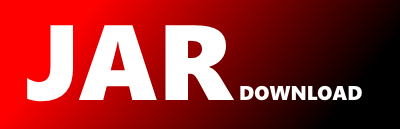
com.azure.resourcemanager.cosmos.fluent.models.DatabaseAccountGetProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-cosmos Show documentation
Show all versions of azure-resourcemanager-cosmos Show documentation
This package contains Microsoft Azure CosmosDB SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.cosmos.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.cosmos.models.AnalyticalStorageConfiguration;
import com.azure.resourcemanager.cosmos.models.ApiProperties;
import com.azure.resourcemanager.cosmos.models.BackupPolicy;
import com.azure.resourcemanager.cosmos.models.Capability;
import com.azure.resourcemanager.cosmos.models.Capacity;
import com.azure.resourcemanager.cosmos.models.ConnectorOffer;
import com.azure.resourcemanager.cosmos.models.ConsistencyPolicy;
import com.azure.resourcemanager.cosmos.models.CorsPolicy;
import com.azure.resourcemanager.cosmos.models.CreateMode;
import com.azure.resourcemanager.cosmos.models.DatabaseAccountKeysMetadata;
import com.azure.resourcemanager.cosmos.models.DatabaseAccountOfferType;
import com.azure.resourcemanager.cosmos.models.FailoverPolicy;
import com.azure.resourcemanager.cosmos.models.IpAddressOrRange;
import com.azure.resourcemanager.cosmos.models.Location;
import com.azure.resourcemanager.cosmos.models.MinimalTlsVersion;
import com.azure.resourcemanager.cosmos.models.NetworkAclBypass;
import com.azure.resourcemanager.cosmos.models.PublicNetworkAccess;
import com.azure.resourcemanager.cosmos.models.RestoreParameters;
import com.azure.resourcemanager.cosmos.models.VirtualNetworkRule;
import java.io.IOException;
import java.util.List;
/**
* Properties for the database account.
*/
@Fluent
public final class DatabaseAccountGetProperties implements JsonSerializable {
/*
* The status of the Cosmos DB account at the time the operation was called. The status can be one of following.
* 'Creating' – the Cosmos DB account is being created. When an account is in Creating state, only properties that
* are specified as input for the Create Cosmos DB account operation are returned. 'Succeeded' – the Cosmos DB
* account is active for use. 'Updating' – the Cosmos DB account is being updated. 'Deleting' – the Cosmos DB
* account is being deleted. 'Failed' – the Cosmos DB account failed creation. 'DeletionFailed' – the Cosmos DB
* account deletion failed.
*/
private String provisioningState;
/*
* The connection endpoint for the Cosmos DB database account.
*/
private String documentEndpoint;
/*
* The offer type for the Cosmos DB database account. Default value: Standard.
*/
private DatabaseAccountOfferType databaseAccountOfferType;
/*
* List of IpRules.
*/
private List ipRules;
/*
* Flag to indicate whether to enable/disable Virtual Network ACL rules.
*/
private Boolean isVirtualNetworkFilterEnabled;
/*
* Enables automatic failover of the write region in the rare event that the region is unavailable due to an outage.
* Automatic failover will result in a new write region for the account and is chosen based on the failover
* priorities configured for the account.
*/
private Boolean enableAutomaticFailover;
/*
* The consistency policy for the Cosmos DB database account.
*/
private ConsistencyPolicy consistencyPolicy;
/*
* List of Cosmos DB capabilities for the account
*/
private List capabilities;
/*
* An array that contains the write location for the Cosmos DB account.
*/
private List writeLocations;
/*
* An array that contains of the read locations enabled for the Cosmos DB account.
*/
private List readLocations;
/*
* An array that contains all of the locations enabled for the Cosmos DB account.
*/
private List locations;
/*
* An array that contains the regions ordered by their failover priorities.
*/
private List failoverPolicies;
/*
* List of Virtual Network ACL rules configured for the Cosmos DB account.
*/
private List virtualNetworkRules;
/*
* List of Private Endpoint Connections configured for the Cosmos DB account.
*/
private List privateEndpointConnections;
/*
* Enables the account to write in multiple locations
*/
private Boolean enableMultipleWriteLocations;
/*
* Enables the cassandra connector on the Cosmos DB C* account
*/
private Boolean enableCassandraConnector;
/*
* The cassandra connector offer type for the Cosmos DB database C* account.
*/
private ConnectorOffer connectorOffer;
/*
* Disable write operations on metadata resources (databases, containers, throughput) via account keys
*/
private Boolean disableKeyBasedMetadataWriteAccess;
/*
* The URI of the key vault
*/
private String keyVaultKeyUri;
/*
* The default identity for accessing key vault used in features like customer managed keys. The default identity
* needs to be explicitly set by the users. It can be "FirstPartyIdentity", "SystemAssignedIdentity" and more.
*/
private String defaultIdentity;
/*
* Whether requests from Public Network are allowed
*/
private PublicNetworkAccess publicNetworkAccess;
/*
* Flag to indicate whether Free Tier is enabled.
*/
private Boolean enableFreeTier;
/*
* API specific properties.
*/
private ApiProperties apiProperties;
/*
* Flag to indicate whether to enable storage analytics.
*/
private Boolean enableAnalyticalStorage;
/*
* Analytical storage specific properties.
*/
private AnalyticalStorageConfiguration analyticalStorageConfiguration;
/*
* A unique identifier assigned to the database account
*/
private String instanceId;
/*
* Enum to indicate the mode of account creation.
*/
private CreateMode createMode;
/*
* Parameters to indicate the information about the restore.
*/
private RestoreParameters restoreParameters;
/*
* The object representing the policy for taking backups on an account.
*/
private BackupPolicy backupPolicy;
/*
* The CORS policy for the Cosmos DB database account.
*/
private List cors;
/*
* Indicates what services are allowed to bypass firewall checks.
*/
private NetworkAclBypass networkAclBypass;
/*
* An array that contains the Resource Ids for Network Acl Bypass for the Cosmos DB account.
*/
private List networkAclBypassResourceIds;
/*
* Opt-out of local authentication and ensure only MSI and AAD can be used exclusively for authentication.
*/
private Boolean disableLocalAuth;
/*
* The object that represents all properties related to capacity enforcement on an account.
*/
private Capacity capacity;
/*
* The object that represents the metadata for the Account Keys of the Cosmos DB account.
*/
private DatabaseAccountKeysMetadata keysMetadata;
/*
* Flag to indicate enabling/disabling of Partition Merge feature on the account
*/
private Boolean enablePartitionMerge;
/*
* Indicates the minimum allowed Tls version. The default value is Tls 1.2. Cassandra and Mongo APIs only work with
* Tls 1.2.
*/
private MinimalTlsVersion minimalTlsVersion;
/*
* Flag to indicate enabling/disabling of Burst Capacity feature on the account
*/
private Boolean enableBurstCapacity;
/*
* Indicates the status of the Customer Managed Key feature on the account. In case there are errors, the property
* provides troubleshooting guidance.
*/
private String customerManagedKeyStatus;
/**
* Creates an instance of DatabaseAccountGetProperties class.
*/
public DatabaseAccountGetProperties() {
}
/**
* Get the provisioningState property: The status of the Cosmos DB account at the time the operation was called. The
* status can be one of following. 'Creating' – the Cosmos DB account is being created. When an account is in
* Creating state, only properties that are specified as input for the Create Cosmos DB account operation are
* returned. 'Succeeded' – the Cosmos DB account is active for use. 'Updating' – the Cosmos DB account is being
* updated. 'Deleting' – the Cosmos DB account is being deleted. 'Failed' – the Cosmos DB account failed creation.
* 'DeletionFailed' – the Cosmos DB account deletion failed.
*
* @return the provisioningState value.
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* Get the documentEndpoint property: The connection endpoint for the Cosmos DB database account.
*
* @return the documentEndpoint value.
*/
public String documentEndpoint() {
return this.documentEndpoint;
}
/**
* Get the databaseAccountOfferType property: The offer type for the Cosmos DB database account. Default value:
* Standard.
*
* @return the databaseAccountOfferType value.
*/
public DatabaseAccountOfferType databaseAccountOfferType() {
return this.databaseAccountOfferType;
}
/**
* Get the ipRules property: List of IpRules.
*
* @return the ipRules value.
*/
public List ipRules() {
return this.ipRules;
}
/**
* Set the ipRules property: List of IpRules.
*
* @param ipRules the ipRules value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withIpRules(List ipRules) {
this.ipRules = ipRules;
return this;
}
/**
* Get the isVirtualNetworkFilterEnabled property: Flag to indicate whether to enable/disable Virtual Network ACL
* rules.
*
* @return the isVirtualNetworkFilterEnabled value.
*/
public Boolean isVirtualNetworkFilterEnabled() {
return this.isVirtualNetworkFilterEnabled;
}
/**
* Set the isVirtualNetworkFilterEnabled property: Flag to indicate whether to enable/disable Virtual Network ACL
* rules.
*
* @param isVirtualNetworkFilterEnabled the isVirtualNetworkFilterEnabled value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withIsVirtualNetworkFilterEnabled(Boolean isVirtualNetworkFilterEnabled) {
this.isVirtualNetworkFilterEnabled = isVirtualNetworkFilterEnabled;
return this;
}
/**
* Get the enableAutomaticFailover property: Enables automatic failover of the write region in the rare event that
* the region is unavailable due to an outage. Automatic failover will result in a new write region for the account
* and is chosen based on the failover priorities configured for the account.
*
* @return the enableAutomaticFailover value.
*/
public Boolean enableAutomaticFailover() {
return this.enableAutomaticFailover;
}
/**
* Set the enableAutomaticFailover property: Enables automatic failover of the write region in the rare event that
* the region is unavailable due to an outage. Automatic failover will result in a new write region for the account
* and is chosen based on the failover priorities configured for the account.
*
* @param enableAutomaticFailover the enableAutomaticFailover value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withEnableAutomaticFailover(Boolean enableAutomaticFailover) {
this.enableAutomaticFailover = enableAutomaticFailover;
return this;
}
/**
* Get the consistencyPolicy property: The consistency policy for the Cosmos DB database account.
*
* @return the consistencyPolicy value.
*/
public ConsistencyPolicy consistencyPolicy() {
return this.consistencyPolicy;
}
/**
* Set the consistencyPolicy property: The consistency policy for the Cosmos DB database account.
*
* @param consistencyPolicy the consistencyPolicy value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withConsistencyPolicy(ConsistencyPolicy consistencyPolicy) {
this.consistencyPolicy = consistencyPolicy;
return this;
}
/**
* Get the capabilities property: List of Cosmos DB capabilities for the account.
*
* @return the capabilities value.
*/
public List capabilities() {
return this.capabilities;
}
/**
* Set the capabilities property: List of Cosmos DB capabilities for the account.
*
* @param capabilities the capabilities value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withCapabilities(List capabilities) {
this.capabilities = capabilities;
return this;
}
/**
* Get the writeLocations property: An array that contains the write location for the Cosmos DB account.
*
* @return the writeLocations value.
*/
public List writeLocations() {
return this.writeLocations;
}
/**
* Get the readLocations property: An array that contains of the read locations enabled for the Cosmos DB account.
*
* @return the readLocations value.
*/
public List readLocations() {
return this.readLocations;
}
/**
* Get the locations property: An array that contains all of the locations enabled for the Cosmos DB account.
*
* @return the locations value.
*/
public List locations() {
return this.locations;
}
/**
* Get the failoverPolicies property: An array that contains the regions ordered by their failover priorities.
*
* @return the failoverPolicies value.
*/
public List failoverPolicies() {
return this.failoverPolicies;
}
/**
* Get the virtualNetworkRules property: List of Virtual Network ACL rules configured for the Cosmos DB account.
*
* @return the virtualNetworkRules value.
*/
public List virtualNetworkRules() {
return this.virtualNetworkRules;
}
/**
* Set the virtualNetworkRules property: List of Virtual Network ACL rules configured for the Cosmos DB account.
*
* @param virtualNetworkRules the virtualNetworkRules value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withVirtualNetworkRules(List virtualNetworkRules) {
this.virtualNetworkRules = virtualNetworkRules;
return this;
}
/**
* Get the privateEndpointConnections property: List of Private Endpoint Connections configured for the Cosmos DB
* account.
*
* @return the privateEndpointConnections value.
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* Get the enableMultipleWriteLocations property: Enables the account to write in multiple locations.
*
* @return the enableMultipleWriteLocations value.
*/
public Boolean enableMultipleWriteLocations() {
return this.enableMultipleWriteLocations;
}
/**
* Set the enableMultipleWriteLocations property: Enables the account to write in multiple locations.
*
* @param enableMultipleWriteLocations the enableMultipleWriteLocations value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withEnableMultipleWriteLocations(Boolean enableMultipleWriteLocations) {
this.enableMultipleWriteLocations = enableMultipleWriteLocations;
return this;
}
/**
* Get the enableCassandraConnector property: Enables the cassandra connector on the Cosmos DB C* account.
*
* @return the enableCassandraConnector value.
*/
public Boolean enableCassandraConnector() {
return this.enableCassandraConnector;
}
/**
* Set the enableCassandraConnector property: Enables the cassandra connector on the Cosmos DB C* account.
*
* @param enableCassandraConnector the enableCassandraConnector value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withEnableCassandraConnector(Boolean enableCassandraConnector) {
this.enableCassandraConnector = enableCassandraConnector;
return this;
}
/**
* Get the connectorOffer property: The cassandra connector offer type for the Cosmos DB database C* account.
*
* @return the connectorOffer value.
*/
public ConnectorOffer connectorOffer() {
return this.connectorOffer;
}
/**
* Set the connectorOffer property: The cassandra connector offer type for the Cosmos DB database C* account.
*
* @param connectorOffer the connectorOffer value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withConnectorOffer(ConnectorOffer connectorOffer) {
this.connectorOffer = connectorOffer;
return this;
}
/**
* Get the disableKeyBasedMetadataWriteAccess property: Disable write operations on metadata resources (databases,
* containers, throughput) via account keys.
*
* @return the disableKeyBasedMetadataWriteAccess value.
*/
public Boolean disableKeyBasedMetadataWriteAccess() {
return this.disableKeyBasedMetadataWriteAccess;
}
/**
* Set the disableKeyBasedMetadataWriteAccess property: Disable write operations on metadata resources (databases,
* containers, throughput) via account keys.
*
* @param disableKeyBasedMetadataWriteAccess the disableKeyBasedMetadataWriteAccess value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties
withDisableKeyBasedMetadataWriteAccess(Boolean disableKeyBasedMetadataWriteAccess) {
this.disableKeyBasedMetadataWriteAccess = disableKeyBasedMetadataWriteAccess;
return this;
}
/**
* Get the keyVaultKeyUri property: The URI of the key vault.
*
* @return the keyVaultKeyUri value.
*/
public String keyVaultKeyUri() {
return this.keyVaultKeyUri;
}
/**
* Set the keyVaultKeyUri property: The URI of the key vault.
*
* @param keyVaultKeyUri the keyVaultKeyUri value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withKeyVaultKeyUri(String keyVaultKeyUri) {
this.keyVaultKeyUri = keyVaultKeyUri;
return this;
}
/**
* Get the defaultIdentity property: The default identity for accessing key vault used in features like customer
* managed keys. The default identity needs to be explicitly set by the users. It can be "FirstPartyIdentity",
* "SystemAssignedIdentity" and more.
*
* @return the defaultIdentity value.
*/
public String defaultIdentity() {
return this.defaultIdentity;
}
/**
* Set the defaultIdentity property: The default identity for accessing key vault used in features like customer
* managed keys. The default identity needs to be explicitly set by the users. It can be "FirstPartyIdentity",
* "SystemAssignedIdentity" and more.
*
* @param defaultIdentity the defaultIdentity value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withDefaultIdentity(String defaultIdentity) {
this.defaultIdentity = defaultIdentity;
return this;
}
/**
* Get the publicNetworkAccess property: Whether requests from Public Network are allowed.
*
* @return the publicNetworkAccess value.
*/
public PublicNetworkAccess publicNetworkAccess() {
return this.publicNetworkAccess;
}
/**
* Set the publicNetworkAccess property: Whether requests from Public Network are allowed.
*
* @param publicNetworkAccess the publicNetworkAccess value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withPublicNetworkAccess(PublicNetworkAccess publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
/**
* Get the enableFreeTier property: Flag to indicate whether Free Tier is enabled.
*
* @return the enableFreeTier value.
*/
public Boolean enableFreeTier() {
return this.enableFreeTier;
}
/**
* Set the enableFreeTier property: Flag to indicate whether Free Tier is enabled.
*
* @param enableFreeTier the enableFreeTier value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withEnableFreeTier(Boolean enableFreeTier) {
this.enableFreeTier = enableFreeTier;
return this;
}
/**
* Get the apiProperties property: API specific properties.
*
* @return the apiProperties value.
*/
public ApiProperties apiProperties() {
return this.apiProperties;
}
/**
* Set the apiProperties property: API specific properties.
*
* @param apiProperties the apiProperties value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withApiProperties(ApiProperties apiProperties) {
this.apiProperties = apiProperties;
return this;
}
/**
* Get the enableAnalyticalStorage property: Flag to indicate whether to enable storage analytics.
*
* @return the enableAnalyticalStorage value.
*/
public Boolean enableAnalyticalStorage() {
return this.enableAnalyticalStorage;
}
/**
* Set the enableAnalyticalStorage property: Flag to indicate whether to enable storage analytics.
*
* @param enableAnalyticalStorage the enableAnalyticalStorage value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withEnableAnalyticalStorage(Boolean enableAnalyticalStorage) {
this.enableAnalyticalStorage = enableAnalyticalStorage;
return this;
}
/**
* Get the analyticalStorageConfiguration property: Analytical storage specific properties.
*
* @return the analyticalStorageConfiguration value.
*/
public AnalyticalStorageConfiguration analyticalStorageConfiguration() {
return this.analyticalStorageConfiguration;
}
/**
* Set the analyticalStorageConfiguration property: Analytical storage specific properties.
*
* @param analyticalStorageConfiguration the analyticalStorageConfiguration value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties
withAnalyticalStorageConfiguration(AnalyticalStorageConfiguration analyticalStorageConfiguration) {
this.analyticalStorageConfiguration = analyticalStorageConfiguration;
return this;
}
/**
* Get the instanceId property: A unique identifier assigned to the database account.
*
* @return the instanceId value.
*/
public String instanceId() {
return this.instanceId;
}
/**
* Get the createMode property: Enum to indicate the mode of account creation.
*
* @return the createMode value.
*/
public CreateMode createMode() {
return this.createMode;
}
/**
* Set the createMode property: Enum to indicate the mode of account creation.
*
* @param createMode the createMode value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withCreateMode(CreateMode createMode) {
this.createMode = createMode;
return this;
}
/**
* Get the restoreParameters property: Parameters to indicate the information about the restore.
*
* @return the restoreParameters value.
*/
public RestoreParameters restoreParameters() {
return this.restoreParameters;
}
/**
* Set the restoreParameters property: Parameters to indicate the information about the restore.
*
* @param restoreParameters the restoreParameters value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withRestoreParameters(RestoreParameters restoreParameters) {
this.restoreParameters = restoreParameters;
return this;
}
/**
* Get the backupPolicy property: The object representing the policy for taking backups on an account.
*
* @return the backupPolicy value.
*/
public BackupPolicy backupPolicy() {
return this.backupPolicy;
}
/**
* Set the backupPolicy property: The object representing the policy for taking backups on an account.
*
* @param backupPolicy the backupPolicy value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withBackupPolicy(BackupPolicy backupPolicy) {
this.backupPolicy = backupPolicy;
return this;
}
/**
* Get the cors property: The CORS policy for the Cosmos DB database account.
*
* @return the cors value.
*/
public List cors() {
return this.cors;
}
/**
* Set the cors property: The CORS policy for the Cosmos DB database account.
*
* @param cors the cors value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withCors(List cors) {
this.cors = cors;
return this;
}
/**
* Get the networkAclBypass property: Indicates what services are allowed to bypass firewall checks.
*
* @return the networkAclBypass value.
*/
public NetworkAclBypass networkAclBypass() {
return this.networkAclBypass;
}
/**
* Set the networkAclBypass property: Indicates what services are allowed to bypass firewall checks.
*
* @param networkAclBypass the networkAclBypass value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withNetworkAclBypass(NetworkAclBypass networkAclBypass) {
this.networkAclBypass = networkAclBypass;
return this;
}
/**
* Get the networkAclBypassResourceIds property: An array that contains the Resource Ids for Network Acl Bypass for
* the Cosmos DB account.
*
* @return the networkAclBypassResourceIds value.
*/
public List networkAclBypassResourceIds() {
return this.networkAclBypassResourceIds;
}
/**
* Set the networkAclBypassResourceIds property: An array that contains the Resource Ids for Network Acl Bypass for
* the Cosmos DB account.
*
* @param networkAclBypassResourceIds the networkAclBypassResourceIds value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withNetworkAclBypassResourceIds(List networkAclBypassResourceIds) {
this.networkAclBypassResourceIds = networkAclBypassResourceIds;
return this;
}
/**
* Get the disableLocalAuth property: Opt-out of local authentication and ensure only MSI and AAD can be used
* exclusively for authentication.
*
* @return the disableLocalAuth value.
*/
public Boolean disableLocalAuth() {
return this.disableLocalAuth;
}
/**
* Set the disableLocalAuth property: Opt-out of local authentication and ensure only MSI and AAD can be used
* exclusively for authentication.
*
* @param disableLocalAuth the disableLocalAuth value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withDisableLocalAuth(Boolean disableLocalAuth) {
this.disableLocalAuth = disableLocalAuth;
return this;
}
/**
* Get the capacity property: The object that represents all properties related to capacity enforcement on an
* account.
*
* @return the capacity value.
*/
public Capacity capacity() {
return this.capacity;
}
/**
* Set the capacity property: The object that represents all properties related to capacity enforcement on an
* account.
*
* @param capacity the capacity value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withCapacity(Capacity capacity) {
this.capacity = capacity;
return this;
}
/**
* Get the keysMetadata property: The object that represents the metadata for the Account Keys of the Cosmos DB
* account.
*
* @return the keysMetadata value.
*/
public DatabaseAccountKeysMetadata keysMetadata() {
return this.keysMetadata;
}
/**
* Get the enablePartitionMerge property: Flag to indicate enabling/disabling of Partition Merge feature on the
* account.
*
* @return the enablePartitionMerge value.
*/
public Boolean enablePartitionMerge() {
return this.enablePartitionMerge;
}
/**
* Set the enablePartitionMerge property: Flag to indicate enabling/disabling of Partition Merge feature on the
* account.
*
* @param enablePartitionMerge the enablePartitionMerge value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withEnablePartitionMerge(Boolean enablePartitionMerge) {
this.enablePartitionMerge = enablePartitionMerge;
return this;
}
/**
* Get the minimalTlsVersion property: Indicates the minimum allowed Tls version. The default value is Tls 1.2.
* Cassandra and Mongo APIs only work with Tls 1.2.
*
* @return the minimalTlsVersion value.
*/
public MinimalTlsVersion minimalTlsVersion() {
return this.minimalTlsVersion;
}
/**
* Set the minimalTlsVersion property: Indicates the minimum allowed Tls version. The default value is Tls 1.2.
* Cassandra and Mongo APIs only work with Tls 1.2.
*
* @param minimalTlsVersion the minimalTlsVersion value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withMinimalTlsVersion(MinimalTlsVersion minimalTlsVersion) {
this.minimalTlsVersion = minimalTlsVersion;
return this;
}
/**
* Get the enableBurstCapacity property: Flag to indicate enabling/disabling of Burst Capacity feature on the
* account.
*
* @return the enableBurstCapacity value.
*/
public Boolean enableBurstCapacity() {
return this.enableBurstCapacity;
}
/**
* Set the enableBurstCapacity property: Flag to indicate enabling/disabling of Burst Capacity feature on the
* account.
*
* @param enableBurstCapacity the enableBurstCapacity value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withEnableBurstCapacity(Boolean enableBurstCapacity) {
this.enableBurstCapacity = enableBurstCapacity;
return this;
}
/**
* Get the customerManagedKeyStatus property: Indicates the status of the Customer Managed Key feature on the
* account. In case there are errors, the property provides troubleshooting guidance.
*
* @return the customerManagedKeyStatus value.
*/
public String customerManagedKeyStatus() {
return this.customerManagedKeyStatus;
}
/**
* Set the customerManagedKeyStatus property: Indicates the status of the Customer Managed Key feature on the
* account. In case there are errors, the property provides troubleshooting guidance.
*
* @param customerManagedKeyStatus the customerManagedKeyStatus value to set.
* @return the DatabaseAccountGetProperties object itself.
*/
public DatabaseAccountGetProperties withCustomerManagedKeyStatus(String customerManagedKeyStatus) {
this.customerManagedKeyStatus = customerManagedKeyStatus;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (ipRules() != null) {
ipRules().forEach(e -> e.validate());
}
if (consistencyPolicy() != null) {
consistencyPolicy().validate();
}
if (capabilities() != null) {
capabilities().forEach(e -> e.validate());
}
if (writeLocations() != null) {
writeLocations().forEach(e -> e.validate());
}
if (readLocations() != null) {
readLocations().forEach(e -> e.validate());
}
if (locations() != null) {
locations().forEach(e -> e.validate());
}
if (failoverPolicies() != null) {
failoverPolicies().forEach(e -> e.validate());
}
if (virtualNetworkRules() != null) {
virtualNetworkRules().forEach(e -> e.validate());
}
if (privateEndpointConnections() != null) {
privateEndpointConnections().forEach(e -> e.validate());
}
if (apiProperties() != null) {
apiProperties().validate();
}
if (analyticalStorageConfiguration() != null) {
analyticalStorageConfiguration().validate();
}
if (restoreParameters() != null) {
restoreParameters().validate();
}
if (backupPolicy() != null) {
backupPolicy().validate();
}
if (cors() != null) {
cors().forEach(e -> e.validate());
}
if (capacity() != null) {
capacity().validate();
}
if (keysMetadata() != null) {
keysMetadata().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeArrayField("ipRules", this.ipRules, (writer, element) -> writer.writeJson(element));
jsonWriter.writeBooleanField("isVirtualNetworkFilterEnabled", this.isVirtualNetworkFilterEnabled);
jsonWriter.writeBooleanField("enableAutomaticFailover", this.enableAutomaticFailover);
jsonWriter.writeJsonField("consistencyPolicy", this.consistencyPolicy);
jsonWriter.writeArrayField("capabilities", this.capabilities, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("virtualNetworkRules", this.virtualNetworkRules,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeBooleanField("enableMultipleWriteLocations", this.enableMultipleWriteLocations);
jsonWriter.writeBooleanField("enableCassandraConnector", this.enableCassandraConnector);
jsonWriter.writeStringField("connectorOffer",
this.connectorOffer == null ? null : this.connectorOffer.toString());
jsonWriter.writeBooleanField("disableKeyBasedMetadataWriteAccess", this.disableKeyBasedMetadataWriteAccess);
jsonWriter.writeStringField("keyVaultKeyUri", this.keyVaultKeyUri);
jsonWriter.writeStringField("defaultIdentity", this.defaultIdentity);
jsonWriter.writeStringField("publicNetworkAccess",
this.publicNetworkAccess == null ? null : this.publicNetworkAccess.toString());
jsonWriter.writeBooleanField("enableFreeTier", this.enableFreeTier);
jsonWriter.writeJsonField("apiProperties", this.apiProperties);
jsonWriter.writeBooleanField("enableAnalyticalStorage", this.enableAnalyticalStorage);
jsonWriter.writeJsonField("analyticalStorageConfiguration", this.analyticalStorageConfiguration);
jsonWriter.writeStringField("createMode", this.createMode == null ? null : this.createMode.toString());
jsonWriter.writeJsonField("restoreParameters", this.restoreParameters);
jsonWriter.writeJsonField("backupPolicy", this.backupPolicy);
jsonWriter.writeArrayField("cors", this.cors, (writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("networkAclBypass",
this.networkAclBypass == null ? null : this.networkAclBypass.toString());
jsonWriter.writeArrayField("networkAclBypassResourceIds", this.networkAclBypassResourceIds,
(writer, element) -> writer.writeString(element));
jsonWriter.writeBooleanField("disableLocalAuth", this.disableLocalAuth);
jsonWriter.writeJsonField("capacity", this.capacity);
jsonWriter.writeBooleanField("enablePartitionMerge", this.enablePartitionMerge);
jsonWriter.writeStringField("minimalTlsVersion",
this.minimalTlsVersion == null ? null : this.minimalTlsVersion.toString());
jsonWriter.writeBooleanField("enableBurstCapacity", this.enableBurstCapacity);
jsonWriter.writeStringField("customerManagedKeyStatus", this.customerManagedKeyStatus);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of DatabaseAccountGetProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of DatabaseAccountGetProperties if the JsonReader was pointing to an instance of it, or null
* if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the DatabaseAccountGetProperties.
*/
public static DatabaseAccountGetProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
DatabaseAccountGetProperties deserializedDatabaseAccountGetProperties = new DatabaseAccountGetProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("provisioningState".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.provisioningState = reader.getString();
} else if ("documentEndpoint".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.documentEndpoint = reader.getString();
} else if ("databaseAccountOfferType".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.databaseAccountOfferType
= DatabaseAccountOfferType.fromString(reader.getString());
} else if ("ipRules".equals(fieldName)) {
List ipRules = reader.readArray(reader1 -> IpAddressOrRange.fromJson(reader1));
deserializedDatabaseAccountGetProperties.ipRules = ipRules;
} else if ("isVirtualNetworkFilterEnabled".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.isVirtualNetworkFilterEnabled
= reader.getNullable(JsonReader::getBoolean);
} else if ("enableAutomaticFailover".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.enableAutomaticFailover
= reader.getNullable(JsonReader::getBoolean);
} else if ("consistencyPolicy".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.consistencyPolicy = ConsistencyPolicy.fromJson(reader);
} else if ("capabilities".equals(fieldName)) {
List capabilities = reader.readArray(reader1 -> Capability.fromJson(reader1));
deserializedDatabaseAccountGetProperties.capabilities = capabilities;
} else if ("writeLocations".equals(fieldName)) {
List writeLocations = reader.readArray(reader1 -> Location.fromJson(reader1));
deserializedDatabaseAccountGetProperties.writeLocations = writeLocations;
} else if ("readLocations".equals(fieldName)) {
List readLocations = reader.readArray(reader1 -> Location.fromJson(reader1));
deserializedDatabaseAccountGetProperties.readLocations = readLocations;
} else if ("locations".equals(fieldName)) {
List locations = reader.readArray(reader1 -> Location.fromJson(reader1));
deserializedDatabaseAccountGetProperties.locations = locations;
} else if ("failoverPolicies".equals(fieldName)) {
List failoverPolicies
= reader.readArray(reader1 -> FailoverPolicy.fromJson(reader1));
deserializedDatabaseAccountGetProperties.failoverPolicies = failoverPolicies;
} else if ("virtualNetworkRules".equals(fieldName)) {
List virtualNetworkRules
= reader.readArray(reader1 -> VirtualNetworkRule.fromJson(reader1));
deserializedDatabaseAccountGetProperties.virtualNetworkRules = virtualNetworkRules;
} else if ("privateEndpointConnections".equals(fieldName)) {
List privateEndpointConnections
= reader.readArray(reader1 -> PrivateEndpointConnectionInner.fromJson(reader1));
deserializedDatabaseAccountGetProperties.privateEndpointConnections = privateEndpointConnections;
} else if ("enableMultipleWriteLocations".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.enableMultipleWriteLocations
= reader.getNullable(JsonReader::getBoolean);
} else if ("enableCassandraConnector".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.enableCassandraConnector
= reader.getNullable(JsonReader::getBoolean);
} else if ("connectorOffer".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.connectorOffer
= ConnectorOffer.fromString(reader.getString());
} else if ("disableKeyBasedMetadataWriteAccess".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.disableKeyBasedMetadataWriteAccess
= reader.getNullable(JsonReader::getBoolean);
} else if ("keyVaultKeyUri".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.keyVaultKeyUri = reader.getString();
} else if ("defaultIdentity".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.defaultIdentity = reader.getString();
} else if ("publicNetworkAccess".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.publicNetworkAccess
= PublicNetworkAccess.fromString(reader.getString());
} else if ("enableFreeTier".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.enableFreeTier
= reader.getNullable(JsonReader::getBoolean);
} else if ("apiProperties".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.apiProperties = ApiProperties.fromJson(reader);
} else if ("enableAnalyticalStorage".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.enableAnalyticalStorage
= reader.getNullable(JsonReader::getBoolean);
} else if ("analyticalStorageConfiguration".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.analyticalStorageConfiguration
= AnalyticalStorageConfiguration.fromJson(reader);
} else if ("instanceId".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.instanceId = reader.getString();
} else if ("createMode".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.createMode = CreateMode.fromString(reader.getString());
} else if ("restoreParameters".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.restoreParameters = RestoreParameters.fromJson(reader);
} else if ("backupPolicy".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.backupPolicy = BackupPolicy.fromJson(reader);
} else if ("cors".equals(fieldName)) {
List cors = reader.readArray(reader1 -> CorsPolicy.fromJson(reader1));
deserializedDatabaseAccountGetProperties.cors = cors;
} else if ("networkAclBypass".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.networkAclBypass
= NetworkAclBypass.fromString(reader.getString());
} else if ("networkAclBypassResourceIds".equals(fieldName)) {
List networkAclBypassResourceIds = reader.readArray(reader1 -> reader1.getString());
deserializedDatabaseAccountGetProperties.networkAclBypassResourceIds = networkAclBypassResourceIds;
} else if ("disableLocalAuth".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.disableLocalAuth
= reader.getNullable(JsonReader::getBoolean);
} else if ("capacity".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.capacity = Capacity.fromJson(reader);
} else if ("keysMetadata".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.keysMetadata
= DatabaseAccountKeysMetadata.fromJson(reader);
} else if ("enablePartitionMerge".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.enablePartitionMerge
= reader.getNullable(JsonReader::getBoolean);
} else if ("minimalTlsVersion".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.minimalTlsVersion
= MinimalTlsVersion.fromString(reader.getString());
} else if ("enableBurstCapacity".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.enableBurstCapacity
= reader.getNullable(JsonReader::getBoolean);
} else if ("customerManagedKeyStatus".equals(fieldName)) {
deserializedDatabaseAccountGetProperties.customerManagedKeyStatus = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedDatabaseAccountGetProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy