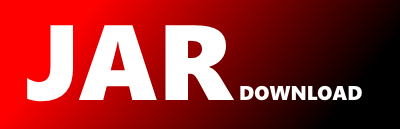
com.azure.resourcemanager.cosmos.implementation.MongoDBResourcesClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-cosmos Show documentation
Show all versions of azure-resourcemanager-cosmos Show documentation
This package contains Microsoft Azure CosmosDB SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.cosmos.implementation;
import com.azure.core.annotation.BodyParam;
import com.azure.core.annotation.Delete;
import com.azure.core.annotation.ExpectedResponses;
import com.azure.core.annotation.Get;
import com.azure.core.annotation.HeaderParam;
import com.azure.core.annotation.Headers;
import com.azure.core.annotation.Host;
import com.azure.core.annotation.HostParam;
import com.azure.core.annotation.PathParam;
import com.azure.core.annotation.Post;
import com.azure.core.annotation.Put;
import com.azure.core.annotation.QueryParam;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceInterface;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.annotation.UnexpectedResponseExceptionType;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.PagedResponse;
import com.azure.core.http.rest.PagedResponseBase;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.RestProxy;
import com.azure.core.management.exception.ManagementException;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.FluxUtil;
import com.azure.core.util.polling.PollerFlux;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.cosmos.fluent.MongoDBResourcesClient;
import com.azure.resourcemanager.cosmos.fluent.models.BackupInformationInner;
import com.azure.resourcemanager.cosmos.fluent.models.MongoDBCollectionGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.MongoDBDatabaseGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.MongoRoleDefinitionGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.MongoUserDefinitionGetResultsInner;
import com.azure.resourcemanager.cosmos.fluent.models.ThroughputSettingsGetResultsInner;
import com.azure.resourcemanager.cosmos.models.ContinuousBackupRestoreLocation;
import com.azure.resourcemanager.cosmos.models.MongoDBCollectionCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.MongoDBCollectionListResult;
import com.azure.resourcemanager.cosmos.models.MongoDBDatabaseCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.MongoDBDatabaseListResult;
import com.azure.resourcemanager.cosmos.models.MongoRoleDefinitionCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.MongoRoleDefinitionListResult;
import com.azure.resourcemanager.cosmos.models.MongoUserDefinitionCreateUpdateParameters;
import com.azure.resourcemanager.cosmos.models.MongoUserDefinitionListResult;
import com.azure.resourcemanager.cosmos.models.ThroughputSettingsUpdateParameters;
import java.nio.ByteBuffer;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* An instance of this class provides access to all the operations defined in MongoDBResourcesClient.
*/
public final class MongoDBResourcesClientImpl implements MongoDBResourcesClient {
/**
* The proxy service used to perform REST calls.
*/
private final MongoDBResourcesService service;
/**
* The service client containing this operation class.
*/
private final CosmosDBManagementClientImpl client;
/**
* Initializes an instance of MongoDBResourcesClientImpl.
*
* @param client the instance of the service client containing this operation class.
*/
MongoDBResourcesClientImpl(CosmosDBManagementClientImpl client) {
this.service
= RestProxy.create(MongoDBResourcesService.class, client.getHttpPipeline(), client.getSerializerAdapter());
this.client = client;
}
/**
* The interface defining all the services for CosmosDBManagementClientMongoDBResources to be used by the proxy
* service to perform REST calls.
*/
@Host("{$host}")
@ServiceInterface(name = "CosmosDBManagementCl")
public interface MongoDBResourcesService {
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listMongoDBDatabases(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getMongoDBDatabase(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Put("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> createUpdateMongoDBDatabase(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @QueryParam("api-version") String apiVersion,
@BodyParam("application/json") MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Accept: application/json;q=0.9", "Content-Type: application/json" })
@Delete("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}")
@ExpectedResponses({ 202, 204 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> deleteMongoDBDatabase(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @QueryParam("api-version") String apiVersion,
Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/throughputSettings/default")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getMongoDBDatabaseThroughput(
@HostParam("$host") String endpoint, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Put("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/throughputSettings/default")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> updateMongoDBDatabaseThroughput(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @QueryParam("api-version") String apiVersion,
@BodyParam("application/json") ThroughputSettingsUpdateParameters updateThroughputParameters,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/throughputSettings/default/migrateToAutoscale")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> migrateMongoDBDatabaseToAutoscale(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/throughputSettings/default/migrateToManualThroughput")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> migrateMongoDBDatabaseToManualThroughput(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listMongoDBCollections(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getMongoDBCollection(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @PathParam("collectionName") String collectionName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Put("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> createUpdateMongoDBCollection(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @PathParam("collectionName") String collectionName,
@QueryParam("api-version") String apiVersion,
@BodyParam("application/json") MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Accept: application/json;q=0.9", "Content-Type: application/json" })
@Delete("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}")
@ExpectedResponses({ 202, 204 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> deleteMongoDBCollection(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @PathParam("collectionName") String collectionName,
@QueryParam("api-version") String apiVersion, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}/throughputSettings/default")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getMongoDBCollectionThroughput(
@HostParam("$host") String endpoint, @PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @PathParam("collectionName") String collectionName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Put("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}/throughputSettings/default")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> updateMongoDBCollectionThroughput(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @PathParam("collectionName") String collectionName,
@QueryParam("api-version") String apiVersion,
@BodyParam("application/json") ThroughputSettingsUpdateParameters updateThroughputParameters,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}/throughputSettings/default/migrateToAutoscale")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> migrateMongoDBCollectionToAutoscale(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @PathParam("collectionName") String collectionName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}/throughputSettings/default/migrateToManualThroughput")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> migrateMongoDBCollectionToManualThroughput(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @PathParam("collectionName") String collectionName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbRoleDefinitions/{mongoRoleDefinitionId}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getMongoRoleDefinition(@HostParam("$host") String endpoint,
@PathParam("mongoRoleDefinitionId") String mongoRoleDefinitionId,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Put("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbRoleDefinitions/{mongoRoleDefinitionId}")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> createUpdateMongoRoleDefinition(@HostParam("$host") String endpoint,
@PathParam("mongoRoleDefinitionId") String mongoRoleDefinitionId,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@QueryParam("api-version") String apiVersion,
@BodyParam("application/json") MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Delete("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbRoleDefinitions/{mongoRoleDefinitionId}")
@ExpectedResponses({ 200, 202, 204 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> deleteMongoRoleDefinition(@HostParam("$host") String endpoint,
@PathParam("mongoRoleDefinitionId") String mongoRoleDefinitionId,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbRoleDefinitions")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listMongoRoleDefinitions(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbUserDefinitions/{mongoUserDefinitionId}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> getMongoUserDefinition(@HostParam("$host") String endpoint,
@PathParam("mongoUserDefinitionId") String mongoUserDefinitionId,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Put("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbUserDefinitions/{mongoUserDefinitionId}")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> createUpdateMongoUserDefinition(@HostParam("$host") String endpoint,
@PathParam("mongoUserDefinitionId") String mongoUserDefinitionId,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@QueryParam("api-version") String apiVersion,
@BodyParam("application/json") MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Delete("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbUserDefinitions/{mongoUserDefinitionId}")
@ExpectedResponses({ 200, 202, 204 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> deleteMongoUserDefinition(@HostParam("$host") String endpoint,
@PathParam("mongoUserDefinitionId") String mongoUserDefinitionId,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbUserDefinitions")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listMongoUserDefinitions(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Post("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DocumentDB/databaseAccounts/{accountName}/mongodbDatabases/{databaseName}/collections/{collectionName}/retrieveContinuousBackupInformation")
@ExpectedResponses({ 200, 202 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono>> retrieveContinuousBackupInformation(@HostParam("$host") String endpoint,
@PathParam("subscriptionId") String subscriptionId,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("accountName") String accountName,
@PathParam("databaseName") String databaseName, @PathParam("collectionName") String collectionName,
@QueryParam("api-version") String apiVersion,
@BodyParam("application/json") ContinuousBackupRestoreLocation location,
@HeaderParam("Accept") String accept, Context context);
}
/**
* Lists the MongoDB databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB databases and their properties along with
* {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>
listMongoDBDatabasesSinglePageAsync(String resourceGroupName, String accountName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.listMongoDBDatabases(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, this.client.getApiVersion(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), null, null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Lists the MongoDB databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB databases and their properties along with
* {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>
listMongoDBDatabasesSinglePageAsync(String resourceGroupName, String accountName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listMongoDBDatabases(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, this.client.getApiVersion(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), null, null));
}
/**
* Lists the MongoDB databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB databases and their properties as paginated
* response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listMongoDBDatabasesAsync(String resourceGroupName,
String accountName) {
return new PagedFlux<>(() -> listMongoDBDatabasesSinglePageAsync(resourceGroupName, accountName));
}
/**
* Lists the MongoDB databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB databases and their properties as paginated
* response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listMongoDBDatabasesAsync(String resourceGroupName,
String accountName, Context context) {
return new PagedFlux<>(() -> listMongoDBDatabasesSinglePageAsync(resourceGroupName, accountName, context));
}
/**
* Lists the MongoDB databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB databases and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listMongoDBDatabases(String resourceGroupName,
String accountName) {
return new PagedIterable<>(listMongoDBDatabasesAsync(resourceGroupName, accountName));
}
/**
* Lists the MongoDB databases under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB databases and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listMongoDBDatabases(String resourceGroupName,
String accountName, Context context) {
return new PagedIterable<>(listMongoDBDatabasesAsync(resourceGroupName, accountName, context));
}
/**
* Gets the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB databases under an existing Azure Cosmos DB database account with the provided name along
* with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getMongoDBDatabaseWithResponseAsync(String resourceGroupName,
String accountName, String databaseName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.getMongoDBDatabase(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Gets the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB databases under an existing Azure Cosmos DB database account with the provided name along
* with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getMongoDBDatabaseWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getMongoDBDatabase(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, databaseName, this.client.getApiVersion(), accept, context);
}
/**
* Gets the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB databases under an existing Azure Cosmos DB database account with the provided name on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getMongoDBDatabaseAsync(String resourceGroupName, String accountName,
String databaseName) {
return getMongoDBDatabaseWithResponseAsync(resourceGroupName, accountName, databaseName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Gets the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB databases under an existing Azure Cosmos DB database account with the provided name along
* with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getMongoDBDatabaseWithResponse(String resourceGroupName,
String accountName, String databaseName, Context context) {
return getMongoDBDatabaseWithResponseAsync(resourceGroupName, accountName, databaseName, context).block();
}
/**
* Gets the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB databases under an existing Azure Cosmos DB database account with the provided name.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MongoDBDatabaseGetResultsInner getMongoDBDatabase(String resourceGroupName, String accountName,
String databaseName) {
return getMongoDBDatabaseWithResponse(resourceGroupName, accountName, databaseName, Context.NONE).getValue();
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB database along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> createUpdateMongoDBDatabaseWithResponseAsync(String resourceGroupName,
String accountName, String databaseName,
MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (createUpdateMongoDBDatabaseParameters == null) {
return Mono.error(new IllegalArgumentException(
"Parameter createUpdateMongoDBDatabaseParameters is required and cannot be null."));
} else {
createUpdateMongoDBDatabaseParameters.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.createUpdateMongoDBDatabase(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, databaseName,
this.client.getApiVersion(), createUpdateMongoDBDatabaseParameters, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB database along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> createUpdateMongoDBDatabaseWithResponseAsync(String resourceGroupName,
String accountName, String databaseName,
MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (createUpdateMongoDBDatabaseParameters == null) {
return Mono.error(new IllegalArgumentException(
"Parameter createUpdateMongoDBDatabaseParameters is required and cannot be null."));
} else {
createUpdateMongoDBDatabaseParameters.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.createUpdateMongoDBDatabase(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, this.client.getApiVersion(),
createUpdateMongoDBDatabaseParameters, accept, context);
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB MongoDB database.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, MongoDBDatabaseGetResultsInner>
beginCreateUpdateMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName,
MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters) {
Mono>> mono = createUpdateMongoDBDatabaseWithResponseAsync(resourceGroupName,
accountName, databaseName, createUpdateMongoDBDatabaseParameters);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), MongoDBDatabaseGetResultsInner.class, MongoDBDatabaseGetResultsInner.class,
this.client.getContext());
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB MongoDB database.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, MongoDBDatabaseGetResultsInner>
beginCreateUpdateMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName,
MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters, Context context) {
context = this.client.mergeContext(context);
Mono>> mono = createUpdateMongoDBDatabaseWithResponseAsync(resourceGroupName,
accountName, databaseName, createUpdateMongoDBDatabaseParameters, context);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), MongoDBDatabaseGetResultsInner.class, MongoDBDatabaseGetResultsInner.class,
context);
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB MongoDB database.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, MongoDBDatabaseGetResultsInner>
beginCreateUpdateMongoDBDatabase(String resourceGroupName, String accountName, String databaseName,
MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters) {
return this
.beginCreateUpdateMongoDBDatabaseAsync(resourceGroupName, accountName, databaseName,
createUpdateMongoDBDatabaseParameters)
.getSyncPoller();
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB MongoDB database.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, MongoDBDatabaseGetResultsInner>
beginCreateUpdateMongoDBDatabase(String resourceGroupName, String accountName, String databaseName,
MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters, Context context) {
return this
.beginCreateUpdateMongoDBDatabaseAsync(resourceGroupName, accountName, databaseName,
createUpdateMongoDBDatabaseParameters, context)
.getSyncPoller();
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB database on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono createUpdateMongoDBDatabaseAsync(String resourceGroupName,
String accountName, String databaseName,
MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters) {
return beginCreateUpdateMongoDBDatabaseAsync(resourceGroupName, accountName, databaseName,
createUpdateMongoDBDatabaseParameters).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB database on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono createUpdateMongoDBDatabaseAsync(String resourceGroupName,
String accountName, String databaseName,
MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters, Context context) {
return beginCreateUpdateMongoDBDatabaseAsync(resourceGroupName, accountName, databaseName,
createUpdateMongoDBDatabaseParameters, context).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB database.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MongoDBDatabaseGetResultsInner createUpdateMongoDBDatabase(String resourceGroupName, String accountName,
String databaseName, MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters) {
return createUpdateMongoDBDatabaseAsync(resourceGroupName, accountName, databaseName,
createUpdateMongoDBDatabaseParameters).block();
}
/**
* Create or updates Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param createUpdateMongoDBDatabaseParameters The parameters to provide for the current MongoDB database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB database.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MongoDBDatabaseGetResultsInner createUpdateMongoDBDatabase(String resourceGroupName, String accountName,
String databaseName, MongoDBDatabaseCreateUpdateParameters createUpdateMongoDBDatabaseParameters,
Context context) {
return createUpdateMongoDBDatabaseAsync(resourceGroupName, accountName, databaseName,
createUpdateMongoDBDatabaseParameters, context).block();
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> deleteMongoDBDatabaseWithResponseAsync(String resourceGroupName,
String accountName, String databaseName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
return FluxUtil
.withContext(
context -> service.deleteMongoDBDatabase(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, this.client.getApiVersion(), context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> deleteMongoDBDatabaseWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
context = this.client.mergeContext(context);
return service.deleteMongoDBDatabase(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, this.client.getApiVersion(), context);
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginDeleteMongoDBDatabaseAsync(String resourceGroupName,
String accountName, String databaseName) {
Mono>> mono
= deleteMongoDBDatabaseWithResponseAsync(resourceGroupName, accountName, databaseName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeleteMongoDBDatabaseAsync(String resourceGroupName,
String accountName, String databaseName, Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= deleteMongoDBDatabaseWithResponseAsync(resourceGroupName, accountName, databaseName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDeleteMongoDBDatabase(String resourceGroupName, String accountName,
String databaseName) {
return this.beginDeleteMongoDBDatabaseAsync(resourceGroupName, accountName, databaseName).getSyncPoller();
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDeleteMongoDBDatabase(String resourceGroupName, String accountName,
String databaseName, Context context) {
return this.beginDeleteMongoDBDatabaseAsync(resourceGroupName, accountName, databaseName, context)
.getSyncPoller();
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName) {
return beginDeleteMongoDBDatabaseAsync(resourceGroupName, accountName, databaseName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deleteMongoDBDatabaseAsync(String resourceGroupName, String accountName, String databaseName,
Context context) {
return beginDeleteMongoDBDatabaseAsync(resourceGroupName, accountName, databaseName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteMongoDBDatabase(String resourceGroupName, String accountName, String databaseName) {
deleteMongoDBDatabaseAsync(resourceGroupName, accountName, databaseName).block();
}
/**
* Deletes an existing Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteMongoDBDatabase(String resourceGroupName, String accountName, String databaseName,
Context context) {
deleteMongoDBDatabaseAsync(resourceGroupName, accountName, databaseName, context).block();
}
/**
* Gets the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getMongoDBDatabaseThroughputWithResponseAsync(
String resourceGroupName, String accountName, String databaseName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getMongoDBDatabaseThroughput(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, databaseName,
this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Gets the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getMongoDBDatabaseThroughputWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getMongoDBDatabaseThroughput(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, this.client.getApiVersion(), accept, context);
}
/**
* Gets the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getMongoDBDatabaseThroughputAsync(String resourceGroupName,
String accountName, String databaseName) {
return getMongoDBDatabaseThroughputWithResponseAsync(resourceGroupName, accountName, databaseName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Gets the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getMongoDBDatabaseThroughputWithResponse(
String resourceGroupName, String accountName, String databaseName, Context context) {
return getMongoDBDatabaseThroughputWithResponseAsync(resourceGroupName, accountName, databaseName, context)
.block();
}
/**
* Gets the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB database under an existing Azure Cosmos DB database account with the
* provided name.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ThroughputSettingsGetResultsInner getMongoDBDatabaseThroughput(String resourceGroupName, String accountName,
String databaseName) {
return getMongoDBDatabaseThroughputWithResponse(resourceGroupName, accountName, databaseName, Context.NONE)
.getValue();
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> updateMongoDBDatabaseThroughputWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (updateThroughputParameters == null) {
return Mono.error(
new IllegalArgumentException("Parameter updateThroughputParameters is required and cannot be null."));
} else {
updateThroughputParameters.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.updateMongoDBDatabaseThroughput(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, databaseName,
this.client.getApiVersion(), updateThroughputParameters, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> updateMongoDBDatabaseThroughputWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters,
Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (updateThroughputParameters == null) {
return Mono.error(
new IllegalArgumentException("Parameter updateThroughputParameters is required and cannot be null."));
} else {
updateThroughputParameters.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.updateMongoDBDatabaseThroughput(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, this.client.getApiVersion(), updateThroughputParameters,
accept, context);
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, ThroughputSettingsGetResultsInner>
beginUpdateMongoDBDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName,
ThroughputSettingsUpdateParameters updateThroughputParameters) {
Mono>> mono = updateMongoDBDatabaseThroughputWithResponseAsync(resourceGroupName,
accountName, databaseName, updateThroughputParameters);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), ThroughputSettingsGetResultsInner.class,
ThroughputSettingsGetResultsInner.class, this.client.getContext());
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, ThroughputSettingsGetResultsInner>
beginUpdateMongoDBDatabaseThroughputAsync(String resourceGroupName, String accountName, String databaseName,
ThroughputSettingsUpdateParameters updateThroughputParameters, Context context) {
context = this.client.mergeContext(context);
Mono>> mono = updateMongoDBDatabaseThroughputWithResponseAsync(resourceGroupName,
accountName, databaseName, updateThroughputParameters, context);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), ThroughputSettingsGetResultsInner.class,
ThroughputSettingsGetResultsInner.class, context);
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateMongoDBDatabaseThroughput(String resourceGroupName, String accountName, String databaseName,
ThroughputSettingsUpdateParameters updateThroughputParameters) {
return this
.beginUpdateMongoDBDatabaseThroughputAsync(resourceGroupName, accountName, databaseName,
updateThroughputParameters)
.getSyncPoller();
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateMongoDBDatabaseThroughput(String resourceGroupName, String accountName, String databaseName,
ThroughputSettingsUpdateParameters updateThroughputParameters, Context context) {
return this
.beginUpdateMongoDBDatabaseThroughputAsync(resourceGroupName, accountName, databaseName,
updateThroughputParameters, context)
.getSyncPoller();
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono updateMongoDBDatabaseThroughputAsync(String resourceGroupName,
String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
return beginUpdateMongoDBDatabaseThroughputAsync(resourceGroupName, accountName, databaseName,
updateThroughputParameters).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono updateMongoDBDatabaseThroughputAsync(String resourceGroupName,
String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters,
Context context) {
return beginUpdateMongoDBDatabaseThroughputAsync(resourceGroupName, accountName, databaseName,
updateThroughputParameters, context).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ThroughputSettingsGetResultsInner updateMongoDBDatabaseThroughput(String resourceGroupName,
String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
return updateMongoDBDatabaseThroughputAsync(resourceGroupName, accountName, databaseName,
updateThroughputParameters).block();
}
/**
* Update RUs per second of the an Azure Cosmos DB MongoDB database.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* database.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ThroughputSettingsGetResultsInner updateMongoDBDatabaseThroughput(String resourceGroupName,
String accountName, String databaseName, ThroughputSettingsUpdateParameters updateThroughputParameters,
Context context) {
return updateMongoDBDatabaseThroughputAsync(resourceGroupName, accountName, databaseName,
updateThroughputParameters, context).block();
}
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> migrateMongoDBDatabaseToAutoscaleWithResponseAsync(String resourceGroupName,
String accountName, String databaseName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.migrateMongoDBDatabaseToAutoscale(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, databaseName,
this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> migrateMongoDBDatabaseToAutoscaleWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.migrateMongoDBDatabaseToAutoscale(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, this.client.getApiVersion(), accept, context);
}
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBDatabaseToAutoscaleAsync(String resourceGroupName, String accountName, String databaseName) {
Mono>> mono
= migrateMongoDBDatabaseToAutoscaleWithResponseAsync(resourceGroupName, accountName, databaseName);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), ThroughputSettingsGetResultsInner.class,
ThroughputSettingsGetResultsInner.class, this.client.getContext());
}
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBDatabaseToAutoscaleAsync(String resourceGroupName, String accountName, String databaseName,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono
= migrateMongoDBDatabaseToAutoscaleWithResponseAsync(resourceGroupName, accountName, databaseName, context);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), ThroughputSettingsGetResultsInner.class,
ThroughputSettingsGetResultsInner.class, context);
}
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBDatabaseToAutoscale(String resourceGroupName, String accountName, String databaseName) {
return this.beginMigrateMongoDBDatabaseToAutoscaleAsync(resourceGroupName, accountName, databaseName)
.getSyncPoller();
}
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBDatabaseToAutoscale(String resourceGroupName, String accountName, String databaseName,
Context context) {
return this.beginMigrateMongoDBDatabaseToAutoscaleAsync(resourceGroupName, accountName, databaseName, context)
.getSyncPoller();
}
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono migrateMongoDBDatabaseToAutoscaleAsync(String resourceGroupName,
String accountName, String databaseName) {
return beginMigrateMongoDBDatabaseToAutoscaleAsync(resourceGroupName, accountName, databaseName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono migrateMongoDBDatabaseToAutoscaleAsync(String resourceGroupName,
String accountName, String databaseName, Context context) {
return beginMigrateMongoDBDatabaseToAutoscaleAsync(resourceGroupName, accountName, databaseName, context).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ThroughputSettingsGetResultsInner migrateMongoDBDatabaseToAutoscale(String resourceGroupName,
String accountName, String databaseName) {
return migrateMongoDBDatabaseToAutoscaleAsync(resourceGroupName, accountName, databaseName).block();
}
/**
* Migrate an Azure Cosmos DB MongoDB database from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ThroughputSettingsGetResultsInner migrateMongoDBDatabaseToAutoscale(String resourceGroupName,
String accountName, String databaseName, Context context) {
return migrateMongoDBDatabaseToAutoscaleAsync(resourceGroupName, accountName, databaseName, context).block();
}
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> migrateMongoDBDatabaseToManualThroughputWithResponseAsync(
String resourceGroupName, String accountName, String databaseName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.migrateMongoDBDatabaseToManualThroughput(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, databaseName,
this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> migrateMongoDBDatabaseToManualThroughputWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.migrateMongoDBDatabaseToManualThroughput(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, databaseName, this.client.getApiVersion(),
accept, context);
}
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBDatabaseToManualThroughputAsync(String resourceGroupName, String accountName,
String databaseName) {
Mono>> mono
= migrateMongoDBDatabaseToManualThroughputWithResponseAsync(resourceGroupName, accountName, databaseName);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), ThroughputSettingsGetResultsInner.class,
ThroughputSettingsGetResultsInner.class, this.client.getContext());
}
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBDatabaseToManualThroughputAsync(String resourceGroupName, String accountName,
String databaseName, Context context) {
context = this.client.mergeContext(context);
Mono>> mono = migrateMongoDBDatabaseToManualThroughputWithResponseAsync(
resourceGroupName, accountName, databaseName, context);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), ThroughputSettingsGetResultsInner.class,
ThroughputSettingsGetResultsInner.class, context);
}
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBDatabaseToManualThroughput(String resourceGroupName, String accountName,
String databaseName) {
return this.beginMigrateMongoDBDatabaseToManualThroughputAsync(resourceGroupName, accountName, databaseName)
.getSyncPoller();
}
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBDatabaseToManualThroughput(String resourceGroupName, String accountName, String databaseName,
Context context) {
return this
.beginMigrateMongoDBDatabaseToManualThroughputAsync(resourceGroupName, accountName, databaseName, context)
.getSyncPoller();
}
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono migrateMongoDBDatabaseToManualThroughputAsync(
String resourceGroupName, String accountName, String databaseName) {
return beginMigrateMongoDBDatabaseToManualThroughputAsync(resourceGroupName, accountName, databaseName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono migrateMongoDBDatabaseToManualThroughputAsync(
String resourceGroupName, String accountName, String databaseName, Context context) {
return beginMigrateMongoDBDatabaseToManualThroughputAsync(resourceGroupName, accountName, databaseName, context)
.last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ThroughputSettingsGetResultsInner migrateMongoDBDatabaseToManualThroughput(String resourceGroupName,
String accountName, String databaseName) {
return migrateMongoDBDatabaseToManualThroughputAsync(resourceGroupName, accountName, databaseName).block();
}
/**
* Migrate an Azure Cosmos DB MongoDB database from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ThroughputSettingsGetResultsInner migrateMongoDBDatabaseToManualThroughput(String resourceGroupName,
String accountName, String databaseName, Context context) {
return migrateMongoDBDatabaseToManualThroughputAsync(resourceGroupName, accountName, databaseName, context)
.block();
}
/**
* Lists the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB collections and their properties along with
* {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>
listMongoDBCollectionsSinglePageAsync(String resourceGroupName, String accountName, String databaseName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.listMongoDBCollections(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, this.client.getApiVersion(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), null, null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Lists the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB collections and their properties along with
* {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listMongoDBCollectionsSinglePageAsync(
String resourceGroupName, String accountName, String databaseName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listMongoDBCollections(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, databaseName, this.client.getApiVersion(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), null, null));
}
/**
* Lists the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB collections and their properties as paginated
* response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listMongoDBCollectionsAsync(String resourceGroupName,
String accountName, String databaseName) {
return new PagedFlux<>(
() -> listMongoDBCollectionsSinglePageAsync(resourceGroupName, accountName, databaseName));
}
/**
* Lists the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB collections and their properties as paginated
* response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listMongoDBCollectionsAsync(String resourceGroupName,
String accountName, String databaseName, Context context) {
return new PagedFlux<>(
() -> listMongoDBCollectionsSinglePageAsync(resourceGroupName, accountName, databaseName, context));
}
/**
* Lists the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB collections and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listMongoDBCollections(String resourceGroupName,
String accountName, String databaseName) {
return new PagedIterable<>(listMongoDBCollectionsAsync(resourceGroupName, accountName, databaseName));
}
/**
* Lists the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List operation response, that contains the MongoDB collections and their properties as paginated
* response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listMongoDBCollections(String resourceGroupName,
String accountName, String databaseName, Context context) {
return new PagedIterable<>(listMongoDBCollectionsAsync(resourceGroupName, accountName, databaseName, context));
}
/**
* Gets the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB collection under an existing Azure Cosmos DB database account along with {@link Response} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getMongoDBCollectionWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getMongoDBCollection(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, databaseName, collectionName,
this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Gets the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB collection under an existing Azure Cosmos DB database account along with {@link Response} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getMongoDBCollectionWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getMongoDBCollection(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, collectionName, this.client.getApiVersion(), accept, context);
}
/**
* Gets the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB collection under an existing Azure Cosmos DB database account on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getMongoDBCollectionAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName) {
return getMongoDBCollectionWithResponseAsync(resourceGroupName, accountName, databaseName, collectionName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Gets the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB collection under an existing Azure Cosmos DB database account along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getMongoDBCollectionWithResponse(String resourceGroupName,
String accountName, String databaseName, String collectionName, Context context) {
return getMongoDBCollectionWithResponseAsync(resourceGroupName, accountName, databaseName, collectionName,
context).block();
}
/**
* Gets the MongoDB collection under an existing Azure Cosmos DB database account.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the MongoDB collection under an existing Azure Cosmos DB database account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MongoDBCollectionGetResultsInner getMongoDBCollection(String resourceGroupName, String accountName,
String databaseName, String collectionName) {
return getMongoDBCollectionWithResponse(resourceGroupName, accountName, databaseName, collectionName,
Context.NONE).getValue();
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB collection along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> createUpdateMongoDBCollectionWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName,
MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
if (createUpdateMongoDBCollectionParameters == null) {
return Mono.error(new IllegalArgumentException(
"Parameter createUpdateMongoDBCollectionParameters is required and cannot be null."));
} else {
createUpdateMongoDBCollectionParameters.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.createUpdateMongoDBCollection(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, databaseName, collectionName,
this.client.getApiVersion(), createUpdateMongoDBCollectionParameters, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB collection along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> createUpdateMongoDBCollectionWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName,
MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
if (createUpdateMongoDBCollectionParameters == null) {
return Mono.error(new IllegalArgumentException(
"Parameter createUpdateMongoDBCollectionParameters is required and cannot be null."));
} else {
createUpdateMongoDBCollectionParameters.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.createUpdateMongoDBCollection(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, collectionName, this.client.getApiVersion(),
createUpdateMongoDBCollectionParameters, accept, context);
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB MongoDB collection.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, MongoDBCollectionGetResultsInner>
beginCreateUpdateMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName,
String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters) {
Mono>> mono = createUpdateMongoDBCollectionWithResponseAsync(resourceGroupName,
accountName, databaseName, collectionName, createUpdateMongoDBCollectionParameters);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), MongoDBCollectionGetResultsInner.class,
MongoDBCollectionGetResultsInner.class, this.client.getContext());
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB MongoDB collection.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, MongoDBCollectionGetResultsInner>
beginCreateUpdateMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName,
String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono = createUpdateMongoDBCollectionWithResponseAsync(resourceGroupName,
accountName, databaseName, collectionName, createUpdateMongoDBCollectionParameters, context);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), MongoDBCollectionGetResultsInner.class,
MongoDBCollectionGetResultsInner.class, context);
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB MongoDB collection.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, MongoDBCollectionGetResultsInner>
beginCreateUpdateMongoDBCollection(String resourceGroupName, String accountName, String databaseName,
String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters) {
return this
.beginCreateUpdateMongoDBCollectionAsync(resourceGroupName, accountName, databaseName, collectionName,
createUpdateMongoDBCollectionParameters)
.getSyncPoller();
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB MongoDB collection.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, MongoDBCollectionGetResultsInner>
beginCreateUpdateMongoDBCollection(String resourceGroupName, String accountName, String databaseName,
String collectionName, MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters,
Context context) {
return this
.beginCreateUpdateMongoDBCollectionAsync(resourceGroupName, accountName, databaseName, collectionName,
createUpdateMongoDBCollectionParameters, context)
.getSyncPoller();
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB collection on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono createUpdateMongoDBCollectionAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName,
MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters) {
return beginCreateUpdateMongoDBCollectionAsync(resourceGroupName, accountName, databaseName, collectionName,
createUpdateMongoDBCollectionParameters).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB collection on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono createUpdateMongoDBCollectionAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName,
MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters, Context context) {
return beginCreateUpdateMongoDBCollectionAsync(resourceGroupName, accountName, databaseName, collectionName,
createUpdateMongoDBCollectionParameters, context).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB collection.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MongoDBCollectionGetResultsInner createUpdateMongoDBCollection(String resourceGroupName, String accountName,
String databaseName, String collectionName,
MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters) {
return createUpdateMongoDBCollectionAsync(resourceGroupName, accountName, databaseName, collectionName,
createUpdateMongoDBCollectionParameters).block();
}
/**
* Create or update an Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param createUpdateMongoDBCollectionParameters The parameters to provide for the current MongoDB Collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB MongoDB collection.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MongoDBCollectionGetResultsInner createUpdateMongoDBCollection(String resourceGroupName, String accountName,
String databaseName, String collectionName,
MongoDBCollectionCreateUpdateParameters createUpdateMongoDBCollectionParameters, Context context) {
return createUpdateMongoDBCollectionAsync(resourceGroupName, accountName, databaseName, collectionName,
createUpdateMongoDBCollectionParameters, context).block();
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> deleteMongoDBCollectionWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
return FluxUtil
.withContext(
context -> service.deleteMongoDBCollection(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, collectionName, this.client.getApiVersion(), context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> deleteMongoDBCollectionWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
context = this.client.mergeContext(context);
return service.deleteMongoDBCollection(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, collectionName, this.client.getApiVersion(), context);
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginDeleteMongoDBCollectionAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName) {
Mono>> mono
= deleteMongoDBCollectionWithResponseAsync(resourceGroupName, accountName, databaseName, collectionName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeleteMongoDBCollectionAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName, Context context) {
context = this.client.mergeContext(context);
Mono>> mono = deleteMongoDBCollectionWithResponseAsync(resourceGroupName, accountName,
databaseName, collectionName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDeleteMongoDBCollection(String resourceGroupName, String accountName,
String databaseName, String collectionName) {
return this.beginDeleteMongoDBCollectionAsync(resourceGroupName, accountName, databaseName, collectionName)
.getSyncPoller();
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDeleteMongoDBCollection(String resourceGroupName, String accountName,
String databaseName, String collectionName, Context context) {
return this
.beginDeleteMongoDBCollectionAsync(resourceGroupName, accountName, databaseName, collectionName, context)
.getSyncPoller();
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName,
String collectionName) {
return beginDeleteMongoDBCollectionAsync(resourceGroupName, accountName, databaseName, collectionName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deleteMongoDBCollectionAsync(String resourceGroupName, String accountName, String databaseName,
String collectionName, Context context) {
return beginDeleteMongoDBCollectionAsync(resourceGroupName, accountName, databaseName, collectionName, context)
.last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteMongoDBCollection(String resourceGroupName, String accountName, String databaseName,
String collectionName) {
deleteMongoDBCollectionAsync(resourceGroupName, accountName, databaseName, collectionName).block();
}
/**
* Deletes an existing Azure Cosmos DB MongoDB Collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteMongoDBCollection(String resourceGroupName, String accountName, String databaseName,
String collectionName, Context context) {
deleteMongoDBCollectionAsync(resourceGroupName, accountName, databaseName, collectionName, context).block();
}
/**
* Gets the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getMongoDBCollectionThroughputWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getMongoDBCollectionThroughput(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, databaseName, collectionName,
this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Gets the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getMongoDBCollectionThroughputWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getMongoDBCollectionThroughput(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, collectionName, this.client.getApiVersion(), accept, context);
}
/**
* Gets the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getMongoDBCollectionThroughputAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName) {
return getMongoDBCollectionThroughputWithResponseAsync(resourceGroupName, accountName, databaseName,
collectionName).flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Gets the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getMongoDBCollectionThroughputWithResponse(
String resourceGroupName, String accountName, String databaseName, String collectionName, Context context) {
return getMongoDBCollectionThroughputWithResponseAsync(resourceGroupName, accountName, databaseName,
collectionName, context).block();
}
/**
* Gets the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the RUs per second of the MongoDB collection under an existing Azure Cosmos DB database account with the
* provided name.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ThroughputSettingsGetResultsInner getMongoDBCollectionThroughput(String resourceGroupName,
String accountName, String databaseName, String collectionName) {
return getMongoDBCollectionThroughputWithResponse(resourceGroupName, accountName, databaseName, collectionName,
Context.NONE).getValue();
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> updateMongoDBCollectionThroughputWithResponseAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName,
ThroughputSettingsUpdateParameters updateThroughputParameters) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
if (updateThroughputParameters == null) {
return Mono.error(
new IllegalArgumentException("Parameter updateThroughputParameters is required and cannot be null."));
} else {
updateThroughputParameters.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.updateMongoDBCollectionThroughput(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, databaseName, collectionName,
this.client.getApiVersion(), updateThroughputParameters, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> updateMongoDBCollectionThroughputWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName,
ThroughputSettingsUpdateParameters updateThroughputParameters, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
if (updateThroughputParameters == null) {
return Mono.error(
new IllegalArgumentException("Parameter updateThroughputParameters is required and cannot be null."));
} else {
updateThroughputParameters.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.updateMongoDBCollectionThroughput(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, collectionName, this.client.getApiVersion(),
updateThroughputParameters, accept, context);
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, ThroughputSettingsGetResultsInner>
beginUpdateMongoDBCollectionThroughputAsync(String resourceGroupName, String accountName, String databaseName,
String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
Mono>> mono = updateMongoDBCollectionThroughputWithResponseAsync(resourceGroupName,
accountName, databaseName, collectionName, updateThroughputParameters);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), ThroughputSettingsGetResultsInner.class,
ThroughputSettingsGetResultsInner.class, this.client.getContext());
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, ThroughputSettingsGetResultsInner>
beginUpdateMongoDBCollectionThroughputAsync(String resourceGroupName, String accountName, String databaseName,
String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters, Context context) {
context = this.client.mergeContext(context);
Mono>> mono = updateMongoDBCollectionThroughputWithResponseAsync(resourceGroupName,
accountName, databaseName, collectionName, updateThroughputParameters, context);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), ThroughputSettingsGetResultsInner.class,
ThroughputSettingsGetResultsInner.class, context);
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateMongoDBCollectionThroughput(String resourceGroupName, String accountName, String databaseName,
String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters) {
return this
.beginUpdateMongoDBCollectionThroughputAsync(resourceGroupName, accountName, databaseName, collectionName,
updateThroughputParameters)
.getSyncPoller();
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, ThroughputSettingsGetResultsInner>
beginUpdateMongoDBCollectionThroughput(String resourceGroupName, String accountName, String databaseName,
String collectionName, ThroughputSettingsUpdateParameters updateThroughputParameters, Context context) {
return this
.beginUpdateMongoDBCollectionThroughputAsync(resourceGroupName, accountName, databaseName, collectionName,
updateThroughputParameters, context)
.getSyncPoller();
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono updateMongoDBCollectionThroughputAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName,
ThroughputSettingsUpdateParameters updateThroughputParameters) {
return beginUpdateMongoDBCollectionThroughputAsync(resourceGroupName, accountName, databaseName, collectionName,
updateThroughputParameters).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono updateMongoDBCollectionThroughputAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName,
ThroughputSettingsUpdateParameters updateThroughputParameters, Context context) {
return beginUpdateMongoDBCollectionThroughputAsync(resourceGroupName, accountName, databaseName, collectionName,
updateThroughputParameters, context).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ThroughputSettingsGetResultsInner updateMongoDBCollectionThroughput(String resourceGroupName,
String accountName, String databaseName, String collectionName,
ThroughputSettingsUpdateParameters updateThroughputParameters) {
return updateMongoDBCollectionThroughputAsync(resourceGroupName, accountName, databaseName, collectionName,
updateThroughputParameters).block();
}
/**
* Update the RUs per second of an Azure Cosmos DB MongoDB collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param updateThroughputParameters The RUs per second of the parameters to provide for the current MongoDB
* collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ThroughputSettingsGetResultsInner updateMongoDBCollectionThroughput(String resourceGroupName,
String accountName, String databaseName, String collectionName,
ThroughputSettingsUpdateParameters updateThroughputParameters, Context context) {
return updateMongoDBCollectionThroughputAsync(resourceGroupName, accountName, databaseName, collectionName,
updateThroughputParameters, context).block();
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> migrateMongoDBCollectionToAutoscaleWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.migrateMongoDBCollectionToAutoscale(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, databaseName, collectionName,
this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> migrateMongoDBCollectionToAutoscaleWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.migrateMongoDBCollectionToAutoscale(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, collectionName, this.client.getApiVersion(), accept, context);
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBCollectionToAutoscaleAsync(String resourceGroupName, String accountName, String databaseName,
String collectionName) {
Mono>> mono = migrateMongoDBCollectionToAutoscaleWithResponseAsync(resourceGroupName,
accountName, databaseName, collectionName);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), ThroughputSettingsGetResultsInner.class,
ThroughputSettingsGetResultsInner.class, this.client.getContext());
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBCollectionToAutoscaleAsync(String resourceGroupName, String accountName, String databaseName,
String collectionName, Context context) {
context = this.client.mergeContext(context);
Mono>> mono = migrateMongoDBCollectionToAutoscaleWithResponseAsync(resourceGroupName,
accountName, databaseName, collectionName, context);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), ThroughputSettingsGetResultsInner.class,
ThroughputSettingsGetResultsInner.class, context);
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBCollectionToAutoscale(String resourceGroupName, String accountName, String databaseName,
String collectionName) {
return this
.beginMigrateMongoDBCollectionToAutoscaleAsync(resourceGroupName, accountName, databaseName, collectionName)
.getSyncPoller();
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBCollectionToAutoscale(String resourceGroupName, String accountName, String databaseName,
String collectionName, Context context) {
return this
.beginMigrateMongoDBCollectionToAutoscaleAsync(resourceGroupName, accountName, databaseName, collectionName,
context)
.getSyncPoller();
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono migrateMongoDBCollectionToAutoscaleAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName) {
return beginMigrateMongoDBCollectionToAutoscaleAsync(resourceGroupName, accountName, databaseName,
collectionName).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono migrateMongoDBCollectionToAutoscaleAsync(String resourceGroupName,
String accountName, String databaseName, String collectionName, Context context) {
return beginMigrateMongoDBCollectionToAutoscaleAsync(resourceGroupName, accountName, databaseName,
collectionName, context).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ThroughputSettingsGetResultsInner migrateMongoDBCollectionToAutoscale(String resourceGroupName,
String accountName, String databaseName, String collectionName) {
return migrateMongoDBCollectionToAutoscaleAsync(resourceGroupName, accountName, databaseName, collectionName)
.block();
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from manual throughput to autoscale.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ThroughputSettingsGetResultsInner migrateMongoDBCollectionToAutoscale(String resourceGroupName,
String accountName, String databaseName, String collectionName, Context context) {
return migrateMongoDBCollectionToAutoscaleAsync(resourceGroupName, accountName, databaseName, collectionName,
context).block();
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> migrateMongoDBCollectionToManualThroughputWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.migrateMongoDBCollectionToManualThroughput(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, databaseName, collectionName,
this.client.getApiVersion(), accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> migrateMongoDBCollectionToManualThroughputWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.migrateMongoDBCollectionToManualThroughput(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, databaseName, collectionName,
this.client.getApiVersion(), accept, context);
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBCollectionToManualThroughputAsync(String resourceGroupName, String accountName,
String databaseName, String collectionName) {
Mono>> mono = migrateMongoDBCollectionToManualThroughputWithResponseAsync(
resourceGroupName, accountName, databaseName, collectionName);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), ThroughputSettingsGetResultsInner.class,
ThroughputSettingsGetResultsInner.class, this.client.getContext());
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBCollectionToManualThroughputAsync(String resourceGroupName, String accountName,
String databaseName, String collectionName, Context context) {
context = this.client.mergeContext(context);
Mono>> mono = migrateMongoDBCollectionToManualThroughputWithResponseAsync(
resourceGroupName, accountName, databaseName, collectionName, context);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), ThroughputSettingsGetResultsInner.class,
ThroughputSettingsGetResultsInner.class, context);
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBCollectionToManualThroughput(String resourceGroupName, String accountName,
String databaseName, String collectionName) {
return this
.beginMigrateMongoDBCollectionToManualThroughputAsync(resourceGroupName, accountName, databaseName,
collectionName)
.getSyncPoller();
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, ThroughputSettingsGetResultsInner>
beginMigrateMongoDBCollectionToManualThroughput(String resourceGroupName, String accountName,
String databaseName, String collectionName, Context context) {
return this
.beginMigrateMongoDBCollectionToManualThroughputAsync(resourceGroupName, accountName, databaseName,
collectionName, context)
.getSyncPoller();
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono migrateMongoDBCollectionToManualThroughputAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName) {
return beginMigrateMongoDBCollectionToManualThroughputAsync(resourceGroupName, accountName, databaseName,
collectionName).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono migrateMongoDBCollectionToManualThroughputAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName, Context context) {
return beginMigrateMongoDBCollectionToManualThroughputAsync(resourceGroupName, accountName, databaseName,
collectionName, context).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ThroughputSettingsGetResultsInner migrateMongoDBCollectionToManualThroughput(String resourceGroupName,
String accountName, String databaseName, String collectionName) {
return migrateMongoDBCollectionToManualThroughputAsync(resourceGroupName, accountName, databaseName,
collectionName).block();
}
/**
* Migrate an Azure Cosmos DB MongoDB collection from autoscale to manual throughput.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB resource throughput.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public ThroughputSettingsGetResultsInner migrateMongoDBCollectionToManualThroughput(String resourceGroupName,
String accountName, String databaseName, String collectionName, Context context) {
return migrateMongoDBCollectionToManualThroughputAsync(resourceGroupName, accountName, databaseName,
collectionName, context).block();
}
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo Role Definition with the given Id.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getMongoRoleDefinitionWithResponseAsync(
String mongoRoleDefinitionId, String resourceGroupName, String accountName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (mongoRoleDefinitionId == null) {
return Mono
.error(new IllegalArgumentException("Parameter mongoRoleDefinitionId is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getMongoRoleDefinition(this.client.getEndpoint(), mongoRoleDefinitionId,
this.client.getSubscriptionId(), resourceGroupName, accountName, this.client.getApiVersion(), accept,
context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo Role Definition with the given Id.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getMongoRoleDefinitionWithResponseAsync(
String mongoRoleDefinitionId, String resourceGroupName, String accountName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (mongoRoleDefinitionId == null) {
return Mono
.error(new IllegalArgumentException("Parameter mongoRoleDefinitionId is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getMongoRoleDefinition(this.client.getEndpoint(), mongoRoleDefinitionId,
this.client.getSubscriptionId(), resourceGroupName, accountName, this.client.getApiVersion(), accept,
context);
}
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo Role Definition with the given Id.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getMongoRoleDefinitionAsync(String mongoRoleDefinitionId,
String resourceGroupName, String accountName) {
return getMongoRoleDefinitionWithResponseAsync(mongoRoleDefinitionId, resourceGroupName, accountName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo Role Definition with the given Id.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getMongoRoleDefinitionWithResponse(String mongoRoleDefinitionId,
String resourceGroupName, String accountName, Context context) {
return getMongoRoleDefinitionWithResponseAsync(mongoRoleDefinitionId, resourceGroupName, accountName, context)
.block();
}
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo Role Definition with the given Id.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MongoRoleDefinitionGetResultsInner getMongoRoleDefinition(String mongoRoleDefinitionId,
String resourceGroupName, String accountName) {
return getMongoRoleDefinitionWithResponse(mongoRoleDefinitionId, resourceGroupName, accountName, Context.NONE)
.getValue();
}
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> createUpdateMongoRoleDefinitionWithResponseAsync(
String mongoRoleDefinitionId, String resourceGroupName, String accountName,
MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (mongoRoleDefinitionId == null) {
return Mono
.error(new IllegalArgumentException("Parameter mongoRoleDefinitionId is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (createUpdateMongoRoleDefinitionParameters == null) {
return Mono.error(new IllegalArgumentException(
"Parameter createUpdateMongoRoleDefinitionParameters is required and cannot be null."));
} else {
createUpdateMongoRoleDefinitionParameters.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.createUpdateMongoRoleDefinition(this.client.getEndpoint(),
mongoRoleDefinitionId, this.client.getSubscriptionId(), resourceGroupName, accountName,
this.client.getApiVersion(), createUpdateMongoRoleDefinitionParameters, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> createUpdateMongoRoleDefinitionWithResponseAsync(
String mongoRoleDefinitionId, String resourceGroupName, String accountName,
MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (mongoRoleDefinitionId == null) {
return Mono
.error(new IllegalArgumentException("Parameter mongoRoleDefinitionId is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (createUpdateMongoRoleDefinitionParameters == null) {
return Mono.error(new IllegalArgumentException(
"Parameter createUpdateMongoRoleDefinitionParameters is required and cannot be null."));
} else {
createUpdateMongoRoleDefinitionParameters.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.createUpdateMongoRoleDefinition(this.client.getEndpoint(), mongoRoleDefinitionId,
this.client.getSubscriptionId(), resourceGroupName, accountName, this.client.getApiVersion(),
createUpdateMongoRoleDefinitionParameters, accept, context);
}
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB Mongo Role Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, MongoRoleDefinitionGetResultsInner>
beginCreateUpdateMongoRoleDefinitionAsync(String mongoRoleDefinitionId, String resourceGroupName,
String accountName, MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters) {
Mono>> mono = createUpdateMongoRoleDefinitionWithResponseAsync(mongoRoleDefinitionId,
resourceGroupName, accountName, createUpdateMongoRoleDefinitionParameters);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), MongoRoleDefinitionGetResultsInner.class,
MongoRoleDefinitionGetResultsInner.class, this.client.getContext());
}
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB Mongo Role Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, MongoRoleDefinitionGetResultsInner>
beginCreateUpdateMongoRoleDefinitionAsync(String mongoRoleDefinitionId, String resourceGroupName,
String accountName, MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono = createUpdateMongoRoleDefinitionWithResponseAsync(mongoRoleDefinitionId,
resourceGroupName, accountName, createUpdateMongoRoleDefinitionParameters, context);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), MongoRoleDefinitionGetResultsInner.class,
MongoRoleDefinitionGetResultsInner.class, context);
}
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB Mongo Role Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, MongoRoleDefinitionGetResultsInner>
beginCreateUpdateMongoRoleDefinition(String mongoRoleDefinitionId, String resourceGroupName, String accountName,
MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters) {
return this
.beginCreateUpdateMongoRoleDefinitionAsync(mongoRoleDefinitionId, resourceGroupName, accountName,
createUpdateMongoRoleDefinitionParameters)
.getSyncPoller();
}
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB Mongo Role Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, MongoRoleDefinitionGetResultsInner>
beginCreateUpdateMongoRoleDefinition(String mongoRoleDefinitionId, String resourceGroupName, String accountName,
MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters, Context context) {
return this
.beginCreateUpdateMongoRoleDefinitionAsync(mongoRoleDefinitionId, resourceGroupName, accountName,
createUpdateMongoRoleDefinitionParameters, context)
.getSyncPoller();
}
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono createUpdateMongoRoleDefinitionAsync(String mongoRoleDefinitionId,
String resourceGroupName, String accountName,
MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters) {
return beginCreateUpdateMongoRoleDefinitionAsync(mongoRoleDefinitionId, resourceGroupName, accountName,
createUpdateMongoRoleDefinitionParameters).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono createUpdateMongoRoleDefinitionAsync(String mongoRoleDefinitionId,
String resourceGroupName, String accountName,
MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters, Context context) {
return beginCreateUpdateMongoRoleDefinitionAsync(mongoRoleDefinitionId, resourceGroupName, accountName,
createUpdateMongoRoleDefinitionParameters, context).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MongoRoleDefinitionGetResultsInner createUpdateMongoRoleDefinition(String mongoRoleDefinitionId,
String resourceGroupName, String accountName,
MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters) {
return createUpdateMongoRoleDefinitionAsync(mongoRoleDefinitionId, resourceGroupName, accountName,
createUpdateMongoRoleDefinitionParameters).block();
}
/**
* Creates or updates an Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoRoleDefinitionParameters The properties required to create or update a Role Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB Mongo Role Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MongoRoleDefinitionGetResultsInner createUpdateMongoRoleDefinition(String mongoRoleDefinitionId,
String resourceGroupName, String accountName,
MongoRoleDefinitionCreateUpdateParameters createUpdateMongoRoleDefinitionParameters, Context context) {
return createUpdateMongoRoleDefinitionAsync(mongoRoleDefinitionId, resourceGroupName, accountName,
createUpdateMongoRoleDefinitionParameters, context).block();
}
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> deleteMongoRoleDefinitionWithResponseAsync(String mongoRoleDefinitionId,
String resourceGroupName, String accountName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (mongoRoleDefinitionId == null) {
return Mono
.error(new IllegalArgumentException("Parameter mongoRoleDefinitionId is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.deleteMongoRoleDefinition(this.client.getEndpoint(), mongoRoleDefinitionId,
this.client.getSubscriptionId(), resourceGroupName, accountName, this.client.getApiVersion(), accept,
context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> deleteMongoRoleDefinitionWithResponseAsync(String mongoRoleDefinitionId,
String resourceGroupName, String accountName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (mongoRoleDefinitionId == null) {
return Mono
.error(new IllegalArgumentException("Parameter mongoRoleDefinitionId is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.deleteMongoRoleDefinition(this.client.getEndpoint(), mongoRoleDefinitionId,
this.client.getSubscriptionId(), resourceGroupName, accountName, this.client.getApiVersion(), accept,
context);
}
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginDeleteMongoRoleDefinitionAsync(String mongoRoleDefinitionId,
String resourceGroupName, String accountName) {
Mono>> mono
= deleteMongoRoleDefinitionWithResponseAsync(mongoRoleDefinitionId, resourceGroupName, accountName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeleteMongoRoleDefinitionAsync(String mongoRoleDefinitionId,
String resourceGroupName, String accountName, Context context) {
context = this.client.mergeContext(context);
Mono>> mono = deleteMongoRoleDefinitionWithResponseAsync(mongoRoleDefinitionId,
resourceGroupName, accountName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDeleteMongoRoleDefinition(String mongoRoleDefinitionId,
String resourceGroupName, String accountName) {
return this.beginDeleteMongoRoleDefinitionAsync(mongoRoleDefinitionId, resourceGroupName, accountName)
.getSyncPoller();
}
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDeleteMongoRoleDefinition(String mongoRoleDefinitionId,
String resourceGroupName, String accountName, Context context) {
return this.beginDeleteMongoRoleDefinitionAsync(mongoRoleDefinitionId, resourceGroupName, accountName, context)
.getSyncPoller();
}
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteMongoRoleDefinitionAsync(String mongoRoleDefinitionId, String resourceGroupName,
String accountName) {
return beginDeleteMongoRoleDefinitionAsync(mongoRoleDefinitionId, resourceGroupName, accountName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deleteMongoRoleDefinitionAsync(String mongoRoleDefinitionId, String resourceGroupName,
String accountName, Context context) {
return beginDeleteMongoRoleDefinitionAsync(mongoRoleDefinitionId, resourceGroupName, accountName, context)
.last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteMongoRoleDefinition(String mongoRoleDefinitionId, String resourceGroupName, String accountName) {
deleteMongoRoleDefinitionAsync(mongoRoleDefinitionId, resourceGroupName, accountName).block();
}
/**
* Deletes an existing Azure Cosmos DB Mongo Role Definition.
*
* @param mongoRoleDefinitionId The ID for the Role Definition {dbName.roleName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteMongoRoleDefinition(String mongoRoleDefinitionId, String resourceGroupName, String accountName,
Context context) {
deleteMongoRoleDefinitionAsync(mongoRoleDefinitionId, resourceGroupName, accountName, context).block();
}
/**
* Retrieves the list of all Azure Cosmos DB Mongo Role Definitions.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Mongo Role Definitions along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>
listMongoRoleDefinitionsSinglePageAsync(String resourceGroupName, String accountName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.listMongoRoleDefinitions(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, this.client.getApiVersion(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), null, null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Retrieves the list of all Azure Cosmos DB Mongo Role Definitions.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Mongo Role Definitions along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>
listMongoRoleDefinitionsSinglePageAsync(String resourceGroupName, String accountName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listMongoRoleDefinitions(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, this.client.getApiVersion(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), null, null));
}
/**
* Retrieves the list of all Azure Cosmos DB Mongo Role Definitions.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Mongo Role Definitions as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listMongoRoleDefinitionsAsync(String resourceGroupName,
String accountName) {
return new PagedFlux<>(() -> listMongoRoleDefinitionsSinglePageAsync(resourceGroupName, accountName));
}
/**
* Retrieves the list of all Azure Cosmos DB Mongo Role Definitions.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Mongo Role Definitions as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listMongoRoleDefinitionsAsync(String resourceGroupName,
String accountName, Context context) {
return new PagedFlux<>(() -> listMongoRoleDefinitionsSinglePageAsync(resourceGroupName, accountName, context));
}
/**
* Retrieves the list of all Azure Cosmos DB Mongo Role Definitions.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Mongo Role Definitions as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listMongoRoleDefinitions(String resourceGroupName,
String accountName) {
return new PagedIterable<>(listMongoRoleDefinitionsAsync(resourceGroupName, accountName));
}
/**
* Retrieves the list of all Azure Cosmos DB Mongo Role Definitions.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant Mongo Role Definitions as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listMongoRoleDefinitions(String resourceGroupName,
String accountName, Context context) {
return new PagedIterable<>(listMongoRoleDefinitionsAsync(resourceGroupName, accountName, context));
}
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo User Definition with the given Id.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getMongoUserDefinitionWithResponseAsync(
String mongoUserDefinitionId, String resourceGroupName, String accountName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (mongoUserDefinitionId == null) {
return Mono
.error(new IllegalArgumentException("Parameter mongoUserDefinitionId is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.getMongoUserDefinition(this.client.getEndpoint(), mongoUserDefinitionId,
this.client.getSubscriptionId(), resourceGroupName, accountName, this.client.getApiVersion(), accept,
context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo User Definition with the given Id.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getMongoUserDefinitionWithResponseAsync(
String mongoUserDefinitionId, String resourceGroupName, String accountName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (mongoUserDefinitionId == null) {
return Mono
.error(new IllegalArgumentException("Parameter mongoUserDefinitionId is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.getMongoUserDefinition(this.client.getEndpoint(), mongoUserDefinitionId,
this.client.getSubscriptionId(), resourceGroupName, accountName, this.client.getApiVersion(), accept,
context);
}
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo User Definition with the given Id.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getMongoUserDefinitionAsync(String mongoUserDefinitionId,
String resourceGroupName, String accountName) {
return getMongoUserDefinitionWithResponseAsync(mongoUserDefinitionId, resourceGroupName, accountName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo User Definition with the given Id.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getMongoUserDefinitionWithResponse(String mongoUserDefinitionId,
String resourceGroupName, String accountName, Context context) {
return getMongoUserDefinitionWithResponseAsync(mongoUserDefinitionId, resourceGroupName, accountName, context)
.block();
}
/**
* Retrieves the properties of an existing Azure Cosmos DB Mongo User Definition with the given Id.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MongoUserDefinitionGetResultsInner getMongoUserDefinition(String mongoUserDefinitionId,
String resourceGroupName, String accountName) {
return getMongoUserDefinitionWithResponse(mongoUserDefinitionId, resourceGroupName, accountName, Context.NONE)
.getValue();
}
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> createUpdateMongoUserDefinitionWithResponseAsync(
String mongoUserDefinitionId, String resourceGroupName, String accountName,
MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (mongoUserDefinitionId == null) {
return Mono
.error(new IllegalArgumentException("Parameter mongoUserDefinitionId is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (createUpdateMongoUserDefinitionParameters == null) {
return Mono.error(new IllegalArgumentException(
"Parameter createUpdateMongoUserDefinitionParameters is required and cannot be null."));
} else {
createUpdateMongoUserDefinitionParameters.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.createUpdateMongoUserDefinition(this.client.getEndpoint(),
mongoUserDefinitionId, this.client.getSubscriptionId(), resourceGroupName, accountName,
this.client.getApiVersion(), createUpdateMongoUserDefinitionParameters, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> createUpdateMongoUserDefinitionWithResponseAsync(
String mongoUserDefinitionId, String resourceGroupName, String accountName,
MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (mongoUserDefinitionId == null) {
return Mono
.error(new IllegalArgumentException("Parameter mongoUserDefinitionId is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (createUpdateMongoUserDefinitionParameters == null) {
return Mono.error(new IllegalArgumentException(
"Parameter createUpdateMongoUserDefinitionParameters is required and cannot be null."));
} else {
createUpdateMongoUserDefinitionParameters.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.createUpdateMongoUserDefinition(this.client.getEndpoint(), mongoUserDefinitionId,
this.client.getSubscriptionId(), resourceGroupName, accountName, this.client.getApiVersion(),
createUpdateMongoUserDefinitionParameters, accept, context);
}
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB User Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, MongoUserDefinitionGetResultsInner>
beginCreateUpdateMongoUserDefinitionAsync(String mongoUserDefinitionId, String resourceGroupName,
String accountName, MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters) {
Mono>> mono = createUpdateMongoUserDefinitionWithResponseAsync(mongoUserDefinitionId,
resourceGroupName, accountName, createUpdateMongoUserDefinitionParameters);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), MongoUserDefinitionGetResultsInner.class,
MongoUserDefinitionGetResultsInner.class, this.client.getContext());
}
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of an Azure Cosmos DB User Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, MongoUserDefinitionGetResultsInner>
beginCreateUpdateMongoUserDefinitionAsync(String mongoUserDefinitionId, String resourceGroupName,
String accountName, MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters,
Context context) {
context = this.client.mergeContext(context);
Mono>> mono = createUpdateMongoUserDefinitionWithResponseAsync(mongoUserDefinitionId,
resourceGroupName, accountName, createUpdateMongoUserDefinitionParameters, context);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), MongoUserDefinitionGetResultsInner.class,
MongoUserDefinitionGetResultsInner.class, context);
}
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB User Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, MongoUserDefinitionGetResultsInner>
beginCreateUpdateMongoUserDefinition(String mongoUserDefinitionId, String resourceGroupName, String accountName,
MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters) {
return this
.beginCreateUpdateMongoUserDefinitionAsync(mongoUserDefinitionId, resourceGroupName, accountName,
createUpdateMongoUserDefinitionParameters)
.getSyncPoller();
}
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of an Azure Cosmos DB User Definition.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, MongoUserDefinitionGetResultsInner>
beginCreateUpdateMongoUserDefinition(String mongoUserDefinitionId, String resourceGroupName, String accountName,
MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters, Context context) {
return this
.beginCreateUpdateMongoUserDefinitionAsync(mongoUserDefinitionId, resourceGroupName, accountName,
createUpdateMongoUserDefinitionParameters, context)
.getSyncPoller();
}
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono createUpdateMongoUserDefinitionAsync(String mongoUserDefinitionId,
String resourceGroupName, String accountName,
MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters) {
return beginCreateUpdateMongoUserDefinitionAsync(mongoUserDefinitionId, resourceGroupName, accountName,
createUpdateMongoUserDefinitionParameters).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono createUpdateMongoUserDefinitionAsync(String mongoUserDefinitionId,
String resourceGroupName, String accountName,
MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters, Context context) {
return beginCreateUpdateMongoUserDefinitionAsync(mongoUserDefinitionId, resourceGroupName, accountName,
createUpdateMongoUserDefinitionParameters, context).last().flatMap(this.client::getLroFinalResultOrError);
}
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MongoUserDefinitionGetResultsInner createUpdateMongoUserDefinition(String mongoUserDefinitionId,
String resourceGroupName, String accountName,
MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters) {
return createUpdateMongoUserDefinitionAsync(mongoUserDefinitionId, resourceGroupName, accountName,
createUpdateMongoUserDefinitionParameters).block();
}
/**
* Creates or updates an Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param createUpdateMongoUserDefinitionParameters The properties required to create or update a User Definition.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return an Azure Cosmos DB User Definition.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public MongoUserDefinitionGetResultsInner createUpdateMongoUserDefinition(String mongoUserDefinitionId,
String resourceGroupName, String accountName,
MongoUserDefinitionCreateUpdateParameters createUpdateMongoUserDefinitionParameters, Context context) {
return createUpdateMongoUserDefinitionAsync(mongoUserDefinitionId, resourceGroupName, accountName,
createUpdateMongoUserDefinitionParameters, context).block();
}
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> deleteMongoUserDefinitionWithResponseAsync(String mongoUserDefinitionId,
String resourceGroupName, String accountName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (mongoUserDefinitionId == null) {
return Mono
.error(new IllegalArgumentException("Parameter mongoUserDefinitionId is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.deleteMongoUserDefinition(this.client.getEndpoint(), mongoUserDefinitionId,
this.client.getSubscriptionId(), resourceGroupName, accountName, this.client.getApiVersion(), accept,
context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> deleteMongoUserDefinitionWithResponseAsync(String mongoUserDefinitionId,
String resourceGroupName, String accountName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (mongoUserDefinitionId == null) {
return Mono
.error(new IllegalArgumentException("Parameter mongoUserDefinitionId is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.deleteMongoUserDefinition(this.client.getEndpoint(), mongoUserDefinitionId,
this.client.getSubscriptionId(), resourceGroupName, accountName, this.client.getApiVersion(), accept,
context);
}
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, Void> beginDeleteMongoUserDefinitionAsync(String mongoUserDefinitionId,
String resourceGroupName, String accountName) {
Mono>> mono
= deleteMongoUserDefinitionWithResponseAsync(mongoUserDefinitionId, resourceGroupName, accountName);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
this.client.getContext());
}
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux, Void> beginDeleteMongoUserDefinitionAsync(String mongoUserDefinitionId,
String resourceGroupName, String accountName, Context context) {
context = this.client.mergeContext(context);
Mono>> mono = deleteMongoUserDefinitionWithResponseAsync(mongoUserDefinitionId,
resourceGroupName, accountName, context);
return this.client.getLroResult(mono, this.client.getHttpPipeline(), Void.class, Void.class,
context);
}
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDeleteMongoUserDefinition(String mongoUserDefinitionId,
String resourceGroupName, String accountName) {
return this.beginDeleteMongoUserDefinitionAsync(mongoUserDefinitionId, resourceGroupName, accountName)
.getSyncPoller();
}
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public SyncPoller, Void> beginDeleteMongoUserDefinition(String mongoUserDefinitionId,
String resourceGroupName, String accountName, Context context) {
return this.beginDeleteMongoUserDefinitionAsync(mongoUserDefinitionId, resourceGroupName, accountName, context)
.getSyncPoller();
}
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteMongoUserDefinitionAsync(String mongoUserDefinitionId, String resourceGroupName,
String accountName) {
return beginDeleteMongoUserDefinitionAsync(mongoUserDefinitionId, resourceGroupName, accountName).last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono deleteMongoUserDefinitionAsync(String mongoUserDefinitionId, String resourceGroupName,
String accountName, Context context) {
return beginDeleteMongoUserDefinitionAsync(mongoUserDefinitionId, resourceGroupName, accountName, context)
.last()
.flatMap(this.client::getLroFinalResultOrError);
}
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteMongoUserDefinition(String mongoUserDefinitionId, String resourceGroupName, String accountName) {
deleteMongoUserDefinitionAsync(mongoUserDefinitionId, resourceGroupName, accountName).block();
}
/**
* Deletes an existing Azure Cosmos DB Mongo User Definition.
*
* @param mongoUserDefinitionId The ID for the User Definition {dbName.userName}.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteMongoUserDefinition(String mongoUserDefinitionId, String resourceGroupName, String accountName,
Context context) {
deleteMongoUserDefinitionAsync(mongoUserDefinitionId, resourceGroupName, accountName, context).block();
}
/**
* Retrieves the list of all Azure Cosmos DB Mongo User Definition.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant User Definition along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>
listMongoUserDefinitionsSinglePageAsync(String resourceGroupName, String accountName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.listMongoUserDefinitions(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, this.client.getApiVersion(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), null, null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Retrieves the list of all Azure Cosmos DB Mongo User Definition.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant User Definition along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>
listMongoUserDefinitionsSinglePageAsync(String resourceGroupName, String accountName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listMongoUserDefinitions(this.client.getEndpoint(), this.client.getSubscriptionId(), resourceGroupName,
accountName, this.client.getApiVersion(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), null, null));
}
/**
* Retrieves the list of all Azure Cosmos DB Mongo User Definition.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant User Definition as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listMongoUserDefinitionsAsync(String resourceGroupName,
String accountName) {
return new PagedFlux<>(() -> listMongoUserDefinitionsSinglePageAsync(resourceGroupName, accountName));
}
/**
* Retrieves the list of all Azure Cosmos DB Mongo User Definition.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant User Definition as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listMongoUserDefinitionsAsync(String resourceGroupName,
String accountName, Context context) {
return new PagedFlux<>(() -> listMongoUserDefinitionsSinglePageAsync(resourceGroupName, accountName, context));
}
/**
* Retrieves the list of all Azure Cosmos DB Mongo User Definition.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant User Definition as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listMongoUserDefinitions(String resourceGroupName,
String accountName) {
return new PagedIterable<>(listMongoUserDefinitionsAsync(resourceGroupName, accountName));
}
/**
* Retrieves the list of all Azure Cosmos DB Mongo User Definition.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the relevant User Definition as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listMongoUserDefinitions(String resourceGroupName,
String accountName, Context context) {
return new PagedIterable<>(listMongoUserDefinitionsAsync(resourceGroupName, accountName, context));
}
/**
* Retrieves continuous backup information for a Mongodb collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param location The name of the continuous backup restore location.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return backup information of a resource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>> retrieveContinuousBackupInformationWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName,
ContinuousBackupRestoreLocation location) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
if (location == null) {
return Mono.error(new IllegalArgumentException("Parameter location is required and cannot be null."));
} else {
location.validate();
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.retrieveContinuousBackupInformation(this.client.getEndpoint(),
this.client.getSubscriptionId(), resourceGroupName, accountName, databaseName, collectionName,
this.client.getApiVersion(), location, accept, context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Retrieves continuous backup information for a Mongodb collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param location The name of the continuous backup restore location.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return backup information of a resource along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono>> retrieveContinuousBackupInformationWithResponseAsync(
String resourceGroupName, String accountName, String databaseName, String collectionName,
ContinuousBackupRestoreLocation location, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (accountName == null) {
return Mono.error(new IllegalArgumentException("Parameter accountName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (collectionName == null) {
return Mono.error(new IllegalArgumentException("Parameter collectionName is required and cannot be null."));
}
if (location == null) {
return Mono.error(new IllegalArgumentException("Parameter location is required and cannot be null."));
} else {
location.validate();
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.retrieveContinuousBackupInformation(this.client.getEndpoint(), this.client.getSubscriptionId(),
resourceGroupName, accountName, databaseName, collectionName, this.client.getApiVersion(), location, accept,
context);
}
/**
* Retrieves continuous backup information for a Mongodb collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param location The name of the continuous backup restore location.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of backup information of a resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
public PollerFlux, BackupInformationInner>
beginRetrieveContinuousBackupInformationAsync(String resourceGroupName, String accountName, String databaseName,
String collectionName, ContinuousBackupRestoreLocation location) {
Mono>> mono = retrieveContinuousBackupInformationWithResponseAsync(resourceGroupName,
accountName, databaseName, collectionName, location);
return this.client.getLroResult(mono,
this.client.getHttpPipeline(), BackupInformationInner.class, BackupInformationInner.class,
this.client.getContext());
}
/**
* Retrieves continuous backup information for a Mongodb collection.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param accountName Cosmos DB database account name.
* @param databaseName Cosmos DB database name.
* @param collectionName Cosmos DB collection name.
* @param location The name of the continuous backup restore location.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of backup information of a resource.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
private PollerFlux