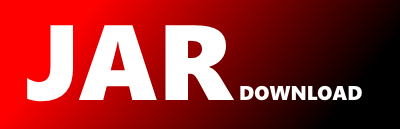
com.azure.resourcemanager.compute.implementation.VirtualMachinesClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-compute Show documentation
Show all versions of azure-resourcemanager-compute Show documentation
This package contains Microsoft Azure Compute Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.compute.implementation;
import com.azure.core.annotation.BodyParam;
import com.azure.core.annotation.Delete;
import com.azure.core.annotation.ExpectedResponses;
import com.azure.core.annotation.Get;
import com.azure.core.annotation.HeaderParam;
import com.azure.core.annotation.Headers;
import com.azure.core.annotation.Host;
import com.azure.core.annotation.HostParam;
import com.azure.core.annotation.Patch;
import com.azure.core.annotation.PathParam;
import com.azure.core.annotation.Post;
import com.azure.core.annotation.Put;
import com.azure.core.annotation.QueryParam;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceInterface;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.annotation.UnexpectedResponseExceptionType;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.PagedResponse;
import com.azure.core.http.rest.PagedResponseBase;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.RestProxy;
import com.azure.core.management.exception.ManagementException;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.FluxUtil;
import com.azure.core.util.polling.PollerFlux;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.compute.fluent.VirtualMachinesClient;
import com.azure.resourcemanager.compute.fluent.models.RetrieveBootDiagnosticsDataResultInner;
import com.azure.resourcemanager.compute.fluent.models.RunCommandResultInner;
import com.azure.resourcemanager.compute.fluent.models.VirtualMachineAssessPatchesResultInner;
import com.azure.resourcemanager.compute.fluent.models.VirtualMachineCaptureResultInner;
import com.azure.resourcemanager.compute.fluent.models.VirtualMachineInner;
import com.azure.resourcemanager.compute.fluent.models.VirtualMachineInstallPatchesResultInner;
import com.azure.resourcemanager.compute.fluent.models.VirtualMachineInstanceViewInner;
import com.azure.resourcemanager.compute.fluent.models.VirtualMachineSizeInner;
import com.azure.resourcemanager.compute.fluent.models.VirtualMachineUpdateInner;
import com.azure.resourcemanager.compute.models.ApiErrorException;
import com.azure.resourcemanager.compute.models.AttachDetachDataDisksRequest;
import com.azure.resourcemanager.compute.models.ExpandTypeForListVMs;
import com.azure.resourcemanager.compute.models.ExpandTypesForListVMs;
import com.azure.resourcemanager.compute.models.InstanceViewTypes;
import com.azure.resourcemanager.compute.models.RunCommandInput;
import com.azure.resourcemanager.compute.models.StorageProfile;
import com.azure.resourcemanager.compute.models.VirtualMachineCaptureParameters;
import com.azure.resourcemanager.compute.models.VirtualMachineInstallPatchesParameters;
import com.azure.resourcemanager.compute.models.VirtualMachineListResult;
import com.azure.resourcemanager.compute.models.VirtualMachineReimageParameters;
import com.azure.resourcemanager.compute.models.VirtualMachineSizeListResult;
import com.azure.resourcemanager.resources.fluentcore.collection.InnerSupportsDelete;
import com.azure.resourcemanager.resources.fluentcore.collection.InnerSupportsGet;
import com.azure.resourcemanager.resources.fluentcore.collection.InnerSupportsListing;
import java.nio.ByteBuffer;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* An instance of this class provides access to all the operations defined in VirtualMachinesClient.
*/
public final class VirtualMachinesClientImpl implements InnerSupportsGet,
InnerSupportsListing, InnerSupportsDelete, VirtualMachinesClient {
/**
* The proxy service used to perform REST calls.
*/
private final VirtualMachinesService service;
/**
* The service client containing this operation class.
*/
private final ComputeManagementClientImpl client;
/**
* Initializes an instance of VirtualMachinesClientImpl.
*
* @param client the instance of the service client containing this operation class.
*/
VirtualMachinesClientImpl(ComputeManagementClientImpl client) {
this.service
= RestProxy.create(VirtualMachinesService.class, client.getHttpPipeline(), client.getSerializerAdapter());
this.client = client;
}
/**
* The interface defining all the services for ComputeManagementClientVirtualMachines to be used by the proxy
* service to perform REST calls.
*/
@Host("{$host}")
@ServiceInterface(name = "ComputeManagementCli")
public interface VirtualMachinesService {
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/providers/Microsoft.Compute/locations/{location}/virtualMachines")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono> listByLocation(@HostParam("$host") String endpoint,
@PathParam("location") String location, @QueryParam("api-version") String apiVersion,
@PathParam("subscriptionId") String subscriptionId, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/capture")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> capture(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@BodyParam("application/json") VirtualMachineCaptureParameters parameters,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Put("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}")
@ExpectedResponses({ 200, 201 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> createOrUpdate(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("If-Match") String ifMatch, @HeaderParam("If-None-Match") String ifNoneMatch,
@BodyParam("application/json") VirtualMachineInner parameters, @HeaderParam("Accept") String accept,
Context context);
@Headers({ "Content-Type: application/json" })
@Patch("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> update(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("If-Match") String ifMatch, @HeaderParam("If-None-Match") String ifNoneMatch,
@BodyParam("application/json") VirtualMachineUpdateInner parameters, @HeaderParam("Accept") String accept,
Context context);
@Headers({ "Content-Type: application/json" })
@Delete("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}")
@ExpectedResponses({ 200, 202, 204 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> delete(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("forceDeletion") Boolean forceDeletion, @QueryParam("api-version") String apiVersion,
@PathParam("subscriptionId") String subscriptionId, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono> getByResourceGroup(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("$expand") InstanceViewTypes expand, @QueryParam("api-version") String apiVersion,
@PathParam("subscriptionId") String subscriptionId, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/instanceView")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono> instanceView(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/convertToManagedDisks")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> convertToManagedDisks(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/deallocate")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> deallocate(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("hibernate") Boolean hibernate, @QueryParam("api-version") String apiVersion,
@PathParam("subscriptionId") String subscriptionId, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/generalize")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono> generalize(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono> listByResourceGroup(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @QueryParam("$filter") String filter,
@QueryParam("$expand") ExpandTypeForListVMs expand, @QueryParam("api-version") String apiVersion,
@PathParam("subscriptionId") String subscriptionId, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/providers/Microsoft.Compute/virtualMachines")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono> list(@HostParam("$host") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@QueryParam("statusOnly") String statusOnly, @QueryParam("$filter") String filter,
@QueryParam("$expand") ExpandTypesForListVMs expand, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/vmSizes")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono> listAvailableSizes(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/powerOff")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> powerOff(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("skipShutdown") Boolean skipShutdown, @QueryParam("api-version") String apiVersion,
@PathParam("subscriptionId") String subscriptionId, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/reapply")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> reapply(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/restart")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> restart(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/start")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> start(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/redeploy")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> redeploy(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/reimage")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> reimage(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@BodyParam("application/json") VirtualMachineReimageParameters parameters,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/retrieveBootDiagnosticsData")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono> retrieveBootDiagnosticsData(
@HostParam("$host") String endpoint, @PathParam("resourceGroupName") String resourceGroupName,
@PathParam("vmName") String vmName,
@QueryParam("sasUriExpirationTimeInMinutes") Integer sasUriExpirationTimeInMinutes,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/performMaintenance")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> performMaintenance(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/simulateEviction")
@ExpectedResponses({ 204 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono> simulateEviction(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/assessPatches")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> assessPatches(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/installPatches")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> installPatches(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@BodyParam("application/json") VirtualMachineInstallPatchesParameters installPatchesInput,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/attachDetachDataDisks")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono>> attachDetachDataDisks(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@PathParam("subscriptionId") String subscriptionId, @QueryParam("api-version") String apiVersion,
@BodyParam("application/json") AttachDetachDataDisksRequest parameters,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachines/{vmName}/runCommand")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> runCommand(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("vmName") String vmName,
@QueryParam("api-version") String apiVersion, @PathParam("subscriptionId") String subscriptionId,
@BodyParam("application/json") RunCommandInput parameters, @HeaderParam("Accept") String accept,
Context context);
@Headers({ "Content-Type: application/json" })
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono> listByLocationNext(
@PathParam(value = "nextLink", encoded = true) String nextLink, @HostParam("$host") String endpoint,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono> listNext(
@PathParam(value = "nextLink", encoded = true) String nextLink, @HostParam("$host") String endpoint,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ApiErrorException.class)
Mono> listAllNext(
@PathParam(value = "nextLink", encoded = true) String nextLink, @HostParam("$host") String endpoint,
@HeaderParam("Accept") String accept, Context context);
}
/**
* Gets all the virtual machines under the specified subscription for the specified location.
*
* @param location The location for which virtual machines under the subscription are queried.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the virtual machines under the specified subscription for the specified location along with
* {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByLocationSinglePageAsync(String location) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (location == null) {
return Mono.error(new IllegalArgumentException("Parameter location is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listByLocation(this.client.getEndpoint(), location, apiVersion,
this.client.getSubscriptionId(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Gets all the virtual machines under the specified subscription for the specified location.
*
* @param location The location for which virtual machines under the subscription are queried.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the virtual machines under the specified subscription for the specified location along with
* {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByLocationSinglePageAsync(String location, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (location == null) {
return Mono.error(new IllegalArgumentException("Parameter location is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listByLocation(this.client.getEndpoint(), location, apiVersion, this.client.getSubscriptionId(), accept,
context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Gets all the virtual machines under the specified subscription for the specified location.
*
* @param location The location for which virtual machines under the subscription are queried.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the virtual machines under the specified subscription for the specified location as paginated
* response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listByLocationAsync(String location) {
return new PagedFlux<>(() -> listByLocationSinglePageAsync(location),
nextLink -> listByLocationNextSinglePageAsync(nextLink));
}
/**
* Gets all the virtual machines under the specified subscription for the specified location.
*
* @param location The location for which virtual machines under the subscription are queried.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the virtual machines under the specified subscription for the specified location as paginated
* response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listByLocationAsync(String location, Context context) {
return new PagedFlux<>(() -> listByLocationSinglePageAsync(location, context),
nextLink -> listByLocationNextSinglePageAsync(nextLink, context));
}
/**
* Gets all the virtual machines under the specified subscription for the specified location.
*
* @param location The location for which virtual machines under the subscription are queried.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the virtual machines under the specified subscription for the specified location as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listByLocation(String location) {
return new PagedIterable<>(listByLocationAsync(location));
}
/**
* Gets all the virtual machines under the specified subscription for the specified location.
*
* @param location The location for which virtual machines under the subscription are queried.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return all the virtual machines under the specified subscription for the specified location as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listByLocation(String location, Context context) {
return new PagedIterable<>(listByLocationAsync(location, context));
}
/**
* Captures the VM by copying virtual hard disks of the VM and outputs a template that can be used to create similar
* VMs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Capture Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return output of virtual machine capture operation along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> captureWithResponseAsync(String resourceGroupName, String vmName,
VirtualMachineCaptureParameters parameters) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (parameters == null) {
return Mono.error(new IllegalArgumentException("Parameter parameters is required and cannot be null."));
} else {
parameters.validate();
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.capture(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), parameters, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Captures the VM by copying virtual hard disks of the VM and outputs a template that can be used to create similar
* VMs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Capture Virtual Machine operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return output of virtual machine capture operation along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> captureWithResponseAsync(String resourceGroupName, String vmName,
VirtualMachineCaptureParameters parameters, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (parameters == null) {
return Mono.error(new IllegalArgumentException("Parameter parameters is required and cannot be null."));
} else {
parameters.validate();
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.capture(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), parameters, accept, context);
}
/**
* Captures the VM by copying virtual hard disks of the VM and outputs a template that can be used to create similar
* VMs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Capture Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of output of virtual machine capture operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, VirtualMachineCaptureResultInner>
beginCaptureAsync(String resourceGroupName, String vmName, VirtualMachineCaptureParameters parameters) {
Mono>> mono = captureWithResponseAsync(resourceGroupName, vmName, parameters);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), VirtualMachineCaptureResultInner.class,
VirtualMachineCaptureResultInner.class, this.client.getContext());
}
/**
* Captures the VM by copying virtual hard disks of the VM and outputs a template that can be used to create similar
* VMs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Capture Virtual Machine operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of output of virtual machine capture operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, VirtualMachineCaptureResultInner>
beginCaptureAsync(String resourceGroupName, String vmName, VirtualMachineCaptureParameters parameters,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= captureWithResponseAsync(resourceGroupName, vmName, parameters, context);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), VirtualMachineCaptureResultInner.class,
VirtualMachineCaptureResultInner.class, context);
}
/**
* Captures the VM by copying virtual hard disks of the VM and outputs a template that can be used to create similar
* VMs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Capture Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of output of virtual machine capture operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, VirtualMachineCaptureResultInner>
beginCapture(String resourceGroupName, String vmName, VirtualMachineCaptureParameters parameters) {
return this.beginCaptureAsync(resourceGroupName, vmName, parameters).getSyncPoller();
}
/**
* Captures the VM by copying virtual hard disks of the VM and outputs a template that can be used to create similar
* VMs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Capture Virtual Machine operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of output of virtual machine capture operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, VirtualMachineCaptureResultInner> beginCapture(
String resourceGroupName, String vmName, VirtualMachineCaptureParameters parameters, Context context) {
return this.beginCaptureAsync(resourceGroupName, vmName, parameters, context).getSyncPoller();
}
/**
* Captures the VM by copying virtual hard disks of the VM and outputs a template that can be used to create similar
* VMs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Capture Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return output of virtual machine capture operation on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono captureAsync(String resourceGroupName, String vmName,
VirtualMachineCaptureParameters parameters) {
return beginCaptureAsync(resourceGroupName, vmName, parameters).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Captures the VM by copying virtual hard disks of the VM and outputs a template that can be used to create similar
* VMs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Capture Virtual Machine operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return output of virtual machine capture operation on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono captureAsync(String resourceGroupName, String vmName,
VirtualMachineCaptureParameters parameters, Context context) {
return beginCaptureAsync(resourceGroupName, vmName, parameters, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Captures the VM by copying virtual hard disks of the VM and outputs a template that can be used to create similar
* VMs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Capture Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return output of virtual machine capture operation.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VirtualMachineCaptureResultInner capture(String resourceGroupName, String vmName,
VirtualMachineCaptureParameters parameters) {
return captureAsync(resourceGroupName, vmName, parameters).block();
}
/**
* Captures the VM by copying virtual hard disks of the VM and outputs a template that can be used to create similar
* VMs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Capture Virtual Machine operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return output of virtual machine capture operation.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VirtualMachineCaptureResultInner capture(String resourceGroupName, String vmName,
VirtualMachineCaptureParameters parameters, Context context) {
return captureAsync(resourceGroupName, vmName, parameters, context).block();
}
/**
* The operation to create or update a virtual machine. Please note some properties can be set only during virtual
* machine creation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Create Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> createOrUpdateWithResponseAsync(String resourceGroupName, String vmName,
VirtualMachineInner parameters, String ifMatch, String ifNoneMatch) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (parameters == null) {
return Mono.error(new IllegalArgumentException("Parameter parameters is required and cannot be null."));
} else {
parameters.validate();
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.createOrUpdate(this.client.getEndpoint(), resourceGroupName, vmName,
apiVersion, this.client.getSubscriptionId(), ifMatch, ifNoneMatch, parameters, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* The operation to create or update a virtual machine. Please note some properties can be set only during virtual
* machine creation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Create Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> createOrUpdateWithResponseAsync(String resourceGroupName, String vmName,
VirtualMachineInner parameters, String ifMatch, String ifNoneMatch, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (parameters == null) {
return Mono.error(new IllegalArgumentException("Parameter parameters is required and cannot be null."));
} else {
parameters.validate();
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.createOrUpdate(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), ifMatch, ifNoneMatch, parameters, accept, context);
}
/**
* The operation to create or update a virtual machine. Please note some properties can be set only during virtual
* machine creation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Create Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, VirtualMachineInner> beginCreateOrUpdateAsync(
String resourceGroupName, String vmName, VirtualMachineInner parameters, String ifMatch, String ifNoneMatch) {
Mono>> mono
= createOrUpdateWithResponseAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch);
return this.client.getLroResult(mono, this.client.getHttpPipeline(),
VirtualMachineInner.class, VirtualMachineInner.class, this.client.getContext());
}
/**
* The operation to create or update a virtual machine. Please note some properties can be set only during virtual
* machine creation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Create Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, VirtualMachineInner>
beginCreateOrUpdateAsync(String resourceGroupName, String vmName, VirtualMachineInner parameters) {
final String ifMatch = null;
final String ifNoneMatch = null;
Mono>> mono
= createOrUpdateWithResponseAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch);
return this.client.getLroResult(mono, this.client.getHttpPipeline(),
VirtualMachineInner.class, VirtualMachineInner.class, this.client.getContext());
}
/**
* The operation to create or update a virtual machine. Please note some properties can be set only during virtual
* machine creation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Create Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, VirtualMachineInner> beginCreateOrUpdateAsync(
String resourceGroupName, String vmName, VirtualMachineInner parameters, String ifMatch, String ifNoneMatch,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= createOrUpdateWithResponseAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(),
VirtualMachineInner.class, VirtualMachineInner.class, context);
}
/**
* The operation to create or update a virtual machine. Please note some properties can be set only during virtual
* machine creation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Create Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, VirtualMachineInner>
beginCreateOrUpdate(String resourceGroupName, String vmName, VirtualMachineInner parameters) {
final String ifMatch = null;
final String ifNoneMatch = null;
return this.beginCreateOrUpdateAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch)
.getSyncPoller();
}
/**
* The operation to create or update a virtual machine. Please note some properties can be set only during virtual
* machine creation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Create Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, VirtualMachineInner> beginCreateOrUpdate(
String resourceGroupName, String vmName, VirtualMachineInner parameters, String ifMatch, String ifNoneMatch,
Context context) {
return this.beginCreateOrUpdateAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch, context)
.getSyncPoller();
}
/**
* The operation to create or update a virtual machine. Please note some properties can be set only during virtual
* machine creation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Create Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono createOrUpdateAsync(String resourceGroupName, String vmName,
VirtualMachineInner parameters, String ifMatch, String ifNoneMatch) {
return beginCreateOrUpdateAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to create or update a virtual machine. Please note some properties can be set only during virtual
* machine creation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Create Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono createOrUpdateAsync(String resourceGroupName, String vmName,
VirtualMachineInner parameters) {
final String ifMatch = null;
final String ifNoneMatch = null;
return beginCreateOrUpdateAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to create or update a virtual machine. Please note some properties can be set only during virtual
* machine creation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Create Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono createOrUpdateAsync(String resourceGroupName, String vmName,
VirtualMachineInner parameters, String ifMatch, String ifNoneMatch, Context context) {
return beginCreateOrUpdateAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to create or update a virtual machine. Please note some properties can be set only during virtual
* machine creation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Create Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VirtualMachineInner createOrUpdate(String resourceGroupName, String vmName, VirtualMachineInner parameters) {
final String ifMatch = null;
final String ifNoneMatch = null;
return createOrUpdateAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch).block();
}
/**
* The operation to create or update a virtual machine. Please note some properties can be set only during virtual
* machine creation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Create Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VirtualMachineInner createOrUpdate(String resourceGroupName, String vmName, VirtualMachineInner parameters,
String ifMatch, String ifNoneMatch, Context context) {
return createOrUpdateAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch, context).block();
}
/**
* The operation to update a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> updateWithResponseAsync(String resourceGroupName, String vmName,
VirtualMachineUpdateInner parameters, String ifMatch, String ifNoneMatch) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (parameters == null) {
return Mono.error(new IllegalArgumentException("Parameter parameters is required and cannot be null."));
} else {
parameters.validate();
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.update(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), ifMatch, ifNoneMatch, parameters, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* The operation to update a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> updateWithResponseAsync(String resourceGroupName, String vmName,
VirtualMachineUpdateInner parameters, String ifMatch, String ifNoneMatch, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (parameters == null) {
return Mono.error(new IllegalArgumentException("Parameter parameters is required and cannot be null."));
} else {
parameters.validate();
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.update(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), ifMatch, ifNoneMatch, parameters, accept, context);
}
/**
* The operation to update a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, VirtualMachineInner> beginUpdateAsync(String resourceGroupName,
String vmName, VirtualMachineUpdateInner parameters, String ifMatch, String ifNoneMatch) {
Mono>> mono
= updateWithResponseAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch);
return this.client.getLroResult(mono, this.client.getHttpPipeline(),
VirtualMachineInner.class, VirtualMachineInner.class, this.client.getContext());
}
/**
* The operation to update a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, VirtualMachineInner> beginUpdateAsync(String resourceGroupName,
String vmName, VirtualMachineUpdateInner parameters) {
final String ifMatch = null;
final String ifNoneMatch = null;
Mono>> mono
= updateWithResponseAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch);
return this.client.getLroResult(mono, this.client.getHttpPipeline(),
VirtualMachineInner.class, VirtualMachineInner.class, this.client.getContext());
}
/**
* The operation to update a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, VirtualMachineInner> beginUpdateAsync(String resourceGroupName,
String vmName, VirtualMachineUpdateInner parameters, String ifMatch, String ifNoneMatch, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= updateWithResponseAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(),
VirtualMachineInner.class, VirtualMachineInner.class, context);
}
/**
* The operation to update a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, VirtualMachineInner> beginUpdate(String resourceGroupName,
String vmName, VirtualMachineUpdateInner parameters) {
final String ifMatch = null;
final String ifNoneMatch = null;
return this.beginUpdateAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch).getSyncPoller();
}
/**
* The operation to update a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, VirtualMachineInner> beginUpdate(String resourceGroupName,
String vmName, VirtualMachineUpdateInner parameters, String ifMatch, String ifNoneMatch, Context context) {
return this.beginUpdateAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch, context)
.getSyncPoller();
}
/**
* The operation to update a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono updateAsync(String resourceGroupName, String vmName,
VirtualMachineUpdateInner parameters, String ifMatch, String ifNoneMatch) {
return beginUpdateAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to update a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono updateAsync(String resourceGroupName, String vmName,
VirtualMachineUpdateInner parameters) {
final String ifMatch = null;
final String ifNoneMatch = null;
return beginUpdateAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to update a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono updateAsync(String resourceGroupName, String vmName,
VirtualMachineUpdateInner parameters, String ifMatch, String ifNoneMatch, Context context) {
return beginUpdateAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to update a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VirtualMachineInner update(String resourceGroupName, String vmName, VirtualMachineUpdateInner parameters) {
final String ifMatch = null;
final String ifNoneMatch = null;
return updateAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch).block();
}
/**
* The operation to update a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Update Virtual Machine operation.
* @param ifMatch The ETag of the transformation. Omit this value to always overwrite the current resource. Specify
* the last-seen ETag value to prevent accidentally overwriting concurrent changes.
* @param ifNoneMatch Set to '*' to allow a new record set to be created, but to prevent updating an existing record
* set. Other values will result in error from server as they are not supported.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VirtualMachineInner update(String resourceGroupName, String vmName, VirtualMachineUpdateInner parameters,
String ifMatch, String ifNoneMatch, Context context) {
return updateAsync(resourceGroupName, vmName, parameters, ifMatch, ifNoneMatch, context).block();
}
/**
* The operation to delete a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param forceDeletion Optional parameter to force delete virtual machines.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> deleteWithResponseAsync(String resourceGroupName, String vmName,
Boolean forceDeletion) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.delete(this.client.getEndpoint(), resourceGroupName, vmName, forceDeletion,
apiVersion, this.client.getSubscriptionId(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* The operation to delete a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param forceDeletion Optional parameter to force delete virtual machines.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> deleteWithResponseAsync(String resourceGroupName, String vmName,
Boolean forceDeletion, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.delete(this.client.getEndpoint(), resourceGroupName, vmName, forceDeletion, apiVersion,
this.client.getSubscriptionId(), accept, context);
}
/**
* The operation to delete a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param forceDeletion Optional parameter to force delete virtual machines.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginDeleteAsync(String resourceGroupName, String vmName,
Boolean forceDeletion) {
Mono>> mono = deleteWithResponseAsync(resourceGroupName, vmName, forceDeletion);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* The operation to delete a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginDeleteAsync(String resourceGroupName, String vmName) {
final Boolean forceDeletion = null;
Mono>> mono = deleteWithResponseAsync(resourceGroupName, vmName, forceDeletion);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* The operation to delete a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param forceDeletion Optional parameter to force delete virtual machines.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeleteAsync(String resourceGroupName, String vmName,
Boolean forceDeletion, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= deleteWithResponseAsync(resourceGroupName, vmName, forceDeletion, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* The operation to delete a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDelete(String resourceGroupName, String vmName) {
final Boolean forceDeletion = null;
return this.beginDeleteAsync(resourceGroupName, vmName, forceDeletion).getSyncPoller();
}
/**
* The operation to delete a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param forceDeletion Optional parameter to force delete virtual machines.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDelete(String resourceGroupName, String vmName,
Boolean forceDeletion, Context context) {
return this.beginDeleteAsync(resourceGroupName, vmName, forceDeletion, context).getSyncPoller();
}
/**
* The operation to delete a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param forceDeletion Optional parameter to force delete virtual machines.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteAsync(String resourceGroupName, String vmName, Boolean forceDeletion) {
return beginDeleteAsync(resourceGroupName, vmName, forceDeletion).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to delete a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteAsync(String resourceGroupName, String vmName) {
final Boolean forceDeletion = null;
return beginDeleteAsync(resourceGroupName, vmName, forceDeletion).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to delete a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param forceDeletion Optional parameter to force delete virtual machines.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deleteAsync(String resourceGroupName, String vmName, Boolean forceDeletion, Context context) {
return beginDeleteAsync(resourceGroupName, vmName, forceDeletion, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to delete a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void delete(String resourceGroupName, String vmName) {
final Boolean forceDeletion = null;
deleteAsync(resourceGroupName, vmName, forceDeletion).block();
}
/**
* The operation to delete a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param forceDeletion Optional parameter to force delete virtual machines.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void delete(String resourceGroupName, String vmName, Boolean forceDeletion, Context context) {
deleteAsync(resourceGroupName, vmName, forceDeletion, context).block();
}
/**
* Retrieves information about the model view or the instance view of a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param expand The expand expression to apply on the operation. 'InstanceView' retrieves a snapshot of the runtime
* properties of the virtual machine that is managed by the platform and can change outside of control plane
* operations. 'UserData' retrieves the UserData property as part of the VM model view that was provided by the user
* during the VM Create/Update operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getByResourceGroupWithResponseAsync(String resourceGroupName,
String vmName, InstanceViewTypes expand) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getByResourceGroup(this.client.getEndpoint(), resourceGroupName, vmName,
expand, apiVersion, this.client.getSubscriptionId(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Retrieves information about the model view or the instance view of a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param expand The expand expression to apply on the operation. 'InstanceView' retrieves a snapshot of the runtime
* properties of the virtual machine that is managed by the platform and can change outside of control plane
* operations. 'UserData' retrieves the UserData property as part of the VM model view that was provided by the user
* during the VM Create/Update operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getByResourceGroupWithResponseAsync(String resourceGroupName,
String vmName, InstanceViewTypes expand, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getByResourceGroup(this.client.getEndpoint(), resourceGroupName, vmName, expand, apiVersion,
this.client.getSubscriptionId(), accept, context);
}
/**
* Retrieves information about the model view or the instance view of a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getByResourceGroupAsync(String resourceGroupName, String vmName) {
final InstanceViewTypes expand = null;
return getByResourceGroupWithResponseAsync(resourceGroupName, vmName, expand)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Retrieves information about the model view or the instance view of a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param expand The expand expression to apply on the operation. 'InstanceView' retrieves a snapshot of the runtime
* properties of the virtual machine that is managed by the platform and can change outside of control plane
* operations. 'UserData' retrieves the UserData property as part of the VM model view that was provided by the user
* during the VM Create/Update operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getByResourceGroupWithResponse(String resourceGroupName, String vmName,
InstanceViewTypes expand, Context context) {
return getByResourceGroupWithResponseAsync(resourceGroupName, vmName, expand, context).block();
}
/**
* Retrieves information about the model view or the instance view of a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return describes a Virtual Machine.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VirtualMachineInner getByResourceGroup(String resourceGroupName, String vmName) {
final InstanceViewTypes expand = null;
return getByResourceGroupWithResponse(resourceGroupName, vmName, expand, Context.NONE).getValue();
}
/**
* Retrieves information about the run-time state of a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the instance view of a virtual machine along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> instanceViewWithResponseAsync(String resourceGroupName,
String vmName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.instanceView(this.client.getEndpoint(), resourceGroupName, vmName,
apiVersion, this.client.getSubscriptionId(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Retrieves information about the run-time state of a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the instance view of a virtual machine along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> instanceViewWithResponseAsync(String resourceGroupName,
String vmName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.instanceView(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), accept, context);
}
/**
* Retrieves information about the run-time state of a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the instance view of a virtual machine on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono instanceViewAsync(String resourceGroupName, String vmName) {
return instanceViewWithResponseAsync(resourceGroupName, vmName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Retrieves information about the run-time state of a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the instance view of a virtual machine along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response instanceViewWithResponse(String resourceGroupName, String vmName,
Context context) {
return instanceViewWithResponseAsync(resourceGroupName, vmName, context).block();
}
/**
* Retrieves information about the run-time state of a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the instance view of a virtual machine.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public VirtualMachineInstanceViewInner instanceView(String resourceGroupName, String vmName) {
return instanceViewWithResponse(resourceGroupName, vmName, Context.NONE).getValue();
}
/**
* Converts virtual machine disks from blob-based to managed disks. Virtual machine must be stop-deallocated before
* invoking this operation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> convertToManagedDisksWithResponseAsync(String resourceGroupName,
String vmName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.convertToManagedDisks(this.client.getEndpoint(), resourceGroupName, vmName,
apiVersion, this.client.getSubscriptionId(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Converts virtual machine disks from blob-based to managed disks. Virtual machine must be stop-deallocated before
* invoking this operation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> convertToManagedDisksWithResponseAsync(String resourceGroupName,
String vmName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.convertToManagedDisks(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), accept, context);
}
/**
* Converts virtual machine disks from blob-based to managed disks. Virtual machine must be stop-deallocated before
* invoking this operation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginConvertToManagedDisksAsync(String resourceGroupName, String vmName) {
Mono>> mono = convertToManagedDisksWithResponseAsync(resourceGroupName, vmName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Converts virtual machine disks from blob-based to managed disks. Virtual machine must be stop-deallocated before
* invoking this operation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginConvertToManagedDisksAsync(String resourceGroupName, String vmName,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= convertToManagedDisksWithResponseAsync(resourceGroupName, vmName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Converts virtual machine disks from blob-based to managed disks. Virtual machine must be stop-deallocated before
* invoking this operation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginConvertToManagedDisks(String resourceGroupName, String vmName) {
return this.beginConvertToManagedDisksAsync(resourceGroupName, vmName).getSyncPoller();
}
/**
* Converts virtual machine disks from blob-based to managed disks. Virtual machine must be stop-deallocated before
* invoking this operation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginConvertToManagedDisks(String resourceGroupName, String vmName,
Context context) {
return this.beginConvertToManagedDisksAsync(resourceGroupName, vmName, context).getSyncPoller();
}
/**
* Converts virtual machine disks from blob-based to managed disks. Virtual machine must be stop-deallocated before
* invoking this operation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono convertToManagedDisksAsync(String resourceGroupName, String vmName) {
return beginConvertToManagedDisksAsync(resourceGroupName, vmName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Converts virtual machine disks from blob-based to managed disks. Virtual machine must be stop-deallocated before
* invoking this operation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono convertToManagedDisksAsync(String resourceGroupName, String vmName, Context context) {
return beginConvertToManagedDisksAsync(resourceGroupName, vmName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Converts virtual machine disks from blob-based to managed disks. Virtual machine must be stop-deallocated before
* invoking this operation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void convertToManagedDisks(String resourceGroupName, String vmName) {
convertToManagedDisksAsync(resourceGroupName, vmName).block();
}
/**
* Converts virtual machine disks from blob-based to managed disks. Virtual machine must be stop-deallocated before
* invoking this operation.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void convertToManagedDisks(String resourceGroupName, String vmName, Context context) {
convertToManagedDisksAsync(resourceGroupName, vmName, context).block();
}
/**
* Shuts down the virtual machine and releases the compute resources. You are not billed for the compute resources
* that this virtual machine uses.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param hibernate Optional parameter to hibernate a virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> deallocateWithResponseAsync(String resourceGroupName, String vmName,
Boolean hibernate) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.deallocate(this.client.getEndpoint(), resourceGroupName, vmName, hibernate,
apiVersion, this.client.getSubscriptionId(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Shuts down the virtual machine and releases the compute resources. You are not billed for the compute resources
* that this virtual machine uses.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param hibernate Optional parameter to hibernate a virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> deallocateWithResponseAsync(String resourceGroupName, String vmName,
Boolean hibernate, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.deallocate(this.client.getEndpoint(), resourceGroupName, vmName, hibernate, apiVersion,
this.client.getSubscriptionId(), accept, context);
}
/**
* Shuts down the virtual machine and releases the compute resources. You are not billed for the compute resources
* that this virtual machine uses.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param hibernate Optional parameter to hibernate a virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginDeallocateAsync(String resourceGroupName, String vmName,
Boolean hibernate) {
Mono>> mono = deallocateWithResponseAsync(resourceGroupName, vmName, hibernate);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Shuts down the virtual machine and releases the compute resources. You are not billed for the compute resources
* that this virtual machine uses.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginDeallocateAsync(String resourceGroupName, String vmName) {
final Boolean hibernate = null;
Mono>> mono = deallocateWithResponseAsync(resourceGroupName, vmName, hibernate);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Shuts down the virtual machine and releases the compute resources. You are not billed for the compute resources
* that this virtual machine uses.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param hibernate Optional parameter to hibernate a virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeallocateAsync(String resourceGroupName, String vmName,
Boolean hibernate, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= deallocateWithResponseAsync(resourceGroupName, vmName, hibernate, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Shuts down the virtual machine and releases the compute resources. You are not billed for the compute resources
* that this virtual machine uses.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDeallocate(String resourceGroupName, String vmName) {
final Boolean hibernate = null;
return this.beginDeallocateAsync(resourceGroupName, vmName, hibernate).getSyncPoller();
}
/**
* Shuts down the virtual machine and releases the compute resources. You are not billed for the compute resources
* that this virtual machine uses.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param hibernate Optional parameter to hibernate a virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDeallocate(String resourceGroupName, String vmName,
Boolean hibernate, Context context) {
return this.beginDeallocateAsync(resourceGroupName, vmName, hibernate, context).getSyncPoller();
}
/**
* Shuts down the virtual machine and releases the compute resources. You are not billed for the compute resources
* that this virtual machine uses.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param hibernate Optional parameter to hibernate a virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deallocateAsync(String resourceGroupName, String vmName, Boolean hibernate) {
return beginDeallocateAsync(resourceGroupName, vmName, hibernate).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Shuts down the virtual machine and releases the compute resources. You are not billed for the compute resources
* that this virtual machine uses.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deallocateAsync(String resourceGroupName, String vmName) {
final Boolean hibernate = null;
return beginDeallocateAsync(resourceGroupName, vmName, hibernate).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Shuts down the virtual machine and releases the compute resources. You are not billed for the compute resources
* that this virtual machine uses.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param hibernate Optional parameter to hibernate a virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deallocateAsync(String resourceGroupName, String vmName, Boolean hibernate, Context context) {
return beginDeallocateAsync(resourceGroupName, vmName, hibernate, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Shuts down the virtual machine and releases the compute resources. You are not billed for the compute resources
* that this virtual machine uses.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deallocate(String resourceGroupName, String vmName) {
final Boolean hibernate = null;
deallocateAsync(resourceGroupName, vmName, hibernate).block();
}
/**
* Shuts down the virtual machine and releases the compute resources. You are not billed for the compute resources
* that this virtual machine uses.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param hibernate Optional parameter to hibernate a virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deallocate(String resourceGroupName, String vmName, Boolean hibernate, Context context) {
deallocateAsync(resourceGroupName, vmName, hibernate, context).block();
}
/**
* Sets the OS state of the virtual machine to generalized. It is recommended to sysprep the virtual machine before
* performing this operation. For Windows, please refer to [Create a managed image of a generalized VM in
* Azure](https://docs.microsoft.com/azure/virtual-machines/windows/capture-image-resource). For Linux, please refer
* to [How to create an image of a virtual machine or
* VHD](https://docs.microsoft.com/azure/virtual-machines/linux/capture-image).
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> generalizeWithResponseAsync(String resourceGroupName, String vmName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.generalize(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Sets the OS state of the virtual machine to generalized. It is recommended to sysprep the virtual machine before
* performing this operation. For Windows, please refer to [Create a managed image of a generalized VM in
* Azure](https://docs.microsoft.com/azure/virtual-machines/windows/capture-image-resource). For Linux, please refer
* to [How to create an image of a virtual machine or
* VHD](https://docs.microsoft.com/azure/virtual-machines/linux/capture-image).
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> generalizeWithResponseAsync(String resourceGroupName, String vmName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.generalize(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), accept, context);
}
/**
* Sets the OS state of the virtual machine to generalized. It is recommended to sysprep the virtual machine before
* performing this operation. For Windows, please refer to [Create a managed image of a generalized VM in
* Azure](https://docs.microsoft.com/azure/virtual-machines/windows/capture-image-resource). For Linux, please refer
* to [How to create an image of a virtual machine or
* VHD](https://docs.microsoft.com/azure/virtual-machines/linux/capture-image).
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono generalizeAsync(String resourceGroupName, String vmName) {
return generalizeWithResponseAsync(resourceGroupName, vmName).flatMap(ignored -> Mono.empty());
}
/**
* Sets the OS state of the virtual machine to generalized. It is recommended to sysprep the virtual machine before
* performing this operation. For Windows, please refer to [Create a managed image of a generalized VM in
* Azure](https://docs.microsoft.com/azure/virtual-machines/windows/capture-image-resource). For Linux, please refer
* to [How to create an image of a virtual machine or
* VHD](https://docs.microsoft.com/azure/virtual-machines/linux/capture-image).
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response generalizeWithResponse(String resourceGroupName, String vmName, Context context) {
return generalizeWithResponseAsync(resourceGroupName, vmName, context).block();
}
/**
* Sets the OS state of the virtual machine to generalized. It is recommended to sysprep the virtual machine before
* performing this operation. For Windows, please refer to [Create a managed image of a generalized VM in
* Azure](https://docs.microsoft.com/azure/virtual-machines/windows/capture-image-resource). For Linux, please refer
* to [How to create an image of a virtual machine or
* VHD](https://docs.microsoft.com/azure/virtual-machines/linux/capture-image).
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void generalize(String resourceGroupName, String vmName) {
generalizeWithResponse(resourceGroupName, vmName, Context.NONE);
}
/**
* Lists all of the virtual machines in the specified resource group. Use the nextLink property in the response to
* get the next page of virtual machines.
*
* @param resourceGroupName The name of the resource group.
* @param filter The system query option to filter VMs returned in the response. Allowed value is
* 'virtualMachineScaleSet/id' eq
* /subscriptions/{subId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachineScaleSets/{vmssName}'.
* @param expand The expand expression to apply on operation. 'instanceView' enables fetching run time status of all
* Virtual Machines, this can only be specified if a valid $filter option is specified.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByResourceGroupSinglePageAsync(String resourceGroupName,
String filter, ExpandTypeForListVMs expand) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listByResourceGroup(this.client.getEndpoint(), resourceGroupName, filter,
expand, apiVersion, this.client.getSubscriptionId(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Lists all of the virtual machines in the specified resource group. Use the nextLink property in the response to
* get the next page of virtual machines.
*
* @param resourceGroupName The name of the resource group.
* @param filter The system query option to filter VMs returned in the response. Allowed value is
* 'virtualMachineScaleSet/id' eq
* /subscriptions/{subId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachineScaleSets/{vmssName}'.
* @param expand The expand expression to apply on operation. 'instanceView' enables fetching run time status of all
* Virtual Machines, this can only be specified if a valid $filter option is specified.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByResourceGroupSinglePageAsync(String resourceGroupName,
String filter, ExpandTypeForListVMs expand, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listByResourceGroup(this.client.getEndpoint(), resourceGroupName, filter, expand, apiVersion,
this.client.getSubscriptionId(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Lists all of the virtual machines in the specified resource group. Use the nextLink property in the response to
* get the next page of virtual machines.
*
* @param resourceGroupName The name of the resource group.
* @param filter The system query option to filter VMs returned in the response. Allowed value is
* 'virtualMachineScaleSet/id' eq
* /subscriptions/{subId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachineScaleSets/{vmssName}'.
* @param expand The expand expression to apply on operation. 'instanceView' enables fetching run time status of all
* Virtual Machines, this can only be specified if a valid $filter option is specified.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listByResourceGroupAsync(String resourceGroupName, String filter,
ExpandTypeForListVMs expand) {
return new PagedFlux<>(() -> listByResourceGroupSinglePageAsync(resourceGroupName, filter, expand),
nextLink -> listNextSinglePageAsync(nextLink));
}
/**
* Lists all of the virtual machines in the specified resource group. Use the nextLink property in the response to
* get the next page of virtual machines.
*
* @param resourceGroupName The name of the resource group.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listByResourceGroupAsync(String resourceGroupName) {
final String filter = null;
final ExpandTypeForListVMs expand = null;
return new PagedFlux<>(() -> listByResourceGroupSinglePageAsync(resourceGroupName, filter, expand),
nextLink -> listNextSinglePageAsync(nextLink));
}
/**
* Lists all of the virtual machines in the specified resource group. Use the nextLink property in the response to
* get the next page of virtual machines.
*
* @param resourceGroupName The name of the resource group.
* @param filter The system query option to filter VMs returned in the response. Allowed value is
* 'virtualMachineScaleSet/id' eq
* /subscriptions/{subId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachineScaleSets/{vmssName}'.
* @param expand The expand expression to apply on operation. 'instanceView' enables fetching run time status of all
* Virtual Machines, this can only be specified if a valid $filter option is specified.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listByResourceGroupAsync(String resourceGroupName, String filter,
ExpandTypeForListVMs expand, Context context) {
return new PagedFlux<>(() -> listByResourceGroupSinglePageAsync(resourceGroupName, filter, expand, context),
nextLink -> listNextSinglePageAsync(nextLink, context));
}
/**
* Lists all of the virtual machines in the specified resource group. Use the nextLink property in the response to
* get the next page of virtual machines.
*
* @param resourceGroupName The name of the resource group.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listByResourceGroup(String resourceGroupName) {
final String filter = null;
final ExpandTypeForListVMs expand = null;
return new PagedIterable<>(listByResourceGroupAsync(resourceGroupName, filter, expand));
}
/**
* Lists all of the virtual machines in the specified resource group. Use the nextLink property in the response to
* get the next page of virtual machines.
*
* @param resourceGroupName The name of the resource group.
* @param filter The system query option to filter VMs returned in the response. Allowed value is
* 'virtualMachineScaleSet/id' eq
* /subscriptions/{subId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachineScaleSets/{vmssName}'.
* @param expand The expand expression to apply on operation. 'instanceView' enables fetching run time status of all
* Virtual Machines, this can only be specified if a valid $filter option is specified.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listByResourceGroup(String resourceGroupName, String filter,
ExpandTypeForListVMs expand, Context context) {
return new PagedIterable<>(listByResourceGroupAsync(resourceGroupName, filter, expand, context));
}
/**
* Lists all of the virtual machines in the specified subscription. Use the nextLink property in the response to get
* the next page of virtual machines.
*
* @param statusOnly statusOnly=true enables fetching run time status of all Virtual Machines in the subscription.
* @param filter The system query option to filter VMs returned in the response. Allowed value is
* 'virtualMachineScaleSet/id' eq
* /subscriptions/{subId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachineScaleSets/{vmssName}'.
* @param expand The expand expression to apply on operation. 'instanceView' enables fetching run time status of all
* Virtual Machines, this can only be specified if a valid $filter option is specified.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listSinglePageAsync(String statusOnly, String filter,
ExpandTypesForListVMs expand) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.list(this.client.getEndpoint(), apiVersion, this.client.getSubscriptionId(),
statusOnly, filter, expand, accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Lists all of the virtual machines in the specified subscription. Use the nextLink property in the response to get
* the next page of virtual machines.
*
* @param statusOnly statusOnly=true enables fetching run time status of all Virtual Machines in the subscription.
* @param filter The system query option to filter VMs returned in the response. Allowed value is
* 'virtualMachineScaleSet/id' eq
* /subscriptions/{subId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachineScaleSets/{vmssName}'.
* @param expand The expand expression to apply on operation. 'instanceView' enables fetching run time status of all
* Virtual Machines, this can only be specified if a valid $filter option is specified.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listSinglePageAsync(String statusOnly, String filter,
ExpandTypesForListVMs expand, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.list(this.client.getEndpoint(), apiVersion, this.client.getSubscriptionId(), statusOnly, filter, expand,
accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Lists all of the virtual machines in the specified subscription. Use the nextLink property in the response to get
* the next page of virtual machines.
*
* @param statusOnly statusOnly=true enables fetching run time status of all Virtual Machines in the subscription.
* @param filter The system query option to filter VMs returned in the response. Allowed value is
* 'virtualMachineScaleSet/id' eq
* /subscriptions/{subId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachineScaleSets/{vmssName}'.
* @param expand The expand expression to apply on operation. 'instanceView' enables fetching run time status of all
* Virtual Machines, this can only be specified if a valid $filter option is specified.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listAsync(String statusOnly, String filter, ExpandTypesForListVMs expand) {
return new PagedFlux<>(() -> listSinglePageAsync(statusOnly, filter, expand),
nextLink -> listAllNextSinglePageAsync(nextLink));
}
/**
* Lists all of the virtual machines in the specified subscription. Use the nextLink property in the response to get
* the next page of virtual machines.
*
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listAsync() {
final String statusOnly = null;
final String filter = null;
final ExpandTypesForListVMs expand = null;
return new PagedFlux<>(() -> listSinglePageAsync(statusOnly, filter, expand),
nextLink -> listAllNextSinglePageAsync(nextLink));
}
/**
* Lists all of the virtual machines in the specified subscription. Use the nextLink property in the response to get
* the next page of virtual machines.
*
* @param statusOnly statusOnly=true enables fetching run time status of all Virtual Machines in the subscription.
* @param filter The system query option to filter VMs returned in the response. Allowed value is
* 'virtualMachineScaleSet/id' eq
* /subscriptions/{subId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachineScaleSets/{vmssName}'.
* @param expand The expand expression to apply on operation. 'instanceView' enables fetching run time status of all
* Virtual Machines, this can only be specified if a valid $filter option is specified.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listAsync(String statusOnly, String filter, ExpandTypesForListVMs expand,
Context context) {
return new PagedFlux<>(() -> listSinglePageAsync(statusOnly, filter, expand, context),
nextLink -> listAllNextSinglePageAsync(nextLink, context));
}
/**
* Lists all of the virtual machines in the specified subscription. Use the nextLink property in the response to get
* the next page of virtual machines.
*
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable list() {
final String statusOnly = null;
final String filter = null;
final ExpandTypesForListVMs expand = null;
return new PagedIterable<>(listAsync(statusOnly, filter, expand));
}
/**
* Lists all of the virtual machines in the specified subscription. Use the nextLink property in the response to get
* the next page of virtual machines.
*
* @param statusOnly statusOnly=true enables fetching run time status of all Virtual Machines in the subscription.
* @param filter The system query option to filter VMs returned in the response. Allowed value is
* 'virtualMachineScaleSet/id' eq
* /subscriptions/{subId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/virtualMachineScaleSets/{vmssName}'.
* @param expand The expand expression to apply on operation. 'instanceView' enables fetching run time status of all
* Virtual Machines, this can only be specified if a valid $filter option is specified.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable list(String statusOnly, String filter, ExpandTypesForListVMs expand,
Context context) {
return new PagedIterable<>(listAsync(statusOnly, filter, expand, context));
}
/**
* Lists all available virtual machine sizes to which the specified virtual machine can be resized.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listAvailableSizesSinglePageAsync(String resourceGroupName,
String vmName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listAvailableSizes(this.client.getEndpoint(), resourceGroupName, vmName,
apiVersion, this.client.getSubscriptionId(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), null, null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Lists all available virtual machine sizes to which the specified virtual machine can be resized.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listAvailableSizesSinglePageAsync(String resourceGroupName,
String vmName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listAvailableSizes(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), null, null));
}
/**
* Lists all available virtual machine sizes to which the specified virtual machine can be resized.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listAvailableSizesAsync(String resourceGroupName, String vmName) {
return new PagedFlux<>(() -> listAvailableSizesSinglePageAsync(resourceGroupName, vmName));
}
/**
* Lists all available virtual machine sizes to which the specified virtual machine can be resized.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listAvailableSizesAsync(String resourceGroupName, String vmName,
Context context) {
return new PagedFlux<>(() -> listAvailableSizesSinglePageAsync(resourceGroupName, vmName, context));
}
/**
* Lists all available virtual machine sizes to which the specified virtual machine can be resized.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listAvailableSizes(String resourceGroupName, String vmName) {
return new PagedIterable<>(listAvailableSizesAsync(resourceGroupName, vmName));
}
/**
* Lists all available virtual machine sizes to which the specified virtual machine can be resized.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List Virtual Machine operation response as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listAvailableSizes(String resourceGroupName, String vmName,
Context context) {
return new PagedIterable<>(listAvailableSizesAsync(resourceGroupName, vmName, context));
}
/**
* The operation to power off (stop) a virtual machine. The virtual machine can be restarted with the same
* provisioned resources. You are still charged for this virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param skipShutdown The parameter to request non-graceful VM shutdown. True value for this flag indicates
* non-graceful shutdown whereas false indicates otherwise. Default value for this flag is false if not specified.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> powerOffWithResponseAsync(String resourceGroupName, String vmName,
Boolean skipShutdown) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.powerOff(this.client.getEndpoint(), resourceGroupName, vmName, skipShutdown,
apiVersion, this.client.getSubscriptionId(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* The operation to power off (stop) a virtual machine. The virtual machine can be restarted with the same
* provisioned resources. You are still charged for this virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param skipShutdown The parameter to request non-graceful VM shutdown. True value for this flag indicates
* non-graceful shutdown whereas false indicates otherwise. Default value for this flag is false if not specified.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> powerOffWithResponseAsync(String resourceGroupName, String vmName,
Boolean skipShutdown, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.powerOff(this.client.getEndpoint(), resourceGroupName, vmName, skipShutdown, apiVersion,
this.client.getSubscriptionId(), accept, context);
}
/**
* The operation to power off (stop) a virtual machine. The virtual machine can be restarted with the same
* provisioned resources. You are still charged for this virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param skipShutdown The parameter to request non-graceful VM shutdown. True value for this flag indicates
* non-graceful shutdown whereas false indicates otherwise. Default value for this flag is false if not specified.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginPowerOffAsync(String resourceGroupName, String vmName,
Boolean skipShutdown) {
Mono>> mono = powerOffWithResponseAsync(resourceGroupName, vmName, skipShutdown);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* The operation to power off (stop) a virtual machine. The virtual machine can be restarted with the same
* provisioned resources. You are still charged for this virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginPowerOffAsync(String resourceGroupName, String vmName) {
final Boolean skipShutdown = null;
Mono>> mono = powerOffWithResponseAsync(resourceGroupName, vmName, skipShutdown);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* The operation to power off (stop) a virtual machine. The virtual machine can be restarted with the same
* provisioned resources. You are still charged for this virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param skipShutdown The parameter to request non-graceful VM shutdown. True value for this flag indicates
* non-graceful shutdown whereas false indicates otherwise. Default value for this flag is false if not specified.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginPowerOffAsync(String resourceGroupName, String vmName,
Boolean skipShutdown, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= powerOffWithResponseAsync(resourceGroupName, vmName, skipShutdown, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* The operation to power off (stop) a virtual machine. The virtual machine can be restarted with the same
* provisioned resources. You are still charged for this virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginPowerOff(String resourceGroupName, String vmName) {
final Boolean skipShutdown = null;
return this.beginPowerOffAsync(resourceGroupName, vmName, skipShutdown).getSyncPoller();
}
/**
* The operation to power off (stop) a virtual machine. The virtual machine can be restarted with the same
* provisioned resources. You are still charged for this virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param skipShutdown The parameter to request non-graceful VM shutdown. True value for this flag indicates
* non-graceful shutdown whereas false indicates otherwise. Default value for this flag is false if not specified.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginPowerOff(String resourceGroupName, String vmName,
Boolean skipShutdown, Context context) {
return this.beginPowerOffAsync(resourceGroupName, vmName, skipShutdown, context).getSyncPoller();
}
/**
* The operation to power off (stop) a virtual machine. The virtual machine can be restarted with the same
* provisioned resources. You are still charged for this virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param skipShutdown The parameter to request non-graceful VM shutdown. True value for this flag indicates
* non-graceful shutdown whereas false indicates otherwise. Default value for this flag is false if not specified.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono powerOffAsync(String resourceGroupName, String vmName, Boolean skipShutdown) {
return beginPowerOffAsync(resourceGroupName, vmName, skipShutdown).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to power off (stop) a virtual machine. The virtual machine can be restarted with the same
* provisioned resources. You are still charged for this virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono powerOffAsync(String resourceGroupName, String vmName) {
final Boolean skipShutdown = null;
return beginPowerOffAsync(resourceGroupName, vmName, skipShutdown).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to power off (stop) a virtual machine. The virtual machine can be restarted with the same
* provisioned resources. You are still charged for this virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param skipShutdown The parameter to request non-graceful VM shutdown. True value for this flag indicates
* non-graceful shutdown whereas false indicates otherwise. Default value for this flag is false if not specified.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono powerOffAsync(String resourceGroupName, String vmName, Boolean skipShutdown, Context context) {
return beginPowerOffAsync(resourceGroupName, vmName, skipShutdown, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to power off (stop) a virtual machine. The virtual machine can be restarted with the same
* provisioned resources. You are still charged for this virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void powerOff(String resourceGroupName, String vmName) {
final Boolean skipShutdown = null;
powerOffAsync(resourceGroupName, vmName, skipShutdown).block();
}
/**
* The operation to power off (stop) a virtual machine. The virtual machine can be restarted with the same
* provisioned resources. You are still charged for this virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param skipShutdown The parameter to request non-graceful VM shutdown. True value for this flag indicates
* non-graceful shutdown whereas false indicates otherwise. Default value for this flag is false if not specified.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void powerOff(String resourceGroupName, String vmName, Boolean skipShutdown, Context context) {
powerOffAsync(resourceGroupName, vmName, skipShutdown, context).block();
}
/**
* The operation to reapply a virtual machine's state.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> reapplyWithResponseAsync(String resourceGroupName, String vmName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.reapply(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* The operation to reapply a virtual machine's state.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> reapplyWithResponseAsync(String resourceGroupName, String vmName,
Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.reapply(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), accept, context);
}
/**
* The operation to reapply a virtual machine's state.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginReapplyAsync(String resourceGroupName, String vmName) {
Mono>> mono = reapplyWithResponseAsync(resourceGroupName, vmName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* The operation to reapply a virtual machine's state.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginReapplyAsync(String resourceGroupName, String vmName,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono = reapplyWithResponseAsync(resourceGroupName, vmName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* The operation to reapply a virtual machine's state.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginReapply(String resourceGroupName, String vmName) {
return this.beginReapplyAsync(resourceGroupName, vmName).getSyncPoller();
}
/**
* The operation to reapply a virtual machine's state.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginReapply(String resourceGroupName, String vmName, Context context) {
return this.beginReapplyAsync(resourceGroupName, vmName, context).getSyncPoller();
}
/**
* The operation to reapply a virtual machine's state.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono reapplyAsync(String resourceGroupName, String vmName) {
return beginReapplyAsync(resourceGroupName, vmName).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to reapply a virtual machine's state.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono reapplyAsync(String resourceGroupName, String vmName, Context context) {
return beginReapplyAsync(resourceGroupName, vmName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to reapply a virtual machine's state.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void reapply(String resourceGroupName, String vmName) {
reapplyAsync(resourceGroupName, vmName).block();
}
/**
* The operation to reapply a virtual machine's state.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void reapply(String resourceGroupName, String vmName, Context context) {
reapplyAsync(resourceGroupName, vmName, context).block();
}
/**
* The operation to restart a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> restartWithResponseAsync(String resourceGroupName, String vmName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.restart(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* The operation to restart a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> restartWithResponseAsync(String resourceGroupName, String vmName,
Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.restart(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), accept, context);
}
/**
* The operation to restart a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginRestartAsync(String resourceGroupName, String vmName) {
Mono>> mono = restartWithResponseAsync(resourceGroupName, vmName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* The operation to restart a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginRestartAsync(String resourceGroupName, String vmName,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono = restartWithResponseAsync(resourceGroupName, vmName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* The operation to restart a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginRestart(String resourceGroupName, String vmName) {
return this.beginRestartAsync(resourceGroupName, vmName).getSyncPoller();
}
/**
* The operation to restart a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginRestart(String resourceGroupName, String vmName, Context context) {
return this.beginRestartAsync(resourceGroupName, vmName, context).getSyncPoller();
}
/**
* The operation to restart a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono restartAsync(String resourceGroupName, String vmName) {
return beginRestartAsync(resourceGroupName, vmName).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to restart a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono restartAsync(String resourceGroupName, String vmName, Context context) {
return beginRestartAsync(resourceGroupName, vmName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to restart a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void restart(String resourceGroupName, String vmName) {
restartAsync(resourceGroupName, vmName).block();
}
/**
* The operation to restart a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void restart(String resourceGroupName, String vmName, Context context) {
restartAsync(resourceGroupName, vmName, context).block();
}
/**
* The operation to start a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> startWithResponseAsync(String resourceGroupName, String vmName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.start(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* The operation to start a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> startWithResponseAsync(String resourceGroupName, String vmName,
Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.start(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), accept, context);
}
/**
* The operation to start a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginStartAsync(String resourceGroupName, String vmName) {
Mono>> mono = startWithResponseAsync(resourceGroupName, vmName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* The operation to start a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginStartAsync(String resourceGroupName, String vmName,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono = startWithResponseAsync(resourceGroupName, vmName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* The operation to start a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginStart(String resourceGroupName, String vmName) {
return this.beginStartAsync(resourceGroupName, vmName).getSyncPoller();
}
/**
* The operation to start a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginStart(String resourceGroupName, String vmName, Context context) {
return this.beginStartAsync(resourceGroupName, vmName, context).getSyncPoller();
}
/**
* The operation to start a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono startAsync(String resourceGroupName, String vmName) {
return beginStartAsync(resourceGroupName, vmName).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to start a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono startAsync(String resourceGroupName, String vmName, Context context) {
return beginStartAsync(resourceGroupName, vmName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* The operation to start a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void start(String resourceGroupName, String vmName) {
startAsync(resourceGroupName, vmName).block();
}
/**
* The operation to start a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void start(String resourceGroupName, String vmName, Context context) {
startAsync(resourceGroupName, vmName, context).block();
}
/**
* Shuts down the virtual machine, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> redeployWithResponseAsync(String resourceGroupName, String vmName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.redeploy(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Shuts down the virtual machine, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> redeployWithResponseAsync(String resourceGroupName, String vmName,
Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.redeploy(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), accept, context);
}
/**
* Shuts down the virtual machine, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginRedeployAsync(String resourceGroupName, String vmName) {
Mono>> mono = redeployWithResponseAsync(resourceGroupName, vmName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Shuts down the virtual machine, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginRedeployAsync(String resourceGroupName, String vmName,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono = redeployWithResponseAsync(resourceGroupName, vmName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Shuts down the virtual machine, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginRedeploy(String resourceGroupName, String vmName) {
return this.beginRedeployAsync(resourceGroupName, vmName).getSyncPoller();
}
/**
* Shuts down the virtual machine, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginRedeploy(String resourceGroupName, String vmName, Context context) {
return this.beginRedeployAsync(resourceGroupName, vmName, context).getSyncPoller();
}
/**
* Shuts down the virtual machine, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono redeployAsync(String resourceGroupName, String vmName) {
return beginRedeployAsync(resourceGroupName, vmName).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Shuts down the virtual machine, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono redeployAsync(String resourceGroupName, String vmName, Context context) {
return beginRedeployAsync(resourceGroupName, vmName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Shuts down the virtual machine, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void redeploy(String resourceGroupName, String vmName) {
redeployAsync(resourceGroupName, vmName).block();
}
/**
* Shuts down the virtual machine, moves it to a new node, and powers it back on.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void redeploy(String resourceGroupName, String vmName, Context context) {
redeployAsync(resourceGroupName, vmName, context).block();
}
/**
* Reimages (upgrade the operating system) a virtual machine which don't have a ephemeral OS disk, for virtual
* machines who have a ephemeral OS disk the virtual machine is reset to initial state. NOTE: The retaining of old
* OS disk depends on the value of deleteOption of OS disk. If deleteOption is detach, the old OS disk will be
* preserved after reimage. If deleteOption is delete, the old OS disk will be deleted after reimage. The
* deleteOption of the OS disk should be updated accordingly before performing the reimage.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Reimage Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> reimageWithResponseAsync(String resourceGroupName, String vmName,
VirtualMachineReimageParameters parameters) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (parameters != null) {
parameters.validate();
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.reimage(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), parameters, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Reimages (upgrade the operating system) a virtual machine which don't have a ephemeral OS disk, for virtual
* machines who have a ephemeral OS disk the virtual machine is reset to initial state. NOTE: The retaining of old
* OS disk depends on the value of deleteOption of OS disk. If deleteOption is detach, the old OS disk will be
* preserved after reimage. If deleteOption is delete, the old OS disk will be deleted after reimage. The
* deleteOption of the OS disk should be updated accordingly before performing the reimage.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Reimage Virtual Machine operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> reimageWithResponseAsync(String resourceGroupName, String vmName,
VirtualMachineReimageParameters parameters, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (parameters != null) {
parameters.validate();
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.reimage(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), parameters, accept, context);
}
/**
* Reimages (upgrade the operating system) a virtual machine which don't have a ephemeral OS disk, for virtual
* machines who have a ephemeral OS disk the virtual machine is reset to initial state. NOTE: The retaining of old
* OS disk depends on the value of deleteOption of OS disk. If deleteOption is detach, the old OS disk will be
* preserved after reimage. If deleteOption is delete, the old OS disk will be deleted after reimage. The
* deleteOption of the OS disk should be updated accordingly before performing the reimage.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Reimage Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginReimageAsync(String resourceGroupName, String vmName,
VirtualMachineReimageParameters parameters) {
Mono>> mono = reimageWithResponseAsync(resourceGroupName, vmName, parameters);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Reimages (upgrade the operating system) a virtual machine which don't have a ephemeral OS disk, for virtual
* machines who have a ephemeral OS disk the virtual machine is reset to initial state. NOTE: The retaining of old
* OS disk depends on the value of deleteOption of OS disk. If deleteOption is detach, the old OS disk will be
* preserved after reimage. If deleteOption is delete, the old OS disk will be deleted after reimage. The
* deleteOption of the OS disk should be updated accordingly before performing the reimage.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginReimageAsync(String resourceGroupName, String vmName) {
final VirtualMachineReimageParameters parameters = null;
Mono>> mono = reimageWithResponseAsync(resourceGroupName, vmName, parameters);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Reimages (upgrade the operating system) a virtual machine which don't have a ephemeral OS disk, for virtual
* machines who have a ephemeral OS disk the virtual machine is reset to initial state. NOTE: The retaining of old
* OS disk depends on the value of deleteOption of OS disk. If deleteOption is detach, the old OS disk will be
* preserved after reimage. If deleteOption is delete, the old OS disk will be deleted after reimage. The
* deleteOption of the OS disk should be updated accordingly before performing the reimage.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Reimage Virtual Machine operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginReimageAsync(String resourceGroupName, String vmName,
VirtualMachineReimageParameters parameters, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= reimageWithResponseAsync(resourceGroupName, vmName, parameters, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Reimages (upgrade the operating system) a virtual machine which don't have a ephemeral OS disk, for virtual
* machines who have a ephemeral OS disk the virtual machine is reset to initial state. NOTE: The retaining of old
* OS disk depends on the value of deleteOption of OS disk. If deleteOption is detach, the old OS disk will be
* preserved after reimage. If deleteOption is delete, the old OS disk will be deleted after reimage. The
* deleteOption of the OS disk should be updated accordingly before performing the reimage.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginReimage(String resourceGroupName, String vmName) {
final VirtualMachineReimageParameters parameters = null;
return this.beginReimageAsync(resourceGroupName, vmName, parameters).getSyncPoller();
}
/**
* Reimages (upgrade the operating system) a virtual machine which don't have a ephemeral OS disk, for virtual
* machines who have a ephemeral OS disk the virtual machine is reset to initial state. NOTE: The retaining of old
* OS disk depends on the value of deleteOption of OS disk. If deleteOption is detach, the old OS disk will be
* preserved after reimage. If deleteOption is delete, the old OS disk will be deleted after reimage. The
* deleteOption of the OS disk should be updated accordingly before performing the reimage.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Reimage Virtual Machine operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginReimage(String resourceGroupName, String vmName,
VirtualMachineReimageParameters parameters, Context context) {
return this.beginReimageAsync(resourceGroupName, vmName, parameters, context).getSyncPoller();
}
/**
* Reimages (upgrade the operating system) a virtual machine which don't have a ephemeral OS disk, for virtual
* machines who have a ephemeral OS disk the virtual machine is reset to initial state. NOTE: The retaining of old
* OS disk depends on the value of deleteOption of OS disk. If deleteOption is detach, the old OS disk will be
* preserved after reimage. If deleteOption is delete, the old OS disk will be deleted after reimage. The
* deleteOption of the OS disk should be updated accordingly before performing the reimage.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Reimage Virtual Machine operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono reimageAsync(String resourceGroupName, String vmName,
VirtualMachineReimageParameters parameters) {
return beginReimageAsync(resourceGroupName, vmName, parameters).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Reimages (upgrade the operating system) a virtual machine which don't have a ephemeral OS disk, for virtual
* machines who have a ephemeral OS disk the virtual machine is reset to initial state. NOTE: The retaining of old
* OS disk depends on the value of deleteOption of OS disk. If deleteOption is detach, the old OS disk will be
* preserved after reimage. If deleteOption is delete, the old OS disk will be deleted after reimage. The
* deleteOption of the OS disk should be updated accordingly before performing the reimage.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono reimageAsync(String resourceGroupName, String vmName) {
final VirtualMachineReimageParameters parameters = null;
return beginReimageAsync(resourceGroupName, vmName, parameters).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Reimages (upgrade the operating system) a virtual machine which don't have a ephemeral OS disk, for virtual
* machines who have a ephemeral OS disk the virtual machine is reset to initial state. NOTE: The retaining of old
* OS disk depends on the value of deleteOption of OS disk. If deleteOption is detach, the old OS disk will be
* preserved after reimage. If deleteOption is delete, the old OS disk will be deleted after reimage. The
* deleteOption of the OS disk should be updated accordingly before performing the reimage.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Reimage Virtual Machine operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono reimageAsync(String resourceGroupName, String vmName, VirtualMachineReimageParameters parameters,
Context context) {
return beginReimageAsync(resourceGroupName, vmName, parameters, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Reimages (upgrade the operating system) a virtual machine which don't have a ephemeral OS disk, for virtual
* machines who have a ephemeral OS disk the virtual machine is reset to initial state. NOTE: The retaining of old
* OS disk depends on the value of deleteOption of OS disk. If deleteOption is detach, the old OS disk will be
* preserved after reimage. If deleteOption is delete, the old OS disk will be deleted after reimage. The
* deleteOption of the OS disk should be updated accordingly before performing the reimage.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void reimage(String resourceGroupName, String vmName) {
final VirtualMachineReimageParameters parameters = null;
reimageAsync(resourceGroupName, vmName, parameters).block();
}
/**
* Reimages (upgrade the operating system) a virtual machine which don't have a ephemeral OS disk, for virtual
* machines who have a ephemeral OS disk the virtual machine is reset to initial state. NOTE: The retaining of old
* OS disk depends on the value of deleteOption of OS disk. If deleteOption is detach, the old OS disk will be
* preserved after reimage. If deleteOption is delete, the old OS disk will be deleted after reimage. The
* deleteOption of the OS disk should be updated accordingly before performing the reimage.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param parameters Parameters supplied to the Reimage Virtual Machine operation.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void reimage(String resourceGroupName, String vmName, VirtualMachineReimageParameters parameters,
Context context) {
reimageAsync(resourceGroupName, vmName, parameters, context).block();
}
/**
* The operation to retrieve SAS URIs for a virtual machine's boot diagnostic logs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param sasUriExpirationTimeInMinutes Expiration duration in minutes for the SAS URIs with a value between 1 to
* 1440 minutes. **Note:** If not specified, SAS URIs will be generated with a default expiration duration of 120
* minutes.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SAS URIs of the console screenshot and serial log blobs along with {@link Response} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> retrieveBootDiagnosticsDataWithResponseAsync(
String resourceGroupName, String vmName, Integer sasUriExpirationTimeInMinutes) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.retrieveBootDiagnosticsData(this.client.getEndpoint(), resourceGroupName,
vmName, sasUriExpirationTimeInMinutes, apiVersion, this.client.getSubscriptionId(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* The operation to retrieve SAS URIs for a virtual machine's boot diagnostic logs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param sasUriExpirationTimeInMinutes Expiration duration in minutes for the SAS URIs with a value between 1 to
* 1440 minutes. **Note:** If not specified, SAS URIs will be generated with a default expiration duration of 120
* minutes.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SAS URIs of the console screenshot and serial log blobs along with {@link Response} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> retrieveBootDiagnosticsDataWithResponseAsync(
String resourceGroupName, String vmName, Integer sasUriExpirationTimeInMinutes, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.retrieveBootDiagnosticsData(this.client.getEndpoint(), resourceGroupName, vmName,
sasUriExpirationTimeInMinutes, apiVersion, this.client.getSubscriptionId(), accept, context);
}
/**
* The operation to retrieve SAS URIs for a virtual machine's boot diagnostic logs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SAS URIs of the console screenshot and serial log blobs on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono retrieveBootDiagnosticsDataAsync(String resourceGroupName,
String vmName) {
final Integer sasUriExpirationTimeInMinutes = null;
return retrieveBootDiagnosticsDataWithResponseAsync(resourceGroupName, vmName, sasUriExpirationTimeInMinutes)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* The operation to retrieve SAS URIs for a virtual machine's boot diagnostic logs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param sasUriExpirationTimeInMinutes Expiration duration in minutes for the SAS URIs with a value between 1 to
* 1440 minutes. **Note:** If not specified, SAS URIs will be generated with a default expiration duration of 120
* minutes.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SAS URIs of the console screenshot and serial log blobs along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response retrieveBootDiagnosticsDataWithResponse(
String resourceGroupName, String vmName, Integer sasUriExpirationTimeInMinutes, Context context) {
return retrieveBootDiagnosticsDataWithResponseAsync(resourceGroupName, vmName, sasUriExpirationTimeInMinutes,
context).block();
}
/**
* The operation to retrieve SAS URIs for a virtual machine's boot diagnostic logs.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SAS URIs of the console screenshot and serial log blobs.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public RetrieveBootDiagnosticsDataResultInner retrieveBootDiagnosticsData(String resourceGroupName, String vmName) {
final Integer sasUriExpirationTimeInMinutes = null;
return retrieveBootDiagnosticsDataWithResponse(resourceGroupName, vmName, sasUriExpirationTimeInMinutes,
Context.NONE).getValue();
}
/**
* The operation to perform maintenance on a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> performMaintenanceWithResponseAsync(String resourceGroupName,
String vmName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.performMaintenance(this.client.getEndpoint(), resourceGroupName, vmName,
apiVersion, this.client.getSubscriptionId(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* The operation to perform maintenance on a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> performMaintenanceWithResponseAsync(String resourceGroupName,
String vmName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (vmName == null) {
return Mono.error(new IllegalArgumentException("Parameter vmName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String apiVersion = "2024-07-01";
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.performMaintenance(this.client.getEndpoint(), resourceGroupName, vmName, apiVersion,
this.client.getSubscriptionId(), accept, context);
}
/**
* The operation to perform maintenance on a virtual machine.
*
* @param resourceGroupName The name of the resource group.
* @param vmName The name of the virtual machine.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ApiErrorException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginPerformMaintenanceAsync(String resourceGroupName, String vmName) {
Mono>> mono = performMaintenanceWithResponseAsync(resourceGroupName, vmName);
return this.client.