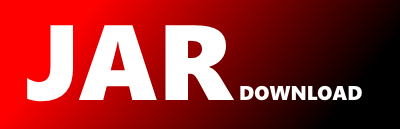
com.azure.resourcemanager.cosmos.models.RestoreParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-cosmos Show documentation
Show all versions of azure-resourcemanager-cosmos Show documentation
This package contains Microsoft Azure CosmosDB SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.cosmos.models;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.OffsetDateTime;
import java.util.List;
/**
* Parameters to indicate the information about the restore.
*/
@Fluent
public final class RestoreParameters extends RestoreParametersBase {
/*
* Describes the mode of the restore.
*/
@JsonProperty(value = "restoreMode")
private RestoreMode restoreMode;
/*
* List of specific databases available for restore.
*/
@JsonProperty(value = "databasesToRestore")
private List databasesToRestore;
/*
* List of specific gremlin databases available for restore.
*/
@JsonProperty(value = "gremlinDatabasesToRestore")
private List gremlinDatabasesToRestore;
/*
* List of specific tables available for restore.
*/
@JsonProperty(value = "tablesToRestore")
private List tablesToRestore;
/**
* Creates an instance of RestoreParameters class.
*/
public RestoreParameters() {
}
/**
* Get the restoreMode property: Describes the mode of the restore.
*
* @return the restoreMode value.
*/
public RestoreMode restoreMode() {
return this.restoreMode;
}
/**
* Set the restoreMode property: Describes the mode of the restore.
*
* @param restoreMode the restoreMode value to set.
* @return the RestoreParameters object itself.
*/
public RestoreParameters withRestoreMode(RestoreMode restoreMode) {
this.restoreMode = restoreMode;
return this;
}
/**
* Get the databasesToRestore property: List of specific databases available for restore.
*
* @return the databasesToRestore value.
*/
public List databasesToRestore() {
return this.databasesToRestore;
}
/**
* Set the databasesToRestore property: List of specific databases available for restore.
*
* @param databasesToRestore the databasesToRestore value to set.
* @return the RestoreParameters object itself.
*/
public RestoreParameters withDatabasesToRestore(List databasesToRestore) {
this.databasesToRestore = databasesToRestore;
return this;
}
/**
* Get the gremlinDatabasesToRestore property: List of specific gremlin databases available for restore.
*
* @return the gremlinDatabasesToRestore value.
*/
public List gremlinDatabasesToRestore() {
return this.gremlinDatabasesToRestore;
}
/**
* Set the gremlinDatabasesToRestore property: List of specific gremlin databases available for restore.
*
* @param gremlinDatabasesToRestore the gremlinDatabasesToRestore value to set.
* @return the RestoreParameters object itself.
*/
public RestoreParameters
withGremlinDatabasesToRestore(List gremlinDatabasesToRestore) {
this.gremlinDatabasesToRestore = gremlinDatabasesToRestore;
return this;
}
/**
* Get the tablesToRestore property: List of specific tables available for restore.
*
* @return the tablesToRestore value.
*/
public List tablesToRestore() {
return this.tablesToRestore;
}
/**
* Set the tablesToRestore property: List of specific tables available for restore.
*
* @param tablesToRestore the tablesToRestore value to set.
* @return the RestoreParameters object itself.
*/
public RestoreParameters withTablesToRestore(List tablesToRestore) {
this.tablesToRestore = tablesToRestore;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public RestoreParameters withRestoreSource(String restoreSource) {
super.withRestoreSource(restoreSource);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public RestoreParameters withRestoreTimestampInUtc(OffsetDateTime restoreTimestampInUtc) {
super.withRestoreTimestampInUtc(restoreTimestampInUtc);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
@Override
public void validate() {
super.validate();
if (databasesToRestore() != null) {
databasesToRestore().forEach(e -> e.validate());
}
if (gremlinDatabasesToRestore() != null) {
gremlinDatabasesToRestore().forEach(e -> e.validate());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy