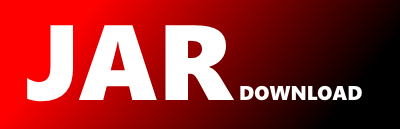
com.azure.resourcemanager.compute.models.ImageDeprecationStatus Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-compute Show documentation
Show all versions of azure-resourcemanager-compute Show documentation
This package contains Microsoft Azure Compute Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.compute.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
/**
* Describes image deprecation status properties on the image.
*/
@Fluent
public final class ImageDeprecationStatus implements JsonSerializable {
/*
* Describes the state of the image.
*/
private ImageState imageState;
/*
* The time, in future, at which this image will be marked as deprecated. This scheduled time is chosen by the
* Publisher.
*/
private OffsetDateTime scheduledDeprecationTime;
/*
* Describes the alternative option specified by the Publisher for this image when this image is deprecated.
*/
private AlternativeOption alternativeOption;
/**
* Creates an instance of ImageDeprecationStatus class.
*/
public ImageDeprecationStatus() {
}
/**
* Get the imageState property: Describes the state of the image.
*
* @return the imageState value.
*/
public ImageState imageState() {
return this.imageState;
}
/**
* Set the imageState property: Describes the state of the image.
*
* @param imageState the imageState value to set.
* @return the ImageDeprecationStatus object itself.
*/
public ImageDeprecationStatus withImageState(ImageState imageState) {
this.imageState = imageState;
return this;
}
/**
* Get the scheduledDeprecationTime property: The time, in future, at which this image will be marked as deprecated.
* This scheduled time is chosen by the Publisher.
*
* @return the scheduledDeprecationTime value.
*/
public OffsetDateTime scheduledDeprecationTime() {
return this.scheduledDeprecationTime;
}
/**
* Set the scheduledDeprecationTime property: The time, in future, at which this image will be marked as deprecated.
* This scheduled time is chosen by the Publisher.
*
* @param scheduledDeprecationTime the scheduledDeprecationTime value to set.
* @return the ImageDeprecationStatus object itself.
*/
public ImageDeprecationStatus withScheduledDeprecationTime(OffsetDateTime scheduledDeprecationTime) {
this.scheduledDeprecationTime = scheduledDeprecationTime;
return this;
}
/**
* Get the alternativeOption property: Describes the alternative option specified by the Publisher for this image
* when this image is deprecated.
*
* @return the alternativeOption value.
*/
public AlternativeOption alternativeOption() {
return this.alternativeOption;
}
/**
* Set the alternativeOption property: Describes the alternative option specified by the Publisher for this image
* when this image is deprecated.
*
* @param alternativeOption the alternativeOption value to set.
* @return the ImageDeprecationStatus object itself.
*/
public ImageDeprecationStatus withAlternativeOption(AlternativeOption alternativeOption) {
this.alternativeOption = alternativeOption;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (alternativeOption() != null) {
alternativeOption().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("imageState", this.imageState == null ? null : this.imageState.toString());
jsonWriter.writeStringField("scheduledDeprecationTime",
this.scheduledDeprecationTime == null
? null
: DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.scheduledDeprecationTime));
jsonWriter.writeJsonField("alternativeOption", this.alternativeOption);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ImageDeprecationStatus from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ImageDeprecationStatus if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the ImageDeprecationStatus.
*/
public static ImageDeprecationStatus fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ImageDeprecationStatus deserializedImageDeprecationStatus = new ImageDeprecationStatus();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("imageState".equals(fieldName)) {
deserializedImageDeprecationStatus.imageState = ImageState.fromString(reader.getString());
} else if ("scheduledDeprecationTime".equals(fieldName)) {
deserializedImageDeprecationStatus.scheduledDeprecationTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("alternativeOption".equals(fieldName)) {
deserializedImageDeprecationStatus.alternativeOption = AlternativeOption.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedImageDeprecationStatus;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy