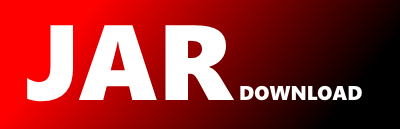
com.azure.resourcemanager.cosmos.fluent.models.DatabaseAccountGetResultsInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-cosmos Show documentation
Show all versions of azure-resourcemanager-cosmos Show documentation
This package contains Microsoft Azure CosmosDB SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.cosmos.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.management.SystemData;
import com.azure.resourcemanager.cosmos.models.AnalyticalStorageConfiguration;
import com.azure.resourcemanager.cosmos.models.ApiProperties;
import com.azure.resourcemanager.cosmos.models.ArmResourceProperties;
import com.azure.resourcemanager.cosmos.models.BackupPolicy;
import com.azure.resourcemanager.cosmos.models.Capability;
import com.azure.resourcemanager.cosmos.models.Capacity;
import com.azure.resourcemanager.cosmos.models.ConnectorOffer;
import com.azure.resourcemanager.cosmos.models.ConsistencyPolicy;
import com.azure.resourcemanager.cosmos.models.CorsPolicy;
import com.azure.resourcemanager.cosmos.models.CreateMode;
import com.azure.resourcemanager.cosmos.models.DatabaseAccountKeysMetadata;
import com.azure.resourcemanager.cosmos.models.DatabaseAccountKind;
import com.azure.resourcemanager.cosmos.models.DatabaseAccountOfferType;
import com.azure.resourcemanager.cosmos.models.FailoverPolicy;
import com.azure.resourcemanager.cosmos.models.IpAddressOrRange;
import com.azure.resourcemanager.cosmos.models.Location;
import com.azure.resourcemanager.cosmos.models.ManagedServiceIdentity;
import com.azure.resourcemanager.cosmos.models.MinimalTlsVersion;
import com.azure.resourcemanager.cosmos.models.NetworkAclBypass;
import com.azure.resourcemanager.cosmos.models.PublicNetworkAccess;
import com.azure.resourcemanager.cosmos.models.RestoreParameters;
import com.azure.resourcemanager.cosmos.models.VirtualNetworkRule;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
import java.util.Map;
/**
* An Azure Cosmos DB database account.
*/
@Fluent
public final class DatabaseAccountGetResultsInner extends ArmResourceProperties {
/*
* Indicates the type of database account. This can only be set at database account creation.
*/
@JsonProperty(value = "kind")
private DatabaseAccountKind kind;
/*
* Identity for the resource.
*/
@JsonProperty(value = "identity")
private ManagedServiceIdentity identity;
/*
* Properties for the database account.
*/
@JsonProperty(value = "properties")
private DatabaseAccountGetProperties innerProperties;
/*
* The system meta data relating to this resource.
*/
@JsonProperty(value = "systemData", access = JsonProperty.Access.WRITE_ONLY)
private SystemData systemData;
/**
* Creates an instance of DatabaseAccountGetResultsInner class.
*/
public DatabaseAccountGetResultsInner() {
}
/**
* Get the kind property: Indicates the type of database account. This can only be set at database account creation.
*
* @return the kind value.
*/
public DatabaseAccountKind kind() {
return this.kind;
}
/**
* Set the kind property: Indicates the type of database account. This can only be set at database account creation.
*
* @param kind the kind value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withKind(DatabaseAccountKind kind) {
this.kind = kind;
return this;
}
/**
* Get the identity property: Identity for the resource.
*
* @return the identity value.
*/
public ManagedServiceIdentity identity() {
return this.identity;
}
/**
* Set the identity property: Identity for the resource.
*
* @param identity the identity value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withIdentity(ManagedServiceIdentity identity) {
this.identity = identity;
return this;
}
/**
* Get the innerProperties property: Properties for the database account.
*
* @return the innerProperties value.
*/
private DatabaseAccountGetProperties innerProperties() {
return this.innerProperties;
}
/**
* Get the systemData property: The system meta data relating to this resource.
*
* @return the systemData value.
*/
public SystemData systemData() {
return this.systemData;
}
/**
* {@inheritDoc}
*/
@Override
public DatabaseAccountGetResultsInner withLocation(String location) {
super.withLocation(location);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public DatabaseAccountGetResultsInner withTags(Map tags) {
super.withTags(tags);
return this;
}
/**
* Get the provisioningState property: The status of the Cosmos DB account at the time the operation was called. The
* status can be one of following. 'Creating' – the Cosmos DB account is being created. When an account is in
* Creating state, only properties that are specified as input for the Create Cosmos DB account operation are
* returned. 'Succeeded' – the Cosmos DB account is active for use. 'Updating' – the Cosmos DB account is being
* updated. 'Deleting' – the Cosmos DB account is being deleted. 'Failed' – the Cosmos DB account failed creation.
* 'DeletionFailed' – the Cosmos DB account deletion failed.
*
* @return the provisioningState value.
*/
public String provisioningState() {
return this.innerProperties() == null ? null : this.innerProperties().provisioningState();
}
/**
* Get the documentEndpoint property: The connection endpoint for the Cosmos DB database account.
*
* @return the documentEndpoint value.
*/
public String documentEndpoint() {
return this.innerProperties() == null ? null : this.innerProperties().documentEndpoint();
}
/**
* Get the databaseAccountOfferType property: The offer type for the Cosmos DB database account. Default value:
* Standard.
*
* @return the databaseAccountOfferType value.
*/
public DatabaseAccountOfferType databaseAccountOfferType() {
return this.innerProperties() == null ? null : this.innerProperties().databaseAccountOfferType();
}
/**
* Get the ipRules property: List of IpRules.
*
* @return the ipRules value.
*/
public List ipRules() {
return this.innerProperties() == null ? null : this.innerProperties().ipRules();
}
/**
* Set the ipRules property: List of IpRules.
*
* @param ipRules the ipRules value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withIpRules(List ipRules) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withIpRules(ipRules);
return this;
}
/**
* Get the isVirtualNetworkFilterEnabled property: Flag to indicate whether to enable/disable Virtual Network ACL
* rules.
*
* @return the isVirtualNetworkFilterEnabled value.
*/
public Boolean isVirtualNetworkFilterEnabled() {
return this.innerProperties() == null ? null : this.innerProperties().isVirtualNetworkFilterEnabled();
}
/**
* Set the isVirtualNetworkFilterEnabled property: Flag to indicate whether to enable/disable Virtual Network ACL
* rules.
*
* @param isVirtualNetworkFilterEnabled the isVirtualNetworkFilterEnabled value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withIsVirtualNetworkFilterEnabled(Boolean isVirtualNetworkFilterEnabled) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withIsVirtualNetworkFilterEnabled(isVirtualNetworkFilterEnabled);
return this;
}
/**
* Get the enableAutomaticFailover property: Enables automatic failover of the write region in the rare event that
* the region is unavailable due to an outage. Automatic failover will result in a new write region for the account
* and is chosen based on the failover priorities configured for the account.
*
* @return the enableAutomaticFailover value.
*/
public Boolean enableAutomaticFailover() {
return this.innerProperties() == null ? null : this.innerProperties().enableAutomaticFailover();
}
/**
* Set the enableAutomaticFailover property: Enables automatic failover of the write region in the rare event that
* the region is unavailable due to an outage. Automatic failover will result in a new write region for the account
* and is chosen based on the failover priorities configured for the account.
*
* @param enableAutomaticFailover the enableAutomaticFailover value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withEnableAutomaticFailover(Boolean enableAutomaticFailover) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withEnableAutomaticFailover(enableAutomaticFailover);
return this;
}
/**
* Get the consistencyPolicy property: The consistency policy for the Cosmos DB database account.
*
* @return the consistencyPolicy value.
*/
public ConsistencyPolicy consistencyPolicy() {
return this.innerProperties() == null ? null : this.innerProperties().consistencyPolicy();
}
/**
* Set the consistencyPolicy property: The consistency policy for the Cosmos DB database account.
*
* @param consistencyPolicy the consistencyPolicy value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withConsistencyPolicy(ConsistencyPolicy consistencyPolicy) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withConsistencyPolicy(consistencyPolicy);
return this;
}
/**
* Get the capabilities property: List of Cosmos DB capabilities for the account.
*
* @return the capabilities value.
*/
public List capabilities() {
return this.innerProperties() == null ? null : this.innerProperties().capabilities();
}
/**
* Set the capabilities property: List of Cosmos DB capabilities for the account.
*
* @param capabilities the capabilities value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withCapabilities(List capabilities) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withCapabilities(capabilities);
return this;
}
/**
* Get the writeLocations property: An array that contains the write location for the Cosmos DB account.
*
* @return the writeLocations value.
*/
public List writeLocations() {
return this.innerProperties() == null ? null : this.innerProperties().writeLocations();
}
/**
* Get the readLocations property: An array that contains of the read locations enabled for the Cosmos DB account.
*
* @return the readLocations value.
*/
public List readLocations() {
return this.innerProperties() == null ? null : this.innerProperties().readLocations();
}
/**
* Get the locations property: An array that contains all of the locations enabled for the Cosmos DB account.
*
* @return the locations value.
*/
public List locations() {
return this.innerProperties() == null ? null : this.innerProperties().locations();
}
/**
* Get the failoverPolicies property: An array that contains the regions ordered by their failover priorities.
*
* @return the failoverPolicies value.
*/
public List failoverPolicies() {
return this.innerProperties() == null ? null : this.innerProperties().failoverPolicies();
}
/**
* Get the virtualNetworkRules property: List of Virtual Network ACL rules configured for the Cosmos DB account.
*
* @return the virtualNetworkRules value.
*/
public List virtualNetworkRules() {
return this.innerProperties() == null ? null : this.innerProperties().virtualNetworkRules();
}
/**
* Set the virtualNetworkRules property: List of Virtual Network ACL rules configured for the Cosmos DB account.
*
* @param virtualNetworkRules the virtualNetworkRules value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withVirtualNetworkRules(List virtualNetworkRules) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withVirtualNetworkRules(virtualNetworkRules);
return this;
}
/**
* Get the privateEndpointConnections property: List of Private Endpoint Connections configured for the Cosmos DB
* account.
*
* @return the privateEndpointConnections value.
*/
public List privateEndpointConnections() {
return this.innerProperties() == null ? null : this.innerProperties().privateEndpointConnections();
}
/**
* Get the enableMultipleWriteLocations property: Enables the account to write in multiple locations.
*
* @return the enableMultipleWriteLocations value.
*/
public Boolean enableMultipleWriteLocations() {
return this.innerProperties() == null ? null : this.innerProperties().enableMultipleWriteLocations();
}
/**
* Set the enableMultipleWriteLocations property: Enables the account to write in multiple locations.
*
* @param enableMultipleWriteLocations the enableMultipleWriteLocations value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withEnableMultipleWriteLocations(Boolean enableMultipleWriteLocations) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withEnableMultipleWriteLocations(enableMultipleWriteLocations);
return this;
}
/**
* Get the enableCassandraConnector property: Enables the cassandra connector on the Cosmos DB C* account.
*
* @return the enableCassandraConnector value.
*/
public Boolean enableCassandraConnector() {
return this.innerProperties() == null ? null : this.innerProperties().enableCassandraConnector();
}
/**
* Set the enableCassandraConnector property: Enables the cassandra connector on the Cosmos DB C* account.
*
* @param enableCassandraConnector the enableCassandraConnector value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withEnableCassandraConnector(Boolean enableCassandraConnector) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withEnableCassandraConnector(enableCassandraConnector);
return this;
}
/**
* Get the connectorOffer property: The cassandra connector offer type for the Cosmos DB database C* account.
*
* @return the connectorOffer value.
*/
public ConnectorOffer connectorOffer() {
return this.innerProperties() == null ? null : this.innerProperties().connectorOffer();
}
/**
* Set the connectorOffer property: The cassandra connector offer type for the Cosmos DB database C* account.
*
* @param connectorOffer the connectorOffer value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withConnectorOffer(ConnectorOffer connectorOffer) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withConnectorOffer(connectorOffer);
return this;
}
/**
* Get the disableKeyBasedMetadataWriteAccess property: Disable write operations on metadata resources (databases,
* containers, throughput) via account keys.
*
* @return the disableKeyBasedMetadataWriteAccess value.
*/
public Boolean disableKeyBasedMetadataWriteAccess() {
return this.innerProperties() == null ? null : this.innerProperties().disableKeyBasedMetadataWriteAccess();
}
/**
* Set the disableKeyBasedMetadataWriteAccess property: Disable write operations on metadata resources (databases,
* containers, throughput) via account keys.
*
* @param disableKeyBasedMetadataWriteAccess the disableKeyBasedMetadataWriteAccess value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner
withDisableKeyBasedMetadataWriteAccess(Boolean disableKeyBasedMetadataWriteAccess) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withDisableKeyBasedMetadataWriteAccess(disableKeyBasedMetadataWriteAccess);
return this;
}
/**
* Get the keyVaultKeyUri property: The URI of the key vault.
*
* @return the keyVaultKeyUri value.
*/
public String keyVaultKeyUri() {
return this.innerProperties() == null ? null : this.innerProperties().keyVaultKeyUri();
}
/**
* Set the keyVaultKeyUri property: The URI of the key vault.
*
* @param keyVaultKeyUri the keyVaultKeyUri value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withKeyVaultKeyUri(String keyVaultKeyUri) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withKeyVaultKeyUri(keyVaultKeyUri);
return this;
}
/**
* Get the defaultIdentity property: The default identity for accessing key vault used in features like customer
* managed keys. The default identity needs to be explicitly set by the users. It can be "FirstPartyIdentity",
* "SystemAssignedIdentity" and more.
*
* @return the defaultIdentity value.
*/
public String defaultIdentity() {
return this.innerProperties() == null ? null : this.innerProperties().defaultIdentity();
}
/**
* Set the defaultIdentity property: The default identity for accessing key vault used in features like customer
* managed keys. The default identity needs to be explicitly set by the users. It can be "FirstPartyIdentity",
* "SystemAssignedIdentity" and more.
*
* @param defaultIdentity the defaultIdentity value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withDefaultIdentity(String defaultIdentity) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withDefaultIdentity(defaultIdentity);
return this;
}
/**
* Get the publicNetworkAccess property: Whether requests from Public Network are allowed.
*
* @return the publicNetworkAccess value.
*/
public PublicNetworkAccess publicNetworkAccess() {
return this.innerProperties() == null ? null : this.innerProperties().publicNetworkAccess();
}
/**
* Set the publicNetworkAccess property: Whether requests from Public Network are allowed.
*
* @param publicNetworkAccess the publicNetworkAccess value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withPublicNetworkAccess(PublicNetworkAccess publicNetworkAccess) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withPublicNetworkAccess(publicNetworkAccess);
return this;
}
/**
* Get the enableFreeTier property: Flag to indicate whether Free Tier is enabled.
*
* @return the enableFreeTier value.
*/
public Boolean enableFreeTier() {
return this.innerProperties() == null ? null : this.innerProperties().enableFreeTier();
}
/**
* Set the enableFreeTier property: Flag to indicate whether Free Tier is enabled.
*
* @param enableFreeTier the enableFreeTier value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withEnableFreeTier(Boolean enableFreeTier) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withEnableFreeTier(enableFreeTier);
return this;
}
/**
* Get the apiProperties property: API specific properties.
*
* @return the apiProperties value.
*/
public ApiProperties apiProperties() {
return this.innerProperties() == null ? null : this.innerProperties().apiProperties();
}
/**
* Set the apiProperties property: API specific properties.
*
* @param apiProperties the apiProperties value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withApiProperties(ApiProperties apiProperties) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withApiProperties(apiProperties);
return this;
}
/**
* Get the enableAnalyticalStorage property: Flag to indicate whether to enable storage analytics.
*
* @return the enableAnalyticalStorage value.
*/
public Boolean enableAnalyticalStorage() {
return this.innerProperties() == null ? null : this.innerProperties().enableAnalyticalStorage();
}
/**
* Set the enableAnalyticalStorage property: Flag to indicate whether to enable storage analytics.
*
* @param enableAnalyticalStorage the enableAnalyticalStorage value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withEnableAnalyticalStorage(Boolean enableAnalyticalStorage) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withEnableAnalyticalStorage(enableAnalyticalStorage);
return this;
}
/**
* Get the analyticalStorageConfiguration property: Analytical storage specific properties.
*
* @return the analyticalStorageConfiguration value.
*/
public AnalyticalStorageConfiguration analyticalStorageConfiguration() {
return this.innerProperties() == null ? null : this.innerProperties().analyticalStorageConfiguration();
}
/**
* Set the analyticalStorageConfiguration property: Analytical storage specific properties.
*
* @param analyticalStorageConfiguration the analyticalStorageConfiguration value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner
withAnalyticalStorageConfiguration(AnalyticalStorageConfiguration analyticalStorageConfiguration) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withAnalyticalStorageConfiguration(analyticalStorageConfiguration);
return this;
}
/**
* Get the instanceId property: A unique identifier assigned to the database account.
*
* @return the instanceId value.
*/
public String instanceId() {
return this.innerProperties() == null ? null : this.innerProperties().instanceId();
}
/**
* Get the createMode property: Enum to indicate the mode of account creation.
*
* @return the createMode value.
*/
public CreateMode createMode() {
return this.innerProperties() == null ? null : this.innerProperties().createMode();
}
/**
* Set the createMode property: Enum to indicate the mode of account creation.
*
* @param createMode the createMode value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withCreateMode(CreateMode createMode) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withCreateMode(createMode);
return this;
}
/**
* Get the restoreParameters property: Parameters to indicate the information about the restore.
*
* @return the restoreParameters value.
*/
public RestoreParameters restoreParameters() {
return this.innerProperties() == null ? null : this.innerProperties().restoreParameters();
}
/**
* Set the restoreParameters property: Parameters to indicate the information about the restore.
*
* @param restoreParameters the restoreParameters value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withRestoreParameters(RestoreParameters restoreParameters) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withRestoreParameters(restoreParameters);
return this;
}
/**
* Get the backupPolicy property: The object representing the policy for taking backups on an account.
*
* @return the backupPolicy value.
*/
public BackupPolicy backupPolicy() {
return this.innerProperties() == null ? null : this.innerProperties().backupPolicy();
}
/**
* Set the backupPolicy property: The object representing the policy for taking backups on an account.
*
* @param backupPolicy the backupPolicy value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withBackupPolicy(BackupPolicy backupPolicy) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withBackupPolicy(backupPolicy);
return this;
}
/**
* Get the cors property: The CORS policy for the Cosmos DB database account.
*
* @return the cors value.
*/
public List cors() {
return this.innerProperties() == null ? null : this.innerProperties().cors();
}
/**
* Set the cors property: The CORS policy for the Cosmos DB database account.
*
* @param cors the cors value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withCors(List cors) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withCors(cors);
return this;
}
/**
* Get the networkAclBypass property: Indicates what services are allowed to bypass firewall checks.
*
* @return the networkAclBypass value.
*/
public NetworkAclBypass networkAclBypass() {
return this.innerProperties() == null ? null : this.innerProperties().networkAclBypass();
}
/**
* Set the networkAclBypass property: Indicates what services are allowed to bypass firewall checks.
*
* @param networkAclBypass the networkAclBypass value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withNetworkAclBypass(NetworkAclBypass networkAclBypass) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withNetworkAclBypass(networkAclBypass);
return this;
}
/**
* Get the networkAclBypassResourceIds property: An array that contains the Resource Ids for Network Acl Bypass for
* the Cosmos DB account.
*
* @return the networkAclBypassResourceIds value.
*/
public List networkAclBypassResourceIds() {
return this.innerProperties() == null ? null : this.innerProperties().networkAclBypassResourceIds();
}
/**
* Set the networkAclBypassResourceIds property: An array that contains the Resource Ids for Network Acl Bypass for
* the Cosmos DB account.
*
* @param networkAclBypassResourceIds the networkAclBypassResourceIds value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withNetworkAclBypassResourceIds(List networkAclBypassResourceIds) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withNetworkAclBypassResourceIds(networkAclBypassResourceIds);
return this;
}
/**
* Get the disableLocalAuth property: Opt-out of local authentication and ensure only MSI and AAD can be used
* exclusively for authentication.
*
* @return the disableLocalAuth value.
*/
public Boolean disableLocalAuth() {
return this.innerProperties() == null ? null : this.innerProperties().disableLocalAuth();
}
/**
* Set the disableLocalAuth property: Opt-out of local authentication and ensure only MSI and AAD can be used
* exclusively for authentication.
*
* @param disableLocalAuth the disableLocalAuth value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withDisableLocalAuth(Boolean disableLocalAuth) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withDisableLocalAuth(disableLocalAuth);
return this;
}
/**
* Get the capacity property: The object that represents all properties related to capacity enforcement on an
* account.
*
* @return the capacity value.
*/
public Capacity capacity() {
return this.innerProperties() == null ? null : this.innerProperties().capacity();
}
/**
* Set the capacity property: The object that represents all properties related to capacity enforcement on an
* account.
*
* @param capacity the capacity value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withCapacity(Capacity capacity) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withCapacity(capacity);
return this;
}
/**
* Get the keysMetadata property: The object that represents the metadata for the Account Keys of the Cosmos DB
* account.
*
* @return the keysMetadata value.
*/
public DatabaseAccountKeysMetadata keysMetadata() {
return this.innerProperties() == null ? null : this.innerProperties().keysMetadata();
}
/**
* Get the enablePartitionMerge property: Flag to indicate enabling/disabling of Partition Merge feature on the
* account.
*
* @return the enablePartitionMerge value.
*/
public Boolean enablePartitionMerge() {
return this.innerProperties() == null ? null : this.innerProperties().enablePartitionMerge();
}
/**
* Set the enablePartitionMerge property: Flag to indicate enabling/disabling of Partition Merge feature on the
* account.
*
* @param enablePartitionMerge the enablePartitionMerge value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withEnablePartitionMerge(Boolean enablePartitionMerge) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withEnablePartitionMerge(enablePartitionMerge);
return this;
}
/**
* Get the minimalTlsVersion property: Indicates the minimum allowed Tls version. The default value is Tls 1.2.
* Cassandra and Mongo APIs only work with Tls 1.2.
*
* @return the minimalTlsVersion value.
*/
public MinimalTlsVersion minimalTlsVersion() {
return this.innerProperties() == null ? null : this.innerProperties().minimalTlsVersion();
}
/**
* Set the minimalTlsVersion property: Indicates the minimum allowed Tls version. The default value is Tls 1.2.
* Cassandra and Mongo APIs only work with Tls 1.2.
*
* @param minimalTlsVersion the minimalTlsVersion value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withMinimalTlsVersion(MinimalTlsVersion minimalTlsVersion) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withMinimalTlsVersion(minimalTlsVersion);
return this;
}
/**
* Get the enableBurstCapacity property: Flag to indicate enabling/disabling of Burst Capacity Preview feature on
* the account.
*
* @return the enableBurstCapacity value.
*/
public Boolean enableBurstCapacity() {
return this.innerProperties() == null ? null : this.innerProperties().enableBurstCapacity();
}
/**
* Set the enableBurstCapacity property: Flag to indicate enabling/disabling of Burst Capacity Preview feature on
* the account.
*
* @param enableBurstCapacity the enableBurstCapacity value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withEnableBurstCapacity(Boolean enableBurstCapacity) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withEnableBurstCapacity(enableBurstCapacity);
return this;
}
/**
* Get the customerManagedKeyStatus property: Indicates the status of the Customer Managed Key feature on the
* account. In case there are errors, the property provides troubleshooting guidance.
*
* @return the customerManagedKeyStatus value.
*/
public String customerManagedKeyStatus() {
return this.innerProperties() == null ? null : this.innerProperties().customerManagedKeyStatus();
}
/**
* Set the customerManagedKeyStatus property: Indicates the status of the Customer Managed Key feature on the
* account. In case there are errors, the property provides troubleshooting guidance.
*
* @param customerManagedKeyStatus the customerManagedKeyStatus value to set.
* @return the DatabaseAccountGetResultsInner object itself.
*/
public DatabaseAccountGetResultsInner withCustomerManagedKeyStatus(String customerManagedKeyStatus) {
if (this.innerProperties() == null) {
this.innerProperties = new DatabaseAccountGetProperties();
}
this.innerProperties().withCustomerManagedKeyStatus(customerManagedKeyStatus);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (identity() != null) {
identity().validate();
}
if (innerProperties() != null) {
innerProperties().validate();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy