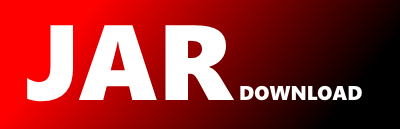
com.azure.resourcemanager.compute.fluent.models.VirtualMachineScaleSetVMInstanceViewInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-compute Show documentation
Show all versions of azure-resourcemanager-compute Show documentation
This package contains Microsoft Azure Compute Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.compute.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.compute.models.BootDiagnosticsInstanceView;
import com.azure.resourcemanager.compute.models.DiskInstanceView;
import com.azure.resourcemanager.compute.models.HyperVGeneration;
import com.azure.resourcemanager.compute.models.InstanceViewStatus;
import com.azure.resourcemanager.compute.models.MaintenanceRedeployStatus;
import com.azure.resourcemanager.compute.models.VirtualMachineAgentInstanceView;
import com.azure.resourcemanager.compute.models.VirtualMachineExtensionInstanceView;
import com.azure.resourcemanager.compute.models.VirtualMachineHealthStatus;
import java.io.IOException;
import java.util.List;
/**
* The instance view of a virtual machine scale set VM.
*/
@Fluent
public final class VirtualMachineScaleSetVMInstanceViewInner
implements JsonSerializable {
/*
* The Update Domain count.
*/
private Integer platformUpdateDomain;
/*
* The Fault Domain count.
*/
private Integer platformFaultDomain;
/*
* The Remote desktop certificate thumbprint.
*/
private String rdpThumbPrint;
/*
* The VM Agent running on the virtual machine.
*/
private VirtualMachineAgentInstanceView vmAgent;
/*
* The Maintenance Operation status on the virtual machine.
*/
private MaintenanceRedeployStatus maintenanceRedeployStatus;
/*
* The disks information.
*/
private List disks;
/*
* The extensions information.
*/
private List extensions;
/*
* The health status for the VM.
*/
private VirtualMachineHealthStatus vmHealth;
/*
* Boot Diagnostics is a debugging feature which allows you to view Console Output and Screenshot to diagnose VM
* status. You can easily view the output of your console log. Azure also enables you to see a screenshot of the VM
* from the hypervisor.
*/
private BootDiagnosticsInstanceView bootDiagnostics;
/*
* The resource status information.
*/
private List statuses;
/*
* Resource id of the dedicated host, on which the virtual machine is allocated through automatic placement, when
* the virtual machine is associated with a dedicated host group that has automatic placement enabled. Minimum
* api-version: 2020-06-01.
*/
private String assignedHost;
/*
* The placement group in which the VM is running. If the VM is deallocated it will not have a placementGroupId.
*/
private String placementGroupId;
/*
* Specifies the host OS name of the virtual machine.
This name cannot be updated after the VM is created.
*
**Max-length (Windows):** 15 characters
**Max-length (Linux):** 64 characters.
For
* naming conventions and restrictions see [Azure infrastructure services implementation
* guidelines](https://docs.microsoft.com/azure/virtual-machines/virtual-machines-linux-infrastructure-subscription-
* accounts-guidelines?toc=%2fazure%2fvirtual-machines%2flinux%2ftoc.json#1-naming-conventions).
*/
private String computerName;
/*
* The Operating System running on the hybrid machine.
*/
private String osName;
/*
* The version of Operating System running on the hybrid machine.
*/
private String osVersion;
/*
* The hypervisor generation of the Virtual Machine [V1, V2]
*/
private HyperVGeneration hyperVGeneration;
/**
* Creates an instance of VirtualMachineScaleSetVMInstanceViewInner class.
*/
public VirtualMachineScaleSetVMInstanceViewInner() {
}
/**
* Get the platformUpdateDomain property: The Update Domain count.
*
* @return the platformUpdateDomain value.
*/
public Integer platformUpdateDomain() {
return this.platformUpdateDomain;
}
/**
* Set the platformUpdateDomain property: The Update Domain count.
*
* @param platformUpdateDomain the platformUpdateDomain value to set.
* @return the VirtualMachineScaleSetVMInstanceViewInner object itself.
*/
public VirtualMachineScaleSetVMInstanceViewInner withPlatformUpdateDomain(Integer platformUpdateDomain) {
this.platformUpdateDomain = platformUpdateDomain;
return this;
}
/**
* Get the platformFaultDomain property: The Fault Domain count.
*
* @return the platformFaultDomain value.
*/
public Integer platformFaultDomain() {
return this.platformFaultDomain;
}
/**
* Set the platformFaultDomain property: The Fault Domain count.
*
* @param platformFaultDomain the platformFaultDomain value to set.
* @return the VirtualMachineScaleSetVMInstanceViewInner object itself.
*/
public VirtualMachineScaleSetVMInstanceViewInner withPlatformFaultDomain(Integer platformFaultDomain) {
this.platformFaultDomain = platformFaultDomain;
return this;
}
/**
* Get the rdpThumbPrint property: The Remote desktop certificate thumbprint.
*
* @return the rdpThumbPrint value.
*/
public String rdpThumbPrint() {
return this.rdpThumbPrint;
}
/**
* Set the rdpThumbPrint property: The Remote desktop certificate thumbprint.
*
* @param rdpThumbPrint the rdpThumbPrint value to set.
* @return the VirtualMachineScaleSetVMInstanceViewInner object itself.
*/
public VirtualMachineScaleSetVMInstanceViewInner withRdpThumbPrint(String rdpThumbPrint) {
this.rdpThumbPrint = rdpThumbPrint;
return this;
}
/**
* Get the vmAgent property: The VM Agent running on the virtual machine.
*
* @return the vmAgent value.
*/
public VirtualMachineAgentInstanceView vmAgent() {
return this.vmAgent;
}
/**
* Set the vmAgent property: The VM Agent running on the virtual machine.
*
* @param vmAgent the vmAgent value to set.
* @return the VirtualMachineScaleSetVMInstanceViewInner object itself.
*/
public VirtualMachineScaleSetVMInstanceViewInner withVmAgent(VirtualMachineAgentInstanceView vmAgent) {
this.vmAgent = vmAgent;
return this;
}
/**
* Get the maintenanceRedeployStatus property: The Maintenance Operation status on the virtual machine.
*
* @return the maintenanceRedeployStatus value.
*/
public MaintenanceRedeployStatus maintenanceRedeployStatus() {
return this.maintenanceRedeployStatus;
}
/**
* Set the maintenanceRedeployStatus property: The Maintenance Operation status on the virtual machine.
*
* @param maintenanceRedeployStatus the maintenanceRedeployStatus value to set.
* @return the VirtualMachineScaleSetVMInstanceViewInner object itself.
*/
public VirtualMachineScaleSetVMInstanceViewInner
withMaintenanceRedeployStatus(MaintenanceRedeployStatus maintenanceRedeployStatus) {
this.maintenanceRedeployStatus = maintenanceRedeployStatus;
return this;
}
/**
* Get the disks property: The disks information.
*
* @return the disks value.
*/
public List disks() {
return this.disks;
}
/**
* Set the disks property: The disks information.
*
* @param disks the disks value to set.
* @return the VirtualMachineScaleSetVMInstanceViewInner object itself.
*/
public VirtualMachineScaleSetVMInstanceViewInner withDisks(List disks) {
this.disks = disks;
return this;
}
/**
* Get the extensions property: The extensions information.
*
* @return the extensions value.
*/
public List extensions() {
return this.extensions;
}
/**
* Set the extensions property: The extensions information.
*
* @param extensions the extensions value to set.
* @return the VirtualMachineScaleSetVMInstanceViewInner object itself.
*/
public VirtualMachineScaleSetVMInstanceViewInner
withExtensions(List extensions) {
this.extensions = extensions;
return this;
}
/**
* Get the vmHealth property: The health status for the VM.
*
* @return the vmHealth value.
*/
public VirtualMachineHealthStatus vmHealth() {
return this.vmHealth;
}
/**
* Get the bootDiagnostics property: Boot Diagnostics is a debugging feature which allows you to view Console Output
* and Screenshot to diagnose VM status. You can easily view the output of your console log. Azure also enables you
* to see a screenshot of the VM from the hypervisor.
*
* @return the bootDiagnostics value.
*/
public BootDiagnosticsInstanceView bootDiagnostics() {
return this.bootDiagnostics;
}
/**
* Set the bootDiagnostics property: Boot Diagnostics is a debugging feature which allows you to view Console Output
* and Screenshot to diagnose VM status. You can easily view the output of your console log. Azure also enables you
* to see a screenshot of the VM from the hypervisor.
*
* @param bootDiagnostics the bootDiagnostics value to set.
* @return the VirtualMachineScaleSetVMInstanceViewInner object itself.
*/
public VirtualMachineScaleSetVMInstanceViewInner withBootDiagnostics(BootDiagnosticsInstanceView bootDiagnostics) {
this.bootDiagnostics = bootDiagnostics;
return this;
}
/**
* Get the statuses property: The resource status information.
*
* @return the statuses value.
*/
public List statuses() {
return this.statuses;
}
/**
* Set the statuses property: The resource status information.
*
* @param statuses the statuses value to set.
* @return the VirtualMachineScaleSetVMInstanceViewInner object itself.
*/
public VirtualMachineScaleSetVMInstanceViewInner withStatuses(List statuses) {
this.statuses = statuses;
return this;
}
/**
* Get the assignedHost property: Resource id of the dedicated host, on which the virtual machine is allocated
* through automatic placement, when the virtual machine is associated with a dedicated host group that has
* automatic placement enabled. Minimum api-version: 2020-06-01.
*
* @return the assignedHost value.
*/
public String assignedHost() {
return this.assignedHost;
}
/**
* Get the placementGroupId property: The placement group in which the VM is running. If the VM is deallocated it
* will not have a placementGroupId.
*
* @return the placementGroupId value.
*/
public String placementGroupId() {
return this.placementGroupId;
}
/**
* Set the placementGroupId property: The placement group in which the VM is running. If the VM is deallocated it
* will not have a placementGroupId.
*
* @param placementGroupId the placementGroupId value to set.
* @return the VirtualMachineScaleSetVMInstanceViewInner object itself.
*/
public VirtualMachineScaleSetVMInstanceViewInner withPlacementGroupId(String placementGroupId) {
this.placementGroupId = placementGroupId;
return this;
}
/**
* Get the computerName property: Specifies the host OS name of the virtual machine. <br><br> This name
* cannot be updated after the VM is created. <br><br> **Max-length (Windows):** 15 characters
* <br><br> **Max-length (Linux):** 64 characters. <br><br> For naming conventions and
* restrictions see [Azure infrastructure services implementation
* guidelines](https://docs.microsoft.com/azure/virtual-machines/virtual-machines-linux-infrastructure-subscription-accounts-guidelines?toc=%2fazure%2fvirtual-machines%2flinux%2ftoc.json#1-naming-conventions).
*
* @return the computerName value.
*/
public String computerName() {
return this.computerName;
}
/**
* Set the computerName property: Specifies the host OS name of the virtual machine. <br><br> This name
* cannot be updated after the VM is created. <br><br> **Max-length (Windows):** 15 characters
* <br><br> **Max-length (Linux):** 64 characters. <br><br> For naming conventions and
* restrictions see [Azure infrastructure services implementation
* guidelines](https://docs.microsoft.com/azure/virtual-machines/virtual-machines-linux-infrastructure-subscription-accounts-guidelines?toc=%2fazure%2fvirtual-machines%2flinux%2ftoc.json#1-naming-conventions).
*
* @param computerName the computerName value to set.
* @return the VirtualMachineScaleSetVMInstanceViewInner object itself.
*/
public VirtualMachineScaleSetVMInstanceViewInner withComputerName(String computerName) {
this.computerName = computerName;
return this;
}
/**
* Get the osName property: The Operating System running on the hybrid machine.
*
* @return the osName value.
*/
public String osName() {
return this.osName;
}
/**
* Set the osName property: The Operating System running on the hybrid machine.
*
* @param osName the osName value to set.
* @return the VirtualMachineScaleSetVMInstanceViewInner object itself.
*/
public VirtualMachineScaleSetVMInstanceViewInner withOsName(String osName) {
this.osName = osName;
return this;
}
/**
* Get the osVersion property: The version of Operating System running on the hybrid machine.
*
* @return the osVersion value.
*/
public String osVersion() {
return this.osVersion;
}
/**
* Set the osVersion property: The version of Operating System running on the hybrid machine.
*
* @param osVersion the osVersion value to set.
* @return the VirtualMachineScaleSetVMInstanceViewInner object itself.
*/
public VirtualMachineScaleSetVMInstanceViewInner withOsVersion(String osVersion) {
this.osVersion = osVersion;
return this;
}
/**
* Get the hyperVGeneration property: The hypervisor generation of the Virtual Machine [V1, V2].
*
* @return the hyperVGeneration value.
*/
public HyperVGeneration hyperVGeneration() {
return this.hyperVGeneration;
}
/**
* Set the hyperVGeneration property: The hypervisor generation of the Virtual Machine [V1, V2].
*
* @param hyperVGeneration the hyperVGeneration value to set.
* @return the VirtualMachineScaleSetVMInstanceViewInner object itself.
*/
public VirtualMachineScaleSetVMInstanceViewInner withHyperVGeneration(HyperVGeneration hyperVGeneration) {
this.hyperVGeneration = hyperVGeneration;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (vmAgent() != null) {
vmAgent().validate();
}
if (maintenanceRedeployStatus() != null) {
maintenanceRedeployStatus().validate();
}
if (disks() != null) {
disks().forEach(e -> e.validate());
}
if (extensions() != null) {
extensions().forEach(e -> e.validate());
}
if (vmHealth() != null) {
vmHealth().validate();
}
if (bootDiagnostics() != null) {
bootDiagnostics().validate();
}
if (statuses() != null) {
statuses().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeNumberField("platformUpdateDomain", this.platformUpdateDomain);
jsonWriter.writeNumberField("platformFaultDomain", this.platformFaultDomain);
jsonWriter.writeStringField("rdpThumbPrint", this.rdpThumbPrint);
jsonWriter.writeJsonField("vmAgent", this.vmAgent);
jsonWriter.writeJsonField("maintenanceRedeployStatus", this.maintenanceRedeployStatus);
jsonWriter.writeArrayField("disks", this.disks, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("extensions", this.extensions, (writer, element) -> writer.writeJson(element));
jsonWriter.writeJsonField("bootDiagnostics", this.bootDiagnostics);
jsonWriter.writeArrayField("statuses", this.statuses, (writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("placementGroupId", this.placementGroupId);
jsonWriter.writeStringField("computerName", this.computerName);
jsonWriter.writeStringField("osName", this.osName);
jsonWriter.writeStringField("osVersion", this.osVersion);
jsonWriter.writeStringField("hyperVGeneration",
this.hyperVGeneration == null ? null : this.hyperVGeneration.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of VirtualMachineScaleSetVMInstanceViewInner from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of VirtualMachineScaleSetVMInstanceViewInner if the JsonReader was pointing to an instance of
* it, or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the VirtualMachineScaleSetVMInstanceViewInner.
*/
public static VirtualMachineScaleSetVMInstanceViewInner fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
VirtualMachineScaleSetVMInstanceViewInner deserializedVirtualMachineScaleSetVMInstanceViewInner
= new VirtualMachineScaleSetVMInstanceViewInner();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("platformUpdateDomain".equals(fieldName)) {
deserializedVirtualMachineScaleSetVMInstanceViewInner.platformUpdateDomain
= reader.getNullable(JsonReader::getInt);
} else if ("platformFaultDomain".equals(fieldName)) {
deserializedVirtualMachineScaleSetVMInstanceViewInner.platformFaultDomain
= reader.getNullable(JsonReader::getInt);
} else if ("rdpThumbPrint".equals(fieldName)) {
deserializedVirtualMachineScaleSetVMInstanceViewInner.rdpThumbPrint = reader.getString();
} else if ("vmAgent".equals(fieldName)) {
deserializedVirtualMachineScaleSetVMInstanceViewInner.vmAgent
= VirtualMachineAgentInstanceView.fromJson(reader);
} else if ("maintenanceRedeployStatus".equals(fieldName)) {
deserializedVirtualMachineScaleSetVMInstanceViewInner.maintenanceRedeployStatus
= MaintenanceRedeployStatus.fromJson(reader);
} else if ("disks".equals(fieldName)) {
List disks = reader.readArray(reader1 -> DiskInstanceView.fromJson(reader1));
deserializedVirtualMachineScaleSetVMInstanceViewInner.disks = disks;
} else if ("extensions".equals(fieldName)) {
List extensions
= reader.readArray(reader1 -> VirtualMachineExtensionInstanceView.fromJson(reader1));
deserializedVirtualMachineScaleSetVMInstanceViewInner.extensions = extensions;
} else if ("vmHealth".equals(fieldName)) {
deserializedVirtualMachineScaleSetVMInstanceViewInner.vmHealth
= VirtualMachineHealthStatus.fromJson(reader);
} else if ("bootDiagnostics".equals(fieldName)) {
deserializedVirtualMachineScaleSetVMInstanceViewInner.bootDiagnostics
= BootDiagnosticsInstanceView.fromJson(reader);
} else if ("statuses".equals(fieldName)) {
List statuses
= reader.readArray(reader1 -> InstanceViewStatus.fromJson(reader1));
deserializedVirtualMachineScaleSetVMInstanceViewInner.statuses = statuses;
} else if ("assignedHost".equals(fieldName)) {
deserializedVirtualMachineScaleSetVMInstanceViewInner.assignedHost = reader.getString();
} else if ("placementGroupId".equals(fieldName)) {
deserializedVirtualMachineScaleSetVMInstanceViewInner.placementGroupId = reader.getString();
} else if ("computerName".equals(fieldName)) {
deserializedVirtualMachineScaleSetVMInstanceViewInner.computerName = reader.getString();
} else if ("osName".equals(fieldName)) {
deserializedVirtualMachineScaleSetVMInstanceViewInner.osName = reader.getString();
} else if ("osVersion".equals(fieldName)) {
deserializedVirtualMachineScaleSetVMInstanceViewInner.osVersion = reader.getString();
} else if ("hyperVGeneration".equals(fieldName)) {
deserializedVirtualMachineScaleSetVMInstanceViewInner.hyperVGeneration
= HyperVGeneration.fromString(reader.getString());
} else {
reader.skipChildren();
}
}
return deserializedVirtualMachineScaleSetVMInstanceViewInner;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy