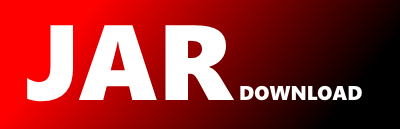
com.azure.resourcemanager.cosmos.implementation.PrivateEndpointConnectionsImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-cosmos Show documentation
Show all versions of azure-resourcemanager-cosmos Show documentation
This package contains Microsoft Azure CosmosDB SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.resourcemanager.cosmos.implementation;
import com.azure.core.http.rest.PagedFlux;
import com.azure.resourcemanager.cosmos.fluent.PrivateEndpointConnectionsClient;
import com.azure.resourcemanager.cosmos.fluent.models.PrivateEndpointConnectionInner;
import com.azure.resourcemanager.cosmos.models.CosmosDBAccount;
import com.azure.resourcemanager.cosmos.models.PrivateEndpointConnection;
import com.azure.resourcemanager.resources.fluentcore.arm.collection.implementation.ExternalChildResourcesCachedImpl;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.azure.resourcemanager.resources.fluentcore.utils.PagedConverter;
/** Represents a private endpoint connection collection. */
class PrivateEndpointConnectionsImpl
extends ExternalChildResourcesCachedImpl<
PrivateEndpointConnectionImpl,
PrivateEndpointConnection,
PrivateEndpointConnectionInner,
CosmosDBAccountImpl,
CosmosDBAccount> {
private final PrivateEndpointConnectionsClient client;
PrivateEndpointConnectionsImpl(PrivateEndpointConnectionsClient client, CosmosDBAccountImpl parent) {
super(parent, parent.taskGroup(), "PrivateEndpointConnection");
this.client = client;
}
public PrivateEndpointConnectionImpl define(String name) {
return this.prepareInlineDefine(name);
}
public PrivateEndpointConnectionImpl update(String name) {
if (this.collection().size() == 0) {
this.cacheCollection();
}
return this.prepareInlineUpdate(name);
}
public void remove(String name) {
if (this.collection().size() == 0) {
this.cacheCollection();
}
this.prepareInlineRemove(name);
}
public Map asMap() {
return asMapAsync().block();
}
public Mono
© 2015 - 2024 Weber Informatics LLC | Privacy Policy