDetachDataDisksRequest parameters);
/**
* Attach and detach data disks to/from a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the attach and detach data disks operation on a Virtual Machine Scale
* Sets VM.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return specifies the storage settings for the virtual machine disks.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
StorageProfile attachDetachDataDisks(String resourceGroupName, String vmScaleSetName, String instanceId,
AttachDetachDataDisksRequest parameters);
/**
* Attach and detach data disks to/from a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the attach and detach data disks operation on a Virtual Machine Scale
* Sets VM.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.resourcemanager.compute.models.ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return specifies the storage settings for the virtual machine disks.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
StorageProfile attachDetachDataDisks(String resourceGroupName, String vmScaleSetName, String instanceId,
AttachDetachDataDisksRequest parameters, Context context);
/**
* Run command on a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Run command operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response body along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> runCommandWithResponseAsync(String resourceGroupName, String vmScaleSetName,
String instanceId, RunCommandInput parameters);
/**
* Run command on a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Run command operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, RunCommandResultInner> beginRunCommandAsync(String resourceGroupName,
String vmScaleSetName, String instanceId, RunCommandInput parameters);
/**
* Run command on a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Run command operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, RunCommandResultInner> beginRunCommand(String resourceGroupName,
String vmScaleSetName, String instanceId, RunCommandInput parameters);
/**
* Run command on a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Run command operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, RunCommandResultInner> beginRunCommand(String resourceGroupName,
String vmScaleSetName, String instanceId, RunCommandInput parameters, Context context);
/**
* Run command on a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Run command operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response body on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono runCommandAsync(String resourceGroupName, String vmScaleSetName, String instanceId,
RunCommandInput parameters);
/**
* Run command on a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Run command operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
RunCommandResultInner runCommand(String resourceGroupName, String vmScaleSetName, String instanceId,
RunCommandInput parameters);
/**
* Run command on a virtual machine in a VM scale set.
*
* @param resourceGroupName The name of the resource group.
* @param vmScaleSetName The name of the VM scale set.
* @param instanceId The instance ID of the virtual machine.
* @param parameters Parameters supplied to the Run command operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
RunCommandResultInner runCommand(String resourceGroupName, String vmScaleSetName, String instanceId,
RunCommandInput parameters, Context context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy