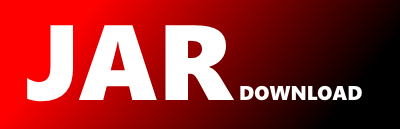
com.azure.resourcemanager.cosmos.fluent.SqlResourcesClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-cosmos Show documentation
Show all versions of azure-resourcemanager-cosmos Show documentation
This package contains Microsoft Azure CosmosDB SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.cosmos.fluent;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.polling.PollerFlux;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.cosmos.fluent.models.BackupInformationInner;
import com.azure.resourcemanager.cosmos.fluent.models.ClientEncryptionKeyGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.SqlContainerGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.SqlDatabaseGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.SqlRoleAssignmentGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.SqlRoleDefinitionGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.SqlStoredProcedureGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.SqlTriggerGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.SqlUserDefinedFunctionGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.ThroughputSettingsGetResultsInner;
import com.azure.resourcemanager.cosmos.models.ClientEncryptionKeyCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.ContinuousBackupRestoreLocation;
import com.azure.resourcemanager.cosmos.models.SqlContainerCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.SqlDatabaseCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.SqlRoleAssignmentCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.SqlRoleDefinitionCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.SqlStoredProcedureCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.SqlTriggerCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.SqlUserDefinedFunctionCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.ThroughputSettingsUpdateParameters;
import java.nio.ByteBuffer;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* An instance of this class provides access to all the operations defined in SqlResourcesClient.
*/
public interface SqlResourcesClient {
/**
* Lists the SQL databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the SQL databases and their properties as paginated response
* with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listSqlDatabasesAsync(String resourceGroupName, String accountName);
/**
* Lists the SQL databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the SQL databases and their properties as paginated response
* with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSqlDatabases(String resourceGroupName, String accountName);
/**
* Lists the SQL databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the SQL databases and their properties as paginated response
* with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSqlDatabases(String resourceGroupName, String accountName,
Context context);
/**
* Gets the SQL database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL database under an existing Azure Cosmos DB database account with the provided name along with
* {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getSqlDatabaseWithResponseAsync(String resourceGroupName,
String accountName, String databaseName);
/**
* Gets the SQL database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL database under an existing Azure Cosmos DB database account with the provided name on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getSqlDatabaseAsync(String resourceGroupName, String accountName,
String databaseName);
/**
* Gets the SQL database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL database under an existing Azure Cosmos DB database account with the provided name along with
* {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getSqlDatabaseWithResponse(String resourceGroupName, String accountName,
String databaseName, Context context);
/**
* Gets the SQL database under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL database under an existing Azure Cosmos DB database account with the provided name.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlDatabaseGetResultsInner getSqlDatabase(String resourceGroupName, String accountName, String databaseName);
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB SQL database along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createUpdateSqlDatabaseWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters);
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB SQL database.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, SqlDatabaseGetResultsInner> beginCreateUpdateSqlDatabaseAsync(
String resourceGroupName, String accountName, String databaseName,
SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters);
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB SQL database.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SqlDatabaseGetResultsInner> beginCreateUpdateSqlDatabase(
String resourceGroupName, String accountName, String databaseName,
SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters);
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB SQL database.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SqlDatabaseGetResultsInner> beginCreateUpdateSqlDatabase(
String resourceGroupName, String accountName, String databaseName,
SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters, Context context);
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB SQL database on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createUpdateSqlDatabaseAsync(String resourceGroupName, String accountName,
String databaseName, SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters);
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB SQL database.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlDatabaseGetResultsInner createUpdateSqlDatabase(String resourceGroupName, String accountName,
String databaseName, SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters);
/**
* Create or update an Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateSqlDatabaseParameters The parameters to provide for the current SQL database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB SQL database.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlDatabaseGetResultsInner createUpdateSqlDatabase(String resourceGroupName, String accountName,
String databaseName, SqlDatabaseCreateUpdateParameters createUpdateSqlDatabaseParameters, Context context);
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deleteSqlDatabaseWithResponseAsync(String resourceGroupName, String accountName,
String databaseName);
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteSqlDatabaseAsync(String resourceGroupName, String accountName,
String databaseName);
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSqlDatabase(String resourceGroupName, String accountName,
String databaseName);
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSqlDatabase(String resourceGroupName, String accountName,
String databaseName, Context context);
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteSqlDatabaseAsync(String resourceGroupName, String accountName, String databaseName);
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSqlDatabase(String resourceGroupName, String accountName, String databaseName);
/**
* Deletes an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSqlDatabase(String resourceGroupName, String accountName, String databaseName, Context context);
/**
* Gets the RUs per second of the SQL database under an existing Azure Cosmos DB database account with the provided
* name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the SQL database under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>
getSqlDatabaseThroughputWithResponseAsync(String resourceGroupName, String accountName, String databaseName);
/**
* Gets the RUs per second of the SQL database under an existing Azure Cosmos DB database account with the provided
* name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the SQL database under an existing Azure Cosmos DB database account with the
* provided name on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getSqlDatabaseThroughputAsync(String resourceGroupName, String accountName,
String databaseName);
/**
* Gets the RUs per second of the SQL database under an existing Azure Cosmos DB database account with the provided
* name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the SQL database under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getSqlDatabaseThroughputWithResponse(String resourceGroupName,
String accountName, String databaseName, Context context);
/**
* Gets the RUs per second of the SQL database under an existing Azure Cosmos DB database account with the provided
* name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the SQL database under an existing Azure Cosmos DB database account with the
* provided name.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner getSqlDatabaseThroughput(String resourceGroupName, String accountName,
String databaseName);
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> updateSqlDatabaseThroughputWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginUpdateSqlDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName,
ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateSqlDatabaseThroughput(String resourceGroupName, String accountName, String databaseName,
ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateSqlDatabaseThroughput(String resourceGroupName, String accountName, String databaseName,
ThroughputSettingsUpdateParameters updateThroughputParameters, Context context);
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono updateSqlDatabaseThroughputAsync(String resourceGroupName,
String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner updateSqlDatabaseThroughput(String resourceGroupName, String accountName,
String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner updateSqlDatabaseThroughput(String resourceGroupName, String accountName,
String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters, Context context);
/**
* Migrate an Azure Cosmos DB SQL database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> migrateSqlDatabaseToAutoscaleWithResponseAsync(String resourceGroupName,
String accountName, String databaseName);
/**
* Migrate an Azure Cosmos DB SQL database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateSqlDatabaseToAutoscaleAsync(String resourceGroupName, String accountName, String databaseName);
/**
* Migrate an Azure Cosmos DB SQL database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateSqlDatabaseToAutoscale(String resourceGroupName, String accountName, String databaseName);
/**
* Migrate an Azure Cosmos DB SQL database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateSqlDatabaseToAutoscale(String resourceGroupName, String accountName, String databaseName,
Context context);
/**
* Migrate an Azure Cosmos DB SQL database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono migrateSqlDatabaseToAutoscaleAsync(String resourceGroupName,
String accountName, String databaseName);
/**
* Migrate an Azure Cosmos DB SQL database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateSqlDatabaseToAutoscale(String resourceGroupName, String accountName,
String databaseName);
/**
* Migrate an Azure Cosmos DB SQL database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateSqlDatabaseToAutoscale(String resourceGroupName, String accountName,
String databaseName, Context context);
/**
* Migrate an Azure Cosmos DB SQL database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> migrateSqlDatabaseToManualThroughputWithResponseAsync(String resourceGroupName,
String accountName, String databaseName);
/**
* Migrate an Azure Cosmos DB SQL database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateSqlDatabaseToManualThroughputAsync(String resourceGroupName, String accountName,
String databaseName);
/**
* Migrate an Azure Cosmos DB SQL database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateSqlDatabaseToManualThroughput(String resourceGroupName, String accountName, String databaseName);
/**
* Migrate an Azure Cosmos DB SQL database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateSqlDatabaseToManualThroughput(String resourceGroupName, String accountName, String databaseName,
Context context);
/**
* Migrate an Azure Cosmos DB SQL database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono migrateSqlDatabaseToManualThroughputAsync(String resourceGroupName,
String accountName, String databaseName);
/**
* Migrate an Azure Cosmos DB SQL database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateSqlDatabaseToManualThroughput(String resourceGroupName, String accountName,
String databaseName);
/**
* Migrate an Azure Cosmos DB SQL database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateSqlDatabaseToManualThroughput(String resourceGroupName, String accountName,
String databaseName, Context context);
/**
* Lists the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the containers and their properties as paginated response with
* {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listSqlContainersAsync(String resourceGroupName, String accountName,
String databaseName);
/**
* Lists the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the containers and their properties as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSqlContainers(String resourceGroupName, String accountName,
String databaseName);
/**
* Lists the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the containers and their properties as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSqlContainers(String resourceGroupName, String accountName,
String databaseName, Context context);
/**
* Gets the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL container under an existing Azure Cosmos DB database account along with {@link Response} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getSqlContainerWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String containerName);
/**
* Gets the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL container under an existing Azure Cosmos DB database account on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getSqlContainerAsync(String resourceGroupName, String accountName,
String databaseName, String containerName);
/**
* Gets the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL container under an existing Azure Cosmos DB database account along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getSqlContainerWithResponse(String resourceGroupName, String accountName,
String databaseName, String containerName, Context context);
/**
* Gets the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL container under an existing Azure Cosmos DB database account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlContainerGetResultsInner getSqlContainer(String resourceGroupName, String accountName, String databaseName,
String containerName);
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB container along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createUpdateSqlContainerWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String containerName,
SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters);
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB container.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, SqlContainerGetResultsInner> beginCreateUpdateSqlContainerAsync(
String resourceGroupName, String accountName, String databaseName, String containerName,
SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters);
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB container.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SqlContainerGetResultsInner> beginCreateUpdateSqlContainer(
String resourceGroupName, String accountName, String databaseName, String containerName,
SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters);
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB container.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SqlContainerGetResultsInner> beginCreateUpdateSqlContainer(
String resourceGroupName, String accountName, String databaseName, String containerName,
SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters, Context context);
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB container on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createUpdateSqlContainerAsync(String resourceGroupName, String accountName,
String databaseName, String containerName,
SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters);
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB container.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlContainerGetResultsInner createUpdateSqlContainer(String resourceGroupName, String accountName,
String databaseName, String containerName,
SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters);
/**
* Create or update an Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param createUpdateSqlContainerParameters The parameters to provide for the current SQL container.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB container.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlContainerGetResultsInner createUpdateSqlContainer(String resourceGroupName, String accountName,
String databaseName, String containerName,
SqlContainerCreateUpdateParameters createUpdateSqlContainerParameters, Context context);
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deleteSqlContainerWithResponseAsync(String resourceGroupName, String accountName,
String databaseName, String containerName);
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteSqlContainerAsync(String resourceGroupName, String accountName,
String databaseName, String containerName);
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSqlContainer(String resourceGroupName, String accountName,
String databaseName, String containerName);
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSqlContainer(String resourceGroupName, String accountName,
String databaseName, String containerName, Context context);
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteSqlContainerAsync(String resourceGroupName, String accountName, String databaseName,
String containerName);
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSqlContainer(String resourceGroupName, String accountName, String databaseName, String containerName);
/**
* Deletes an existing Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSqlContainer(String resourceGroupName, String accountName, String databaseName, String containerName,
Context context);
/**
* Gets the RUs per second of the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the SQL container under an existing Azure Cosmos DB database account along with
* {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getSqlContainerThroughputWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String containerName);
/**
* Gets the RUs per second of the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the SQL container under an existing Azure Cosmos DB database account on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getSqlContainerThroughputAsync(String resourceGroupName, String accountName,
String databaseName, String containerName);
/**
* Gets the RUs per second of the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the SQL container under an existing Azure Cosmos DB database account along with
* {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getSqlContainerThroughputWithResponse(String resourceGroupName,
String accountName, String databaseName, String containerName, Context context);
/**
* Gets the RUs per second of the SQL container under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the SQL container under an existing Azure Cosmos DB database account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner getSqlContainerThroughput(String resourceGroupName, String accountName,
String databaseName, String containerName);
/**
* Update RUs per second of an Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> updateSqlContainerThroughputWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String containerName,
ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginUpdateSqlContainerThroughputAsync(String resourceGroupName, String accountName, String databaseName,
String containerName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateSqlContainerThroughput(String resourceGroupName, String accountName, String databaseName,
String containerName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL container.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateSqlContainerThroughput(String resourceGroupName, String accountName, String databaseName,
String containerName, ThroughputSettingsUpdateParameters updateThroughputParameters, Context context);
/**
* Update RUs per second of an Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono updateSqlContainerThroughputAsync(String resourceGroupName,
String accountName, String databaseName, String containerName,
ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner updateSqlContainerThroughput(String resourceGroupName, String accountName,
String databaseName, String containerName, ThroughputSettingsUpdateParameters updateThroughputParameters);
/**
* Update RUs per second of an Azure Cosmos DB SQL container.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param updateThroughputParameters The parameters to provide for the RUs per second of the current SQL container.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner updateSqlContainerThroughput(String resourceGroupName, String accountName,
String databaseName, String containerName, ThroughputSettingsUpdateParameters updateThroughputParameters,
Context context);
/**
* Migrate an Azure Cosmos DB SQL container from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> migrateSqlContainerToAutoscaleWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String containerName);
/**
* Migrate an Azure Cosmos DB SQL container from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateSqlContainerToAutoscaleAsync(String resourceGroupName, String accountName, String databaseName,
String containerName);
/**
* Migrate an Azure Cosmos DB SQL container from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateSqlContainerToAutoscale(String resourceGroupName, String accountName, String databaseName,
String containerName);
/**
* Migrate an Azure Cosmos DB SQL container from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateSqlContainerToAutoscale(String resourceGroupName, String accountName, String databaseName,
String containerName, Context context);
/**
* Migrate an Azure Cosmos DB SQL container from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono migrateSqlContainerToAutoscaleAsync(String resourceGroupName,
String accountName, String databaseName, String containerName);
/**
* Migrate an Azure Cosmos DB SQL container from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateSqlContainerToAutoscale(String resourceGroupName, String accountName,
String databaseName, String containerName);
/**
* Migrate an Azure Cosmos DB SQL container from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateSqlContainerToAutoscale(String resourceGroupName, String accountName,
String databaseName, String containerName, Context context);
/**
* Migrate an Azure Cosmos DB SQL container from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> migrateSqlContainerToManualThroughputWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String containerName);
/**
* Migrate an Azure Cosmos DB SQL container from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateSqlContainerToManualThroughputAsync(String resourceGroupName, String accountName,
String databaseName, String containerName);
/**
* Migrate an Azure Cosmos DB SQL container from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateSqlContainerToManualThroughput(String resourceGroupName, String accountName, String databaseName,
String containerName);
/**
* Migrate an Azure Cosmos DB SQL container from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateSqlContainerToManualThroughput(String resourceGroupName, String accountName, String databaseName,
String containerName, Context context);
/**
* Migrate an Azure Cosmos DB SQL container from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono migrateSqlContainerToManualThroughputAsync(String resourceGroupName,
String accountName, String databaseName, String containerName);
/**
* Migrate an Azure Cosmos DB SQL container from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateSqlContainerToManualThroughput(String resourceGroupName,
String accountName, String databaseName, String containerName);
/**
* Migrate an Azure Cosmos DB SQL container from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ThroughputSettingsGetResultsInner migrateSqlContainerToManualThroughput(String resourceGroupName,
String accountName, String databaseName, String containerName, Context context);
/**
* Lists the ClientEncryptionKeys under an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the client encryption keys and their properties as paginated
* response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listClientEncryptionKeysAsync(String resourceGroupName,
String accountName, String databaseName);
/**
* Lists the ClientEncryptionKeys under an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the client encryption keys and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listClientEncryptionKeys(String resourceGroupName,
String accountName, String databaseName);
/**
* Lists the ClientEncryptionKeys under an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the client encryption keys and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listClientEncryptionKeys(String resourceGroupName,
String accountName, String databaseName, Context context);
/**
* Gets the ClientEncryptionKey under an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param clientEncryptionKeyName Cosmos DB ClientEncryptionKey name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ClientEncryptionKey under an existing Azure Cosmos DB SQL database along with {@link Response} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getClientEncryptionKeyWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String clientEncryptionKeyName);
/**
* Gets the ClientEncryptionKey under an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param clientEncryptionKeyName Cosmos DB ClientEncryptionKey name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ClientEncryptionKey under an existing Azure Cosmos DB SQL database on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getClientEncryptionKeyAsync(String resourceGroupName, String accountName,
String databaseName, String clientEncryptionKeyName);
/**
* Gets the ClientEncryptionKey under an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param clientEncryptionKeyName Cosmos DB ClientEncryptionKey name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ClientEncryptionKey under an existing Azure Cosmos DB SQL database along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getClientEncryptionKeyWithResponse(String resourceGroupName,
String accountName, String databaseName, String clientEncryptionKeyName, Context context);
/**
* Gets the ClientEncryptionKey under an existing Azure Cosmos DB SQL database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param clientEncryptionKeyName Cosmos DB ClientEncryptionKey name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ClientEncryptionKey under an existing Azure Cosmos DB SQL database.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ClientEncryptionKeyGetResultsInner getClientEncryptionKey(String resourceGroupName, String accountName,
String databaseName, String clientEncryptionKeyName);
/**
* Create or update a ClientEncryptionKey. This API is meant to be invoked via tools such as the Azure Powershell
* (instead of directly).
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param clientEncryptionKeyName Cosmos DB ClientEncryptionKey name.
* @param createUpdateClientEncryptionKeyParameters The parameters to provide for the client encryption key.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return client Encryption Key along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createUpdateClientEncryptionKeyWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String clientEncryptionKeyName,
ClientEncryptionKeyCreateUpdateParameters createUpdateClientEncryptionKeyParameters);
/**
* Create or update a ClientEncryptionKey. This API is meant to be invoked via tools such as the Azure Powershell
* (instead of directly).
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param clientEncryptionKeyName Cosmos DB ClientEncryptionKey name.
* @param createUpdateClientEncryptionKeyParameters The parameters to provide for the client encryption key.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of client Encryption Key.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, ClientEncryptionKeyGetResultsInner>
beginCreateUpdateClientEncryptionKeyAsync(String resourceGroupName, String accountName, String databaseName,
String clientEncryptionKeyName,
ClientEncryptionKeyCreateUpdateParameters createUpdateClientEncryptionKeyParameters);
/**
* Create or update a ClientEncryptionKey. This API is meant to be invoked via tools such as the Azure Powershell
* (instead of directly).
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param clientEncryptionKeyName Cosmos DB ClientEncryptionKey name.
* @param createUpdateClientEncryptionKeyParameters The parameters to provide for the client encryption key.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of client Encryption Key.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ClientEncryptionKeyGetResultsInner>
beginCreateUpdateClientEncryptionKey(String resourceGroupName, String accountName, String databaseName,
String clientEncryptionKeyName,
ClientEncryptionKeyCreateUpdateParameters createUpdateClientEncryptionKeyParameters);
/**
* Create or update a ClientEncryptionKey. This API is meant to be invoked via tools such as the Azure Powershell
* (instead of directly).
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param clientEncryptionKeyName Cosmos DB ClientEncryptionKey name.
* @param createUpdateClientEncryptionKeyParameters The parameters to provide for the client encryption key.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of client Encryption Key.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, ClientEncryptionKeyGetResultsInner>
beginCreateUpdateClientEncryptionKey(String resourceGroupName, String accountName, String databaseName,
String clientEncryptionKeyName,
ClientEncryptionKeyCreateUpdateParameters createUpdateClientEncryptionKeyParameters, Context context);
/**
* Create or update a ClientEncryptionKey. This API is meant to be invoked via tools such as the Azure Powershell
* (instead of directly).
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param clientEncryptionKeyName Cosmos DB ClientEncryptionKey name.
* @param createUpdateClientEncryptionKeyParameters The parameters to provide for the client encryption key.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return client Encryption Key on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createUpdateClientEncryptionKeyAsync(String resourceGroupName,
String accountName, String databaseName, String clientEncryptionKeyName,
ClientEncryptionKeyCreateUpdateParameters createUpdateClientEncryptionKeyParameters);
/**
* Create or update a ClientEncryptionKey. This API is meant to be invoked via tools such as the Azure Powershell
* (instead of directly).
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param clientEncryptionKeyName Cosmos DB ClientEncryptionKey name.
* @param createUpdateClientEncryptionKeyParameters The parameters to provide for the client encryption key.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return client Encryption Key.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ClientEncryptionKeyGetResultsInner createUpdateClientEncryptionKey(String resourceGroupName, String accountName,
String databaseName, String clientEncryptionKeyName,
ClientEncryptionKeyCreateUpdateParameters createUpdateClientEncryptionKeyParameters);
/**
* Create or update a ClientEncryptionKey. This API is meant to be invoked via tools such as the Azure Powershell
* (instead of directly).
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param clientEncryptionKeyName Cosmos DB ClientEncryptionKey name.
* @param createUpdateClientEncryptionKeyParameters The parameters to provide for the client encryption key.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return client Encryption Key.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ClientEncryptionKeyGetResultsInner createUpdateClientEncryptionKey(String resourceGroupName, String accountName,
String databaseName, String clientEncryptionKeyName,
ClientEncryptionKeyCreateUpdateParameters createUpdateClientEncryptionKeyParameters, Context context);
/**
* Lists the SQL storedProcedure under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the storedProcedures and their properties as paginated
* response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listSqlStoredProceduresAsync(String resourceGroupName,
String accountName, String databaseName, String containerName);
/**
* Lists the SQL storedProcedure under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the storedProcedures and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSqlStoredProcedures(String resourceGroupName,
String accountName, String databaseName, String containerName);
/**
* Lists the SQL storedProcedure under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the storedProcedures and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSqlStoredProcedures(String resourceGroupName,
String accountName, String databaseName, String containerName, Context context);
/**
* Gets the SQL storedProcedure under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL storedProcedure under an existing Azure Cosmos DB database account along with {@link Response} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getSqlStoredProcedureWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String containerName, String storedProcedureName);
/**
* Gets the SQL storedProcedure under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL storedProcedure under an existing Azure Cosmos DB database account on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getSqlStoredProcedureAsync(String resourceGroupName, String accountName,
String databaseName, String containerName, String storedProcedureName);
/**
* Gets the SQL storedProcedure under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL storedProcedure under an existing Azure Cosmos DB database account along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getSqlStoredProcedureWithResponse(String resourceGroupName,
String accountName, String databaseName, String containerName, String storedProcedureName, Context context);
/**
* Gets the SQL storedProcedure under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL storedProcedure under an existing Azure Cosmos DB database account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlStoredProcedureGetResultsInner getSqlStoredProcedure(String resourceGroupName, String accountName,
String databaseName, String containerName, String storedProcedureName);
/**
* Create or update an Azure Cosmos DB SQL storedProcedure.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @param createUpdateSqlStoredProcedureParameters The parameters to provide for the current SQL storedProcedure.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB storedProcedure along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createUpdateSqlStoredProcedureWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String containerName, String storedProcedureName,
SqlStoredProcedureCreateUpdateParameters createUpdateSqlStoredProcedureParameters);
/**
* Create or update an Azure Cosmos DB SQL storedProcedure.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @param createUpdateSqlStoredProcedureParameters The parameters to provide for the current SQL storedProcedure.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB storedProcedure.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, SqlStoredProcedureGetResultsInner>
beginCreateUpdateSqlStoredProcedureAsync(String resourceGroupName, String accountName, String databaseName,
String containerName, String storedProcedureName,
SqlStoredProcedureCreateUpdateParameters createUpdateSqlStoredProcedureParameters);
/**
* Create or update an Azure Cosmos DB SQL storedProcedure.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @param createUpdateSqlStoredProcedureParameters The parameters to provide for the current SQL storedProcedure.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB storedProcedure.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SqlStoredProcedureGetResultsInner>
beginCreateUpdateSqlStoredProcedure(String resourceGroupName, String accountName, String databaseName,
String containerName, String storedProcedureName,
SqlStoredProcedureCreateUpdateParameters createUpdateSqlStoredProcedureParameters);
/**
* Create or update an Azure Cosmos DB SQL storedProcedure.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @param createUpdateSqlStoredProcedureParameters The parameters to provide for the current SQL storedProcedure.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB storedProcedure.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SqlStoredProcedureGetResultsInner>
beginCreateUpdateSqlStoredProcedure(String resourceGroupName, String accountName, String databaseName,
String containerName, String storedProcedureName,
SqlStoredProcedureCreateUpdateParameters createUpdateSqlStoredProcedureParameters, Context context);
/**
* Create or update an Azure Cosmos DB SQL storedProcedure.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @param createUpdateSqlStoredProcedureParameters The parameters to provide for the current SQL storedProcedure.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB storedProcedure on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createUpdateSqlStoredProcedureAsync(String resourceGroupName,
String accountName, String databaseName, String containerName, String storedProcedureName,
SqlStoredProcedureCreateUpdateParameters createUpdateSqlStoredProcedureParameters);
/**
* Create or update an Azure Cosmos DB SQL storedProcedure.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @param createUpdateSqlStoredProcedureParameters The parameters to provide for the current SQL storedProcedure.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB storedProcedure.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlStoredProcedureGetResultsInner createUpdateSqlStoredProcedure(String resourceGroupName, String accountName,
String databaseName, String containerName, String storedProcedureName,
SqlStoredProcedureCreateUpdateParameters createUpdateSqlStoredProcedureParameters);
/**
* Create or update an Azure Cosmos DB SQL storedProcedure.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @param createUpdateSqlStoredProcedureParameters The parameters to provide for the current SQL storedProcedure.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB storedProcedure.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlStoredProcedureGetResultsInner createUpdateSqlStoredProcedure(String resourceGroupName, String accountName,
String databaseName, String containerName, String storedProcedureName,
SqlStoredProcedureCreateUpdateParameters createUpdateSqlStoredProcedureParameters, Context context);
/**
* Deletes an existing Azure Cosmos DB SQL storedProcedure.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deleteSqlStoredProcedureWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String containerName, String storedProcedureName);
/**
* Deletes an existing Azure Cosmos DB SQL storedProcedure.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteSqlStoredProcedureAsync(String resourceGroupName, String accountName,
String databaseName, String containerName, String storedProcedureName);
/**
* Deletes an existing Azure Cosmos DB SQL storedProcedure.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSqlStoredProcedure(String resourceGroupName, String accountName,
String databaseName, String containerName, String storedProcedureName);
/**
* Deletes an existing Azure Cosmos DB SQL storedProcedure.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSqlStoredProcedure(String resourceGroupName, String accountName,
String databaseName, String containerName, String storedProcedureName, Context context);
/**
* Deletes an existing Azure Cosmos DB SQL storedProcedure.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteSqlStoredProcedureAsync(String resourceGroupName, String accountName, String databaseName,
String containerName, String storedProcedureName);
/**
* Deletes an existing Azure Cosmos DB SQL storedProcedure.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSqlStoredProcedure(String resourceGroupName, String accountName, String databaseName,
String containerName, String storedProcedureName);
/**
* Deletes an existing Azure Cosmos DB SQL storedProcedure.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param storedProcedureName Cosmos DB storedProcedure name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSqlStoredProcedure(String resourceGroupName, String accountName, String databaseName,
String containerName, String storedProcedureName, Context context);
/**
* Lists the SQL userDefinedFunction under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the userDefinedFunctions and their properties as paginated
* response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listSqlUserDefinedFunctionsAsync(String resourceGroupName,
String accountName, String databaseName, String containerName);
/**
* Lists the SQL userDefinedFunction under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the userDefinedFunctions and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSqlUserDefinedFunctions(String resourceGroupName,
String accountName, String databaseName, String containerName);
/**
* Lists the SQL userDefinedFunction under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the userDefinedFunctions and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSqlUserDefinedFunctions(String resourceGroupName,
String accountName, String databaseName, String containerName, Context context);
/**
* Gets the SQL userDefinedFunction under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL userDefinedFunction under an existing Azure Cosmos DB database account along with
* {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getSqlUserDefinedFunctionWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String containerName,
String userDefinedFunctionName);
/**
* Gets the SQL userDefinedFunction under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL userDefinedFunction under an existing Azure Cosmos DB database account on successful completion
* of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getSqlUserDefinedFunctionAsync(String resourceGroupName,
String accountName, String databaseName, String containerName, String userDefinedFunctionName);
/**
* Gets the SQL userDefinedFunction under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL userDefinedFunction under an existing Azure Cosmos DB database account along with
* {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getSqlUserDefinedFunctionWithResponse(String resourceGroupName,
String accountName, String databaseName, String containerName, String userDefinedFunctionName, Context context);
/**
* Gets the SQL userDefinedFunction under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL userDefinedFunction under an existing Azure Cosmos DB database account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlUserDefinedFunctionGetResultsInner getSqlUserDefinedFunction(String resourceGroupName, String accountName,
String databaseName, String containerName, String userDefinedFunctionName);
/**
* Create or update an Azure Cosmos DB SQL userDefinedFunction.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @param createUpdateSqlUserDefinedFunctionParameters The parameters to provide for the current SQL
* userDefinedFunction.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB userDefinedFunction along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createUpdateSqlUserDefinedFunctionWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String containerName, String userDefinedFunctionName,
SqlUserDefinedFunctionCreateUpdateParameters createUpdateSqlUserDefinedFunctionParameters);
/**
* Create or update an Azure Cosmos DB SQL userDefinedFunction.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @param createUpdateSqlUserDefinedFunctionParameters The parameters to provide for the current SQL
* userDefinedFunction.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB userDefinedFunction.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, SqlUserDefinedFunctionGetResultsInner>
beginCreateUpdateSqlUserDefinedFunctionAsync(String resourceGroupName, String accountName, String databaseName,
String containerName, String userDefinedFunctionName,
SqlUserDefinedFunctionCreateUpdateParameters createUpdateSqlUserDefinedFunctionParameters);
/**
* Create or update an Azure Cosmos DB SQL userDefinedFunction.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @param createUpdateSqlUserDefinedFunctionParameters The parameters to provide for the current SQL
* userDefinedFunction.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB userDefinedFunction.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SqlUserDefinedFunctionGetResultsInner>
beginCreateUpdateSqlUserDefinedFunction(String resourceGroupName, String accountName, String databaseName,
String containerName, String userDefinedFunctionName,
SqlUserDefinedFunctionCreateUpdateParameters createUpdateSqlUserDefinedFunctionParameters);
/**
* Create or update an Azure Cosmos DB SQL userDefinedFunction.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @param createUpdateSqlUserDefinedFunctionParameters The parameters to provide for the current SQL
* userDefinedFunction.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB userDefinedFunction.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SqlUserDefinedFunctionGetResultsInner>
beginCreateUpdateSqlUserDefinedFunction(String resourceGroupName, String accountName, String databaseName,
String containerName, String userDefinedFunctionName,
SqlUserDefinedFunctionCreateUpdateParameters createUpdateSqlUserDefinedFunctionParameters, Context context);
/**
* Create or update an Azure Cosmos DB SQL userDefinedFunction.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @param createUpdateSqlUserDefinedFunctionParameters The parameters to provide for the current SQL
* userDefinedFunction.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB userDefinedFunction on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createUpdateSqlUserDefinedFunctionAsync(String resourceGroupName,
String accountName, String databaseName, String containerName, String userDefinedFunctionName,
SqlUserDefinedFunctionCreateUpdateParameters createUpdateSqlUserDefinedFunctionParameters);
/**
* Create or update an Azure Cosmos DB SQL userDefinedFunction.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @param createUpdateSqlUserDefinedFunctionParameters The parameters to provide for the current SQL
* userDefinedFunction.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB userDefinedFunction.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlUserDefinedFunctionGetResultsInner createUpdateSqlUserDefinedFunction(String resourceGroupName,
String accountName, String databaseName, String containerName, String userDefinedFunctionName,
SqlUserDefinedFunctionCreateUpdateParameters createUpdateSqlUserDefinedFunctionParameters);
/**
* Create or update an Azure Cosmos DB SQL userDefinedFunction.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @param createUpdateSqlUserDefinedFunctionParameters The parameters to provide for the current SQL
* userDefinedFunction.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB userDefinedFunction.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlUserDefinedFunctionGetResultsInner createUpdateSqlUserDefinedFunction(String resourceGroupName,
String accountName, String databaseName, String containerName, String userDefinedFunctionName,
SqlUserDefinedFunctionCreateUpdateParameters createUpdateSqlUserDefinedFunctionParameters, Context context);
/**
* Deletes an existing Azure Cosmos DB SQL userDefinedFunction.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deleteSqlUserDefinedFunctionWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String containerName, String userDefinedFunctionName);
/**
* Deletes an existing Azure Cosmos DB SQL userDefinedFunction.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteSqlUserDefinedFunctionAsync(String resourceGroupName,
String accountName, String databaseName, String containerName, String userDefinedFunctionName);
/**
* Deletes an existing Azure Cosmos DB SQL userDefinedFunction.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSqlUserDefinedFunction(String resourceGroupName, String accountName,
String databaseName, String containerName, String userDefinedFunctionName);
/**
* Deletes an existing Azure Cosmos DB SQL userDefinedFunction.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSqlUserDefinedFunction(String resourceGroupName, String accountName,
String databaseName, String containerName, String userDefinedFunctionName, Context context);
/**
* Deletes an existing Azure Cosmos DB SQL userDefinedFunction.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteSqlUserDefinedFunctionAsync(String resourceGroupName, String accountName, String databaseName,
String containerName, String userDefinedFunctionName);
/**
* Deletes an existing Azure Cosmos DB SQL userDefinedFunction.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSqlUserDefinedFunction(String resourceGroupName, String accountName, String databaseName,
String containerName, String userDefinedFunctionName);
/**
* Deletes an existing Azure Cosmos DB SQL userDefinedFunction.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param userDefinedFunctionName Cosmos DB userDefinedFunction name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSqlUserDefinedFunction(String resourceGroupName, String accountName, String databaseName,
String containerName, String userDefinedFunctionName, Context context);
/**
* Lists the SQL trigger under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the triggers and their properties as paginated response with
* {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listSqlTriggersAsync(String resourceGroupName, String accountName,
String databaseName, String containerName);
/**
* Lists the SQL trigger under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the triggers and their properties as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSqlTriggers(String resourceGroupName, String accountName,
String databaseName, String containerName);
/**
* Lists the SQL trigger under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the triggers and their properties as paginated response with
* {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSqlTriggers(String resourceGroupName, String accountName,
String databaseName, String containerName, Context context);
/**
* Gets the SQL trigger under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL trigger under an existing Azure Cosmos DB database account along with {@link Response} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getSqlTriggerWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String containerName, String triggerName);
/**
* Gets the SQL trigger under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL trigger under an existing Azure Cosmos DB database account on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getSqlTriggerAsync(String resourceGroupName, String accountName,
String databaseName, String containerName, String triggerName);
/**
* Gets the SQL trigger under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL trigger under an existing Azure Cosmos DB database account along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getSqlTriggerWithResponse(String resourceGroupName, String accountName,
String databaseName, String containerName, String triggerName, Context context);
/**
* Gets the SQL trigger under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the SQL trigger under an existing Azure Cosmos DB database account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlTriggerGetResultsInner getSqlTrigger(String resourceGroupName, String accountName, String databaseName,
String containerName, String triggerName);
/**
* Create or update an Azure Cosmos DB SQL trigger.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @param createUpdateSqlTriggerParameters The parameters to provide for the current SQL trigger.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB trigger along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createUpdateSqlTriggerWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String containerName, String triggerName,
SqlTriggerCreateUpdateParameters createUpdateSqlTriggerParameters);
/**
* Create or update an Azure Cosmos DB SQL trigger.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @param createUpdateSqlTriggerParameters The parameters to provide for the current SQL trigger.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB trigger.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, SqlTriggerGetResultsInner> beginCreateUpdateSqlTriggerAsync(
String resourceGroupName, String accountName, String databaseName, String containerName, String triggerName,
SqlTriggerCreateUpdateParameters createUpdateSqlTriggerParameters);
/**
* Create or update an Azure Cosmos DB SQL trigger.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @param createUpdateSqlTriggerParameters The parameters to provide for the current SQL trigger.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB trigger.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SqlTriggerGetResultsInner> beginCreateUpdateSqlTrigger(
String resourceGroupName, String accountName, String databaseName, String containerName, String triggerName,
SqlTriggerCreateUpdateParameters createUpdateSqlTriggerParameters);
/**
* Create or update an Azure Cosmos DB SQL trigger.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @param createUpdateSqlTriggerParameters The parameters to provide for the current SQL trigger.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB trigger.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SqlTriggerGetResultsInner> beginCreateUpdateSqlTrigger(
String resourceGroupName, String accountName, String databaseName, String containerName, String triggerName,
SqlTriggerCreateUpdateParameters createUpdateSqlTriggerParameters, Context context);
/**
* Create or update an Azure Cosmos DB SQL trigger.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @param createUpdateSqlTriggerParameters The parameters to provide for the current SQL trigger.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB trigger on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createUpdateSqlTriggerAsync(String resourceGroupName, String accountName,
String databaseName, String containerName, String triggerName,
SqlTriggerCreateUpdateParameters createUpdateSqlTriggerParameters);
/**
* Create or update an Azure Cosmos DB SQL trigger.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @param createUpdateSqlTriggerParameters The parameters to provide for the current SQL trigger.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB trigger.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlTriggerGetResultsInner createUpdateSqlTrigger(String resourceGroupName, String accountName, String databaseName,
String containerName, String triggerName, SqlTriggerCreateUpdateParameters createUpdateSqlTriggerParameters);
/**
* Create or update an Azure Cosmos DB SQL trigger.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @param createUpdateSqlTriggerParameters The parameters to provide for the current SQL trigger.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB trigger.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlTriggerGetResultsInner createUpdateSqlTrigger(String resourceGroupName, String accountName, String databaseName,
String containerName, String triggerName, SqlTriggerCreateUpdateParameters createUpdateSqlTriggerParameters,
Context context);
/**
* Deletes an existing Azure Cosmos DB SQL trigger.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deleteSqlTriggerWithResponseAsync(String resourceGroupName, String accountName,
String databaseName, String containerName, String triggerName);
/**
* Deletes an existing Azure Cosmos DB SQL trigger.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteSqlTriggerAsync(String resourceGroupName, String accountName,
String databaseName, String containerName, String triggerName);
/**
* Deletes an existing Azure Cosmos DB SQL trigger.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSqlTrigger(String resourceGroupName, String accountName,
String databaseName, String containerName, String triggerName);
/**
* Deletes an existing Azure Cosmos DB SQL trigger.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSqlTrigger(String resourceGroupName, String accountName,
String databaseName, String containerName, String triggerName, Context context);
/**
* Deletes an existing Azure Cosmos DB SQL trigger.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteSqlTriggerAsync(String resourceGroupName, String accountName, String databaseName,
String containerName, String triggerName);
/**
* Deletes an existing Azure Cosmos DB SQL trigger.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSqlTrigger(String resourceGroupName, String accountName, String databaseName, String containerName,
String triggerName);
/**
* Deletes an existing Azure Cosmos DB SQL trigger.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param triggerName Cosmos DB trigger name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSqlTrigger(String resourceGroupName, String accountName, String databaseName, String containerName,
String triggerName, Context context);
/**
* Retrieves the properties of an existing Azure Cosmos DB SQL Role Definition with the given Id.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB SQL Role Definition along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getSqlRoleDefinitionWithResponseAsync(String roleDefinitionId,
String resourceGroupName, String accountName);
/**
* Retrieves the properties of an existing Azure Cosmos DB SQL Role Definition with the given Id.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB SQL Role Definition on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getSqlRoleDefinitionAsync(String roleDefinitionId, String resourceGroupName,
String accountName);
/**
* Retrieves the properties of an existing Azure Cosmos DB SQL Role Definition with the given Id.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB SQL Role Definition along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getSqlRoleDefinitionWithResponse(String roleDefinitionId,
String resourceGroupName, String accountName, Context context);
/**
* Retrieves the properties of an existing Azure Cosmos DB SQL Role Definition with the given Id.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB SQL Role Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlRoleDefinitionGetResultsInner getSqlRoleDefinition(String roleDefinitionId, String resourceGroupName,
String accountName);
/**
* Creates or updates an Azure Cosmos DB SQL Role Definition.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateSqlRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB SQL Role Definition along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createUpdateSqlRoleDefinitionWithResponseAsync(String roleDefinitionId,
String resourceGroupName, String accountName,
SqlRoleDefinitionCreateUpdateParameters createUpdateSqlRoleDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB SQL Role Definition.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateSqlRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB SQL Role Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, SqlRoleDefinitionGetResultsInner>
beginCreateUpdateSqlRoleDefinitionAsync(String roleDefinitionId, String resourceGroupName, String accountName,
SqlRoleDefinitionCreateUpdateParameters createUpdateSqlRoleDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB SQL Role Definition.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateSqlRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB SQL Role Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SqlRoleDefinitionGetResultsInner>
beginCreateUpdateSqlRoleDefinition(String roleDefinitionId, String resourceGroupName, String accountName,
SqlRoleDefinitionCreateUpdateParameters createUpdateSqlRoleDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB SQL Role Definition.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateSqlRoleDefinitionParameters The properties required to create or update a Role Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB SQL Role Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SqlRoleDefinitionGetResultsInner>
beginCreateUpdateSqlRoleDefinition(String roleDefinitionId, String resourceGroupName, String accountName,
SqlRoleDefinitionCreateUpdateParameters createUpdateSqlRoleDefinitionParameters, Context context);
/**
* Creates or updates an Azure Cosmos DB SQL Role Definition.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateSqlRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB SQL Role Definition on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createUpdateSqlRoleDefinitionAsync(String roleDefinitionId,
String resourceGroupName, String accountName,
SqlRoleDefinitionCreateUpdateParameters createUpdateSqlRoleDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB SQL Role Definition.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateSqlRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB SQL Role Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlRoleDefinitionGetResultsInner createUpdateSqlRoleDefinition(String roleDefinitionId, String resourceGroupName,
String accountName, SqlRoleDefinitionCreateUpdateParameters createUpdateSqlRoleDefinitionParameters);
/**
* Creates or updates an Azure Cosmos DB SQL Role Definition.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateSqlRoleDefinitionParameters The properties required to create or update a Role Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB SQL Role Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlRoleDefinitionGetResultsInner createUpdateSqlRoleDefinition(String roleDefinitionId, String resourceGroupName,
String accountName, SqlRoleDefinitionCreateUpdateParameters createUpdateSqlRoleDefinitionParameters,
Context context);
/**
* Deletes an existing Azure Cosmos DB SQL Role Definition.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deleteSqlRoleDefinitionWithResponseAsync(String roleDefinitionId,
String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB SQL Role Definition.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteSqlRoleDefinitionAsync(String roleDefinitionId,
String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB SQL Role Definition.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSqlRoleDefinition(String roleDefinitionId, String resourceGroupName,
String accountName);
/**
* Deletes an existing Azure Cosmos DB SQL Role Definition.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSqlRoleDefinition(String roleDefinitionId, String resourceGroupName,
String accountName, Context context);
/**
* Deletes an existing Azure Cosmos DB SQL Role Definition.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteSqlRoleDefinitionAsync(String roleDefinitionId, String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB SQL Role Definition.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSqlRoleDefinition(String roleDefinitionId, String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB SQL Role Definition.
*
* @param roleDefinitionId The GUID for the Role Definition.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSqlRoleDefinition(String roleDefinitionId, String resourceGroupName, String accountName,
Context context);
/**
* Retrieves the list of all Azure Cosmos DB SQL Role Definitions.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Role Definitions as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listSqlRoleDefinitionsAsync(String resourceGroupName,
String accountName);
/**
* Retrieves the list of all Azure Cosmos DB SQL Role Definitions.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Role Definitions as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSqlRoleDefinitions(String resourceGroupName,
String accountName);
/**
* Retrieves the list of all Azure Cosmos DB SQL Role Definitions.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Role Definitions as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSqlRoleDefinitions(String resourceGroupName, String accountName,
Context context);
/**
* Retrieves the properties of an existing Azure Cosmos DB SQL Role Assignment with the given Id.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Role Assignment along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getSqlRoleAssignmentWithResponseAsync(String roleAssignmentId,
String resourceGroupName, String accountName);
/**
* Retrieves the properties of an existing Azure Cosmos DB SQL Role Assignment with the given Id.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Role Assignment on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getSqlRoleAssignmentAsync(String roleAssignmentId, String resourceGroupName,
String accountName);
/**
* Retrieves the properties of an existing Azure Cosmos DB SQL Role Assignment with the given Id.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Role Assignment along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getSqlRoleAssignmentWithResponse(String roleAssignmentId,
String resourceGroupName, String accountName, Context context);
/**
* Retrieves the properties of an existing Azure Cosmos DB SQL Role Assignment with the given Id.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Role Assignment.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlRoleAssignmentGetResultsInner getSqlRoleAssignment(String roleAssignmentId, String resourceGroupName,
String accountName);
/**
* Creates or updates an Azure Cosmos DB SQL Role Assignment.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateSqlRoleAssignmentParameters The properties required to create or update a Role Assignment.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Role Assignment along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createUpdateSqlRoleAssignmentWithResponseAsync(String roleAssignmentId,
String resourceGroupName, String accountName,
SqlRoleAssignmentCreateUpdateParameters createUpdateSqlRoleAssignmentParameters);
/**
* Creates or updates an Azure Cosmos DB SQL Role Assignment.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateSqlRoleAssignmentParameters The properties required to create or update a Role Assignment.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB Role Assignment.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, SqlRoleAssignmentGetResultsInner>
beginCreateUpdateSqlRoleAssignmentAsync(String roleAssignmentId, String resourceGroupName, String accountName,
SqlRoleAssignmentCreateUpdateParameters createUpdateSqlRoleAssignmentParameters);
/**
* Creates or updates an Azure Cosmos DB SQL Role Assignment.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateSqlRoleAssignmentParameters The properties required to create or update a Role Assignment.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB Role Assignment.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SqlRoleAssignmentGetResultsInner>
beginCreateUpdateSqlRoleAssignment(String roleAssignmentId, String resourceGroupName, String accountName,
SqlRoleAssignmentCreateUpdateParameters createUpdateSqlRoleAssignmentParameters);
/**
* Creates or updates an Azure Cosmos DB SQL Role Assignment.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateSqlRoleAssignmentParameters The properties required to create or update a Role Assignment.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB Role Assignment.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, SqlRoleAssignmentGetResultsInner>
beginCreateUpdateSqlRoleAssignment(String roleAssignmentId, String resourceGroupName, String accountName,
SqlRoleAssignmentCreateUpdateParameters createUpdateSqlRoleAssignmentParameters, Context context);
/**
* Creates or updates an Azure Cosmos DB SQL Role Assignment.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateSqlRoleAssignmentParameters The properties required to create or update a Role Assignment.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Role Assignment on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createUpdateSqlRoleAssignmentAsync(String roleAssignmentId,
String resourceGroupName, String accountName,
SqlRoleAssignmentCreateUpdateParameters createUpdateSqlRoleAssignmentParameters);
/**
* Creates or updates an Azure Cosmos DB SQL Role Assignment.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateSqlRoleAssignmentParameters The properties required to create or update a Role Assignment.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Role Assignment.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlRoleAssignmentGetResultsInner createUpdateSqlRoleAssignment(String roleAssignmentId, String resourceGroupName,
String accountName, SqlRoleAssignmentCreateUpdateParameters createUpdateSqlRoleAssignmentParameters);
/**
* Creates or updates an Azure Cosmos DB SQL Role Assignment.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateSqlRoleAssignmentParameters The properties required to create or update a Role Assignment.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Role Assignment.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
SqlRoleAssignmentGetResultsInner createUpdateSqlRoleAssignment(String roleAssignmentId, String resourceGroupName,
String accountName, SqlRoleAssignmentCreateUpdateParameters createUpdateSqlRoleAssignmentParameters,
Context context);
/**
* Deletes an existing Azure Cosmos DB SQL Role Assignment.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> deleteSqlRoleAssignmentWithResponseAsync(String roleAssignmentId,
String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB SQL Role Assignment.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginDeleteSqlRoleAssignmentAsync(String roleAssignmentId,
String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB SQL Role Assignment.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSqlRoleAssignment(String roleAssignmentId, String resourceGroupName,
String accountName);
/**
* Deletes an existing Azure Cosmos DB SQL Role Assignment.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginDeleteSqlRoleAssignment(String roleAssignmentId, String resourceGroupName,
String accountName, Context context);
/**
* Deletes an existing Azure Cosmos DB SQL Role Assignment.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteSqlRoleAssignmentAsync(String roleAssignmentId, String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB SQL Role Assignment.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSqlRoleAssignment(String roleAssignmentId, String resourceGroupName, String accountName);
/**
* Deletes an existing Azure Cosmos DB SQL Role Assignment.
*
* @param roleAssignmentId The GUID for the Role Assignment.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void deleteSqlRoleAssignment(String roleAssignmentId, String resourceGroupName, String accountName,
Context context);
/**
* Retrieves the list of all Azure Cosmos DB SQL Role Assignments.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Role Assignments as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listSqlRoleAssignmentsAsync(String resourceGroupName,
String accountName);
/**
* Retrieves the list of all Azure Cosmos DB SQL Role Assignments.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Role Assignments as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSqlRoleAssignments(String resourceGroupName,
String accountName);
/**
* Retrieves the list of all Azure Cosmos DB SQL Role Assignments.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Role Assignments as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listSqlRoleAssignments(String resourceGroupName, String accountName,
Context context);
/**
* Retrieves continuous backup information for a container resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param location The name of the continuous backup restore location.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return backup information of a resource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> retrieveContinuousBackupInformationWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String containerName, ContinuousBackupRestoreLocation location);
/**
* Retrieves continuous backup information for a container resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param location The name of the continuous backup restore location.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of backup information of a resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, BackupInformationInner>
beginRetrieveContinuousBackupInformationAsync(String resourceGroupName, String accountName, String databaseName,
String containerName, ContinuousBackupRestoreLocation location);
/**
* Retrieves continuous backup information for a container resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param location The name of the continuous backup restore location.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of backup information of a resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, BackupInformationInner> beginRetrieveContinuousBackupInformation(
String resourceGroupName, String accountName, String databaseName, String containerName,
ContinuousBackupRestoreLocation location);
/**
* Retrieves continuous backup information for a container resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param location The name of the continuous backup restore location.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of backup information of a resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, BackupInformationInner> beginRetrieveContinuousBackupInformation(
String resourceGroupName, String accountName, String databaseName, String containerName,
ContinuousBackupRestoreLocation location, Context context);
/**
* Retrieves continuous backup information for a container resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param location The name of the continuous backup restore location.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return backup information of a resource on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono retrieveContinuousBackupInformationAsync(String resourceGroupName, String accountName,
String databaseName, String containerName, ContinuousBackupRestoreLocation location);
/**
* Retrieves continuous backup information for a container resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param location The name of the continuous backup restore location.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return backup information of a resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
BackupInformationInner retrieveContinuousBackupInformation(String resourceGroupName, String accountName,
String databaseName, String containerName, ContinuousBackupRestoreLocation location);
/**
* Retrieves continuous backup information for a container resource.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param containerName Cosmos DB container name.
* @param location The name of the continuous backup restore location.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return backup information of a resource.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
BackupInformationInner retrieveContinuousBackupInformation(String resourceGroupName, String accountName,
String databaseName, String containerName, ContinuousBackupRestoreLocation location, Context context);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy