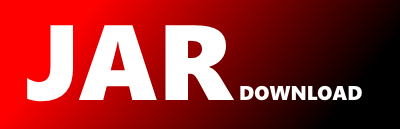
com.azure.resourcemanager.compute.fluent.models.VirtualMachineScaleSetUpdateProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-compute Show documentation
Show all versions of azure-resourcemanager-compute Show documentation
This package contains Microsoft Azure Compute Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.compute.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.SubResource;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.compute.models.AdditionalCapabilities;
import com.azure.resourcemanager.compute.models.AutomaticRepairsPolicy;
import com.azure.resourcemanager.compute.models.PriorityMixPolicy;
import com.azure.resourcemanager.compute.models.ResiliencyPolicy;
import com.azure.resourcemanager.compute.models.ScaleInPolicy;
import com.azure.resourcemanager.compute.models.SkuProfile;
import com.azure.resourcemanager.compute.models.SpotRestorePolicy;
import com.azure.resourcemanager.compute.models.UpgradePolicy;
import com.azure.resourcemanager.compute.models.VirtualMachineScaleSetUpdateVMProfile;
import com.azure.resourcemanager.compute.models.ZonalPlatformFaultDomainAlignMode;
import java.io.IOException;
/**
* Describes the properties of a Virtual Machine Scale Set.
*/
@Fluent
public final class VirtualMachineScaleSetUpdateProperties
implements JsonSerializable {
/*
* The upgrade policy.
*/
private UpgradePolicy upgradePolicy;
/*
* Policy for automatic repairs.
*/
private AutomaticRepairsPolicy automaticRepairsPolicy;
/*
* The virtual machine profile.
*/
private VirtualMachineScaleSetUpdateVMProfile virtualMachineProfile;
/*
* Specifies whether the Virtual Machine Scale Set should be overprovisioned.
*/
private Boolean overprovision;
/*
* When Overprovision is enabled, extensions are launched only on the requested number of VMs which are finally
* kept. This property will hence ensure that the extensions do not run on the extra overprovisioned VMs.
*/
private Boolean doNotRunExtensionsOnOverprovisionedVMs;
/*
* When true this limits the scale set to a single placement group, of max size 100 virtual machines. NOTE: If
* singlePlacementGroup is true, it may be modified to false. However, if singlePlacementGroup is false, it may not
* be modified to true.
*/
private Boolean singlePlacementGroup;
/*
* Specifies additional capabilities enabled or disabled on the Virtual Machines in the Virtual Machine Scale Set.
* For instance: whether the Virtual Machines have the capability to support attaching managed data disks with
* UltraSSD_LRS storage account type.
*/
private AdditionalCapabilities additionalCapabilities;
/*
* Specifies the policies applied when scaling in Virtual Machines in the Virtual Machine Scale Set.
*/
private ScaleInPolicy scaleInPolicy;
/*
* Specifies information about the proximity placement group that the virtual machine scale set should be assigned
* to.
Minimum api-version: 2018-04-01.
*/
private SubResource proximityPlacementGroup;
/*
* Specifies the desired targets for mixing Spot and Regular priority VMs within the same VMSS Flex instance.
*/
private PriorityMixPolicy priorityMixPolicy;
/*
* Specifies the Spot Restore properties for the virtual machine scale set.
*/
private SpotRestorePolicy spotRestorePolicy;
/*
* Policy for Resiliency
*/
private ResiliencyPolicy resiliencyPolicy;
/*
* Specifies the align mode between Virtual Machine Scale Set compute and storage Fault Domain count.
*/
private ZonalPlatformFaultDomainAlignMode zonalPlatformFaultDomainAlignMode;
/*
* Specifies the sku profile for the virtual machine scale set.
*/
private SkuProfile skuProfile;
/**
* Creates an instance of VirtualMachineScaleSetUpdateProperties class.
*/
public VirtualMachineScaleSetUpdateProperties() {
}
/**
* Get the upgradePolicy property: The upgrade policy.
*
* @return the upgradePolicy value.
*/
public UpgradePolicy upgradePolicy() {
return this.upgradePolicy;
}
/**
* Set the upgradePolicy property: The upgrade policy.
*
* @param upgradePolicy the upgradePolicy value to set.
* @return the VirtualMachineScaleSetUpdateProperties object itself.
*/
public VirtualMachineScaleSetUpdateProperties withUpgradePolicy(UpgradePolicy upgradePolicy) {
this.upgradePolicy = upgradePolicy;
return this;
}
/**
* Get the automaticRepairsPolicy property: Policy for automatic repairs.
*
* @return the automaticRepairsPolicy value.
*/
public AutomaticRepairsPolicy automaticRepairsPolicy() {
return this.automaticRepairsPolicy;
}
/**
* Set the automaticRepairsPolicy property: Policy for automatic repairs.
*
* @param automaticRepairsPolicy the automaticRepairsPolicy value to set.
* @return the VirtualMachineScaleSetUpdateProperties object itself.
*/
public VirtualMachineScaleSetUpdateProperties
withAutomaticRepairsPolicy(AutomaticRepairsPolicy automaticRepairsPolicy) {
this.automaticRepairsPolicy = automaticRepairsPolicy;
return this;
}
/**
* Get the virtualMachineProfile property: The virtual machine profile.
*
* @return the virtualMachineProfile value.
*/
public VirtualMachineScaleSetUpdateVMProfile virtualMachineProfile() {
return this.virtualMachineProfile;
}
/**
* Set the virtualMachineProfile property: The virtual machine profile.
*
* @param virtualMachineProfile the virtualMachineProfile value to set.
* @return the VirtualMachineScaleSetUpdateProperties object itself.
*/
public VirtualMachineScaleSetUpdateProperties
withVirtualMachineProfile(VirtualMachineScaleSetUpdateVMProfile virtualMachineProfile) {
this.virtualMachineProfile = virtualMachineProfile;
return this;
}
/**
* Get the overprovision property: Specifies whether the Virtual Machine Scale Set should be overprovisioned.
*
* @return the overprovision value.
*/
public Boolean overprovision() {
return this.overprovision;
}
/**
* Set the overprovision property: Specifies whether the Virtual Machine Scale Set should be overprovisioned.
*
* @param overprovision the overprovision value to set.
* @return the VirtualMachineScaleSetUpdateProperties object itself.
*/
public VirtualMachineScaleSetUpdateProperties withOverprovision(Boolean overprovision) {
this.overprovision = overprovision;
return this;
}
/**
* Get the doNotRunExtensionsOnOverprovisionedVMs property: When Overprovision is enabled, extensions are launched
* only on the requested number of VMs which are finally kept. This property will hence ensure that the extensions
* do not run on the extra overprovisioned VMs.
*
* @return the doNotRunExtensionsOnOverprovisionedVMs value.
*/
public Boolean doNotRunExtensionsOnOverprovisionedVMs() {
return this.doNotRunExtensionsOnOverprovisionedVMs;
}
/**
* Set the doNotRunExtensionsOnOverprovisionedVMs property: When Overprovision is enabled, extensions are launched
* only on the requested number of VMs which are finally kept. This property will hence ensure that the extensions
* do not run on the extra overprovisioned VMs.
*
* @param doNotRunExtensionsOnOverprovisionedVMs the doNotRunExtensionsOnOverprovisionedVMs value to set.
* @return the VirtualMachineScaleSetUpdateProperties object itself.
*/
public VirtualMachineScaleSetUpdateProperties
withDoNotRunExtensionsOnOverprovisionedVMs(Boolean doNotRunExtensionsOnOverprovisionedVMs) {
this.doNotRunExtensionsOnOverprovisionedVMs = doNotRunExtensionsOnOverprovisionedVMs;
return this;
}
/**
* Get the singlePlacementGroup property: When true this limits the scale set to a single placement group, of max
* size 100 virtual machines. NOTE: If singlePlacementGroup is true, it may be modified to false. However, if
* singlePlacementGroup is false, it may not be modified to true.
*
* @return the singlePlacementGroup value.
*/
public Boolean singlePlacementGroup() {
return this.singlePlacementGroup;
}
/**
* Set the singlePlacementGroup property: When true this limits the scale set to a single placement group, of max
* size 100 virtual machines. NOTE: If singlePlacementGroup is true, it may be modified to false. However, if
* singlePlacementGroup is false, it may not be modified to true.
*
* @param singlePlacementGroup the singlePlacementGroup value to set.
* @return the VirtualMachineScaleSetUpdateProperties object itself.
*/
public VirtualMachineScaleSetUpdateProperties withSinglePlacementGroup(Boolean singlePlacementGroup) {
this.singlePlacementGroup = singlePlacementGroup;
return this;
}
/**
* Get the additionalCapabilities property: Specifies additional capabilities enabled or disabled on the Virtual
* Machines in the Virtual Machine Scale Set. For instance: whether the Virtual Machines have the capability to
* support attaching managed data disks with UltraSSD_LRS storage account type.
*
* @return the additionalCapabilities value.
*/
public AdditionalCapabilities additionalCapabilities() {
return this.additionalCapabilities;
}
/**
* Set the additionalCapabilities property: Specifies additional capabilities enabled or disabled on the Virtual
* Machines in the Virtual Machine Scale Set. For instance: whether the Virtual Machines have the capability to
* support attaching managed data disks with UltraSSD_LRS storage account type.
*
* @param additionalCapabilities the additionalCapabilities value to set.
* @return the VirtualMachineScaleSetUpdateProperties object itself.
*/
public VirtualMachineScaleSetUpdateProperties
withAdditionalCapabilities(AdditionalCapabilities additionalCapabilities) {
this.additionalCapabilities = additionalCapabilities;
return this;
}
/**
* Get the scaleInPolicy property: Specifies the policies applied when scaling in Virtual Machines in the Virtual
* Machine Scale Set.
*
* @return the scaleInPolicy value.
*/
public ScaleInPolicy scaleInPolicy() {
return this.scaleInPolicy;
}
/**
* Set the scaleInPolicy property: Specifies the policies applied when scaling in Virtual Machines in the Virtual
* Machine Scale Set.
*
* @param scaleInPolicy the scaleInPolicy value to set.
* @return the VirtualMachineScaleSetUpdateProperties object itself.
*/
public VirtualMachineScaleSetUpdateProperties withScaleInPolicy(ScaleInPolicy scaleInPolicy) {
this.scaleInPolicy = scaleInPolicy;
return this;
}
/**
* Get the proximityPlacementGroup property: Specifies information about the proximity placement group that the
* virtual machine scale set should be assigned to. <br><br>Minimum api-version: 2018-04-01.
*
* @return the proximityPlacementGroup value.
*/
public SubResource proximityPlacementGroup() {
return this.proximityPlacementGroup;
}
/**
* Set the proximityPlacementGroup property: Specifies information about the proximity placement group that the
* virtual machine scale set should be assigned to. <br><br>Minimum api-version: 2018-04-01.
*
* @param proximityPlacementGroup the proximityPlacementGroup value to set.
* @return the VirtualMachineScaleSetUpdateProperties object itself.
*/
public VirtualMachineScaleSetUpdateProperties withProximityPlacementGroup(SubResource proximityPlacementGroup) {
this.proximityPlacementGroup = proximityPlacementGroup;
return this;
}
/**
* Get the priorityMixPolicy property: Specifies the desired targets for mixing Spot and Regular priority VMs within
* the same VMSS Flex instance.
*
* @return the priorityMixPolicy value.
*/
public PriorityMixPolicy priorityMixPolicy() {
return this.priorityMixPolicy;
}
/**
* Set the priorityMixPolicy property: Specifies the desired targets for mixing Spot and Regular priority VMs within
* the same VMSS Flex instance.
*
* @param priorityMixPolicy the priorityMixPolicy value to set.
* @return the VirtualMachineScaleSetUpdateProperties object itself.
*/
public VirtualMachineScaleSetUpdateProperties withPriorityMixPolicy(PriorityMixPolicy priorityMixPolicy) {
this.priorityMixPolicy = priorityMixPolicy;
return this;
}
/**
* Get the spotRestorePolicy property: Specifies the Spot Restore properties for the virtual machine scale set.
*
* @return the spotRestorePolicy value.
*/
public SpotRestorePolicy spotRestorePolicy() {
return this.spotRestorePolicy;
}
/**
* Set the spotRestorePolicy property: Specifies the Spot Restore properties for the virtual machine scale set.
*
* @param spotRestorePolicy the spotRestorePolicy value to set.
* @return the VirtualMachineScaleSetUpdateProperties object itself.
*/
public VirtualMachineScaleSetUpdateProperties withSpotRestorePolicy(SpotRestorePolicy spotRestorePolicy) {
this.spotRestorePolicy = spotRestorePolicy;
return this;
}
/**
* Get the resiliencyPolicy property: Policy for Resiliency.
*
* @return the resiliencyPolicy value.
*/
public ResiliencyPolicy resiliencyPolicy() {
return this.resiliencyPolicy;
}
/**
* Set the resiliencyPolicy property: Policy for Resiliency.
*
* @param resiliencyPolicy the resiliencyPolicy value to set.
* @return the VirtualMachineScaleSetUpdateProperties object itself.
*/
public VirtualMachineScaleSetUpdateProperties withResiliencyPolicy(ResiliencyPolicy resiliencyPolicy) {
this.resiliencyPolicy = resiliencyPolicy;
return this;
}
/**
* Get the zonalPlatformFaultDomainAlignMode property: Specifies the align mode between Virtual Machine Scale Set
* compute and storage Fault Domain count.
*
* @return the zonalPlatformFaultDomainAlignMode value.
*/
public ZonalPlatformFaultDomainAlignMode zonalPlatformFaultDomainAlignMode() {
return this.zonalPlatformFaultDomainAlignMode;
}
/**
* Set the zonalPlatformFaultDomainAlignMode property: Specifies the align mode between Virtual Machine Scale Set
* compute and storage Fault Domain count.
*
* @param zonalPlatformFaultDomainAlignMode the zonalPlatformFaultDomainAlignMode value to set.
* @return the VirtualMachineScaleSetUpdateProperties object itself.
*/
public VirtualMachineScaleSetUpdateProperties
withZonalPlatformFaultDomainAlignMode(ZonalPlatformFaultDomainAlignMode zonalPlatformFaultDomainAlignMode) {
this.zonalPlatformFaultDomainAlignMode = zonalPlatformFaultDomainAlignMode;
return this;
}
/**
* Get the skuProfile property: Specifies the sku profile for the virtual machine scale set.
*
* @return the skuProfile value.
*/
public SkuProfile skuProfile() {
return this.skuProfile;
}
/**
* Set the skuProfile property: Specifies the sku profile for the virtual machine scale set.
*
* @param skuProfile the skuProfile value to set.
* @return the VirtualMachineScaleSetUpdateProperties object itself.
*/
public VirtualMachineScaleSetUpdateProperties withSkuProfile(SkuProfile skuProfile) {
this.skuProfile = skuProfile;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (upgradePolicy() != null) {
upgradePolicy().validate();
}
if (automaticRepairsPolicy() != null) {
automaticRepairsPolicy().validate();
}
if (virtualMachineProfile() != null) {
virtualMachineProfile().validate();
}
if (additionalCapabilities() != null) {
additionalCapabilities().validate();
}
if (scaleInPolicy() != null) {
scaleInPolicy().validate();
}
if (priorityMixPolicy() != null) {
priorityMixPolicy().validate();
}
if (spotRestorePolicy() != null) {
spotRestorePolicy().validate();
}
if (resiliencyPolicy() != null) {
resiliencyPolicy().validate();
}
if (skuProfile() != null) {
skuProfile().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("upgradePolicy", this.upgradePolicy);
jsonWriter.writeJsonField("automaticRepairsPolicy", this.automaticRepairsPolicy);
jsonWriter.writeJsonField("virtualMachineProfile", this.virtualMachineProfile);
jsonWriter.writeBooleanField("overprovision", this.overprovision);
jsonWriter.writeBooleanField("doNotRunExtensionsOnOverprovisionedVMs",
this.doNotRunExtensionsOnOverprovisionedVMs);
jsonWriter.writeBooleanField("singlePlacementGroup", this.singlePlacementGroup);
jsonWriter.writeJsonField("additionalCapabilities", this.additionalCapabilities);
jsonWriter.writeJsonField("scaleInPolicy", this.scaleInPolicy);
jsonWriter.writeJsonField("proximityPlacementGroup", this.proximityPlacementGroup);
jsonWriter.writeJsonField("priorityMixPolicy", this.priorityMixPolicy);
jsonWriter.writeJsonField("spotRestorePolicy", this.spotRestorePolicy);
jsonWriter.writeJsonField("resiliencyPolicy", this.resiliencyPolicy);
jsonWriter.writeStringField("zonalPlatformFaultDomainAlignMode",
this.zonalPlatformFaultDomainAlignMode == null ? null : this.zonalPlatformFaultDomainAlignMode.toString());
jsonWriter.writeJsonField("skuProfile", this.skuProfile);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of VirtualMachineScaleSetUpdateProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of VirtualMachineScaleSetUpdateProperties if the JsonReader was pointing to an instance of
* it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the VirtualMachineScaleSetUpdateProperties.
*/
public static VirtualMachineScaleSetUpdateProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
VirtualMachineScaleSetUpdateProperties deserializedVirtualMachineScaleSetUpdateProperties
= new VirtualMachineScaleSetUpdateProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("upgradePolicy".equals(fieldName)) {
deserializedVirtualMachineScaleSetUpdateProperties.upgradePolicy = UpgradePolicy.fromJson(reader);
} else if ("automaticRepairsPolicy".equals(fieldName)) {
deserializedVirtualMachineScaleSetUpdateProperties.automaticRepairsPolicy
= AutomaticRepairsPolicy.fromJson(reader);
} else if ("virtualMachineProfile".equals(fieldName)) {
deserializedVirtualMachineScaleSetUpdateProperties.virtualMachineProfile
= VirtualMachineScaleSetUpdateVMProfile.fromJson(reader);
} else if ("overprovision".equals(fieldName)) {
deserializedVirtualMachineScaleSetUpdateProperties.overprovision
= reader.getNullable(JsonReader::getBoolean);
} else if ("doNotRunExtensionsOnOverprovisionedVMs".equals(fieldName)) {
deserializedVirtualMachineScaleSetUpdateProperties.doNotRunExtensionsOnOverprovisionedVMs
= reader.getNullable(JsonReader::getBoolean);
} else if ("singlePlacementGroup".equals(fieldName)) {
deserializedVirtualMachineScaleSetUpdateProperties.singlePlacementGroup
= reader.getNullable(JsonReader::getBoolean);
} else if ("additionalCapabilities".equals(fieldName)) {
deserializedVirtualMachineScaleSetUpdateProperties.additionalCapabilities
= AdditionalCapabilities.fromJson(reader);
} else if ("scaleInPolicy".equals(fieldName)) {
deserializedVirtualMachineScaleSetUpdateProperties.scaleInPolicy = ScaleInPolicy.fromJson(reader);
} else if ("proximityPlacementGroup".equals(fieldName)) {
deserializedVirtualMachineScaleSetUpdateProperties.proximityPlacementGroup
= SubResource.fromJson(reader);
} else if ("priorityMixPolicy".equals(fieldName)) {
deserializedVirtualMachineScaleSetUpdateProperties.priorityMixPolicy
= PriorityMixPolicy.fromJson(reader);
} else if ("spotRestorePolicy".equals(fieldName)) {
deserializedVirtualMachineScaleSetUpdateProperties.spotRestorePolicy
= SpotRestorePolicy.fromJson(reader);
} else if ("resiliencyPolicy".equals(fieldName)) {
deserializedVirtualMachineScaleSetUpdateProperties.resiliencyPolicy
= ResiliencyPolicy.fromJson(reader);
} else if ("zonalPlatformFaultDomainAlignMode".equals(fieldName)) {
deserializedVirtualMachineScaleSetUpdateProperties.zonalPlatformFaultDomainAlignMode
= ZonalPlatformFaultDomainAlignMode.fromString(reader.getString());
} else if ("skuProfile".equals(fieldName)) {
deserializedVirtualMachineScaleSetUpdateProperties.skuProfile = SkuProfile.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedVirtualMachineScaleSetUpdateProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy