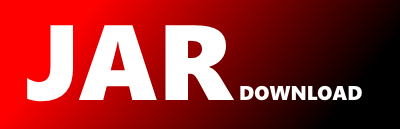
com.azure.resourcemanager.cosmos.fluent.MongoDBResourcesClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-cosmos Show documentation
Show all versions of azure-resourcemanager-cosmos Show documentation
This package contains Microsoft Azure CosmosDB SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.cosmos.fluent;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.polling.PollerFlux;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.cosmos.fluent.models.BackupInformationInner;
import com.azure.resourcemanager.cosmos.fluent.models.MongoDBCollectionGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.MongoDBDatabaseGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.MongoRoleDefinitionGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.MongoUserDefinitionGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.ThroughputSettingsGetResultsInner;
import com.azure.resourcemanager.cosmos.models.ContinuousBackupRestoreLocation;
import com.azure.resourcemanager.cosmos.models.MongoDBCollectionCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.MongoDBDatabaseCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.MongoRoleDefinitionCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.MongoUserDefinitionCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.ThroughputSettingsUpdateParameters;
import java.nio.ByteBuffer;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* An instance of this class provides access to all the operations defined in MongoDBResourcesClient.
*/
public interface MongoDBResourcesClient {
/**
* Lists the MongoDB databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB databases and their properties as paginated
* response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listMongoDBDatabasesAsync(String resourceGroupName, String accountName);
/**
* Lists the MongoDB databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB databases and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listMongoDBDatabases(String resourceGroupName, String accountName);
/**
* Lists the MongoDB databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB databases and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listMongoDBDatabases(String resourceGroupName, String accountName,
Context context);
/**
* Gets the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB databases under an existing Azure Cosmos DB database account with the provided name along
* with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getMongoDBDatabaseWithResponseAsync(String resourceGroupName,
String accountName, String databaseName);
/**
* Gets the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB databases under an existing Azure Cosmos DB database account with the provided name on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getMongoDBDatabaseAsync(String resourceGroupName, String accountName,
String databaseName);
/**
* Gets the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB databases under an existing Azure Cosmos DB database account with the provided name along
* with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getMongoDBDatabaseWithResponse(String resourceGroupName,
String accountName, String databaseName, Context context);
/**
* Gets the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MongoDBDatabaseGetResultsInner getMongoDBDatabase(String resourceGroupName, String accountName,
String databaseName);
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB database along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createUpdateMongoDBDatabaseWithResponseAsync(String resourceGroupName,
String accountName, String databaseName,
MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters);
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB MongoDB database.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, MongoDBDatabaseGetResultsInner>
beginCreateUpdateMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName,
MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters);
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB MongoDB database.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, MongoDBDatabaseGetResultsInner>
beginCreateUpdateMongoDBDatabase(String resourceGroupName, String accountName, String databaseName,
MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters);
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB MongoDB database.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, MongoDBDatabaseGetResultsInner>
beginCreateUpdateMongoDBDatabase(String resourceGroupName, String accountName, String databaseName,
MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters, Context context);
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB database on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createUpdateMongoDBDatabaseAsync(String resourceGroupName, String accountName,
String databaseName, MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters);
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB database.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MongoDBDatabaseGetResultsInner createUpdateMongoDBDatabase(String resourceGroupName, String accountName,
String databaseName, MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters);
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB database.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MongoDBDatabaseGetResultsInner createUpdateMongoDBDatabase(String resourceGroupName, String accountName,
String databaseName, MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters,
Context context);
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deleteMongoDBDatabaseWithResponseAsync(String resourceGroupName,
String accountName, String databaseName);
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteMongoDBDatabaseAsync(String resourceGroupName, String accountName,
String databaseName);
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteMongoDBDatabase(String resourceGroupName, String accountName,
String databaseName);
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteMongoDBDatabase(String resourceGroupName, String accountName,
String databaseName, Context context);
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName);
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteMongoDBDatabase(String resourceGroupName, String accountName, String databaseName);
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteMongoDBDatabase(String resourceGroupName, String accountName, String databaseName, Context context);
/**
* Gets the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getMongoDBDatabaseThroughputWithResponseAsync(
String resourceGroupName, String accountName, String databaseName);
/**
* Gets the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getMongoDBDatabaseThroughputAsync(String resourceGroupName,
String accountName, String databaseName);
/**
* Gets the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getMongoDBDatabaseThroughputWithResponse(String resourceGroupName,
String accountName, String databaseName, Context context);
/**
* Gets the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner getMongoDBDatabaseThroughput(String resourceGroupName, String accountName,
String databaseName);
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> updateMongoDBDatabaseThroughputWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginUpdateMongoDBDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName,
ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateMongoDBDatabaseThroughput(String resourceGroupName, String accountName, String databaseName,
ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateMongoDBDatabaseThroughput(String resourceGroupName, String accountName, String databaseName,
ThroughputSettingsUpdateParameters updateThroughputParameters, Context context);
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono updateMongoDBDatabaseThroughputAsync(String resourceGroupName,
String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner updateMongoDBDatabaseThroughput(String resourceGroupName, String accountName,
String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner updateMongoDBDatabaseThroughput(String resourceGroupName, String accountName,
String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters, Context context);
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> migrateMongoDBDatabaseToAutoscaleWithResponseAsync(String resourceGroupName,
String accountName, String databaseName);
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBDatabaseToAutoscaleAsync(String resourceGroupName, String accountName, String databaseName);
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBDatabaseToAutoscale(String resourceGroupName, String accountName, String databaseName);
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBDatabaseToAutoscale(String resourceGroupName, String accountName, String databaseName,
Context context);
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono migrateMongoDBDatabaseToAutoscaleAsync(String resourceGroupName,
String accountName, String databaseName);
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateMongoDBDatabaseToAutoscale(String resourceGroupName, String accountName,
String databaseName);
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateMongoDBDatabaseToAutoscale(String resourceGroupName, String accountName,
String databaseName, Context context);
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> migrateMongoDBDatabaseToManualThroughputWithResponseAsync(String resourceGroupName,
String accountName, String databaseName);
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBDatabaseToManualThroughputAsync(String resourceGroupName, String accountName,
String databaseName);
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBDatabaseToManualThroughput(String resourceGroupName, String accountName,
String databaseName);
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBDatabaseToManualThroughput(String resourceGroupName, String accountName, String databaseName,
Context context);
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono migrateMongoDBDatabaseToManualThroughputAsync(String resourceGroupName,
String accountName, String databaseName);
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateMongoDBDatabaseToManualThroughput(String resourceGroupName,
String accountName, String databaseName);
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateMongoDBDatabaseToManualThroughput(String resourceGroupName,
String accountName, String databaseName, Context context);
/**
* Lists the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB collections and their properties as paginated
* response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listMongoDBCollectionsAsync(String resourceGroupName,
String accountName, String databaseName);
/**
* Lists the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB collections and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listMongoDBCollections(String resourceGroupName, String accountName,
String databaseName);
/**
* Lists the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB collections and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listMongoDBCollections(String resourceGroupName, String accountName,
String databaseName, Context context);
/**
* Gets the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB collection under an existing Azure Cosmos DB database account along with {@link Response} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getMongoDBCollectionWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName);
/**
* Gets the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB collection under an existing Azure Cosmos DB database account on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getMongoDBCollectionAsync(String resourceGroupName, String accountName,
String databaseName, String collectionName);
/**
* Gets the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB collection under an existing Azure Cosmos DB database account along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getMongoDBCollectionWithResponse(String resourceGroupName,
String accountName, String databaseName, String collectionName, Context context);
/**
* Gets the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB collection under an existing Azure Cosmos DB database account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MongoDBCollectionGetResultsInner getMongoDBCollection(String resourceGroupName, String accountName,
String databaseName, String collectionName);
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB collection along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createUpdateMongoDBCollectionWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName,
MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters);
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB MongoDB collection.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, MongoDBCollectionGetResultsInner>
beginCreateUpdateMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName,
String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters);
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB MongoDB collection.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, MongoDBCollectionGetResultsInner>
beginCreateUpdateMongoDBCollection(String resourceGroupName, String accountName, String databaseName,
String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters);
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB MongoDB collection.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, MongoDBCollectionGetResultsInner>
beginCreateUpdateMongoDBCollection(String resourceGroupName, String accountName, String databaseName,
String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters,
Context context);
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB collection on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createUpdateMongoDBCollectionAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName,
MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters);
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB collection.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MongoDBCollectionGetResultsInner createUpdateMongoDBCollection(String resourceGroupName, String accountName,
String databaseName, String collectionName,
MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters);
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB collection.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MongoDBCollectionGetResultsInner createUpdateMongoDBCollection(String resourceGroupName, String accountName,
String databaseName, String collectionName,
MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters, Context context);
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deleteMongoDBCollectionWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName);
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteMongoDBCollectionAsync(String resourceGroupName, String accountName,
String databaseName, String collectionName);
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteMongoDBCollection(String resourceGroupName, String accountName,
String databaseName, String collectionName);
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteMongoDBCollection(String resourceGroupName, String accountName,
String databaseName, String collectionName, Context context);
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName,
String collectionName);
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteMongoDBCollection(String resourceGroupName, String accountName, String databaseName,
String collectionName);
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteMongoDBCollection(String resourceGroupName, String accountName, String databaseName,
String collectionName, Context context);
/**
* Gets the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getMongoDBCollectionThroughputWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName);
/**
* Gets the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getMongoDBCollectionThroughputAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName);
/**
* Gets the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getMongoDBCollectionThroughputWithResponse(String resourceGroupName,
String accountName, String databaseName, String collectionName, Context context);
/**
* Gets the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner getMongoDBCollectionThroughput(String resourceGroupName, String accountName,
String databaseName, String collectionName);
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> updateMongoDBCollectionThroughputWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName,
ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginUpdateMongoDBCollectionThroughputAsync(String resourceGroupName, String accountName, String databaseName,
String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateMongoDBCollectionThroughput(String resourceGroupName, String accountName, String databaseName,
String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateMongoDBCollectionThroughput(String resourceGroupName, String accountName, String databaseName,
String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters, Context context);
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono updateMongoDBCollectionThroughputAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName,
ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner updateMongoDBCollectionThroughput(String resourceGroupName, String accountName,
String databaseName, String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner updateMongoDBCollectionThroughput(String resourceGroupName, String accountName,
String databaseName, String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters,
Context context);
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> migrateMongoDBCollectionToAutoscaleWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName);
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBCollectionToAutoscaleAsync(String resourceGroupName, String accountName, String databaseName,
String collectionName);
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBCollectionToAutoscale(String resourceGroupName, String accountName, String databaseName,
String collectionName);
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBCollectionToAutoscale(String resourceGroupName, String accountName, String databaseName,
String collectionName, Context context);
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono migrateMongoDBCollectionToAutoscaleAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName);
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateMongoDBCollectionToAutoscale(String resourceGroupName, String accountName,
String databaseName, String collectionName);
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateMongoDBCollectionToAutoscale(String resourceGroupName, String accountName,
String databaseName, String collectionName, Context context);
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> migrateMongoDBCollectionToManualThroughputWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName);
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBCollectionToManualThroughputAsync(String resourceGroupName, String accountName,
String databaseName, String collectionName);
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBCollectionToManualThroughput(String resourceGroupName, String accountName,
String databaseName, String collectionName);
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBCollectionToManualThroughput(String resourceGroupName, String accountName,
String databaseName, String collectionName, Context context);
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono migrateMongoDBCollectionToManualThroughputAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName);
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateMongoDBCollectionToManualThroughput(String resourceGroupName,
String accountName, String databaseName, String collectionName);
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateMongoDBCollectionToManualThroughput(String resourceGroupName,
String accountName, String databaseName, String collectionName, Context context);
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo Role Definition with the given Id.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getMongoRoleDefinitionWithResponseAsync(
String mongoRoleDefinitionId, String resourceGroupName, String accountName);
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo Role Definition with the given Id.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getMongoRoleDefinitionAsync(String mongoRoleDefinitionId,
String resourceGroupName, String accountName);
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo Role Definition with the given Id.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getMongoRoleDefinitionWithResponse(String mongoRoleDefinitionId,
String resourceGroupName, String accountName, Context context);
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo Role Definition with the given Id.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MongoRoleDefinitionGetResultsInner getMongoRoleDefinition(String mongoRoleDefinitionId, String resourceGroupName,
String accountName);
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createUpdateMongoRoleDefinitionWithResponseAsync(String mongoRoleDefinitionId,
String resourceGroupName, String accountName,
MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB Mongo Role Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, MongoRoleDefinitionGetResultsInner>
beginCreateUpdateMongoRoleDefinitionAsync(String mongoRoleDefinitionId, String resourceGroupName,
String accountName, MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB Mongo Role Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, MongoRoleDefinitionGetResultsInner>
beginCreateUpdateMongoRoleDefinition(String mongoRoleDefinitionId, String resourceGroupName, String accountName,
MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB Mongo Role Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, MongoRoleDefinitionGetResultsInner>
beginCreateUpdateMongoRoleDefinition(String mongoRoleDefinitionId, String resourceGroupName, String accountName,
MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters, Context context);
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createUpdateMongoRoleDefinitionAsync(String mongoRoleDefinitionId,
String resourceGroupName, String accountName,
MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MongoRoleDefinitionGetResultsInner createUpdateMongoRoleDefinition(String mongoRoleDefinitionId,
String resourceGroupName, String accountName,
MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MongoRoleDefinitionGetResultsInner createUpdateMongoRoleDefinition(String mongoRoleDefinitionId,
String resourceGroupName, String accountName,
MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters, Context context);
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deleteMongoRoleDefinitionWithResponseAsync(String mongoRoleDefinitionId,
String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteMongoRoleDefinitionAsync(String mongoRoleDefinitionId,
String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteMongoRoleDefinition(String mongoRoleDefinitionId,
String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteMongoRoleDefinition(String mongoRoleDefinitionId,
String resourceGroupName, String accountName, Context context);
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteMongoRoleDefinitionAsync(String mongoRoleDefinitionId, String resourceGroupName,
String accountName);
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteMongoRoleDefinition(String mongoRoleDefinitionId, String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteMongoRoleDefinition(String mongoRoleDefinitionId, String resourceGroupName, String accountName,
Context context);
/**
* Retrieves the list of all Azure Cosmos DB Mongo Role Definitions.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Mongo Role Definitions as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listMongoRoleDefinitionsAsync(String resourceGroupName,
String accountName);
/**
* Retrieves the list of all Azure Cosmos DB Mongo Role Definitions.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Mongo Role Definitions as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listMongoRoleDefinitions(String resourceGroupName,
String accountName);
/**
* Retrieves the list of all Azure Cosmos DB Mongo Role Definitions.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Mongo Role Definitions as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listMongoRoleDefinitions(String resourceGroupName,
String accountName, Context context);
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo User Definition with the given Id.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getMongoUserDefinitionWithResponseAsync(
String mongoUserDefinitionId, String resourceGroupName, String accountName);
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo User Definition with the given Id.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getMongoUserDefinitionAsync(String mongoUserDefinitionId,
String resourceGroupName, String accountName);
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo User Definition with the given Id.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getMongoUserDefinitionWithResponse(String mongoUserDefinitionId,
String resourceGroupName, String accountName, Context context);
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo User Definition with the given Id.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MongoUserDefinitionGetResultsInner getMongoUserDefinition(String mongoUserDefinitionId, String resourceGroupName,
String accountName);
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createUpdateMongoUserDefinitionWithResponseAsync(String mongoUserDefinitionId,
String resourceGroupName, String accountName,
MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB User Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, MongoUserDefinitionGetResultsInner>
beginCreateUpdateMongoUserDefinitionAsync(String mongoUserDefinitionId, String resourceGroupName,
String accountName, MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB User Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, MongoUserDefinitionGetResultsInner>
beginCreateUpdateMongoUserDefinition(String mongoUserDefinitionId, String resourceGroupName, String accountName,
MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB User Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, MongoUserDefinitionGetResultsInner>
beginCreateUpdateMongoUserDefinition(String mongoUserDefinitionId, String resourceGroupName, String accountName,
MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters, Context context);
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createUpdateMongoUserDefinitionAsync(String mongoUserDefinitionId,
String resourceGroupName, String accountName,
MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MongoUserDefinitionGetResultsInner createUpdateMongoUserDefinition(String mongoUserDefinitionId,
String resourceGroupName, String accountName,
MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
MongoUserDefinitionGetResultsInner createUpdateMongoUserDefinition(String mongoUserDefinitionId,
String resourceGroupName, String accountName,
MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters, Context context);
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deleteMongoUserDefinitionWithResponseAsync(String mongoUserDefinitionId,
String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteMongoUserDefinitionAsync(String mongoUserDefinitionId,
String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteMongoUserDefinition(String mongoUserDefinitionId,
String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteMongoUserDefinition(String mongoUserDefinitionId,
String resourceGroupName, String accountName, Context context);
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteMongoUserDefinitionAsync(String mongoUserDefinitionId, String resourceGroupName,
String accountName);
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteMongoUserDefinition(String mongoUserDefinitionId, String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteMongoUserDefinition(String mongoUserDefinitionId, String resourceGroupName, String accountName,
Context context);
/**
* Retrieves the list of all Azure Cosmos DB Mongo User Definition.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant User Definition as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listMongoUserDefinitionsAsync(String resourceGroupName,
String accountName);
/**
* Retrieves the list of all Azure Cosmos DB Mongo User Definition.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant User Definition as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listMongoUserDefinitions(String resourceGroupName,
String accountName);
/**
* Retrieves the list of all Azure Cosmos DB Mongo User Definition.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant User Definition as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listMongoUserDefinitions(String resourceGroupName,
String accountName, Context context);
/**
* Retrieves continuous backup information for a Mongodb collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param location The name of the continuous backup restore location.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return backup information of a resource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> retrieveContinuousBackupInformationWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName, ContinuousBackupRestoreLocation location);
/**
* Retrieves continuous backup information for a Mongodb collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param location The name of the continuous backup restore location.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of backup information of a resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, BackupInformationInner>
beginRetrieveContinuousBackupInformationAsync(String resourceGroupName, String accountName, String databaseName,
String collectionName, ContinuousBackupRestoreLocation location);
/**
* Retrieves continuous backup information for a Mongodb collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param location The name of the continuous backup restore location.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of backup information of a resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, BackupInformationInner> beginRetrieveContinuousBackupInformation(
String resourceGroupName, String accountName, String databaseName, String collectionName,
ContinuousBackupRestoreLocation location);
/**
* Retrieves continuous backup information for a Mongodb collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param location The name of the continuous backup restore location.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of backup information of a resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, BackupInformationInner> beginRetrieveContinuousBackupInformation(
String resourceGroupName, String accountName, String databaseName, String collectionName,
ContinuousBackupRestoreLocation location, Context context);
/**
* Retrieves continuous backup information for a Mongodb collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param location The name of the continuous backup restore location.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return backup information of a resource on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono retrieveContinuousBackupInformationAsync(String resourceGroupName, String accountName,
String databaseName, String collectionName, ContinuousBackupRestoreLocation location);
/**
* Retrieves continuous backup information for a Mongodb collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param location The name of the continuous backup restore location.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return backup information of a resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
BackupInformationInner retrieveContinuousBackupInformation(String resourceGroupName, String accountName,
String databaseName, String collectionName, ContinuousBackupRestoreLocation location);
/**
* Retrieves continuous backup information for a Mongodb collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param location The name of the continuous backup restore location.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return backup information of a resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
BackupInformationInner retrieveContinuousBackupInformation(String resourceGroupName, String accountName,
String databaseName, String collectionName, ContinuousBackupRestoreLocation location, Context context);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy