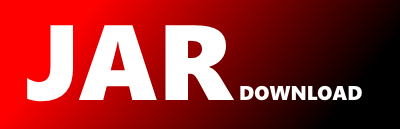
com.azure.resourcemanager.cosmos.fluent.CassandraResourcesClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-cosmos Show documentation
Show all versions of azure-resourcemanager-cosmos Show documentation
This package contains Microsoft Azure CosmosDB SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.cosmos.fluent;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.polling.PollerFlux;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.cosmos.fluent.models.CassandraKeyspaceGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.CassandraTableGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.ThroughputSettingsGetResultsInner;
import com.azure.resourcemanager.cosmos.models.CassandraKeyspaceCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.CassandraTableCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.ThroughputSettingsUpdateParameters;
import java.nio.ByteBuffer;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* An instance of this class provides access to all the operations defined in CassandraResourcesClient.
*/
public interface CassandraResourcesClient {
/**
* Lists the Cassandra keyspaces under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the Cassandra keyspaces and their properties as paginated
* response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listCassandraKeyspacesAsync(String resourceGroupName,
String accountName);
/**
* Lists the Cassandra keyspaces under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the Cassandra keyspaces and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listCassandraKeyspaces(String resourceGroupName,
String accountName);
/**
* Lists the Cassandra keyspaces under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the Cassandra keyspaces and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listCassandraKeyspaces(String resourceGroupName, String accountName,
Context context);
/**
* Gets the Cassandra keyspaces under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Cassandra keyspaces under an existing Azure Cosmos DB database account with the provided name along
* with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getCassandraKeyspaceWithResponseAsync(String resourceGroupName,
String accountName, String keyspaceName);
/**
* Gets the Cassandra keyspaces under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Cassandra keyspaces under an existing Azure Cosmos DB database account with the provided name on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getCassandraKeyspaceAsync(String resourceGroupName, String accountName,
String keyspaceName);
/**
* Gets the Cassandra keyspaces under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Cassandra keyspaces under an existing Azure Cosmos DB database account with the provided name along
* with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getCassandraKeyspaceWithResponse(String resourceGroupName,
String accountName, String keyspaceName, Context context);
/**
* Gets the Cassandra keyspaces under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Cassandra keyspaces under an existing Azure Cosmos DB database account with the provided name.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
CassandraKeyspaceGetResultsInner getCassandraKeyspace(String resourceGroupName, String accountName,
String keyspaceName);
/**
* Create or update an Azure Cosmos DB Cassandra keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param createUpdateCassandraKeyspaceParameters The parameters to provide for the current Cassandra keyspace.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Cassandra keyspace along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createUpdateCassandraKeyspaceWithResponseAsync(String resourceGroupName,
String accountName, String keyspaceName,
CassandraKeyspaceCreateUpdateParameters createUpdateCassandraKeyspaceParameters);
/**
* Create or update an Azure Cosmos DB Cassandra keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param createUpdateCassandraKeyspaceParameters The parameters to provide for the current Cassandra keyspace.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB Cassandra keyspace.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, CassandraKeyspaceGetResultsInner>
beginCreateUpdateCassandraKeyspaceAsync(String resourceGroupName, String accountName, String keyspaceName,
CassandraKeyspaceCreateUpdateParameters createUpdateCassandraKeyspaceParameters);
/**
* Create or update an Azure Cosmos DB Cassandra keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param createUpdateCassandraKeyspaceParameters The parameters to provide for the current Cassandra keyspace.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB Cassandra keyspace.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, CassandraKeyspaceGetResultsInner>
beginCreateUpdateCassandraKeyspace(String resourceGroupName, String accountName, String keyspaceName,
CassandraKeyspaceCreateUpdateParameters createUpdateCassandraKeyspaceParameters);
/**
* Create or update an Azure Cosmos DB Cassandra keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param createUpdateCassandraKeyspaceParameters The parameters to provide for the current Cassandra keyspace.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB Cassandra keyspace.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, CassandraKeyspaceGetResultsInner>
beginCreateUpdateCassandraKeyspace(String resourceGroupName, String accountName, String keyspaceName,
CassandraKeyspaceCreateUpdateParameters createUpdateCassandraKeyspaceParameters, Context context);
/**
* Create or update an Azure Cosmos DB Cassandra keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param createUpdateCassandraKeyspaceParameters The parameters to provide for the current Cassandra keyspace.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Cassandra keyspace on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createUpdateCassandraKeyspaceAsync(String resourceGroupName,
String accountName, String keyspaceName,
CassandraKeyspaceCreateUpdateParameters createUpdateCassandraKeyspaceParameters);
/**
* Create or update an Azure Cosmos DB Cassandra keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param createUpdateCassandraKeyspaceParameters The parameters to provide for the current Cassandra keyspace.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Cassandra keyspace.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
CassandraKeyspaceGetResultsInner createUpdateCassandraKeyspace(String resourceGroupName, String accountName,
String keyspaceName, CassandraKeyspaceCreateUpdateParameters createUpdateCassandraKeyspaceParameters);
/**
* Create or update an Azure Cosmos DB Cassandra keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param createUpdateCassandraKeyspaceParameters The parameters to provide for the current Cassandra keyspace.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Cassandra keyspace.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
CassandraKeyspaceGetResultsInner createUpdateCassandraKeyspace(String resourceGroupName, String accountName,
String keyspaceName, CassandraKeyspaceCreateUpdateParameters createUpdateCassandraKeyspaceParameters,
Context context);
/**
* Deletes an existing Azure Cosmos DB Cassandra keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deleteCassandraKeyspaceWithResponseAsync(String resourceGroupName,
String accountName, String keyspaceName);
/**
* Deletes an existing Azure Cosmos DB Cassandra keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteCassandraKeyspaceAsync(String resourceGroupName, String accountName,
String keyspaceName);
/**
* Deletes an existing Azure Cosmos DB Cassandra keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteCassandraKeyspace(String resourceGroupName, String accountName,
String keyspaceName);
/**
* Deletes an existing Azure Cosmos DB Cassandra keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteCassandraKeyspace(String resourceGroupName, String accountName,
String keyspaceName, Context context);
/**
* Deletes an existing Azure Cosmos DB Cassandra keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteCassandraKeyspaceAsync(String resourceGroupName, String accountName, String keyspaceName);
/**
* Deletes an existing Azure Cosmos DB Cassandra keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteCassandraKeyspace(String resourceGroupName, String accountName, String keyspaceName);
/**
* Deletes an existing Azure Cosmos DB Cassandra keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteCassandraKeyspace(String resourceGroupName, String accountName, String keyspaceName, Context context);
/**
* Gets the RUs per second of the Cassandra Keyspace under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the Cassandra Keyspace under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getCassandraKeyspaceThroughputWithResponseAsync(
String resourceGroupName, String accountName, String keyspaceName);
/**
* Gets the RUs per second of the Cassandra Keyspace under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the Cassandra Keyspace under an existing Azure Cosmos DB database account with the
* provided name on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getCassandraKeyspaceThroughputAsync(String resourceGroupName,
String accountName, String keyspaceName);
/**
* Gets the RUs per second of the Cassandra Keyspace under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the Cassandra Keyspace under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getCassandraKeyspaceThroughputWithResponse(String resourceGroupName,
String accountName, String keyspaceName, Context context);
/**
* Gets the RUs per second of the Cassandra Keyspace under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the Cassandra Keyspace under an existing Azure Cosmos DB database account with the
* provided name.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner getCassandraKeyspaceThroughput(String resourceGroupName, String accountName,
String keyspaceName);
/**
* Update RUs per second of an Azure Cosmos DB Cassandra Keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current Cassandra
* Keyspace.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> updateCassandraKeyspaceThroughputWithResponseAsync(String resourceGroupName,
String accountName, String keyspaceName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB Cassandra Keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current Cassandra
* Keyspace.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginUpdateCassandraKeyspaceThroughputAsync(String resourceGroupName, String accountName, String keyspaceName,
ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB Cassandra Keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current Cassandra
* Keyspace.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateCassandraKeyspaceThroughput(String resourceGroupName, String accountName, String keyspaceName,
ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB Cassandra Keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current Cassandra
* Keyspace.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateCassandraKeyspaceThroughput(String resourceGroupName, String accountName, String keyspaceName,
ThroughputSettingsUpdateParameters updateThroughputParameters, Context context);
/**
* Update RUs per second of an Azure Cosmos DB Cassandra Keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current Cassandra
* Keyspace.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono updateCassandraKeyspaceThroughputAsync(String resourceGroupName,
String accountName, String keyspaceName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB Cassandra Keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current Cassandra
* Keyspace.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner updateCassandraKeyspaceThroughput(String resourceGroupName, String accountName,
String keyspaceName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB Cassandra Keyspace.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current Cassandra
* Keyspace.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner updateCassandraKeyspaceThroughput(String resourceGroupName, String accountName,
String keyspaceName, ThroughputSettingsUpdateParameters updateThroughputParameters, Context context);
/**
* Migrate an Azure Cosmos DB Cassandra Keyspace from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> migrateCassandraKeyspaceToAutoscaleWithResponseAsync(String resourceGroupName,
String accountName, String keyspaceName);
/**
* Migrate an Azure Cosmos DB Cassandra Keyspace from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateCassandraKeyspaceToAutoscaleAsync(String resourceGroupName, String accountName,
String keyspaceName);
/**
* Migrate an Azure Cosmos DB Cassandra Keyspace from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateCassandraKeyspaceToAutoscale(String resourceGroupName, String accountName, String keyspaceName);
/**
* Migrate an Azure Cosmos DB Cassandra Keyspace from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateCassandraKeyspaceToAutoscale(String resourceGroupName, String accountName, String keyspaceName,
Context context);
/**
* Migrate an Azure Cosmos DB Cassandra Keyspace from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono migrateCassandraKeyspaceToAutoscaleAsync(String resourceGroupName,
String accountName, String keyspaceName);
/**
* Migrate an Azure Cosmos DB Cassandra Keyspace from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateCassandraKeyspaceToAutoscale(String resourceGroupName, String accountName,
String keyspaceName);
/**
* Migrate an Azure Cosmos DB Cassandra Keyspace from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateCassandraKeyspaceToAutoscale(String resourceGroupName, String accountName,
String keyspaceName, Context context);
/**
* Migrate an Azure Cosmos DB Cassandra Keyspace from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> migrateCassandraKeyspaceToManualThroughputWithResponseAsync(
String resourceGroupName, String accountName, String keyspaceName);
/**
* Migrate an Azure Cosmos DB Cassandra Keyspace from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateCassandraKeyspaceToManualThroughputAsync(String resourceGroupName, String accountName,
String keyspaceName);
/**
* Migrate an Azure Cosmos DB Cassandra Keyspace from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateCassandraKeyspaceToManualThroughput(String resourceGroupName, String accountName,
String keyspaceName);
/**
* Migrate an Azure Cosmos DB Cassandra Keyspace from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateCassandraKeyspaceToManualThroughput(String resourceGroupName, String accountName,
String keyspaceName, Context context);
/**
* Migrate an Azure Cosmos DB Cassandra Keyspace from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono migrateCassandraKeyspaceToManualThroughputAsync(String resourceGroupName,
String accountName, String keyspaceName);
/**
* Migrate an Azure Cosmos DB Cassandra Keyspace from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateCassandraKeyspaceToManualThroughput(String resourceGroupName,
String accountName, String keyspaceName);
/**
* Migrate an Azure Cosmos DB Cassandra Keyspace from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateCassandraKeyspaceToManualThroughput(String resourceGroupName,
String accountName, String keyspaceName, Context context);
/**
* Lists the Cassandra table under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the Cassandra tables and their properties as paginated
* response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listCassandraTablesAsync(String resourceGroupName, String accountName,
String keyspaceName);
/**
* Lists the Cassandra table under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the Cassandra tables and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listCassandraTables(String resourceGroupName, String accountName,
String keyspaceName);
/**
* Lists the Cassandra table under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the Cassandra tables and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listCassandraTables(String resourceGroupName, String accountName,
String keyspaceName, Context context);
/**
* Gets the Cassandra table under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Cassandra table under an existing Azure Cosmos DB database account along with {@link Response} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getCassandraTableWithResponseAsync(String resourceGroupName,
String accountName, String keyspaceName, String tableName);
/**
* Gets the Cassandra table under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Cassandra table under an existing Azure Cosmos DB database account on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getCassandraTableAsync(String resourceGroupName, String accountName,
String keyspaceName, String tableName);
/**
* Gets the Cassandra table under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Cassandra table under an existing Azure Cosmos DB database account along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getCassandraTableWithResponse(String resourceGroupName, String accountName,
String keyspaceName, String tableName, Context context);
/**
* Gets the Cassandra table under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the Cassandra table under an existing Azure Cosmos DB database account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
CassandraTableGetResultsInner getCassandraTable(String resourceGroupName, String accountName, String keyspaceName,
String tableName);
/**
* Create or update an Azure Cosmos DB Cassandra Table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param createUpdateCassandraTableParameters The parameters to provide for the current Cassandra Table.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Cassandra table along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createUpdateCassandraTableWithResponseAsync(String resourceGroupName,
String accountName, String keyspaceName, String tableName,
CassandraTableCreateUpdateParameters createUpdateCassandraTableParameters);
/**
* Create or update an Azure Cosmos DB Cassandra Table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param createUpdateCassandraTableParameters The parameters to provide for the current Cassandra Table.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB Cassandra table.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, CassandraTableGetResultsInner>
beginCreateUpdateCassandraTableAsync(String resourceGroupName, String accountName, String keyspaceName,
String tableName, CassandraTableCreateUpdateParameters createUpdateCassandraTableParameters);
/**
* Create or update an Azure Cosmos DB Cassandra Table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param createUpdateCassandraTableParameters The parameters to provide for the current Cassandra Table.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB Cassandra table.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, CassandraTableGetResultsInner>
beginCreateUpdateCassandraTable(String resourceGroupName, String accountName, String keyspaceName,
String tableName, CassandraTableCreateUpdateParameters createUpdateCassandraTableParameters);
/**
* Create or update an Azure Cosmos DB Cassandra Table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param createUpdateCassandraTableParameters The parameters to provide for the current Cassandra Table.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB Cassandra table.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, CassandraTableGetResultsInner>
beginCreateUpdateCassandraTable(String resourceGroupName, String accountName, String keyspaceName,
String tableName, CassandraTableCreateUpdateParameters createUpdateCassandraTableParameters,
Context context);
/**
* Create or update an Azure Cosmos DB Cassandra Table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param createUpdateCassandraTableParameters The parameters to provide for the current Cassandra Table.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Cassandra table on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createUpdateCassandraTableAsync(String resourceGroupName, String accountName,
String keyspaceName, String tableName,
CassandraTableCreateUpdateParameters createUpdateCassandraTableParameters);
/**
* Create or update an Azure Cosmos DB Cassandra Table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param createUpdateCassandraTableParameters The parameters to provide for the current Cassandra Table.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Cassandra table.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
CassandraTableGetResultsInner createUpdateCassandraTable(String resourceGroupName, String accountName,
String keyspaceName, String tableName,
CassandraTableCreateUpdateParameters createUpdateCassandraTableParameters);
/**
* Create or update an Azure Cosmos DB Cassandra Table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param createUpdateCassandraTableParameters The parameters to provide for the current Cassandra Table.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Cassandra table.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
CassandraTableGetResultsInner createUpdateCassandraTable(String resourceGroupName, String accountName,
String keyspaceName, String tableName,
CassandraTableCreateUpdateParameters createUpdateCassandraTableParameters, Context context);
/**
* Deletes an existing Azure Cosmos DB Cassandra table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deleteCassandraTableWithResponseAsync(String resourceGroupName, String accountName,
String keyspaceName, String tableName);
/**
* Deletes an existing Azure Cosmos DB Cassandra table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteCassandraTableAsync(String resourceGroupName, String accountName,
String keyspaceName, String tableName);
/**
* Deletes an existing Azure Cosmos DB Cassandra table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteCassandraTable(String resourceGroupName, String accountName,
String keyspaceName, String tableName);
/**
* Deletes an existing Azure Cosmos DB Cassandra table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteCassandraTable(String resourceGroupName, String accountName,
String keyspaceName, String tableName, Context context);
/**
* Deletes an existing Azure Cosmos DB Cassandra table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteCassandraTableAsync(String resourceGroupName, String accountName, String keyspaceName,
String tableName);
/**
* Deletes an existing Azure Cosmos DB Cassandra table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteCassandraTable(String resourceGroupName, String accountName, String keyspaceName, String tableName);
/**
* Deletes an existing Azure Cosmos DB Cassandra table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteCassandraTable(String resourceGroupName, String accountName, String keyspaceName, String tableName,
Context context);
/**
* Gets the RUs per second of the Cassandra table under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the Cassandra table under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getCassandraTableThroughputWithResponseAsync(
String resourceGroupName, String accountName, String keyspaceName, String tableName);
/**
* Gets the RUs per second of the Cassandra table under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the Cassandra table under an existing Azure Cosmos DB database account with the
* provided name on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getCassandraTableThroughputAsync(String resourceGroupName,
String accountName, String keyspaceName, String tableName);
/**
* Gets the RUs per second of the Cassandra table under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the Cassandra table under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getCassandraTableThroughputWithResponse(String resourceGroupName,
String accountName, String keyspaceName, String tableName, Context context);
/**
* Gets the RUs per second of the Cassandra table under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the Cassandra table under an existing Azure Cosmos DB database account with the
* provided name.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner getCassandraTableThroughput(String resourceGroupName, String accountName,
String keyspaceName, String tableName);
/**
* Update RUs per second of an Azure Cosmos DB Cassandra table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current Cassandra
* table.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> updateCassandraTableThroughputWithResponseAsync(String resourceGroupName,
String accountName, String keyspaceName, String tableName,
ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB Cassandra table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current Cassandra
* table.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginUpdateCassandraTableThroughputAsync(String resourceGroupName, String accountName, String keyspaceName,
String tableName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB Cassandra table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current Cassandra
* table.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateCassandraTableThroughput(String resourceGroupName, String accountName, String keyspaceName,
String tableName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB Cassandra table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current Cassandra
* table.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateCassandraTableThroughput(String resourceGroupName, String accountName, String keyspaceName,
String tableName, ThroughputSettingsUpdateParameters updateThroughputParameters, Context context);
/**
* Update RUs per second of an Azure Cosmos DB Cassandra table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current Cassandra
* table.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono updateCassandraTableThroughputAsync(String resourceGroupName,
String accountName, String keyspaceName, String tableName,
ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB Cassandra table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current Cassandra
* table.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner updateCassandraTableThroughput(String resourceGroupName, String accountName,
String keyspaceName, String tableName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB Cassandra table.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current Cassandra
* table.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner updateCassandraTableThroughput(String resourceGroupName, String accountName,
String keyspaceName, String tableName, ThroughputSettingsUpdateParameters updateThroughputParameters,
Context context);
/**
* Migrate an Azure Cosmos DB Cassandra table from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> migrateCassandraTableToAutoscaleWithResponseAsync(String resourceGroupName,
String accountName, String keyspaceName, String tableName);
/**
* Migrate an Azure Cosmos DB Cassandra table from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateCassandraTableToAutoscaleAsync(String resourceGroupName, String accountName, String keyspaceName,
String tableName);
/**
* Migrate an Azure Cosmos DB Cassandra table from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateCassandraTableToAutoscale(String resourceGroupName, String accountName, String keyspaceName,
String tableName);
/**
* Migrate an Azure Cosmos DB Cassandra table from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateCassandraTableToAutoscale(String resourceGroupName, String accountName, String keyspaceName,
String tableName, Context context);
/**
* Migrate an Azure Cosmos DB Cassandra table from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono migrateCassandraTableToAutoscaleAsync(String resourceGroupName,
String accountName, String keyspaceName, String tableName);
/**
* Migrate an Azure Cosmos DB Cassandra table from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateCassandraTableToAutoscale(String resourceGroupName, String accountName,
String keyspaceName, String tableName);
/**
* Migrate an Azure Cosmos DB Cassandra table from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateCassandraTableToAutoscale(String resourceGroupName, String accountName,
String keyspaceName, String tableName, Context context);
/**
* Migrate an Azure Cosmos DB Cassandra table from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> migrateCassandraTableToManualThroughputWithResponseAsync(String resourceGroupName,
String accountName, String keyspaceName, String tableName);
/**
* Migrate an Azure Cosmos DB Cassandra table from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateCassandraTableToManualThroughputAsync(String resourceGroupName, String accountName,
String keyspaceName, String tableName);
/**
* Migrate an Azure Cosmos DB Cassandra table from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateCassandraTableToManualThroughput(String resourceGroupName, String accountName, String keyspaceName,
String tableName);
/**
* Migrate an Azure Cosmos DB Cassandra table from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateCassandraTableToManualThroughput(String resourceGroupName, String accountName, String keyspaceName,
String tableName, Context context);
/**
* Migrate an Azure Cosmos DB Cassandra table from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono migrateCassandraTableToManualThroughputAsync(String resourceGroupName,
String accountName, String keyspaceName, String tableName);
/**
* Migrate an Azure Cosmos DB Cassandra table from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateCassandraTableToManualThroughput(String resourceGroupName,
String accountName, String keyspaceName, String tableName);
/**
* Migrate an Azure Cosmos DB Cassandra table from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param keyspaceName Cosmos DB keyspace name.
* @param tableName Cosmos DB table name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateCassandraTableToManualThroughput(String resourceGroupName,
String accountName, String keyspaceName, String tableName, Context context);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy