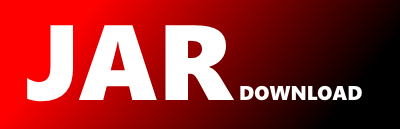
com.azure.resourcemanager.compute.models.ImageReference Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-compute Show documentation
Show all versions of azure-resourcemanager-compute Show documentation
This package contains Microsoft Azure Compute Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.compute.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.SubResource;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Specifies information about the image to use. You can specify information about platform images, marketplace images,
* or virtual machine images. This element is required when you want to use a platform image, marketplace image, or
* virtual machine image, but is not used in other creation operations. NOTE: Image reference publisher and offer can
* only be set when you create the scale set.
*/
@Fluent
public final class ImageReference extends SubResource {
/*
* The image publisher.
*/
private String publisher;
/*
* Specifies the offer of the platform image or marketplace image used to create the virtual machine.
*/
private String offer;
/*
* The image SKU.
*/
private String sku;
/*
* Specifies the version of the platform image or marketplace image used to create the virtual machine. The allowed
* formats are Major.Minor.Build or 'latest'. Major, Minor, and Build are decimal numbers. Specify 'latest' to use
* the latest version of an image available at deploy time. Even if you use 'latest', the VM image will not
* automatically update after deploy time even if a new version becomes available. Please do not use field 'version'
* for gallery image deployment, gallery image should always use 'id' field for deployment, to use 'latest' version
* of gallery image, just set
* '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/galleries/{
* galleryName}/images/{imageName}' in the 'id' field without version input.
*/
private String version;
/*
* Specifies in decimal numbers, the version of platform image or marketplace image used to create the virtual
* machine. This readonly field differs from 'version', only if the value specified in 'version' field is 'latest'.
*/
private String exactVersion;
/*
* Specified the shared gallery image unique id for vm deployment. This can be fetched from shared gallery image GET
* call.
*/
private String sharedGalleryImageId;
/*
* Specified the community gallery image unique id for vm deployment. This can be fetched from community gallery
* image GET call.
*/
private String communityGalleryImageId;
/**
* Creates an instance of ImageReference class.
*/
public ImageReference() {
}
/**
* Get the publisher property: The image publisher.
*
* @return the publisher value.
*/
public String publisher() {
return this.publisher;
}
/**
* Set the publisher property: The image publisher.
*
* @param publisher the publisher value to set.
* @return the ImageReference object itself.
*/
public ImageReference withPublisher(String publisher) {
this.publisher = publisher;
return this;
}
/**
* Get the offer property: Specifies the offer of the platform image or marketplace image used to create the virtual
* machine.
*
* @return the offer value.
*/
public String offer() {
return this.offer;
}
/**
* Set the offer property: Specifies the offer of the platform image or marketplace image used to create the virtual
* machine.
*
* @param offer the offer value to set.
* @return the ImageReference object itself.
*/
public ImageReference withOffer(String offer) {
this.offer = offer;
return this;
}
/**
* Get the sku property: The image SKU.
*
* @return the sku value.
*/
public String sku() {
return this.sku;
}
/**
* Set the sku property: The image SKU.
*
* @param sku the sku value to set.
* @return the ImageReference object itself.
*/
public ImageReference withSku(String sku) {
this.sku = sku;
return this;
}
/**
* Get the version property: Specifies the version of the platform image or marketplace image used to create the
* virtual machine. The allowed formats are Major.Minor.Build or 'latest'. Major, Minor, and Build are decimal
* numbers. Specify 'latest' to use the latest version of an image available at deploy time. Even if you use
* 'latest', the VM image will not automatically update after deploy time even if a new version becomes available.
* Please do not use field 'version' for gallery image deployment, gallery image should always use 'id' field for
* deployment, to use 'latest' version of gallery image, just set
* '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/galleries/{galleryName}/images/{imageName}'
* in the 'id' field without version input.
*
* @return the version value.
*/
public String version() {
return this.version;
}
/**
* Set the version property: Specifies the version of the platform image or marketplace image used to create the
* virtual machine. The allowed formats are Major.Minor.Build or 'latest'. Major, Minor, and Build are decimal
* numbers. Specify 'latest' to use the latest version of an image available at deploy time. Even if you use
* 'latest', the VM image will not automatically update after deploy time even if a new version becomes available.
* Please do not use field 'version' for gallery image deployment, gallery image should always use 'id' field for
* deployment, to use 'latest' version of gallery image, just set
* '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/galleries/{galleryName}/images/{imageName}'
* in the 'id' field without version input.
*
* @param version the version value to set.
* @return the ImageReference object itself.
*/
public ImageReference withVersion(String version) {
this.version = version;
return this;
}
/**
* Get the exactVersion property: Specifies in decimal numbers, the version of platform image or marketplace image
* used to create the virtual machine. This readonly field differs from 'version', only if the value specified in
* 'version' field is 'latest'.
*
* @return the exactVersion value.
*/
public String exactVersion() {
return this.exactVersion;
}
/**
* Get the sharedGalleryImageId property: Specified the shared gallery image unique id for vm deployment. This can
* be fetched from shared gallery image GET call.
*
* @return the sharedGalleryImageId value.
*/
public String sharedGalleryImageId() {
return this.sharedGalleryImageId;
}
/**
* Set the sharedGalleryImageId property: Specified the shared gallery image unique id for vm deployment. This can
* be fetched from shared gallery image GET call.
*
* @param sharedGalleryImageId the sharedGalleryImageId value to set.
* @return the ImageReference object itself.
*/
public ImageReference withSharedGalleryImageId(String sharedGalleryImageId) {
this.sharedGalleryImageId = sharedGalleryImageId;
return this;
}
/**
* Get the communityGalleryImageId property: Specified the community gallery image unique id for vm deployment. This
* can be fetched from community gallery image GET call.
*
* @return the communityGalleryImageId value.
*/
public String communityGalleryImageId() {
return this.communityGalleryImageId;
}
/**
* Set the communityGalleryImageId property: Specified the community gallery image unique id for vm deployment. This
* can be fetched from community gallery image GET call.
*
* @param communityGalleryImageId the communityGalleryImageId value to set.
* @return the ImageReference object itself.
*/
public ImageReference withCommunityGalleryImageId(String communityGalleryImageId) {
this.communityGalleryImageId = communityGalleryImageId;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public ImageReference withId(String id) {
super.withId(id);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("id", id());
jsonWriter.writeStringField("publisher", this.publisher);
jsonWriter.writeStringField("offer", this.offer);
jsonWriter.writeStringField("sku", this.sku);
jsonWriter.writeStringField("version", this.version);
jsonWriter.writeStringField("sharedGalleryImageId", this.sharedGalleryImageId);
jsonWriter.writeStringField("communityGalleryImageId", this.communityGalleryImageId);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ImageReference from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ImageReference if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the ImageReference.
*/
public static ImageReference fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ImageReference deserializedImageReference = new ImageReference();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("id".equals(fieldName)) {
deserializedImageReference.withId(reader.getString());
} else if ("publisher".equals(fieldName)) {
deserializedImageReference.publisher = reader.getString();
} else if ("offer".equals(fieldName)) {
deserializedImageReference.offer = reader.getString();
} else if ("sku".equals(fieldName)) {
deserializedImageReference.sku = reader.getString();
} else if ("version".equals(fieldName)) {
deserializedImageReference.version = reader.getString();
} else if ("exactVersion".equals(fieldName)) {
deserializedImageReference.exactVersion = reader.getString();
} else if ("sharedGalleryImageId".equals(fieldName)) {
deserializedImageReference.sharedGalleryImageId = reader.getString();
} else if ("communityGalleryImageId".equals(fieldName)) {
deserializedImageReference.communityGalleryImageId = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedImageReference;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy